Barcode Scanner App Development
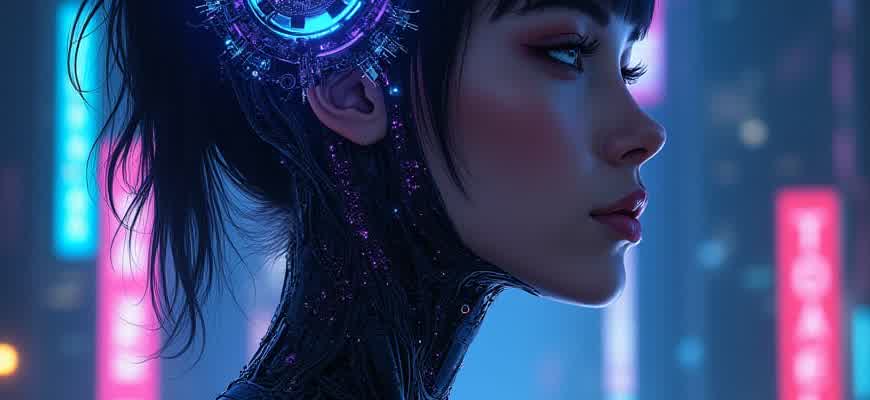
Creating an application for scanning barcodes involves integrating optical character recognition (OCR) with mobile devices. This technology enables users to easily scan product codes, retrieve information, and perform actions like purchasing, tracking, or analyzing data. The process begins with selecting an appropriate framework and ensuring compatibility with various devices, operating systems, and barcode types.
The development process can be broken down into the following key stages:
- Choosing the platform (iOS, Android, or cross-platform).
- Integrating the camera functionality with barcode scanning libraries.
- Ensuring accurate decoding of multiple barcode formats.
- Developing a user interface that enhances the scanning experience.
Key considerations for optimal barcode scanning: Performance, accuracy, and support for various barcode types are crucial for a successful app.
Barcode scanning apps must support different types of barcodes, each requiring specific decoding mechanisms. Below is a table outlining common barcode formats and their characteristics:
Barcode Format | Typical Use | Supported Devices |
---|---|---|
QR Code | URL and product information | Smartphones, tablets |
UPC | Retail products | POS systems, scanners |
EAN | Product identification | Retail scanners |
Choosing the Optimal Tech Stack for a Barcode Scanning Application
When developing a barcode scanning application, selecting the appropriate technology stack is critical for ensuring both performance and compatibility across different devices. Developers need to balance several factors, including ease of integration, scanning efficiency, and support for different types of barcodes. The right choice will depend on the specific needs of the project, such as platform preference (iOS, Android, or cross-platform), the speed of data processing, and user interface considerations.
The technology stack must also take into account the preferred programming languages and frameworks, as well as libraries and APIs for barcode recognition. Performance optimization is key to providing a smooth scanning experience, ensuring that the app can quickly read barcodes even in challenging conditions like low light or blurred images. It's also important to consider future scalability and maintenance requirements when choosing your stack.
Key Technologies for Barcode Scanning Apps
- Programming Languages: Java/Kotlin (Android), Swift/Objective-C (iOS), Dart (Flutter)
- Libraries and APIs: ZXing, ZBar, Scandit, ML Kit
- Frameworks: React Native, Flutter
- Barcode Formats: QR Codes, UPC, EAN, DataMatrix
Commonly Used Barcode Scanning Libraries
Library | Platform Support | Key Features |
---|---|---|
ZXing | Android, iOS | Supports a wide variety of barcode formats, open-source, easy integration |
ZBar | Android, iOS | Lightweight, good for low-powered devices |
Scandit | Android, iOS | High-performance, supports 1D/2D barcodes, accurate in challenging environments |
ML Kit | Android, iOS | Google's machine learning tools, barcode scanning with fast recognition |
Choosing the right combination of libraries and frameworks is crucial for balancing performance and user experience. Popular choices like ZXing or Scandit provide great versatility but may require more resources, whereas lightweight options like ZBar may be better suited for simpler applications or devices with limited hardware capabilities.
Important Considerations
- Platform-Specific Optimization: Tailor your stack to the target platform for better performance.
