Ios App Development Projects for Beginners
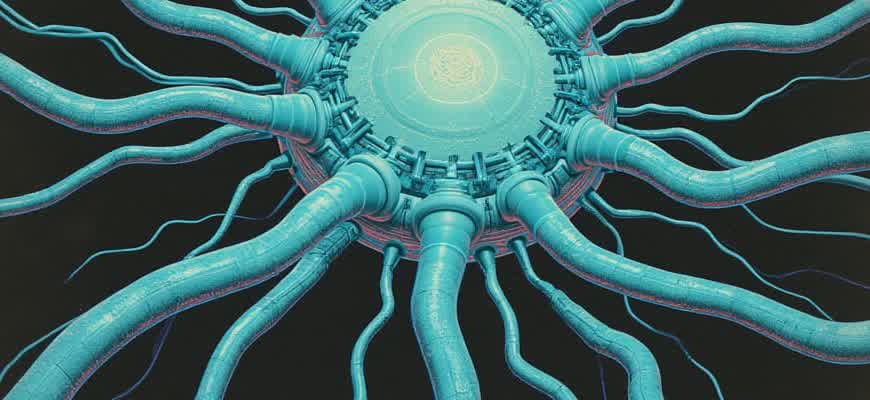
Starting with iOS app development can be overwhelming, but by focusing on small and manageable projects, beginners can gradually build their skills. These projects help in understanding the fundamentals of Swift, Xcode, and the iOS framework. Here's a breakdown of a few essential projects that can be a great starting point.
Key Concepts to Master:
Learning the basics of Swift, understanding UI elements, and getting hands-on experience with Xcode's interface are foundational steps.
- Understanding of MVC architecture in iOS
- Familiarity with UIKit framework
- Core concepts of Swift like Optionals, Arrays, and Functions
Beginner-Friendly Project Ideas:
- Simple To-Do List App: Learn how to manage user input, display lists, and save data locally.
- Weather Forecast App: Practice working with APIs and displaying dynamic content based on real-time data.
- Currency Converter App: Focus on basic mathematical calculations and UI design.
Project Difficulty Table:
Project | Difficulty Level | Key Learning Areas |
---|---|---|
To-Do List | Beginner | Basic UI Elements, Data Storage, Table Views |
Weather App | Intermediate | API Integration, Networking, JSON Parsing |
Currency Converter | Beginner | UI Design, User Input Handling, Basic Calculations |
How to Begin Your First iOS App Project Without Prior Experience
Starting your first iOS app can seem daunting, but with the right approach, it can be an exciting and rewarding experience. By breaking down the process into manageable steps, even beginners can successfully create their first app. This guide will help you get started with the basic tools, concepts, and strategies to avoid feeling overwhelmed during the process.
Before diving into coding, it’s important to understand the development environment and tools that you’ll be using. Apple’s development ecosystem includes Xcode, which is the main Integrated Development Environment (IDE) for iOS app creation. You’ll also need to learn Swift, Apple's programming language, which is designed to be beginner-friendly yet powerful.
Step-by-Step Approach to Start Your iOS App Development
- Set up Xcode: Download and install Xcode from the Mac App Store. This will be your primary tool for building iOS applications.
- Learn Swift Basics: Familiarize yourself with Swift programming language. Focus on basic concepts like variables, loops, functions, and control flow.
- Understand the Interface Builder: Xcode comes with a visual tool called Interface Builder, which helps you design the user interface (UI) without needing to write extensive code.
- Start with a Simple App: Begin with something basic, like a to-do list or a simple calculator. This allows you to focus on core concepts like handling user input and managing data.
- Use Online Resources: Take advantage of tutorials and forums where you can get help from the developer community. Apple’s documentation is also a great resource for learning.
Key Concepts to Focus On
Understanding MVC (Model-View-Controller): This is a design pattern used in iOS development to separate data management (Model), the user interface (View), and the business logic (Controller). Learning how to apply MVC will make your code more organized and scalable.
