Cross Platform App Development Technologies
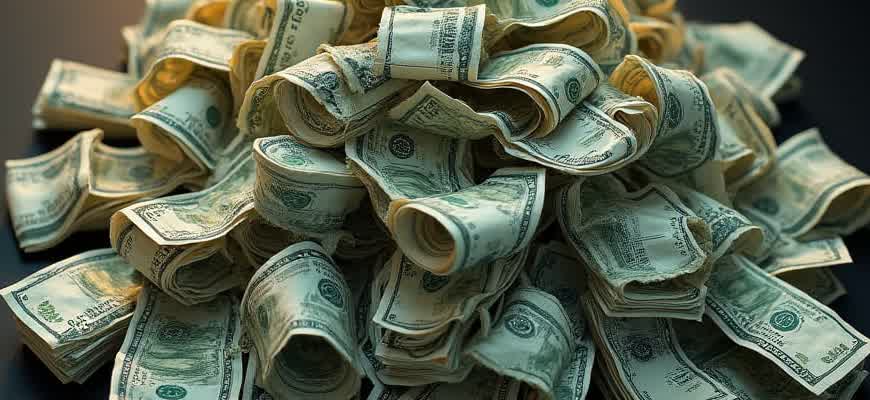
Building applications that run on multiple platforms with minimal effort is a key objective in modern software development. Several frameworks and technologies have emerged, allowing developers to create mobile apps that can function on both Android and iOS devices without duplicating code. Below are some of the most commonly used technologies in cross-platform development:
- Flutter: Developed by Google, it allows for natively compiled applications for mobile, web, and desktop from a single codebase.
- React Native: A popular framework based on React that enables developers to write applications using JavaScript and React.
- Xamarin: A Microsoft-owned technology that enables developers to create cross-platform apps using C# and .NET.
These frameworks provide powerful tools and resources to streamline development, but each has its strengths and weaknesses. To better understand which framework best suits your project, consider the following comparison:
Technology | Primary Language | Performance | Community Support |
---|---|---|---|
Flutter | Dart | High | Growing |
React Native | JavaScript | Moderate | Very High |
Xamarin | C# | High | Good |
Flutter is known for its exceptional performance and native look and feel, while React Native boasts the largest community and extensive libraries.
Choosing the Right Framework for Cross-Platform App Development
When selecting a framework for developing cross-platform applications, developers must consider various factors that can influence the app's performance, maintainability, and user experience across different platforms. A good framework should provide a balance between native performance and the flexibility of code sharing between different operating systems like iOS and Android. The most suitable framework will depend on the specific project requirements, such as the type of app, team expertise, and desired features.
Each framework offers distinct advantages and trade-offs, making it essential to evaluate them based on the technical needs of the project. Key factors like development speed, scalability, access to device features, and community support should be prioritized. Below are some important points to consider when making a choice:
- Performance: Native-like performance is crucial for apps with heavy graphical or computational requirements.
- Code Reusability: The ability to share most of the codebase across platforms reduces time and costs in the long run.
- Developer Tools: Consider the quality of the development tools and libraries that the framework supports.
- Community Support: A strong community can provide valuable resources, tutorials, and troubleshooting help.
Popular cross-platform frameworks include:
- Flutter: Known for its high performance and ability to deliver a near-native experience with a single codebase. It’s ideal for developers aiming for excellent UI consistency across platforms.
- React Native: Offers a rich ecosystem with a wide range of pre-built components. It’s well-suited for teams already familiar with JavaScript and React.
- Xamarin: A Microsoft-backed framework that allows developers to write in C#. Ideal for those targeting both mobile and desktop applications.
"Choosing a framework is not just about the technology. It's about matching the right tool with the specific needs of the project and the skills of your development team."
Here’s a brief comparison of key frameworks:
Framework | Primary Language | Performance | Community Support |
---|---|---|---|
Flutter | Dart | High | Strong |
React Native | JavaScript | Good | Very Strong |
Xamarin | C# | Good | Moderate |
Optimizing Performance in Cross-Platform Apps
Optimizing the performance of cross-platform applications is crucial for delivering a smooth and responsive user experience across different devices and platforms. While cross-platform frameworks like React Native, Flutter, and Xamarin offer a unified codebase, they can sometimes introduce performance challenges due to the need for abstraction and compatibility with multiple operating systems. Developers must use a variety of strategies to ensure that the app performs efficiently without sacrificing features or functionality.
