Ios App Building Course
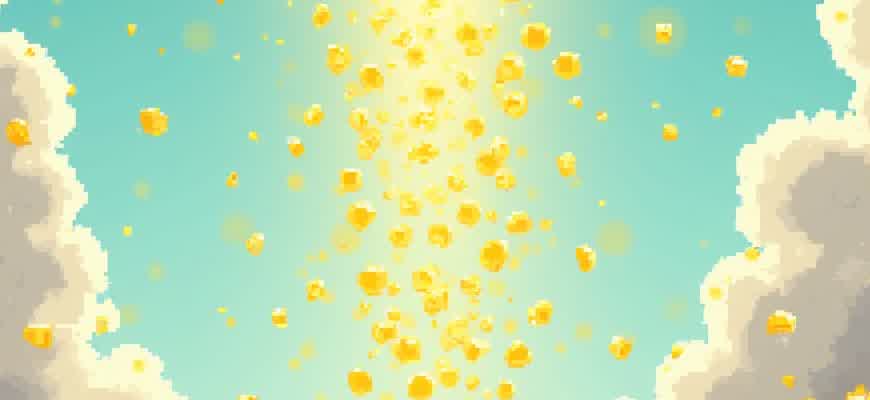
In the rapidly evolving world of mobile development, mastering the process of building iOS apps is a valuable skill for developers. This course offers a hands-on approach to creating applications for Apple devices, focusing on both the theory and practical implementation. Students will be introduced to key concepts such as user interface design, Swift programming, and app deployment.
Key Topics Covered:
- Swift Fundamentals and Advanced Features
- Designing User-Friendly Interfaces with SwiftUI
- Integrating APIs and Managing Data
- Publishing and Maintaining Apps on the App Store
“The course is designed to provide not just theoretical knowledge, but also the real-world skills needed to successfully build and launch iOS applications.”
Course Structure:
Week | Topics |
---|---|
1 | Introduction to Swift and Xcode |
2 | UI Design Principles and SwiftUI Basics |
3 | Networking and Working with APIs |
4 | App Debugging and Optimization |
5 | Publishing on the App Store |
Mastering iOS App Development: A Comprehensive Guide
Building iOS applications requires a deep understanding of both the programming language and the ecosystem. This course is designed to provide you with a thorough grounding in all aspects of iOS development. Whether you're new to programming or an experienced developer looking to refine your skills, this guide offers a structured approach to mastering mobile app development on Apple platforms.
Throughout this course, you will learn how to use essential tools like Xcode, Swift, and Interface Builder, all while developing a range of projects. Each module will cover key concepts such as UI design, data management, and performance optimization, ensuring that you gain both theoretical knowledge and practical experience.
Key Skills You'll Acquire
- Mastering Swift programming language for iOS development
- Building responsive user interfaces with Interface Builder
- Utilizing Apple's development tools, such as Xcode
- Integrating third-party libraries and APIs
- Debugging and optimizing apps for performance and usability
Course Structure
- Introduction to iOS Development – Setting up the development environment and understanding iOS architecture
- Swift Basics – Learning the syntax and core concepts of Swift
- Building User Interfaces – Mastering Interface Builder to create intuitive and functional UI
- Advanced Features – Implementing networking, databases, and advanced UI elements
- App Optimization – Techniques for improving performance, responsiveness, and memory management
- Deployment and Publishing – Preparing your app for the App Store and managing app releases
Effective mobile app development is not just about coding; it’s about crafting seamless experiences for users. This course emphasizes building apps that are both functional and intuitive.
Tools and Technologies Covered
Tool | Description |
---|---|
Xcode | Apple's IDE for creating iOS applications, with a range of integrated tools for design, coding, and testing. |
Swift | Modern programming language used for building high-performance iOS apps. |
Interface Builder | Graphical tool in Xcode for creating UI elements without writing code. |
Choosing the Right Tools for Building Your First iOS App
When starting your iOS development journey, selecting the appropriate tools can significantly impact your learning curve and the success of your first app. The right set of tools ensures a smooth development process, providing you with essential resources to efficiently code, debug, and test your app. In this section, we will cover the most common tools you should consider using for building your first iOS app.