- Library/Framework Compatibility: Ensure libraries work well with your chosen tech stack.
- Performance & Speed: Minimize latency for fast and accurate barcode scanning.
- Future Scalability: Choose technologies that can scale as your app grows or adds features.
Integrating Various Barcode Types: Challenges and Solutions
Barcode scanning apps need to support a variety of formats to accommodate different industries, such as retail, logistics, and healthcare. This variety can pose significant challenges when it comes to ensuring compatibility, fast detection, and accurate data extraction. Each barcode type, from traditional 1D formats to modern 2D codes like QR, has its own encoding schemes and data storage methods, which can complicate integration efforts. For developers, it's essential to address these challenges to provide a seamless user experience across various formats.
One of the main obstacles is the differences in the structure and complexity of barcode formats. A traditional 1D barcode typically contains limited information, while 2D codes like QR codes and Data Matrix can store much larger amounts of data in a smaller area. Therefore, the app needs to differentiate between these formats and apply the correct decoding algorithms. Below are the key issues developers face and potential solutions to address them.
Key Challenges and Solutions
- Decoding Speed: Different formats require different decoding techniques, affecting speed and accuracy.
- Compatibility: Ensuring the scanner works across various devices and operating systems can be tricky.
- Visual Quality: Poor lighting or blurred barcodes can hinder scanning, especially for 2D codes.
Solutions
- Cross-format Libraries: Use libraries that support multiple barcode types, such as ZXing or ZBar, to unify the scanning process.
- Adaptive Algorithms: Implement algorithms that adjust to barcode quality, lighting, and format automatically.
- Regular Updates: Regularly update the scanning algorithms to include new formats and improve existing ones.
"Developing a barcode scanner app is not just about supporting more formats but ensuring the app works seamlessly under diverse conditions."
Barcode Type | Data Capacity | Common Use Cases |
---|---|---|
1D Barcodes | Up to 20 characters | Retail, Logistics |
QR Codes | Up to 4000 characters | Marketing, Mobile Payments |
Data Matrix | Up to 2,000 characters | Healthcare, Aerospace |
Enhancing Barcode Scanning Performance on Mobile Devices
To ensure a seamless experience in barcode scanning applications, both speed and accuracy are crucial. The challenges of optimizing scanning performance arise due to the diversity in mobile hardware and environmental factors such as lighting and distance from the barcode. Developers must implement strategies that adapt to these variables while delivering fast and accurate results.
Achieving a balance between scanning speed and precision requires a mix of software optimizations, hardware utilization, and user interface adjustments. The following techniques can significantly enhance scanning efficiency.
Techniques for Improving Speed and Accuracy
- Camera Resolution and Focus Optimization: Ensure the camera’s resolution is adequate for clear barcode capture. Implement auto-focus algorithms that adjust in real-time to maintain clarity.
- Image Preprocessing: Use algorithms to filter out noise and enhance the contrast, making it easier to detect barcodes in various lighting conditions.
- Barcode Detection Algorithm Optimization: Choose or develop fast algorithms that can quickly locate barcodes within an image, such as those based on machine learning or edge detection techniques.
Strategies for Handling Environmental Variables
- Adaptive Lighting Compensation: Adjust the brightness and contrast in real-time to optimize barcode visibility, especially in low-light environments.
- Barcode Size and Distance Calibration: Adjust the scanning range to accommodate different barcode sizes and user distances from the target.
- Fast Retry Mechanisms: Implement retry logic when the first scan attempt fails, allowing for multiple attempts without significant delay.
Properly handling environmental factors and leveraging advanced algorithms is key to reducing scan failures and ensuring a smooth user experience.
Key Metrics for Evaluating Performance
Metric | Description | Impact on Performance |
---|---|---|
Scan Time | Time taken to recognize and decode the barcode. | Directly affects user experience; faster scan times lead to better app usability. |
Scan Accuracy | Percentage of successful scans relative to total attempts. | Higher accuracy reduces user frustration and the need for manual corrections. |
Environmental Adaptability | App's ability to function in varying lighting and distance conditions. | Improves reliability across different scanning scenarios. |
Handling Real-Time Data Processing in Barcode Scanner Apps
Real-time data processing plays a crucial role in the functionality of barcode scanner applications. As the user scans a barcode, the app must instantly interpret the data and provide immediate feedback or take action based on the result. This process involves various steps, such as decoding the barcode, cross-referencing it with a database, and updating the user interface in real time. Achieving seamless and fast data processing is vital to ensure a smooth user experience and avoid delays that could impact the app's effectiveness.
