Android App Development Viva Questions
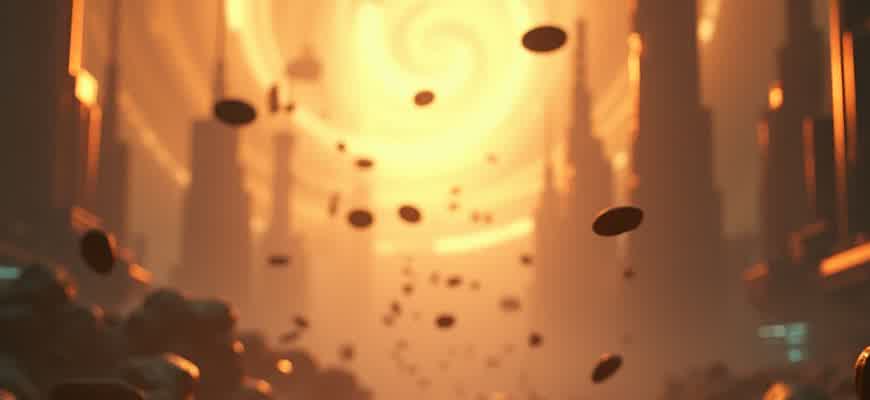
Android application development is a field that requires both theoretical knowledge and practical expertise. During the viva, candidates may be asked a variety of questions covering different aspects of Android programming and design. Below are some of the key areas that are frequently explored during the viva process:
- Core components of Android apps
- Architecture and design patterns
- App lifecycle management
- Integration with third-party libraries
- Handling memory and performance optimization
Below is a table outlining some typical questions you might encounter:
Topic | Sample Question |
---|---|
Activities and Fragments | What is the difference between an Activity and a Fragment? |
Intents | How are Intents used to pass data between components? |
Services | What are the different types of services in Android? |
UI Design | How would you implement a responsive UI in Android? |
Tip: It is crucial to understand both the theoretical and practical aspects of Android development to provide well-rounded answers during the viva.
Core Elements of an Android Application
Android applications are built using several critical components that define their structure and functionality. Understanding these components is essential for effective app development. These components are the building blocks that allow the app to interact with the user, manage data, and respond to system events.
Each Android app is primarily composed of four key components: Activities, Services, Broadcast Receivers, and Content Providers. Below is a breakdown of these components and their roles in Android development.
Key Components Overview
- Activities: An activity represents a single screen with a user interface. Each activity is responsible for interacting with the user, handling input, and displaying data.
- Services: Services are components that run in the background to perform long-running operations without a user interface, such as playing music or downloading files.
- Broadcast Receivers: These components allow the app to listen for system-wide or app-specific events, such as a change in network connectivity or the arrival of a new message.
- Content Providers: Content providers manage access to shared data stored in databases or files, enabling data exchange between different applications.
Detailed Component Breakdown
- Activities: Activities serve as the entry point for user interaction. An app may have multiple activities that are used to navigate between different screens.
- Services: Services do not interact directly with users, but they handle background tasks like fetching data from a server, playing audio, or performing updates while the user is not actively using the app.
- Broadcast Receivers: These components respond to broadcasts from other applications or the Android system itself. For example, an app might register to receive notifications about battery changes or Wi-Fi status updates.
- Content Providers: These components allow apps to access and share data securely. For example, an app might use a content provider to access contacts stored in the system’s database.
Key Differences Between Components
Component | Functionality | User Interaction |
---|---|---|
Activity | Manages UI and user interaction | Yes |
Service | Performs background tasks | No |
Broadcast Receiver | Listens to system-wide events | No |
Content Provider | Shares and manages data | No |
Each component serves a distinct role in building a complete Android application. Understanding their purpose and interaction is crucial for developers aiming to create efficient and responsive apps.
Managing Application Lifecycle and States in Android
In Android, managing the lifecycle and states of an application is essential to ensure smooth user experience and efficient use of resources. The lifecycle of an Android app is determined by a set of system events that dictate how an app transitions between different states. Developers need to understand how to handle these transitions, especially when the app goes in and out of the background, or when system resources are constrained.
Effective management involves responding to specific lifecycle events, such as starting, pausing, or stopping an activity. Each lifecycle stage provides developers with hooks to save data, release resources, or initiate background processes. This allows the app to be resilient to interruptions and continue functioning correctly after being reopened.