Choosing the right software depends on your goals, preferences, and the complexity of the app you want to build. Fortunately, there are several popular tools in the iOS development ecosystem, each suited for different needs. Here's a breakdown of key considerations when choosing the tools for your project.
1. Integrated Development Environment (IDE)
For most iOS developers, Apple's Xcode is the go-to IDE. It provides a powerful suite of tools for coding, debugging, and testing iOS applications. Here's why you should consider it:
- Swift and Objective-C support: Xcode supports both languages, but Swift is the recommended choice for new developers.
- Interface Builder: A visual tool that simplifies the UI design process without needing to code every UI element manually.
- Simulator: Xcode includes an iOS simulator to test apps on different devices and iOS versions.
Note: Xcode is free to download, but it requires a Mac to run. It is the standard tool recommended by Apple for iOS development.
2. Version Control System
Version control is essential for tracking changes to your code and collaborating with others. Git is the most popular system used by developers, and you can manage your code using GitHub or GitLab for remote repositories.
- Git: A distributed version control system that allows you to track code changes and collaborate with others.
- GitHub: A platform for hosting Git repositories and collaborating with developers worldwide.
3. Testing and Debugging Tools
Ensuring your app works perfectly is crucial. Xcode provides excellent debugging tools, but you might also need additional testing frameworks for unit and UI tests.
- XCTest: A testing framework integrated into Xcode for unit and UI testing.
- Firebase: A backend-as-a-service that provides tools for crash reporting and app analytics.
4. Third-party Libraries and Dependencies
To save time and effort, many developers use third-party libraries. CocoaPods and Swift Package Manager are two popular dependency managers that help integrate external code into your app.
- CocoaPods: A dependency manager that simplifies integration of external libraries into your project.
- Swift Package Manager: Integrated into Xcode, this manager makes it easier to manage packages in Swift projects.
Tool Comparison
Tool | Purpose | Advantages |
---|---|---|
Xcode | IDE for coding, UI design, debugging, and testing | All-in-one solution with great support for Swift |
Git/GitHub | Version control and collaboration | Track code changes and work with other developers |
CocoaPods | Manage third-party libraries | Simplifies the integration of external dependencies |
Setting Up Your macOS Environment for Seamless iOS Development
Before diving into iOS app development, it’s crucial to configure your macOS environment correctly to avoid potential issues later. Proper setup ensures that the development process is smooth, efficient, and free from unnecessary distractions. The most important tools for iOS development on macOS are Xcode and its dependencies. In this guide, we’ll walk through the essential steps to get you started with a clean and optimized setup.
Here’s a breakdown of the steps to set up your macOS environment and start building your iOS app. Follow these instructions carefully to avoid missing important details that may cause roadblocks later on. Once everything is configured, you’ll be able to write, test, and deploy your apps seamlessly.
1. Install Xcode
To begin building iOS apps, the first tool you’ll need is Xcode. Xcode is Apple's integrated development environment (IDE), containing everything you need to create iOS applications.
- Open the Mac App Store.
- Search for Xcode and click "Get".
- Wait for the download and installation to complete.
- After installation, open Xcode and accept the license agreement to finalize the setup.
Once installed, Xcode will automatically install the necessary command line tools, including Git, which is essential for version control in your projects.
2. Update macOS and Xcode Tools
To avoid compatibility issues, it’s vital to keep your system and development tools up-to-date. Regular updates provide bug fixes, new features, and improvements for your development experience.
- Check for macOS updates by opening System Preferences > Software Update.
- Ensure Xcode is updated through the Mac App Store.
- Open Xcode and install any additional components if prompted.
Important: Always ensure your Xcode is updated to the latest stable version to avoid potential incompatibility with iOS SDK versions.
3. Set Up Command Line Tools
Command Line Tools are essential for managing and configuring your development environment directly from the terminal. These tools include essential utilities like git
and clang
for compiling code.
