Build a Social Media App with Javascript
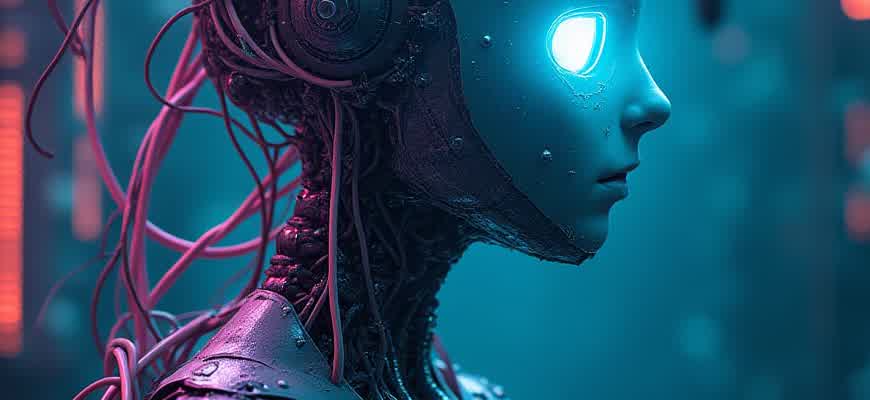
Building a dynamic social media platform requires a blend of front-end and back-end technologies. JavaScript plays a crucial role in the development of interactive user interfaces and handling data communication between the server and client. In this guide, we will break down the key steps to creating a social media app, starting with setting up the front-end using JavaScript.
JavaScript frameworks like React and Vue can significantly speed up the development process for creating responsive and scalable UI components.
The first task in developing your social media application is setting up the user interface. Here’s a quick overview of how to get started:
- Create the main layout structure using HTML and CSS.
- Integrate JavaScript for dynamic content updates and event handling.
- Utilize API calls to retrieve and display user data.
Once the front-end is ready, it’s important to think about how user data will be managed and stored. This is where back-end development comes into play, which can be done using Node.js and a database like MongoDB.
Feature | Description |
---|---|
User Authentication | Handle user login and registration with secure sessions. |
Post Creation | Allow users to create, edit, and delete posts. |
Real-time Messaging | Enable users to send and receive messages in real time. |
Choosing the Best JavaScript Framework for Your Social Media Application
When developing a social media platform, selecting the right JavaScript framework is crucial for performance, scalability, and user experience. Your choice will determine how efficiently you can manage state, handle user interactions, and scale your app as it grows. Each framework offers unique benefits and challenges, making the decision complex but important.
Popular JavaScript frameworks vary significantly in terms of flexibility, speed, and ecosystem support. It's essential to assess your app's requirements–whether it's real-time communication, data-heavy features, or rapid development–and choose a framework that aligns with your goals. Here's a comparison of the most commonly used frameworks for building social media apps.
Framework Comparison
Framework | Performance | Real-time Support | Community Support |
---|---|---|---|
React | High | Good (with libraries like Firebase) | Large and Active |
Vue.js | High | Moderate | Growing but Smaller |
Angular | Moderate | Strong (with RxJS) | Large and Mature |
For social media apps, consider the following when choosing a framework:
- Real-time data updates: Social apps require quick and efficient real-time data synchronization, so choose a framework with good real-time libraries or built-in support.
- Scalability: A framework should be able to handle a large number of users without compromising performance. React is often preferred for high-performance applications, but Angular may be more suited for large, enterprise-level apps.
- Community and Ecosystem: A well-supported framework ensures that you'll have access to resources, libraries, and a robust developer community to help with troubleshooting and development.
"React is favored by many developers for its flexibility, speed, and huge ecosystem, making it an ideal choice for social media platforms requiring high interactivity and real-time data handling."
Implementing Real-Time Messaging with WebSockets in JavaScript
Integrating real-time chat into a social media application is essential for user engagement. One of the most efficient ways to achieve this is by using WebSockets, which allow for bidirectional communication between the client and server. Unlike traditional HTTP requests, WebSockets keep the connection open, enabling instant message delivery and reducing latency. In this section, we will walk through setting up WebSocket functionality in a social media app using JavaScript.
WebSocket provides a reliable, low-latency solution for real-time interactions. By maintaining an open connection, it eliminates the need for continuous polling, making it an optimal choice for applications like messaging platforms where instant communication is crucial.
Steps to Implement Real-Time Chat with WebSockets
- Set up a WebSocket server (e.g., using Node.js and the 'ws' package).
- Connect the client to the WebSocket server using the WebSocket API in JavaScript.