Key Android Activity Lifecycle States
- onCreate(): Called when the activity is first created. This is where you initialize resources, setup UI, and handle initial configurations.
- onStart(): Called when the activity becomes visible to the user.
- onResume(): Called when the activity starts interacting with the user. At this point, the activity is in the foreground.
- onPause(): Called when the system is about to start resuming another activity. Use this to pause ongoing operations.
- onStop(): Called when the activity is no longer visible. It’s a good place to release resources like network connections or database handles.
- onRestart(): Called when the activity is coming back to the foreground after being stopped.
- onDestroy(): Called before the activity is destroyed. Use this to perform final cleanup like releasing memory or saving data.
State Management in Android: Techniques and Best Practices
State management is crucial for retaining user data and app settings across activity lifecycle changes. The onSaveInstanceState() method allows you to store UI states before an activity is destroyed. These states can be restored in onRestoreInstanceState() or onCreate() when the activity is recreated.
Tip: Always manage long-running tasks such as network calls or database queries in background threads to avoid blocking the UI thread.
Activity Lifecycle Table
Lifecycle Method | Description |
---|---|
onCreate() | Called when the activity is created. Initialize the UI and resources. |
onStart() | Called when the activity is becoming visible to the user. |
onResume() | Called when the activity is interacting with the user, in the foreground. |
onPause() | Called when another activity is coming to the foreground. Release resources here. |
onStop() | Called when the activity is no longer visible. Can be used for cleanup. |
onRestart() | Called when the activity is coming back to the foreground after being stopped. |
onDestroy() | Called before the activity is destroyed. Perform final cleanup operations. |
Managing Multi-Threading and Asynchronous Operations in Android
Handling concurrent tasks is crucial in Android development to ensure smooth user experience without blocking the main UI thread. Android provides several mechanisms for managing multi-threading and performing tasks asynchronously, such as AsyncTask (deprecated), ExecutorService, and Kotlin Coroutines. Each of these tools serves specific use cases and offers different ways to manage background work while maintaining responsiveness in the app.
In modern Android development, Kotlin Coroutines are widely used as they provide a simpler and more flexible way to handle background operations. They allow tasks to be suspended and resumed without blocking threads, making the code more readable and less error-prone. Below are some common approaches to handle multi-threading and async tasks in Android applications.
Common Techniques for Managing Background Tasks
- AsyncTask (Deprecated): Was used for short tasks on a background thread and updates on the UI thread. It is no longer recommended due to limited functionality and the introduction of more efficient solutions.
- ExecutorService: A higher-level replacement for managing a pool of threads. It's more suitable for executing concurrent tasks with better control and handling of multiple threads.
- Kotlin Coroutines: Provides a more flexible and less error-prone way to handle asynchronous tasks by allowing code to be written in a sequential style while managing background work in a non-blocking way.
Example Using Kotlin Coroutines
Kotlin Coroutines make it easy to manage async tasks. The following example demonstrates a basic usage of coroutines to fetch data in the background and update the UI:
// Launch a coroutine on the main thread GlobalScope.launch(Dispatchers.Main) { // Perform the background task val result = withContext(Dispatchers.IO) { fetchDataFromNetwork() } // Update UI with the result updateUI(result) }
Comparison Table: AsyncTask vs ExecutorService vs Kotlin Coroutines
Feature | AsyncTask | ExecutorService | Kotlin Coroutines |
---|---|---|---|
Usage | Short background tasks | Pool of threads for concurrent tasks | Asynchronous tasks with better control |
UI Thread Updates | Directly updates UI thread | Requires manual handling of UI updates | Supports structured and safe UI updates |
Code Readability | Less readable, error-prone | Can be complex | Highly readable and maintainable |
Performance | Limited for complex tasks | Efficient for large-scale tasks | Optimized for performance with less overhead |
How to Implement UI Design Principles in Android Apps?
Effective UI design is critical to building user-friendly and intuitive Android applications. By following key design principles, developers can ensure their apps are visually appealing and easy to navigate. Understanding how to incorporate these principles into your app can significantly improve user satisfaction and engagement. Below are essential guidelines for implementing UI design in Android development.
The Android platform provides a variety of tools and frameworks that allow developers to create responsive, scalable, and accessible interfaces. Key concepts like consistency, feedback, and simplicity should be prioritized to create an optimal user experience. By focusing on these aspects, developers can enhance usability and ensure that the app meets the needs of its users.