There are several effective approaches for improving the performance of cross-platform applications. The most common methods include minimizing the use of unnecessary resources, optimizing rendering processes, and leveraging native code when possible. Developers should also focus on profiling the app to identify bottlenecks, testing on various devices, and taking advantage of platform-specific performance enhancements.
Key Optimization Techniques
- Code Efficiency - Writing efficient and optimized code reduces the load on the app’s processing power.
- Lazy Loading - Load resources such as images and data only when they are needed, instead of preloading everything at once.
- Memory Management - Proper management of memory usage, such as minimizing memory leaks and reducing large object allocations, helps improve app performance.
Platform-Specific Strategies
While cross-platform frameworks provide a shared codebase, developers can still optimize performance by using native components and platform-specific APIs in certain cases. These optimizations ensure that the app leverages the full capabilities of each platform.
- iOS: Use Metal for rendering complex graphics for improved performance.
- Android: Utilize hardware acceleration and optimize the use of background tasks to minimize CPU usage.
Remember, each platform has its own strengths and weaknesses, so understanding platform-specific behavior is essential for achieving the best performance.
Performance Testing
Regular performance testing is key to ensuring optimal app behavior. Utilizing profiling tools such as Xcode for iOS and Android Profiler for Android can help developers identify areas that need improvement. Testing on a wide range of devices ensures that performance remains consistent regardless of hardware differences.
Platform | Optimization Focus |
---|---|
iOS | Graphics rendering, memory usage |
Android | Battery usage, background processes |
Understanding the Advantages of Reusing Code Across Multiple Platforms
In modern app development, creating applications that function seamlessly on multiple platforms can be a significant challenge. One of the key strategies to address this challenge is through the reuse of code across different platforms. This approach allows developers to write a single codebase that can be deployed on various operating systems and devices. As a result, it reduces the time and resources needed for development and maintenance, leading to greater efficiency in the long term.
Code reusability is especially important for cross-platform development because it minimizes the need for platform-specific adjustments. By leveraging frameworks and tools designed for cross-platform compatibility, developers can ensure that the app functions consistently on different devices without duplicating the effort for each one. This method not only speeds up development but also enhances the overall stability and user experience of the app.
Key Benefits of Code Reusability
- Time Efficiency: Reusing code reduces the need to write separate code for each platform, thus speeding up the development process.
- Cost Reduction: With less time spent on coding and testing, overall development costs decrease.
- Consistency Across Platforms: Reusing code ensures a uniform user experience and feature set on different platforms.
- Easy Maintenance: Since only one codebase is maintained, bug fixes and updates can be implemented across all platforms simultaneously.
Reusable code leads to faster development cycles and more consistent application performance, ultimately contributing to greater business value.
Practical Examples of Code Reusability
- React Native: React Native allows developers to write components that work across both iOS and Android, saving time while maintaining platform-specific performance.
- Flutter: With Flutter, the same codebase can target both Android and iOS, offering a near-native performance and feel on both platforms.
- Xamarin: Xamarin uses a shared codebase written in C# to develop apps for Android, iOS, and Windows, enabling high levels of code reusability across these platforms.
Reusability Comparison
Technology | Platform Support | Code Reusability | Performance |
---|---|---|---|
React Native | iOS, Android | High | Near-native |
Flutter | iOS, Android | Very High | Native |
Xamarin | iOS, Android, Windows | High | Native |
Key Differences Between Native and Cross-Platform App Development
Native app development involves creating software specifically for a particular platform, utilizing the platform's own programming languages and frameworks. In contrast, cross-platform development enables the creation of applications that run on multiple platforms with a single codebase, leveraging frameworks that facilitate compatibility with various operating systems.
Both approaches have their advantages and disadvantages, depending on factors like performance, development speed, and platform-specific features. Below, we compare the key aspects of native and cross-platform development.
Performance
Native apps are typically faster because they are built directly for a specific platform, allowing full utilization of the hardware and OS capabilities. Cross-platform apps, however, may experience slight performance limitations due to the abstraction layer introduced by the framework.
Native apps provide superior performance, particularly for resource-intensive tasks like gaming or high-end graphics processing.
Development Speed
Cross-platform development is often faster because developers write a single codebase that works across multiple platforms. Native app development requires separate codebases for each platform, which can result in longer development times and higher costs.