- Implement event listeners for message handling, user connection, and disconnection.
- Broadcast messages to all connected clients or send them to specific users based on their identifiers.
Once the connection is established, clients can send and receive messages in real-time. The WebSocket API simplifies this process by offering methods like send() to transmit data and onmessage to handle incoming messages.
Important: WebSocket connections should be securely handled, especially in production environments. Always use wss:// for secure connections and consider implementing authentication to ensure only authorized users can access the chat.
Sample WebSocket Server Setup
Step | Code Example |
---|---|
Install 'ws' package | npm install ws |
Set up server | const WebSocket = require('ws'); |
Handle incoming connections | wss.on('connection', ws => { |
Creating a Secure Authentication System with JWT in Node.js
Building a secure user authentication system is a crucial part of any web application. With the rise of single-page apps and RESTful APIs, JSON Web Tokens (JWT) have become the go-to method for managing user sessions. JWT provides a compact, URL-safe means of representing claims to be transferred between two parties. In a Node.js environment, implementing JWT can help ensure that user authentication is seamless and secure.
The basic flow involves generating a JWT after the user successfully logs in, which is then stored in the client (usually in a cookie or localStorage). This token is sent with each subsequent request to validate the user's identity without requiring them to log in again. Below is a breakdown of how to implement JWT authentication in a Node.js application.
Steps to Implement JWT Authentication
- Set up Node.js and required packages: Install essential packages like express, jsonwebtoken, and dotenv to handle authentication logic.
- Create the Login Endpoint: When a user submits their credentials, validate them against the database. If valid, generate a JWT and send it back to the client.
- Protect Routes with Middleware: Use middleware to check if the JWT is present in the request header. If the token is invalid or missing, deny access to the protected route.
- Handle Token Expiration: Tokens typically expire after a set period. Set up token renewal or log out functionality to handle expired tokens.
JWT tokens should never store sensitive user data directly. Instead, they should only store the necessary claims, such as user ID, role, or permissions. The payload can be decoded without a secret, so sensitive information must be avoided in this part of the token.
Example Authentication Flow
- User logs in by submitting their username and password.
- The server validates the credentials against the database.
- If valid, a JWT is created and returned to the user.
- The client stores the token, typically in localStorage or cookies.
- For each subsequent request, the client sends the token in the Authorization header.
- The server validates the token using a secret key before granting access to protected resources.
JWT Token Structure
Part | Description |
---|---|
Header | Contains the type of token (JWT) and the signing algorithm (e.g., HS256). |
Payload | Contains the claims (user data, permissions, etc.). |
Signature | Used to verify the integrity of the token and ensure it wasn't tampered with. |
Incorporating Media Uploads in a Social Networking Platform
Integrating image and video uploads is a key feature in any modern social networking platform. By allowing users to share their visual content, you enhance user engagement and provide a richer experience. However, handling large media files efficiently is essential to maintaining the overall performance of the app.
For this, you need to implement file uploading, validation, and storage solutions that handle both images and videos. The process generally involves the front-end collecting user input, sending it to the back-end server, and storing the media either on your server or a cloud service like AWS S3 or Google Cloud Storage.
Steps to Implement Image and Video Uploads
- User Interface: Create a file input element that allows users to select multiple files for upload. Ensure that the interface is responsive and offers an easy-to-use drag-and-drop feature.
- Client-side Validation: Before uploading, validate file types (e.g., JPG, PNG for images; MP4, MOV for videos) and size limits on the client side.
- Back-end Handling: Once the file is uploaded, process it on the back-end. You may need to compress the media or resize images to save bandwidth.
- Cloud Storage: Store media files in cloud storage services to ensure scalability and security. Use signed URLs to grant secure access to uploaded content.
Considerations for Video Uploads
- Video Compression: Large video files can slow down the app, so consider compressing the videos before uploading or providing automatic compression on the server.
- File Formats: Ensure your platform supports various formats, such as MP4, WebM, or Ogg, for cross-platform compatibility.
- Streaming: To improve user experience, stream videos instead of loading them entirely, especially for longer content.
Tip: Always limit the size of uploaded media to avoid excessive server load and ensure quick upload times. Implementing both client-side and server-side validation for file size and type will save bandwidth and improve user experience.
Example Media File Upload Process
Step | Action |
---|---|
1 | File selection from user interface |
2 | Client-side validation (check file type, size) |
3 | Upload file to server or cloud storage |
4 | Store file URL and associate with user profile |
Designing a Scalable Backend with Express.js for Social Media Applications
Building a robust backend for a social media platform requires careful planning, particularly when it comes to scalability. Express.js, a minimalist web framework for Node.js, is a great choice for developing highly scalable and efficient server-side applications. It allows developers to quickly build APIs, handle HTTP requests, and manage routing effectively. However, when designing a backend architecture that supports millions of users, it's essential to implement strategies that ensure high performance and easy scalability.
