Android App Development Herve Franceschi Pdf
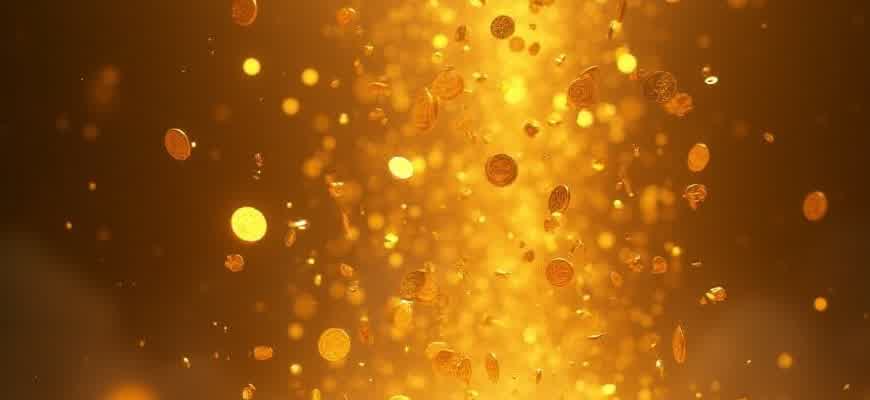
In the field of mobile application development, Android remains one of the most dominant platforms. The book by Herve Franceschi provides a thorough approach to mastering Android development. By focusing on practical examples and detailed explanations, it covers everything from the basics of app creation to advanced techniques that ensure smooth user experiences.
"Mastering Android development requires a solid understanding of Java, XML, and Android SDK, as well as the ability to integrate various tools and libraries effectively."
The following key topics are explored in the book:
- Setting up the Android Development Environment
- Understanding UI components and layout management
- Working with databases and content providers
- Integrating APIs and external libraries
- Optimizing apps for performance and user experience
The book breaks down each concept into manageable chunks, ensuring that developers of all levels can follow along easily. Below is an overview of the development process outlined in the book:
Phase | Description |
---|---|
Initial Setup | Install necessary tools and configure the development environment. |
Design | Create layouts and design user interfaces using XML and Android Studio. |
Implementation | Write Java code to implement app functionality and handle user interactions. |
Testing | Test the app using Android emulators and real devices to ensure stability. |
Deployment | Prepare the app for distribution via the Google Play Store. |
Android App Development with Herve Franceschi: A Practical Guide
Developing mobile applications for Android can be a complex task, but with the right approach, it becomes much more manageable. Herve Franceschi offers a practical guide for both beginners and seasoned developers who want to improve their skills in Android app development. His book takes a hands-on approach to explain essential concepts, tools, and techniques needed to create efficient and functional Android applications.
Franceschi emphasizes a modular approach, guiding developers through the stages of application creation. From setting up the development environment to publishing the app on the Google Play Store, the guide ensures that readers gain comprehensive knowledge. The focus is not only on coding but also on the practical application of development practices and design principles.
Key Concepts of Android Development
Herve Franceschi’s guide covers several important aspects of Android app development, including:
- Java and Kotlin programming languages: The guide demonstrates how to use both Java and Kotlin to build Android applications, offering a clear comparison of their features and usage.
- Android SDK tools: The author explains how to use essential tools like Android Studio and the Android Emulator, which help in developing, testing, and debugging applications.
- User Interface (UI) design: Practical advice on how to create intuitive and responsive user interfaces that enhance the overall user experience.
Development Process Overview
The development process is broken down into clear steps to guide developers through each stage:
- Setup and Environment Configuration: Installing Android Studio and setting up the necessary SDKs.
- Building Core Features: Implementing basic functionalities like navigation, networking, and data handling.
- Debugging and Testing: Learning how to test apps and resolve common issues that arise during development.
- Publishing: Preparing the app for distribution on the Google Play Store and ensuring compliance with guidelines.
Franceschi encourages developers to focus on clean code and user-centric design, ensuring that their apps are not only functional but also enjoyable to use.
Essential Tools for Android App Development
Tool | Purpose |
---|---|
Android Studio | IDE for Android development, providing features like code completion, debugging, and performance analysis. |
Android Emulator | Allows developers to test applications on various virtual devices without needing physical devices. |
Firebase | Provides backend services like real-time databases, authentication, and analytics for Android apps. |
Understanding the Basics of Android App Development with Herve Franceschi
Android application development has become a core skill for many software developers. With the growing popularity of mobile devices, learning how to create apps for the Android operating system is crucial for staying competitive. Herve Franceschi’s approach to teaching Android development offers a structured and comprehensive guide for beginners and intermediate developers. His book presents the essential concepts in a clear and digestible format, making it easier for readers to grasp the fundamentals and apply them in real-world scenarios.