- Cross-Platform: Single codebase for iOS and Android
- Native: Separate codebase for each platform (e.g., Swift for iOS, Kotlin for Android)
Platform-Specific Features
Native development allows better integration with platform-specific features, offering the ability to fully leverage device capabilities. Cross-platform apps might have limitations in accessing some native features or may require additional workarounds.
For unique platform functionalities like push notifications or background processing, native development provides more flexibility.
Cost Efficiency
While native app development is generally more expensive due to separate codebases, cross-platform development can reduce costs by enabling developers to reuse code across multiple platforms.
Factor | Native Development | Cross-Platform Development |
---|---|---|
Development Cost | Higher | Lower |
Performance | Optimal | May have limitations |
Time to Market | Longer | Faster |
Ensuring Consistency in UI/UX Across Multiple Platforms
In the process of building cross-platform applications, one of the most challenging aspects is maintaining a consistent user interface (UI) and user experience (UX) across various devices. Each platform, whether it’s mobile, tablet, or desktop, presents unique characteristics and limitations, making it essential to design with flexibility while ensuring a cohesive experience. By considering the specific needs of each device, developers can ensure that the application feels native on all screens while maintaining the same functionality and visual consistency.
To achieve this, it is important to establish design principles and guidelines that apply to all platforms while adapting the interface to meet the requirements of each. This can include managing layout adjustments, color schemes, typography, and interaction patterns to ensure they work seamlessly on different screen sizes and orientations. A unified approach ensures that users will have a smooth and predictable experience regardless of the device they are using.
Key Strategies for Managing UI/UX Consistency
- Responsive Design: Implement flexible grid systems and layouts that adjust based on screen size and resolution.
- Component Reusability: Use reusable components that adapt to different screen sizes and functionality across platforms.
- Platform-Specific Guidelines: Follow the native guidelines for each platform (iOS, Android, Web) while maintaining overall consistency.
One of the most effective ways to manage UI consistency is by designing with a modular approach. This involves creating reusable components that adjust to different screen sizes and resolutions. By using the same components across all platforms, developers can ensure that the UI elements look and behave consistently, regardless of where they are displayed.
Design Considerations for Cross-Device Compatibility
- Touch vs. Mouse Input: Ensure that interactive elements are appropriately sized and placed for both touchscreens and mouse navigation.
- Performance Optimization: Test UI components for performance across all platforms to ensure smooth operation without lags or delays.
- Adaptable Typography: Use scalable fonts and adjust text sizes for better readability on various devices.
"A cohesive cross-platform experience is not just about uniformity in design but about maintaining seamless interactions and user satisfaction on any device."
Tools for Streamlining UI Consistency
Tool | Description |
---|---|
Figma | A design tool that enables collaboration and consistency in UI/UX design, offering responsive templates for different platforms. |
Flutter | A framework that allows developers to build native-like experiences across iOS, Android, and Web with consistent UI elements. |
React Native | Helps in building cross-platform mobile applications with reusable UI components and seamless performance. |
Incorporating External Libraries in Multi-Platform Mobile Applications
One of the primary benefits of cross-platform mobile development is the ability to leverage third-party libraries to accelerate development. External libraries provide pre-built solutions to common challenges, allowing developers to focus on core functionalities while saving time on routine tasks. Integrating these libraries, however, requires careful consideration of compatibility, licensing, and maintenance across multiple platforms.
When integrating third-party libraries, it's essential to evaluate the library's support for both iOS and Android. Libraries that offer seamless cross-platform functionality reduce the need for platform-specific customizations, enabling developers to maintain a single codebase while targeting multiple environments. Proper integration strategies, such as managing dependencies and version control, are also crucial for ensuring smooth app performance.
Steps for Successful Library Integration
- Evaluate Compatibility: Ensure the library supports both iOS and Android, and check for any known issues or limitations on each platform.
- Manage Dependencies: Use dependency managers like CocoaPods for iOS and Gradle for Android to streamline integration.
- Testing and Debugging: Thoroughly test the integrated library on both platforms to identify any platform-specific quirks or performance issues.