One key aspect to consider when using Express.js is how to manage large amounts of data, user interactions, and real-time features. Social media applications often include features like posts, comments, likes, and direct messaging, each requiring its own database schema and an efficient way of handling connections. In this section, we’ll dive into best practices for building a scalable backend using Express.js and discuss strategies for managing traffic and user data.
1. Efficient Routing and Middleware Management
In a social media app, handling different types of user requests and managing their flow efficiently is crucial. Using middleware and creating modular routes can significantly improve the app's performance. Express allows for easy routing of requests, but managing large amounts of traffic requires proper middleware integration. Some of the best practices include:
- Use middleware for authentication and data validation.
- Implement caching mechanisms for frequently accessed data.
- Structure routes in a modular way to prevent bloating.
2. Data Management and Database Optimization
As user interactions scale, the database layer becomes a bottleneck. Social media platforms need to store massive amounts of data, from user profiles to interactions like posts and comments. It’s essential to use efficient database models and design queries to optimize performance. Consider the following strategies:
- Use NoSQL databases like MongoDB for flexible data storage and quick access.
- Implement database sharding to split data across multiple servers for load balancing.
- Leverage indexing to speed up query execution times, especially on large collections.
Tip: For applications with real-time data, using WebSockets can help push updates to clients without the need for constant polling.
3. Handling User Traffic and Load Balancing
Scalability not only involves managing data efficiently but also dealing with high traffic volumes. With increasing users, server performance could degrade, causing delays in response time. To handle large numbers of users, consider:
- Implementing a load balancer to distribute traffic across multiple instances of your application.
- Using horizontal scaling to add more server instances as traffic increases.
- Setting up automated scaling on cloud platforms like AWS or Azure for flexibility.
4. Real-Time Features and Event-Driven Architecture
Real-time updates are crucial for social media applications, enabling instant notifications, live chat, and activity feeds. Express.js can be combined with libraries like Socket.IO to create real-time bidirectional communication between clients and servers. This event-driven approach helps deliver instantaneous data to users, keeping them engaged. Here’s a basic structure of how real-time communication can be managed:
Component | Responsibility |
---|---|
Socket.IO | Real-time communication between server and client |
Express.js | Handle API requests and manage HTTP routes |
Redis | Pub/Sub for message brokering and data synchronization |
Creating Personalized News Feeds with JavaScript and MongoDB
Building a custom news feed is a critical feature in most social media platforms. By leveraging JavaScript and MongoDB, developers can dynamically generate personalized content for each user. MongoDB, with its NoSQL structure, allows for quick retrieval and flexible storage of user-specific data, while JavaScript enables real-time updates and seamless interactivity in the browser.
To create an efficient personalized feed, you need to store and retrieve relevant data based on user preferences, activity, and social connections. This involves integrating MongoDB to handle the database operations and JavaScript to manage the client-side experience, ensuring users always have the latest updates in their feeds.
Key Concepts for Building the Feed
- User Profiles: Each user has a profile containing preferences, interests, and connections.
- Posts and Content: Posts can be text, images, or videos, stored in MongoDB and linked to user profiles.
- Feed Generation: The news feed is generated by querying posts that match a user's interests, connections, or recent activity.
- Real-Time Updates: JavaScript’s asynchronous capabilities, like using WebSockets, ensure the feed is updated without refreshing the page.
Database Structure in MongoDB
For optimal performance, your MongoDB database should store documents that reflect both user activity and content. A simple structure could include two main collections: users and posts.
Collection | Field | Description |
---|---|---|
Users | _id | Unique user identifier |
Users | preferences | Interests and categories the user follows |
Posts | user_id | References the user who created the post |
Posts | content | The actual content of the post (text, image, etc.) |
Posts | timestamp | Date and time the post was created |
By indexing frequently queried fields (such as user preferences or post timestamps), MongoDB can efficiently return personalized feed content, reducing loading times and improving user experience.
Using JavaScript for Dynamic Feed Updates
To dynamically generate the feed in the browser, JavaScript can be used to make asynchronous requests to the server for new posts. This can be done using AJAX calls, ensuring content is loaded in the background without interrupting the user’s interaction. With frameworks like React or Vue.js, the feed can be updated in real-time by monitoring changes to the data model.
- AJAX Requests: Fetch new posts based on user activity and preferences.