In his work, Franceschi dives deep into the architecture of Android applications, covering everything from the basic structure to advanced features like multi-threading and integrating APIs. He emphasizes hands-on learning through practical examples, enabling readers to build functioning apps from the ground up. This method helps learners gain confidence and develop a solid foundation in Android development, setting them up for success in their own projects.
Key Concepts of Android Development
- Application Components: Understanding the key components such as Activities, Services, Broadcast Receivers, and Content Providers is essential for creating Android apps.
- UI Design: Franceschi stresses the importance of designing user-friendly interfaces using XML layout files and Android Studio’s tools.
- Data Management: Managing data using SQLite databases and content providers is another critical skill for developers.
- Networking: Making network requests to retrieve and send data from APIs is covered in detail, along with tips on handling responses efficiently.
Step-by-Step Guide to Building Your First App
- Set up the development environment: Install Android Studio, the official IDE for Android development, and set up your first project.
- Define app components: Create Activities, set up Intents for navigation, and configure Services and Broadcast Receivers as needed.
- Design the user interface: Use XML to design the layout and add elements such as buttons, text fields, and images.
- Integrate functionality: Write Java or Kotlin code to define how the app behaves and responds to user interactions.
- Test the app: Run the app on an emulator or a physical device to check for bugs and optimize performance.
"The key to mastering Android development lies in understanding the core components and how they work together to create functional, efficient applications." – Herve Franceschi
Understanding Android Architecture
Component | Description |
---|---|
Activity | Handles the user interface and user interactions. |
Service | Performs background tasks such as data synchronization or media playback. |
Broadcast Receiver | Receives and responds to system-wide broadcast messages (e.g., battery low, network connectivity changes). |
Content Provider | Manages shared data between different apps, such as contacts or media files. |
Key Components of Android Application Development: What You Need to Understand
When diving into Android application development, it is essential to familiarize yourself with the various components that make up a mobile app. Each part plays a crucial role in ensuring that the app functions properly and offers a smooth user experience. From UI elements to back-end services, understanding the building blocks will allow you to design effective and scalable applications.
Android app development is a multi-layered process that requires knowledge in both the front-end and back-end. The Android ecosystem is built around a robust framework that allows developers to create apps for smartphones, tablets, and other devices. Below are the fundamental components you need to focus on for a successful Android project.
Important Elements in Android App Architecture
- Activities - An Activity serves as the entry point for interacting with the user. Each screen of an app typically represents an Activity.
- Fragments - Fragments are modular sections of an Activity, allowing for more flexible UI designs and user interactions.
- Services - Services run in the background to perform long-running operations like playing music or syncing data.
- Broadcast Receivers - Broadcast Receivers listen for global system messages, such as network connectivity changes or battery warnings.
- Content Providers - These facilitate data sharing between different apps, enabling access to databases and other resources.
Steps for Efficient Android App Development
- Planning and Prototyping - Before you start coding, clearly outline the features and design layout of your app. This is essential for smooth development.
- Setting Up the Development Environment - Install Android Studio and ensure you have the necessary SDK tools.
- Building the UI - Use XML and UI components like buttons, text fields, and lists to create an intuitive design.
- Implementing Business Logic - Develop the core functionality of the app, focusing on efficient code and minimizing bugs.
- Testing and Debugging - Use Android's built-in testing tools to check for errors and optimize the app's performance.
- Publishing - Once the app is ready, submit it to the Google Play Store after thorough testing.
Important Tools and Libraries for Android Development
Tool/Library | Purpose |
---|---|
Android Studio | Official IDE for Android development, offering debugging, emulation, and code suggestions. |
Retrofit | A powerful library for network requests and API handling. |
Glide | Used for image loading and caching in Android apps. |
Room | A database library to manage local storage using SQLite databases. |
Mastering Android development requires not only understanding the key components but also knowing how to combine them seamlessly. Keep in mind that each component serves a distinct purpose and contributes to the overall user experience.
Setting Up the Development Environment for Android Applications
To start developing Android applications, setting up the right environment is crucial. Android Studio is the official integrated development environment (IDE) for building Android apps. It provides all the tools you need to write, test, and debug your application. Here’s how you can set up your development environment for Android app development.