Essential Principles for UI Implementation
- Consistency - Consistent design elements like colors, buttons, and fonts ensure a cohesive user experience across all screens.
- Clarity - Clear, readable text and well-defined icons improve accessibility and ease of use.
- Feedback - Providing visual or auditory feedback on user actions helps to reinforce interaction patterns.
- Simplicity - Keep the interface simple by minimizing the number of actions and elements on the screen.
Designing for Different Screen Sizes
Android devices come in a variety of screen sizes and resolutions, so it’s important to ensure your app's UI adapts accordingly. Utilize responsive layouts, such as ConstraintLayout or LinearLayout, and employ resources like dimension and drawable folders to create scalable UI components.
- Use flexible layouts to handle different screen sizes.
- Optimize image sizes for varying screen densities.
- Test the UI on multiple devices to ensure compatibility.
"A good user interface is not just about how it looks, but also how it works."
Key Considerations for Accessibility
Ensuring accessibility is crucial for providing a seamless experience for all users. Make use of Android's accessibility features, such as content descriptions for images and buttons, as well as contrast and font size adjustments. These improvements will make your app more inclusive, especially for users with disabilities.
Accessibility Feature | Description |
---|---|
Content Descriptions | Provide meaningful descriptions for non-text content like images and buttons. |
Text Size | Allow users to adjust text size based on their preferences. |
Color Contrast | Ensure sufficient contrast between text and background for better readability. |
Understanding the Functionality of Intents and Broadcast Receivers in Android Development
In Android development, managing communication between components within an application and between different applications is a fundamental task. This is where Intents and Broadcast Receivers come into play, providing mechanisms to facilitate inter-component communication and system-wide notifications. They are essential tools for developing responsive and interactive applications.
Intents are used for invoking activities, services, or delivering broadcast messages to interested components. Broadcast Receivers, on the other hand, listen for specific broadcast messages and respond accordingly. Together, they enable Android applications to handle external events, such as changes in network connectivity or battery status, in an efficient manner.
Intents
- Explicit Intents: Used to specify a target component (activity, service, etc.) within the same application or across applications.
- Implicit Intents: Do not specify the target component directly but instead declare the action to be performed. The system finds a suitable component to handle the intent.
- Intent Filters: Define the types of intents that a component can handle, based on action, category, or data.
Broadcast Receivers
- System Broadcasts: Sent by the Android system to notify all registered applications about certain events (e.g., battery low, network connectivity changes).
- Custom Broadcasts: Created by applications to notify other parts of the same or other applications about internal events.
Key Point: Intents and Broadcast Receivers enable seamless interaction within and between applications, providing an efficient mechanism for event-driven programming in Android.
Comparison of Intents and Broadcast Receivers
Feature | Intents | Broadcast Receivers |
---|---|---|
Usage | Communication between components (activities, services, etc.) | Listening and responding to system-wide or custom events |
Scope | Limited to components of the application or specific apps | Wide-reaching, affecting all or specific apps |
Response | Triggers actions, like starting an activity or service | Receives and reacts to broadcast messages |
Managing Database Operations with SQLite and Room in Android
Handling databases effectively is a crucial part of Android app development. SQLite is a lightweight, serverless relational database management system that allows developers to store structured data. Room is an abstraction layer built on top of SQLite, offering a more robust and simplified API for database operations. Room minimizes the complexities of direct SQL queries and provides a more object-oriented approach to data management.
Using SQLite and Room in Android requires setting up data entities, creating database access objects (DAOs), and managing database migrations. SQLite is often utilized directly for applications needing a simple and flexible database solution, while Room simplifies tasks like database creation, data access, and interaction with entities.
SQLite Database Operations
- Create a database: Developers directly use SQLiteDatabase class to create and manage databases.
- CRUD operations: Data insertion, reading, updating, and deletion is achieved through raw SQL queries.
- Database connection: Use SQLiteOpenHelper to manage database versioning and provide an easy way to open and close the database.
Room Database Operations
- Entities: Define data models as @Entity classes.
- DAO: Create interfaces annotated with @Dao to define methods for accessing the database.
- Database: Use @Database annotation to define the Room database class and integrate DAOs.
Note: Room provides built-in support for LiveData and RxJava, which simplifies asynchronous database queries and real-time data updates.