- Open Terminal and run the following command to install command line tools:
xcode-select --install
Once installed, you’ll be able to use command line features in Xcode or any terminal-based operations for version control and managing your project.
4. Verify Your Setup
After setting up the necessary tools, you should verify that everything is correctly installed and working as expected. Here's a simple checklist to ensure that your macOS environment is ready for iOS development:
Tool | Verification Command |
---|---|
Xcode | Open Xcode and check version under About Xcode |
Command Line Tools | Run xcode-select -p to confirm installation path. |
Git | Run git --version to verify proper installation. |
Tip: Always restart your system after updates or installations to ensure all components are loaded correctly.
Understanding Swift: Key Language Features for Building Robust iOS Apps
Swift is a powerful and intuitive programming language for building iOS applications. Developed by Apple, it combines modern features with a high level of performance, making it ideal for mobile app development. Its syntax is clean and easy to read, which helps developers write safer and more efficient code. With Swift, developers can easily integrate advanced features into their apps, from custom UI elements to network connectivity, while ensuring strong performance and security.
To take full advantage of Swift, it's essential to understand some key features that make it stand out. Below, we’ll explore the language's core attributes, which are particularly useful when developing scalable and reliable iOS applications.
Core Features of Swift for Robust iOS App Development
- Optionals and Optional Binding: Swift introduces the concept of optionals, allowing variables to have a "no value" state. This prevents common errors like null pointer exceptions, which are frequent in other languages.
- Type Safety and Type Inference: Swift enforces strict type safety, preventing developers from accidentally assigning an incorrect type to a variable. Its type inference mechanism automatically detects types, reducing the need for explicit declarations.
- Closures and First-Class Functions: Swift supports closures, which are self-contained blocks of functionality that can be passed around and used in your code. Closures in Swift allow for more functional programming techniques and cleaner code.
- Memory Management with Automatic Reference Counting (ARC): Swift’s memory management is handled through ARC, ensuring objects are only kept in memory as long as needed, avoiding memory leaks or excessive memory consumption.
Swift Syntax and Features in Action
Example of Optional Binding: Swift allows you to safely unwrap optionals using if let or guard let, preventing crashes when a value is unexpectedly nil.
if let name = userName { print("Hello, \(name)!") } else { print("No user found.") }
Comparison of Key Swift Features
Feature | Benefit | Example |
---|---|---|
Optionals | Helps handle the absence of values safely | let name: String? = "John" |
Type Safety | Reduces runtime errors by ensuring variables have the correct type | let age: Int = 25 |
Automatic Reference Counting (ARC) | Manages memory automatically, preventing leaks | class ViewController { var label: UILabel? } |
Mastering Xcode Interface: Navigating the Core Development Platform
Understanding the Xcode interface is crucial for building iOS applications efficiently. The platform is packed with features that, when properly mastered, enable a streamlined workflow for developing, debugging, and deploying apps. From code editing to user interface design, Xcode serves as the one-stop tool for all development tasks. Familiarizing yourself with its layout, navigation tools, and key components will enhance your productivity and make app-building a more intuitive process.
The Xcode workspace consists of several core sections that developers use regularly. Learning how to navigate through these sections effectively will help you quickly access tools and resources you need to complete various tasks. Let’s dive deeper into the main areas that you will interact with daily, such as the code editor, interface builder, and the debugging tools.
Key Sections of Xcode
- Navigator Pane: Located on the left side, this section is essential for browsing files, viewing error messages, and accessing various project components.
- Editor Area: This is where you write and modify your code. It also shows UI designs when you're using Interface Builder.
- Utility Area: This section provides information about the selected item, such as properties of UI elements or code methods.
- Debug Area: This is where you can see logs, debug your application, and monitor its performance.
Using the Interface Builder
The Interface Builder allows developers to create and configure their app’s UI without writing code manually. It offers a drag-and-drop interface for adding UI elements, as well as various layout tools to fine-tune the design.