The first step is to install Android Studio, the recommended IDE for Android development. It includes all the necessary SDKs, build tools, and emulators required for development. Make sure your computer meets the system requirements for Android Studio to run efficiently.
Installation Steps
- Download Android Studio from the official website.
- Run the installer and follow the on-screen instructions.
- Once the installation is complete, open Android Studio and configure the initial setup.
- Install the Android SDK through the SDK Manager within Android Studio.
Configuring the Android SDK
The Android SDK provides the essential tools to build and test Android applications. During the installation process, you need to download the SDK components that include Android platforms, build tools, and device emulators. It’s important to update these components regularly to avoid compatibility issues.
Note: Be sure to check for updates regularly in the SDK Manager to ensure your environment is up-to-date with the latest features and fixes.
Setting Up Emulators
To test your applications, Android Studio allows you to create virtual devices through its emulator. You can select from various Android device configurations, which will simulate different screen sizes and Android versions.
Device Type | Screen Size | Android Version |
---|---|---|
Pixel 5 | 6.0 inches | Android 11 |
Pixel 3 | 5.5 inches | Android 9 |
Setting Up Version Control
- Install Git for version control management.
- Set up a GitHub repository to store your projects online.
- Ensure your project is connected to Git for easy collaboration and version management.
Step-by-Step Guide to Creating Your First Android App
Starting your journey in Android app development can seem daunting, but with a clear, structured approach, you can quickly build your first app. This guide will walk you through the necessary steps, from setting up the development environment to testing and running your app on an Android device or emulator.
Before diving into the code, ensure you have the required tools. Android Studio is the official IDE for Android development, providing all the necessary features, such as code completion, debugging, and emulators to test your apps.
Step 1: Set Up Your Development Environment
- Download and install Android Studio from the official website.
- Launch Android Studio and follow the setup instructions to install necessary SDK components.
- Make sure your system meets the minimum requirements for Android Studio to run smoothly.
Step 2: Create a New Project
- Open Android Studio and click on “Start a new Android Studio project.”
- Select a project template. For beginners, choose “Empty Activity” to keep things simple.
- Set your application name, package name, and save location. Make sure to choose a language (either Java or Kotlin).
- Click on “Finish” to generate the basic project structure.
Step 3: Understanding the Project Structure
Folder | Description |
---|---|
app/src/main/java/ | Contains your main app code and classes. |
app/src/main/res/ | Holds resources like images, layout files, and strings. |
app/src/main/AndroidManifest.xml | Defines app components and permissions. |
Tip: Always check the Android Manifest file when adding new features or permissions to your app.
Step 4: Build Your First UI
- Navigate to the “res/layout/activity_main.xml” file to define your app's interface.
- Use XML to create views like buttons, text fields, and images. For example, add a Button element:
Step 5: Add Logic to Your App
Open the MainActivity.java (or MainActivity.kt if using Kotlin) to add functionality to the button you created. For example, you can set up an OnClickListener to respond to the button click.
Button myButton = findViewById(R.id.button); myButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Toast.makeText(MainActivity.this, "Button clicked!", Toast.LENGTH_SHORT).show(); } });
Step 6: Test Your App
- Use the built-in Android Emulator or connect a physical device to test your app.
- Click on the “Run” button in Android Studio to deploy the app to your device or emulator.
- Ensure there are no crashes or bugs, and verify that your app works as expected.
Debugging and Testing Best Practices for Android Apps
When developing Android applications, debugging and testing are crucial steps in ensuring the stability and reliability of your app. Proper testing helps to identify bugs early in the development process, while debugging aids in fixing issues that arise during testing. Adopting a structured approach to these tasks ensures that your app performs smoothly across various devices and Android versions.
Effective debugging and testing techniques not only improve the user experience but also contribute to a more maintainable and scalable app. Using the right tools and following best practices will help you streamline the development process and avoid costly errors in the long term.
Debugging Techniques
To streamline the debugging process, consider the following practices:
- Use Logcat for Detailed Logs: Logcat is essential for understanding what happens during runtime. By adding custom log messages, you can track the flow of the application and identify potential issues.
- Employ Breakpoints: Using breakpoints within your IDE allows you to pause execution and inspect variables and memory. This helps to identify the root cause of unexpected behavior.
- Debug on Multiple Devices: Testing on different devices ensures that your app behaves as expected across various screen sizes and hardware configurations.