Comparison Table: SQLite vs Room
Feature | SQLite | Room |
---|---|---|
Abstraction Layer | None | Yes, Room provides an abstraction layer |
Querying | Raw SQL Queries | Method calls through DAOs |
Data Models | Custom Java objects | Entities annotated with @Entity |
Support for LiveData | No | Yes, built-in LiveData support |
Types of Android App Architectures
Android app architecture defines the structure of an application, dictating how different components interact and ensuring maintainability, scalability, and ease of testing. A well-structured app architecture provides a framework for organizing code, handling user interactions, and managing data efficiently. In Android development, several architectural patterns are commonly used, each offering distinct advantages depending on the project's needs.
Different architectural patterns can be applied to Android apps to improve development workflows. These include common approaches like Model-View-Controller (MVC), Model-View-ViewModel (MVVM), and Clean Architecture, each focusing on separating concerns, ensuring better scalability, and reducing complexity.
Key Android App Architectures
- Model-View-Controller (MVC) - This classic pattern separates the app into three layers: Model, View, and Controller. The model represents data, the view displays it, and the controller handles the interaction between the two.
- Model-View-ViewModel (MVVM) - MVVM focuses on separating the logic behind the UI (ViewModel) from the UI itself (View). This structure promotes better testability and reusability by allowing UI components to react to data changes automatically.
- Clean Architecture - This approach emphasizes separation of concerns by organizing the code into layers with specific responsibilities. It enables flexibility and scalability, ensuring that the core business logic is independent of frameworks and external services.
- Reactive Architecture - It is based on the principles of reactive programming, where the UI reacts to data changes in real time. It uses frameworks like RxJava or Kotlin Coroutines to handle asynchronous tasks and manage state changes.
Comparison of Architectures
Architecture | Separation of Concerns | Testability | Complexity |
---|---|---|---|
MVC | Low | Moderate | Low |
MVVM | High | High | Moderate |
Clean Architecture | Very High | Very High | High |
Reactive | Moderate | High | Moderate |
Choosing the right architecture depends on factors like app complexity, team expertise, and the need for future scalability. It's crucial to balance between the simplicity of MVC and the flexibility of Clean Architecture based on project requirements.
Optimizing Performance and Debugging Techniques in Android Studio
Efficient performance and effective debugging are critical for the success of Android applications. Android Studio provides various tools and techniques to enhance app speed, reduce latency, and ensure smoother user experiences. Developers need to focus on memory management, layout rendering, and network usage to boost the app’s overall performance.
Additionally, debugging is a crucial part of the development lifecycle. By utilizing Android Studio's built-in features, developers can easily identify and resolve issues during development. It's essential to integrate debugging techniques such as logcat filtering, breakpoints, and performance profiling to diagnose and fix potential bugs.
Performance Optimization Strategies
- Memory Management: Minimize memory consumption by reducing object allocation, optimizing data structures, and managing memory leaks.
- Lazy Loading: Load resources and data only when needed to improve the initial app startup time.
- Efficient Network Calls: Optimize API calls and data fetching by using asynchronous operations and minimizing the frequency of network requests.
- View Hierarchy Optimization: Simplify the view hierarchy to reduce the time spent rendering layouts. Use
ConstraintLayout
instead of nestedLinearLayout
orRelativeLayout
.
Debugging Tools and Techniques
- Logcat: Use logcat to capture and view runtime logs. You can filter log output based on tags or log levels for targeted debugging.
- Breakpoints: Set breakpoints in the code to pause execution and inspect variables, call stacks, and memory state at specific points.
- Profiler: Use the Android Profiler to monitor CPU, memory, and network usage in real time, helping you identify performance bottlenecks.
- StrictMode: Enable
StrictMode
to detect disk and network operations on the main thread, which can lead to ANRs (Application Not Responding errors).
Important Debugging and Optimization Insights
Optimizing your app involves focusing not only on performance during execution but also on the development process. Android Studio’s integrated tools like the Profiler and Logcat make debugging and optimization simpler and more precise. Always remember to test on real devices to identify potential issues that may not appear on emulators.
Useful Tools and Features in Android Studio
Tool | Purpose |
---|---|
Android Profiler | Monitor real-time performance of your app, including CPU, memory, and network usage. |
Layout Inspector | Inspect the current layout of your app to optimize the UI rendering process. |
Heap Dump | Analyze memory usage and locate memory leaks. |