- Drag and Drop Elements: Add UI elements such as buttons, labels, and text fields directly onto the canvas.
- Auto Layout: Use constraints to ensure that your UI elements adjust correctly on different screen sizes.
- Connecting Code and UI: Link UI elements to your code using IBOutlets and IBActions to trigger events and actions.
Tip: Regularly using Interface Builder in combination with the code editor will help you build more efficient, well-structured applications.
Debugging Tools Overview
Debugging in Xcode is made easy with its integrated debugging tools. The Debug Area allows developers to track the app's state, monitor variables, and inspect the flow of execution during runtime.
Tool | Description |
---|---|
Breakpoints | Pause execution at a specific line of code to inspect values and behavior. |
Console | View logs and output messages to troubleshoot issues. |
LLDB Debugger | Advanced tool to evaluate expressions, manipulate data, and interact with running code. |
Mastering these sections and tools will improve your ability to build high-quality iOS apps efficiently. By knowing where to find each tool and how to use it effectively, you will save valuable time during development and be better equipped to troubleshoot and optimize your code.
Designing Seamless User Interfaces with SwiftUI and UIKit
When developing an iOS app, creating a user-friendly and intuitive interface is critical to ensuring a positive user experience. SwiftUI and UIKit are two powerful frameworks that can help achieve this goal, each offering unique approaches to UI design. SwiftUI is a modern declarative framework that simplifies the process of creating dynamic interfaces, while UIKit offers more control and flexibility with its imperative programming style.
Both frameworks enable developers to craft beautiful, responsive, and easy-to-navigate user interfaces. Understanding the strengths and limitations of each can help choose the right tool depending on the project’s requirements, allowing for efficient design and development processes.
Using SwiftUI for Modern UI Design
SwiftUI provides a straightforward and declarative way to design user interfaces, meaning developers can describe the layout and behavior of the UI with minimal code. It offers several built-in components such as buttons, lists, and text fields that adapt automatically to different screen sizes and orientations. This makes it ideal for creating apps that work across various iOS devices seamlessly.
- SwiftUI simplifies layout with flexible components like VStack and HStack, making vertical and horizontal layouts easier to implement.
- It supports dynamic content changes, automatically updating the interface when data changes through state variables.
- The framework features live previews in Xcode, allowing real-time design changes and faster iteration.
UIKit for Detailed and Custom UI Control
UIKit, on the other hand, offers more granular control over the user interface. It uses an imperative approach where developers need to specify how the UI elements should behave. This can be more complex but provides greater flexibility, especially for creating custom designs or managing complex UI components.
- UIKit supports advanced animations and transitions, enabling developers to implement custom visual effects.
- It allows fine-tuning of UI elements with precise control over their layout, appearance, and interactions.
- UIKit's mature ecosystem means access to numerous third-party libraries and tools that can extend functionality.
To make the most of both frameworks, many developers choose to integrate SwiftUI with UIKit, combining the best of both worlds. For example, SwiftUI views can be embedded in UIKit-based applications, and UIKit components can be used within SwiftUI interfaces.
Comparison Table
Feature | SwiftUI | UIKit |
---|---|---|
Approach | Declarative | Imperative |
Ease of Use | Simple, less code | More control, requires more code |
Customization | Limited, pre-built components | Highly customizable |
Device Compatibility | Automatic for all iOS devices | Customizable for specific devices |
Building Functional Features: Handling User Input, Networking, and Databases
When developing an iOS app, implementing functional features is a crucial aspect of the process. One key area to focus on is the handling of user input, which allows the app to be interactive and responsive to the user's needs. Capturing user input through forms, gestures, or touch events is essential for creating a seamless experience. Another important component is networking, which enables the app to communicate with remote servers or APIs to fetch data or send requests. Finally, databases are used to persist data locally or remotely, ensuring that the app can store and retrieve information efficiently.
In this section, we will explore how to handle these features, including techniques for managing user input, making network requests, and interacting with databases. Each of these aspects plays a significant role in making an app functional and dynamic.