Best Practices for Testing
Testing should be an ongoing activity throughout the development cycle. Some key testing practices include:
- Unit Testing: Write unit tests for each component of your application to ensure that individual modules function correctly in isolation.
- UI Testing: Automate UI testing using tools like Espresso to simulate user interactions and verify the UI behaves as expected under different conditions.
- Integration Testing: Validate that different modules of your app work together seamlessly.
- Performance Testing: Use tools like Android Profiler to identify performance bottlenecks and optimize your app’s resource usage.
Key Tools for Debugging and Testing
Tool | Purpose |
---|---|
Android Studio Debugger | Breakpoints, memory inspection, and live debugging. |
JUnit | Unit testing framework for testing Java code. |
Espresso | UI testing for user interactions and UI components. |
Android Profiler | Performance profiling tool for identifying memory and CPU usage issues. |
Tip: Always test on real devices, as emulators might not replicate the exact behavior of physical hardware, especially when it comes to performance and sensor interactions.
Integrating APIs and External Libraries in Android Development
Integrating third-party APIs and libraries into an Android application can significantly enhance its functionality and performance. External libraries help developers save time by providing ready-made solutions for complex tasks. APIs, on the other hand, allow your app to interact with external services, such as social media platforms, weather data providers, or payment gateways.
To successfully integrate these resources into your Android app, it's essential to follow a structured process. This process typically involves adding the necessary dependencies, configuring network calls for APIs, and managing authentication. Below is a step-by-step guide to integrating APIs and external libraries in your Android project.
Steps to Integrate APIs and Libraries
- Identify the required library or API: Determine what functionality your app needs and find the relevant library or API that suits your requirements.
- Include dependencies in the project: Use the Gradle build system to add libraries to your project. For APIs, you can add the necessary dependencies in the build.gradle file.
- API Configuration: For API integration, configure network settings such as base URLs and authentication tokens.
- Make network requests: Use libraries like Retrofit or Volley to make API calls and handle responses asynchronously.
- Handle data response: Parse the response data, typically in JSON format, and update the UI accordingly.
Important: Ensure that the library or API you choose is actively maintained and compatible with the latest version of Android. This will ensure better security and functionality.
Example of Library Integration
Library | Purpose | Gradle Dependency |
---|---|---|
Retrofit | Network library for API calls | implementation 'com.squareup.retrofit2:retrofit:2.9.0' |
Gson | For JSON parsing | implementation 'com.google.code.gson:gson:2.8.6' |
Once the libraries are added to your project, you can begin making API calls or using library methods in your app's code. Proper error handling, security, and efficient data management are key to a smooth integration process.
Optimizing Your Android App for Performance and User Experience
Efficiently developing an Android app requires a careful balance between performance and user experience. To ensure an app performs optimally, developers must focus on areas like memory management, responsiveness, and efficient use of resources. These elements are critical for creating an app that runs smoothly across a variety of devices, providing users with an enjoyable and seamless experience.
Performance optimization directly influences the speed, responsiveness, and overall smoothness of your app. A sluggish app will lead to user frustration and higher uninstallation rates. By focusing on performance while keeping the user interface intuitive and easy to navigate, developers can deliver an experience that keeps users engaged and satisfied.
Key Areas to Focus on for Optimizing Performance
- Memory Management: Efficiently managing memory helps prevent app crashes and slowdowns. Keep an eye on memory leaks and use tools like Android Profiler to identify areas of improvement.
- Network Efficiency: Reduce the frequency of network requests and optimize data retrieval to prevent delays in loading content. Use caching mechanisms to speed up data loading.
- Thread Management: Perform heavy tasks in the background to avoid blocking the main thread, ensuring smooth UI interactions.
Enhancing User Experience
- UI Responsiveness: The app should respond to user actions immediately. Avoid delays in button presses or screen transitions to maintain a fluid interaction.
- Consistency: Use standard Android UI patterns to help users feel familiar with the app's interface, reducing the learning curve.
- Feedback: Provide visual or haptic feedback to show that the app is processing user actions, keeping users informed about the status of their request.
"Optimizing for both performance and user experience is not just about speed; it’s about making the app feel natural and enjoyable to use. Every detail matters."
Tools for Performance Monitoring and Improvement
Tool | Purpose |
---|---|
Android Profiler | Monitor memory usage, CPU usage, and network activity to detect performance issues. |
LeakCanary | Identify and fix memory leaks that can slow down your app. |
Firebase Performance Monitoring | Analyze app performance across real-world conditions, providing insights into areas of improvement. |