- Event Listeners: Monitor interactions like liking or commenting on posts to trigger feed updates.
- Dynamic Rendering: Use JavaScript to insert new posts into the feed as they become available.
Optimizing Your Social Media Application for Mobile Usage
Creating a seamless user experience on mobile devices is crucial for any modern social media platform. As mobile browsing continues to dominate, ensuring that your app functions smoothly on smartphones and tablets is essential. Poor mobile optimization can lead to slow load times, interface glitches, and ultimately, user frustration. Therefore, mobile optimization should be a priority when developing your app.
When working on a mobile-friendly version of your social media app, focus on performance, usability, and adaptability. Ensure that your application is lightweight and responsive, providing a smooth browsing experience across various screen sizes and orientations. Below are some key strategies to consider during development.
Key Mobile Optimization Techniques
- Responsive Design: Use flexible layouts and CSS media queries to ensure that your app looks great on all devices, from phones to tablets.
- Image Optimization: Compress images and use modern formats like WebP to reduce load times without sacrificing quality.
- Lazy Loading: Implement lazy loading for images and videos to improve page speed by only loading resources as needed.
- Touch-Friendly Interface: Ensure all clickable elements, such as buttons and links, are easy to tap with a finger.
Important: Test your app thoroughly on different devices to identify any performance or usability issues before release.
Performance Optimization Strategies
- Minimize HTTP Requests: Reduce the number of requests made by your app, including external resources, by combining and minifying scripts and stylesheets.
- Use Caching: Leverage browser caching to store frequently accessed content locally and avoid re-downloading on every visit.
- Optimize JavaScript: Keep your JavaScript lightweight, avoiding large frameworks unless necessary. Consider using progressive loading techniques.
Comparison of Mobile vs Desktop Performance
Aspect | Mobile | Desktop |
---|---|---|
Screen Size | Smaller, requires adaptive layout | Larger, fixed layout |
Input Method | Touch-based | Mouse/keyboard |
Network Speed | Variable, may use slower connections | More consistent, generally faster |
Protecting User Data and Addressing Security Threats in JavaScript Applications
When building a social media application, safeguarding user information is paramount. JavaScript, being widely used for both client and server-side scripting, introduces several security risks that developers must address. Data exposure, unauthorized access, and malicious attacks can undermine the integrity of the platform. It's essential to implement strategies that protect sensitive data and prevent common vulnerabilities in the codebase.
Understanding and mitigating security threats are crucial steps for creating a secure social media app. Below are some important practices to follow to protect user data and minimize vulnerabilities in your JavaScript application.
Key Security Practices for JavaScript Development
- Use HTTPS: Ensure that all data exchanges between the client and server are encrypted by enforcing HTTPS. This prevents attackers from intercepting sensitive information through man-in-the-middle attacks.
- Sanitize User Inputs: Always validate and sanitize user input to prevent XSS (Cross-Site Scripting) attacks. This reduces the risk of malicious scripts being executed on the browser.
- Secure Authentication: Use secure authentication mechanisms, such as JWT (JSON Web Tokens) or OAuth, to manage user sessions and minimize the risk of session hijacking.
- Implement Proper Error Handling: Avoid exposing sensitive server information or stack traces in error messages. Instead, display user-friendly error messages to prevent attackers from gaining insight into the system.
Important: Always update third-party libraries and dependencies to ensure that known vulnerabilities are patched. Use tools like npm audit or Snyk to detect security flaws in your packages.
Common Vulnerabilities in JavaScript and How to Avoid Them
- Cross-Site Scripting (XSS): This vulnerability occurs when malicious scripts are injected into a web page. To mitigate this, always escape data and avoid direct embedding of untrusted content in the DOM.
- Cross-Site Request Forgery (CSRF): Attackers trick users into making unwanted requests. Protect against CSRF by using anti-CSRF tokens in forms and verifying the origin of requests.
- Insecure Direct Object References (IDOR): This flaw allows users to access unauthorized resources. Ensure that access control checks are implemented properly at the server-side.
- SQL Injection: Avoid this vulnerability by using prepared statements and parameterized queries when interacting with databases, preventing the execution of arbitrary SQL code.
Summary Table: Security Measures
Vulnerability | Prevention |
---|---|
Cross-Site Scripting (XSS) | Sanitize inputs, use Content Security Policy (CSP) |
Cross-Site Request Forgery (CSRF) | Use CSRF tokens, check referer headers |
SQL Injection | Use parameterized queries, avoid dynamic queries |
Insecure Direct Object References (IDOR) | Implement access control checks, validate user permissions |