Standard App Templates in Xcode for Ios Development
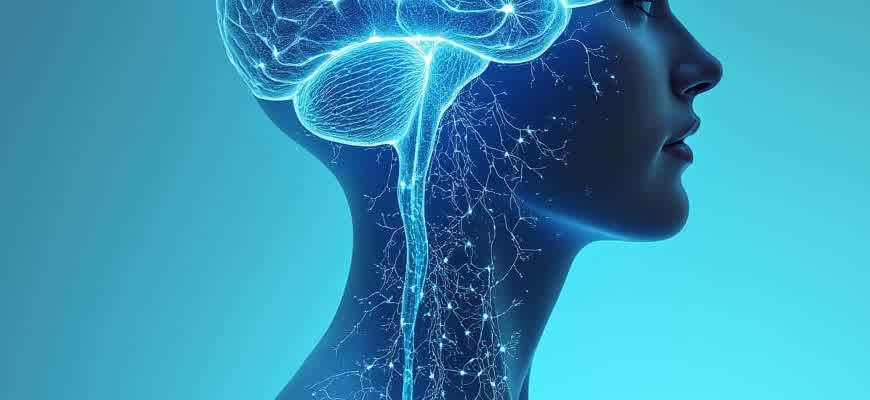
Xcode provides a variety of pre-built application templates that serve as a foundation for iOS development. These templates streamline the development process, enabling developers to focus on functionality rather than starting from scratch. The templates come with pre-configured views, controllers, and some basic functionality tailored to different types of applications.
The most commonly used Xcode templates include:
- Single View App – A minimal template for applications with a single screen interface.
- Tabbed App – A template designed for apps with multiple views, each accessible via a tab bar.
- Master-Detail App – Ideal for apps requiring navigation between a list of items and their details.
- Game – A template for building games with pre-configured assets and functionality.
These templates are not only time-savers but also provide best practices for structuring iOS applications. Below is a summary table of key templates and their use cases:
Template | Use Case |
---|---|
Single View App | Simple apps with one screen and minimal navigation. |
Tabbed App | Multi-screen apps with tab-based navigation. |
Master-Detail App | Apps that involve a list of items and a detailed view for each item. |
Game | For building game apps, with built-in support for sprites, animations, and physics. |
"Using the right template can significantly reduce the time spent on setting up your project and allow you to focus on customizing the core features."
Boost Your iOS Development with Standard App Templates in Xcode
Developing iOS applications can be a time-consuming process, especially when you're starting from scratch with every project. However, Xcode offers a range of pre-built app templates that can significantly speed up your development process. These templates provide a structured foundation, so you can focus more on the functionality and user experience rather than reinventing the wheel. By leveraging these templates, developers can save time and reduce errors, ensuring a more efficient development workflow.
Standard app templates in Xcode are not just time-savers–they also help developers learn best practices by showing how to structure apps according to Apple's guidelines. These templates are ideal for both novice and experienced developers, offering flexibility and scalability. Here's how you can take advantage of them:
Key Benefits of Using Standard Templates
- Faster Development: Templates provide a starting point with built-in components, reducing the need for repetitive tasks.
- Consistency: They follow Apple's design principles, ensuring your app maintains a consistent user experience across devices.
- Built-in Features: Most templates come with commonly used features like navigation, tab bars, and data handling setups, so you don’t have to build these from the ground up.
- Customization Options: You can easily customize templates to fit your unique app requirements, making them flexible for different types of projects.
Popular Templates in Xcode
- Single View App: Best for simple applications with one screen, ideal for small projects or prototypes.
- Tabbed App: Ideal for apps that need a navigation system with multiple tabs for different content sections.
- Master-Detail App: A great choice for apps that involve a list of items with detailed views, perfect for data-heavy applications.
- Game Template: A starting point for building iOS games with essential game development features already set up.
Tip: You can always modify and extend these templates with additional features, third-party libraries, and custom UI components to make the app truly your own.
Template Comparison
Template Type | Use Case | Customization Level |
---|---|---|
Single View App | Simple apps with minimal screens | High |
Tabbed App | Apps with multiple sections and tabs | Medium |
Master-Detail App | Data-driven apps with a master list | High |
Game Template | Basic games with interactive elements | Medium |
How to Begin with Default App Templates in Xcode for iOS Projects
Using the built-in templates in Xcode is an effective way to quickly start developing iOS applications. These templates provide a structured framework, allowing you to focus on custom functionality rather than building everything from scratch. By choosing an appropriate template for your project, you can save time and reduce the complexity of the development process.
Xcode offers various templates for different types of applications, including single-view apps, tab-based apps, and apps with more advanced features. These templates come pre-configured with essential code, layouts, and interfaces, giving you a solid starting point. Understanding how to select and utilize these templates effectively is key to maximizing productivity in iOS development.