Handling User Input
Handling user input effectively ensures smooth interaction with the app. You can capture different types of user input such as text, touch gestures, and sensor data. To manage this, you can use the following methods:
- Text Input: UITextField and UITextView are commonly used to capture text from the user. They can be customized to accept different types of input, such as email addresses or phone numbers.
- Touch Events: Gesture recognizers, such as tap, pinch, or swipe, are used to capture touch-based input, allowing you to build more interactive interfaces.
- Device Sensors: Using Core Motion, you can capture data from sensors like the accelerometer or gyroscope to respond to user actions.
Networking in iOS
Networking allows an app to communicate with external servers or APIs. iOS provides multiple ways to handle networking tasks, such as making HTTP requests and parsing responses. The following tools are commonly used:
- URLSession: This API handles HTTP requests and responses. You can use it to make GET, POST, PUT, and DELETE requests to fetch or send data.
- JSON Parsing: Once data is fetched, it is typically in JSON format. The Codable protocol is used to decode this data into Swift objects.
- Third-Party Libraries: Alamofire and other libraries can simplify networking tasks, making it easier to manage requests, handle errors, and parse responses.
Working with Databases
To persist data within your app, you can use either local storage solutions or connect to a remote database. Here are the most commonly used options:
Database Type | Description | Use Case |
---|---|---|
Core Data | A powerful framework for managing a local SQLite database. | Use it for complex data models and relational data. |
Realm | An easy-to-use alternative to Core Data with a focus on performance. | Great for handling large data sets with minimal overhead. |
Firebase | A cloud-based database that supports real-time syncing and offline capabilities. | Ideal for apps that require real-time data sharing or synchronization across multiple devices. |
Tip: Always handle user input and network responses asynchronously to avoid blocking the main thread and ensure a smooth user experience.
Debugging and Testing iOS Apps: Strategies for Improving App Quality
Efficient debugging and testing are essential steps in the development cycle of any iOS application. Proper debugging techniques help developers identify and resolve issues early in the development process, ensuring smoother performance. Testing, on the other hand, plays a pivotal role in confirming that the app functions as expected across various devices and scenarios.
By adopting effective strategies for both debugging and testing, developers can greatly enhance the reliability and user experience of their apps. Let’s explore some essential approaches and tools that can significantly contribute to the quality improvement of iOS apps.
Debugging Techniques for iOS Development
Debugging is the process of identifying and fixing errors or bugs within the app code. One of the most important practices during this phase is using the built-in Xcode debugging tools effectively. Xcode’s features such as breakpoints, logging, and memory management tools provide valuable insights into app behavior.
- Breakpoints: They allow developers to pause execution at specific lines of code, enabling them to inspect variable values and app state.
- Console Logs: Using print statements or logging APIs helps track the flow of code and identify unexpected behaviors.
- Instruments: A powerful tool in Xcode, Instruments helps developers analyze memory usage, CPU usage, and detect potential memory leaks.
Testing iOS Apps: Key Approaches
Testing ensures that the app performs well under various conditions and is free of critical bugs. The two main types of testing for iOS apps are unit testing and UI testing.
- Unit Testing: This type of testing focuses on verifying individual components of the codebase, such as functions or classes, to ensure they behave correctly in isolation.
- UI Testing: UI tests simulate user interaction with the app to check whether the interface behaves as expected and to validate that UI elements respond correctly to touch events.
Effective Strategies for Quality Assurance
To maintain high-quality standards, developers should adopt a combination of automated and manual testing techniques. Automated tests can run frequently during the development process, while manual testing ensures the app’s user experience is intuitive and functional across different scenarios.
Testing Type | Focus | Tools |
---|---|---|
Unit Testing | Testing individual components or functions | XCTest |
UI Testing | Simulating user interaction | XCUITest |
Integration Testing | Verifying multiple components working together | Appium |
Effective debugging and thorough testing are the cornerstones of a successful iOS application. By utilizing the right tools and adopting best practices, developers can ensure that their apps provide a seamless and bug-free experience for users.