Efficient real-time data handling in barcode scanning apps involves optimizing performance across several layers, including image processing, decoding algorithms, and network communication. The system must be designed to handle multiple inputs without lag and ensure quick interaction between the scanner and external data sources. Below are some key aspects that influence the performance of real-time data processing in barcode scanner apps:
Key Considerations for Real-Time Data Handling
- Barcode Decoding Speed: The app must decode the barcode within milliseconds to keep the user experience smooth.
- Network Latency: When the app fetches data from a remote server, minimizing network delays is essential for fast responses.
- Multithreading: Utilizing multiple threads to handle background tasks allows for more efficient processing of data and camera input simultaneously.
To optimize real-time processing, developers often use advanced algorithms and hardware acceleration techniques. For instance, QR codes can be processed much faster with hardware-supported features such as GPU utilization. Furthermore, employing machine learning models for image recognition helps reduce errors and improve decoding accuracy.
Important Tip: Prioritize local data storage or caching techniques to ensure offline functionality, especially when network access is intermittent.
Common Challenges in Real-Time Data Processing
- Data Synchronization: Keeping data synchronized between the barcode scanner and the backend database can be tricky, especially with large volumes of real-time transactions.
- Device Performance: Mobile devices have limited computational power, which can hinder real-time processing if not properly optimized.
- Error Handling: Unexpected errors during scanning or data retrieval need to be handled gracefully to prevent app crashes or poor user experience.
Data Flow in Real-Time Barcode Scanning
Step | Action | Performance Impact |
---|---|---|
Scanning | Barcode is captured using the device's camera. | High resolution and fast frame rates lead to better accuracy. |
Decoding | Decoding of the barcode to extract data. | Efficiency of decoding algorithm directly affects processing time. |
Data Retrieval | App fetches product or user information from a database. | Network speed and local caching can significantly reduce delay. |
UI Update | Displaying the retrieved information on the app's interface. | Fast UI updates keep the experience responsive and engaging. |
Designing Intuitive Interfaces for Barcode Scanning Applications
Creating a user-friendly interface for barcode scanning apps is essential for providing a seamless experience. It should focus on simplicity, speed, and accessibility, ensuring that users can scan items quickly without confusion. A streamlined design improves the app's efficiency, and user interactions should be intuitive, requiring minimal input. Emphasis should be placed on visual clarity, ensuring all elements are easily distinguishable and functional on both small and large screens.
The interface must guide users through the scanning process with minimal steps. The visual hierarchy should be clear, and the scanning process itself should be straightforward, with minimal distractions. Furthermore, accessibility features must be incorporated, allowing users of all abilities to navigate the app with ease.
Key Considerations for Designing Barcode Scanning Interfaces
- Clear Scanning Area: The scanning area should be clearly marked and easily identifiable. Users should instantly know where to position the barcode.
- Real-Time Feedback: Provide instant visual or auditory feedback when a barcode is successfully scanned to confirm the action has been completed.
- Minimalist Design: Avoid cluttering the screen with unnecessary buttons or features. Focus on the most important elements of the scanning process.
- High Contrast and Readable Fonts: Ensure that text is legible with a high contrast background. The use of clear fonts makes the app easier to use in various lighting conditions.
Best Practices for Enhancing User Experience
- Quick Launch Time: Users expect the app to start scanning immediately after launching. Long load times or unnecessary screens can lead to frustration.
- Wide Barcode Compatibility: Ensure the app can scan various types of barcodes (QR, UPC, EAN) without issues, as different industries may use different standards.
- Minimal User Input: If possible, avoid requiring the user to enter information manually after scanning. Autofill data is preferable.