Steps to Start a New Project Using Xcode's Templates
- Launch Xcode: Open Xcode and select “Create a new Xcode project” from the startup window.
- Choose a Template: In the template selection window, you'll see different categories like "App", "Game", "Framework", and more. Choose the one that best suits your project’s needs, such as a "Single View App" or "Tab Bar App".
- Configure Project Settings: Set the name of your project, choose a location for it, and select the appropriate language (Swift or Objective-C). Ensure that the options match your development preferences.
- Create Your Project: After configuring the project, click “Create.” This will generate a folder structure and a ready-to-go workspace for your app.
Remember, choosing the right template will streamline your project setup and provide an essential foundation, saving you time on configuration and structure.
Popular Templates Available in Xcode
Template | Description |
---|---|
Single View App | A simple, blank app for basic projects with one view controller. |
Tabbed App | Provides a layout with multiple view controllers, each accessible via a tab bar. |
Master-Detail App | Ideal for apps that display a list of items and detailed content for each selection. |
Game | Optimized for building iOS games using SpriteKit or SceneKit frameworks. |
By choosing the right template, you can streamline your workflow and avoid unnecessary rework. Once you’ve set up your project, you can start customizing the app’s UI and functionality according to your project’s goals.
Choosing the Right Template for Your iOS Application
When starting a new iOS project in Xcode, selecting the appropriate template is crucial to streamline the development process. Templates serve as starting points, providing a pre-configured structure tailored for specific types of applications. Choosing the wrong template can lead to unnecessary complexity or missing functionality, thus delaying development. Therefore, understanding the various available options and aligning them with the project goals is essential.
Xcode offers several templates, each designed for specific types of applications. The choice should depend on the app's requirements, including the desired user interface, functionality, and overall architecture. This decision will impact how the app is structured, the frameworks used, and the resources available from the start.
Key Considerations for Template Selection
- App Type: Is your app a simple utility, a media-based application, or something more complex like a game or a messaging app?
- User Interface Design: Consider whether the app requires a standard view or a more complex layout with multiple screens and navigation features.
- Integration Requirements: Will the app need integration with cloud services, databases, or APIs?
Choosing the wrong template early can lead to unnecessary complexity or missing features, ultimately hindering your app’s development.
Popular Template Options
Template | Best For | Features |
---|---|---|
Single View App | Simple, standalone applications with one screen | Basic structure, minimal navigation, best for prototypes |
Tab Bar App | Apps requiring multiple main sections with tab-based navigation | Pre-configured tab bar and view controllers, suitable for apps with multiple functionalities |
Master-Detail App | Apps with a list of items and detailed views | Split-view controller, ideal for data-driven apps |
Always ensure the chosen template aligns with your app's functionality to minimize rework and ensure a smooth development process.
Customizing Prebuilt UI Components in Xcode Templates
When creating an iOS app using Xcode’s prebuilt templates, the user interface (UI) elements come with a default design. These elements are usually meant to provide a starting point, but they can often be modified to meet the specific needs of your app. Customizing these UI components is a crucial skill for developers to ensure that their app's design aligns with the branding or user experience goals.
To customize the UI components in the standard templates, developers can make changes both visually and programmatically. This includes altering properties of prebuilt elements such as buttons, text fields, and navigation bars. By using Xcode's Interface Builder or modifying the underlying Swift code, developers can tailor these components to reflect unique styles and functionality.
Customizing UI Elements: Key Approaches
- Using Interface Builder: Modify properties like color, size, and layout directly through drag-and-drop interfaces.
- Programming Custom Behavior: Change the functionality of buttons, labels, and other UI elements by altering their corresponding Swift code.
- Custom Styles with UIKit or SwiftUI: Utilize libraries or custom extensions to apply themes or animations to the UI components.
Examples of Customization
- Changing Button Appearance: Alter the button’s background color, font, and corner radius for a unique look.
- Modifying Navigation Bars: Update the title, add custom buttons, or change the background image of navigation bars.
- Adjusting Table View Cells: Customize cell content, style, and behavior depending on user interaction.
Important Considerations
When making customizations, ensure that the UI elements still follow iOS Human Interface Guidelines to maintain a consistent user experience across all devices.
Common Modifications in Standard Templates
Element | Customization Options |
---|---|
Button | Title, font, background color, border radius |
Label | Text size, color, alignment |
Table View Cell | Content, layout, background |
Integrating Core Features Like Navigation and Data Storage in Standard Templates
When working with standard templates in Xcode, it is crucial to understand how essential features like navigation and data management are integrated. These features lay the foundation for building functional and scalable applications. Each template provides a starting point for managing user flow and data persistence, allowing developers to focus on creating unique user experiences rather than building these features from scratch.