Common Challenges and Solutions
Challenge | Solution |
---|---|
Version Incompatibility | Regularly update libraries and test new versions to avoid conflicts. |
Platform-Specific Customization | Use conditionals or create platform-specific implementations to handle unique platform behaviors. |
Performance Degradation | Optimize the integration by profiling and addressing bottlenecks introduced by the library. |
Important: Always check the licensing terms of third-party libraries to ensure compliance with your project’s requirements and avoid potential legal issues.
Conclusion
Incorporating third-party libraries into cross-platform apps can significantly speed up development and enhance functionality. However, it is crucial to manage the integration process effectively, considering potential challenges such as compatibility, performance, and version control. By following best practices and carefully evaluating each library, developers can create robust applications that provide an excellent user experience on both iOS and Android platforms.
Testing Approaches for Multi-Platform Mobile Applications
When developing mobile applications that run across multiple platforms, effective testing is crucial to ensure consistent performance and functionality. Cross-platform frameworks allow developers to write code once and deploy it on both iOS and Android, but the testing process requires special strategies to ensure compatibility with different environments. These strategies help address unique challenges, such as device fragmentation, platform-specific features, and varying screen sizes.
A well-rounded testing approach includes functional, usability, performance, and security testing. Each of these areas needs to be covered across different devices and operating systems to ensure a seamless user experience. It’s also essential to consider the interaction between the native code and the cross-platform code to detect any potential issues early on in the development process.
Key Testing Techniques
- Unit Testing: Ensures individual functions and methods work as expected across platforms.
- Integration Testing: Verifies that different parts of the application work together seamlessly.
- UI Testing: Focuses on the user interface and checks for consistency across different devices and screen sizes.
- Performance Testing: Evaluates how the app performs under different conditions, such as varying network speeds or limited device resources.
- Security Testing: Ensures that the application handles sensitive data securely across all platforms.
Testing Tools for Cross-Platform Apps
Tool | Purpose | Supported Platforms |
---|---|---|
Appium | Automated UI testing | iOS, Android, Windows |
Flutter Test | Unit and widget testing | iOS, Android |
Detox | End-to-end testing for React Native | iOS, Android |
BrowserStack | Cross-device testing on real devices | iOS, Android |
"Effective testing strategies are essential for ensuring that a cross-platform application meets the quality standards expected by end users. Incorporating various testing tools helps streamline the process and improves the reliability of the app."
Cost Considerations When Developing Cross Platform Apps
Developing cross-platform applications involves several factors that can influence the overall cost. While the ability to deploy an app across multiple platforms with a single codebase offers long-term savings, there are still significant upfront costs associated with choosing the right development tools, frameworks, and resources. Additionally, choosing between a hybrid app or a more native-like solution can have a direct impact on the project budget and timeline.
When evaluating the financial implications of cross-platform development, it's essential to take into account not only the development time but also the costs associated with ongoing maintenance, updates, and potential scalability needs. Below are some of the primary factors that contribute to the cost of cross-platform app development.
Key Cost Factors
- Development Tools and Frameworks: The choice of development framework (e.g., React Native, Flutter, Xamarin) can influence the overall cost. Some frameworks offer more out-of-the-box functionality, while others may require additional plugins or third-party libraries, increasing both initial and ongoing expenses.
- Development Team Expertise: Hiring developers with experience in specific cross-platform technologies may come at a premium. The complexity of the project can also affect the team size and skill set required.
- Design and User Experience: Cross-platform apps often need additional attention in terms of design to ensure consistent performance and user experience across various platforms, which can add to the development costs.
- App Testing: Testing on multiple devices and operating systems can be resource-intensive. The cost can rise depending on the number of platforms the app needs to support and the depth of testing required.
Additional Considerations
Note: While cross-platform development can reduce costs in the long run by minimizing the need for separate native apps, developers must still ensure that the app performs well on all target devices and platforms.
Moreover, businesses should also consider long-term costs associated with app updates, bug fixes, and feature enhancements. Depending on the framework and architecture chosen, some updates may require substantial rework. The decision to use a cross-platform approach should weigh not just initial development costs but also long-term maintenance and flexibility needs.
Factor | Impact on Cost |
---|---|
Framework Choice | Can reduce development time but may require additional plugins or third-party libraries. |
Developer Expertise | Highly skilled developers are generally more expensive, but the quality of code and efficiency increases. |
Testing | Higher number of devices and platforms requires more resources for comprehensive testing. |
Maintenance | Cross-platform apps often require ongoing updates, which may involve rework for specific platforms. |