Important Usability Tips
Aspect | Recommended Action |
---|---|
Scanning Speed | Optimize the scanning process to be quick and efficient, reducing the time spent waiting for a successful scan. |
Accessibility Features | Implement voice commands and screen reader support to make the app more accessible to users with disabilities. |
Multilingual Support | Include language options for global users, ensuring the app is usable in different regions. |
Tip: User feedback should be gathered continuously to improve the interface and ensure that the app meets the expectations of its target audience.
Security Aspects in Barcode Scanner Application Development
Barcode scanner apps are integral to many industries, providing essential functionality for inventory management, logistics, and retail operations. However, security risks associated with barcode scanning must be taken into account during the development process. Sensitive data transmitted through scanned barcodes can be vulnerable to interception, manipulation, and misuse if not properly secured. Developers must implement measures to safeguard both the scanned data and the app’s overall integrity.
One of the key security considerations is ensuring that the barcode data is processed securely and protected from external threats. This includes encrypting the data, using secure communication channels, and validating the integrity of the scanned data to prevent attacks such as code injection or data spoofing. Additionally, the app should implement strict user authentication to control access to sensitive features.
Key Security Measures
- Data Encryption: All scanned barcode data should be encrypted during transmission to avoid unauthorized access. This is particularly important when transmitting sensitive customer or financial information.
- Secure Storage: Data stored on the device must be encrypted as well. Developers should ensure that barcode data is stored in a secure, isolated environment to prevent unauthorized access from other applications.
- Authentication and Access Control: Implement robust user authentication methods to limit access to sensitive features. Consider multi-factor authentication (MFA) for additional security.
Common Security Risks in Barcode Scanner Apps
- Data Interception: Without proper encryption, data transmitted from the scanner to the app or server can be intercepted by malicious actors.
- Barcode Spoofing: Attackers could use counterfeit barcodes to mislead the app, potentially leading to fraudulent transactions or inventory errors.
- Injection Attacks: Unfiltered or improperly validated input from barcode data may allow attackers to inject harmful code into the system, compromising the app's security.
"Security must be considered from the very start of the app development process. A barcode scanner is only as secure as the measures in place to protect the data it processes."
Security Best Practices
Security Feature | Benefit |
---|---|
End-to-End Encryption | Protects sensitive data from being accessed or tampered with during transmission. |
Secure Data Storage | Prevents unauthorized access to sensitive data stored locally on the device. |
Regular Software Updates | Fixes potential vulnerabilities and keeps the app secure from newly discovered threats. |
Testing and Debugging Barcode Scanning Features on Different Devices
When developing a barcode scanning application, it's essential to thoroughly test the scanning functionality across various devices. Different devices may have varying camera qualities, screen sizes, and processing capabilities that can impact the performance of barcode scanning features. Therefore, ensuring that your app performs well on both high-end and budget devices is crucial to providing a seamless user experience.
To effectively test and debug the barcode scanning features, developers must pay attention to multiple factors, including the quality of the camera, the operating system version, and device-specific hardware components. Since barcode scanning is highly dependent on the camera’s ability to focus and capture clear images, testing on various devices with different camera resolutions and features is necessary to identify and resolve issues before the app is released.
Key Testing Considerations
- Camera resolution and autofocus capabilities
- Different screen sizes and their impact on barcode display and scanning
- Operating system versions and compatibility with scanning libraries
- Hardware-specific limitations (e.g., processing power and memory)
Testing Process
- Test on a range of devices with different camera specifications.
- Verify that barcodes are recognized quickly and accurately across devices.
- Ensure the app responds correctly to different lighting conditions and angles.
- Test with various barcode types (QR codes, UPC, EAN, etc.) to ensure compatibility.
- Monitor performance to ensure the app doesn't crash or become unresponsive during scanning.
Important: Always perform tests on real devices rather than relying solely on emulators, as real-world testing provides more accurate results for scanning behavior.
Common Debugging Strategies
Issue | Possible Solution |
---|---|
Slow scanning speed | Optimize the barcode detection algorithm and reduce unnecessary background processes. |
Barcode not recognized | Ensure the barcode is properly lit and positioned within the camera’s field of view. Use higher-quality libraries for better recognition. |
App crashes on specific devices | Check for memory leaks and perform stress testing to identify potential performance issues. |