The most common templates in Xcode, such as "Single View App" and "Master-Detail App," come with pre-configured setups that handle basic navigation and data storage. These templates offer built-in support for navigation controllers, table views, and local databases like Core Data. This integration streamlines the development process by providing reusable code structures that can be easily customized to fit project requirements.
Navigation Integration
Navigation is one of the key aspects of most mobile apps. Xcode provides several navigation controllers within its templates to manage the flow between different screens. Here’s a quick overview of how navigation is generally handled in standard templates:
- Navigation Controller: It is used to push and pop view controllers in a stack-based approach, making it easier to manage transitions.
- Tab Bar Controller: This is commonly seen in apps requiring quick access to multiple sections through a bottom navigation bar.
- Modal Presentation: For displaying content over the current screen without leaving the current navigation stack.
Each of these controllers is pre-configured in most templates, and developers can customize them by adding additional view controllers or modifying their behavior.
Data Storage Integration
Data management is another critical feature integrated into Xcode's standard templates. The most popular way to handle persistent data in iOS apps is by using Core Data, which is embedded into templates like the "Master-Detail App." Core Data provides an object-oriented interface to interact with a SQLite database, allowing developers to manage the app’s data model efficiently.
Here’s a breakdown of how data storage is handled in these templates:
- Core Data Model: Templates include a pre-defined data model file, which contains entities and attributes that define the structure of the app's data.
- NSFetchedResultsController: It is often used to fetch and manage data, keeping the user interface in sync with changes in the data.
- Data Persistence: Changes to the data are automatically saved in the underlying SQLite database, ensuring that user data is retained between app launches.
Core Data integration simplifies working with persistent data, reducing the need to manually handle storage and retrieval operations.
In conclusion, understanding the integration of navigation and data storage in Xcode templates is essential for developers looking to build efficient and scalable iOS applications. These features allow you to focus on customizing the user experience, while the underlying framework handles the heavy lifting.
Optimizing App Performance with Xcode’s Built-in Tools and Templates
When developing an iOS app, ensuring optimal performance is crucial for delivering a smooth user experience. Xcode, Apple's integrated development environment, offers several tools and templates to help developers enhance app performance from the start. These resources allow for efficient debugging, profiling, and fine-tuning of your application.
Utilizing Xcode’s built-in tools not only aids in identifying bottlenecks but also provides a streamlined approach to maintain high performance as your app grows. By integrating these tools with standardized app templates, you can ensure your app remains responsive and efficient throughout the development lifecycle.
Key Tools and Techniques for Performance Optimization
- Instruments: A powerful suite for profiling your app's performance, helping you analyze memory usage, CPU load, and network activity.
- Activity Monitor: Track real-time performance metrics to spot issues like excessive memory consumption or CPU spikes.
- Leaks and Allocations: Detect memory leaks and ensure efficient memory management by tracking allocations and deallocations over time.
- Energy Log: Optimize your app’s power usage by identifying functions or tasks that consume excessive energy.
Effective Use of Xcode Templates
Xcode provides various app templates designed to set up a project with optimized performance in mind. Using these templates can save time and effort when starting a new project, ensuring that best practices are followed from the outset.
- Single View Application: Ideal for simple apps, it minimizes initial overhead and provides a clean slate for adding performance-enhancing features.
- Master-Detail Application: This template is great for apps that require efficient data handling and supports navigation controllers, which help in reducing memory consumption.
- Tab Bar Application: Best suited for apps that require multiple sections or features, it allows for optimized layout handling and memory management across tabs.
By using these templates, you can leverage pre-configured settings and components that follow Apple’s best practices, ensuring your app is built on a strong performance foundation.
Key Considerations for Optimal Performance
Factor | Recommendation |
---|---|
Memory Usage | Optimize memory by releasing objects when no longer needed and using lazy loading techniques. |
CPU Load | Avoid blocking the main thread; use background threads for heavy tasks to keep the UI responsive. |
Network Efficiency | Use asynchronous calls for network requests to prevent delays in user interactions. |
Testing Your App Using Xcode's Pre-built Templates: A Detailed Walkthrough
When creating an app with Xcode, utilizing its built-in templates can significantly speed up the development process. These templates provide pre-designed project structures that you can customize based on your needs. However, ensuring your app works properly requires thorough testing. In this guide, we will walk you through the process of testing your app with Xcode's default templates, highlighting key steps and best practices.
Testing is an essential part of the app development cycle. Xcode offers several testing tools and strategies that help verify your app’s functionality, performance, and user experience. This guide breaks down how you can use these tools in the context of an app created with a standard template in Xcode.
Steps to Test Your App in Xcode
Follow the steps below to start testing your app effectively:
- Build the App - First, ensure your project builds without any errors. Click on the “Build” button in Xcode to compile your app.
- Run the App on Simulator - Choose an iOS simulator device from the top bar and run your app. This helps to test the app on different device configurations.
- Use Unit Testing - Xcode supports unit testing through XCTest. Create test cases to check the functionality of specific functions or features in your app.
- Run UI Tests - Perform automated UI tests by interacting with your app in the simulator. You can simulate gestures and verify that the app responds as expected.
Important Tips for Testing in Xcode
To ensure your testing process is smooth, keep these tips in mind:
- Use Debugging Tools - Leverage Xcode’s debugger to find and fix issues. Breakpoints, variable inspection, and stack traces are invaluable during testing.
- Test on Real Devices - While simulators are useful, testing on physical devices ensures that your app performs well in a real-world environment.
- Monitor Performance - Use Instruments in Xcode to measure your app’s memory usage, CPU load, and network performance.
Common Issues and Solutions
Issue | Solution |
---|---|
App crashes on launch | Check for nil values, uninitialized variables, or incorrect storyboard connections. |
UI misalignment on different devices | Use Auto Layout to create flexible and adaptive user interfaces. |
Slow performance | Optimize heavy operations and consider using background threads for intensive tasks. |
Remember: Always test your app under various conditions, including different network speeds, device orientations, and screen sizes, to ensure it works across all use cases.
Common Challenges When Working with Xcode App Templates and Solutions
When developing iOS applications using Xcode templates, developers often encounter several obstacles. These templates are designed to speed up the development process, but they can also introduce some complexities if not managed properly. Below, we discuss some common issues developers face and how to address them effectively.
One of the major challenges when using Xcode templates is the lack of flexibility in customizing the pre-configured UI components. While templates provide a starting point, it can be difficult to modify elements like navigation bars, table views, and other UI components without breaking the overall layout. Additionally, developers may struggle with outdated or deprecated code within templates that doesn't align with the latest iOS development practices.
Common Challenges
- Limited UI Customization: Pre-set UI components may not meet the specific design needs of the app.
- Outdated Code: Templates may include deprecated methods or components, leading to compatibility issues with newer iOS versions.
- Over-Complicated Structure: Some templates have complex project structures that can be confusing, especially for beginners.
Solutions
- Customizing UI Components: It's crucial to understand the base code of the template. Modify components incrementally to maintain the layout's integrity. Use SwiftUI or UIKit, depending on the template, and ensure custom views align with Apple's design guidelines.
- Updating Deprecated Code: Regularly check for deprecated methods or libraries. Replace old API calls with modern alternatives and keep track of updates in the iOS SDK.
- Streamlining Project Structure: Take time to restructure the project and remove unnecessary files. This can make the project easier to maintain and more manageable for future updates.
Tip: Before using any template, thoroughly review its contents. Make sure the template’s structure and components are compatible with your app's needs to avoid time-consuming rework.
Example Table: Comparing Templates
Template Type | Customization Flexibility | Code Modernity |
---|---|---|
Single View App | High | Medium |
Tab Bar App | Medium | High |
Master-Detail App | Low | Medium |
Scaling Your iOS Project with Built-In Templates for Enhanced Functionality
When developing a complex iOS application, using standard templates in Xcode can significantly streamline the process of adding advanced features. Xcode offers various built-in templates that provide foundational structures for common app components. These templates help developers save time by providing the necessary starting points, while also allowing for scalability as your project grows in complexity. Templates such as the "Single View App," "Tab Bar App," or "Master-Detail App" serve as efficient blueprints to begin integrating more sophisticated functionalities, whether you're building a simple tool or a feature-rich application.
By utilizing these templates, developers can focus more on adding custom features rather than constructing boilerplate code. For example, the "Master-Detail App" template already sets up a navigation controller and a split-view interface, enabling the easy addition of features like data handling, list management, and split views for large screens. As the app evolves, you can build upon these initial structures, integrating advanced elements such as cloud storage, real-time data updates, or deep integration with device hardware.
Key Advantages of Using Standard Templates for Scaling
- Faster initial setup for commonly used app structures.
- Flexibility to add custom functionalities on top of existing layouts.
- Built-in support for modern design patterns and best practices.
Example Use Cases
- Real-Time Collaboration: Start with the "Master-Detail App" template to handle data and use Firebase for real-time syncing.
- Content Management App: Use the "Tab Bar App" template to integrate content categorization and seamless navigation.
- Media Playback: Build upon the "Single View App" template to incorporate advanced video and audio features.
"Xcode templates are designed to save time on repetitive tasks, allowing developers to focus on the unique aspects of their applications."
Template | Key Features | Ideal For |
---|---|---|
Single View App | Basic UI, no navigation | Simple apps, quick prototypes |
Tab Bar App | Tab-based navigation, easy to extend | Apps with multiple sections or views |
Master-Detail App | Data-driven, split-view support | Apps displaying lists or hierarchical data |