- Model: Handles data and business logic.
- View: The user interface elements like buttons, text fields, and labels.
- Controller: Manages interaction between the Model and the View.
Additional Tips for Success
Tip | Description |
---|---|
Practice Regularly | Consistent coding will help you improve your skills and speed up the learning process. |
Start with Templates | Using Xcode templates for common app types can help you avoid starting from scratch. |
Debugging Skills | Learn how to use Xcode’s debugging tools to troubleshoot errors in your app. |
Choosing the Right Tools and Frameworks for Beginner iOS Developers
For newcomers to iOS app development, selecting the right tools and frameworks is crucial for success. While there are numerous options available, some are better suited for beginners, offering simplicity and comprehensive support. The goal is to choose tools that allow you to learn quickly, while also providing flexibility as your skills grow. Below are some key considerations when deciding which tools to use for building iOS apps.
It’s important to start with the essential software that powers the development process. The core environment for iOS development is Apple's Xcode, which is specifically designed for building apps for iPhones, iPads, and other Apple devices. Other supplementary frameworks like SwiftUI or UIKit will also shape the way your apps function and look. Understanding the strengths of each can help streamline your development journey.
Essential Tools for iOS App Development
- Xcode: The primary Integrated Development Environment (IDE) for building iOS apps. It includes everything you need to create, test, and debug your app.
- Swift: Apple's programming language, designed to be safe, fast, and easy to use. It’s the standard for modern iOS app development.
- SwiftUI: A user interface toolkit that uses declarative syntax, making it easier to design apps with less code. It’s ideal for beginners who want to quickly prototype apps.
- UIKit: The older framework for building UIs, offering more control over the app’s interface. It’s still widely used and beneficial for learning how app interfaces are structured.
Choosing the Right Frameworks
- SwiftUI vs. UIKit: SwiftUI is easier for beginners because it simplifies UI design with declarative syntax, while UIKit offers more control for complex UI elements.
- Third-Party Libraries: Frameworks like Alamofire (for networking) or Realm (for databases) can help speed up development, but beginners should focus on the core tools first.
Remember, the key is not to overwhelm yourself with too many frameworks or tools. Start with the basics and gradually explore other options as your skills improve.
Summary of Tools and Frameworks
Tool/Framework | Use Case | Suitability for Beginners |
---|---|---|
Xcode | Primary development environment for iOS | Essential |
Swift | Programming language for iOS apps | Highly recommended |
SwiftUI | UI design with declarative syntax | Great for beginners |
UIKit | Traditional UI design | Good for advanced control |
Step-by-Step Guide to Setting Up Xcode for Your First App
Before diving into app development, you need to set up Xcode, Apple's integrated development environment (IDE), on your Mac. Xcode is essential for building iOS applications, as it provides all the tools needed for writing code, designing interfaces, and debugging. In this guide, we will walk you through the process of setting up Xcode, step by step, so you can begin your journey into iOS app development.
Once you have Xcode installed, you can create your first app project. This guide will cover the basic steps, including downloading Xcode, setting up a new project, and running it on your iOS simulator. Let’s go over the necessary steps to get started.
Installing Xcode
To begin, you’ll need to install Xcode from the Mac App Store. Here's how to do it:
- Open the Mac App Store on your Mac.
- Search for Xcode in the search bar.
- Click the Get button to start the download.
- Once downloaded, open Xcode from your Applications folder.
After installation, Xcode will need to install additional components, which will be done automatically when you first open the app. It may take a few minutes, so be patient.
Creating a New Project
Once Xcode is installed and set up, you can start a new project:
- Open Xcode and select Create a new Xcode project from the welcome screen.
- Choose a template for your app. The App template is a good starting point for beginners.
- Enter a name for your project, choose a location to save it, and click Create.
Now, you have a basic app project set up, and you’re ready to start coding!
Running Your App on the Simulator
To see your app in action, you can run it on the iOS simulator that comes with Xcode:
- In the toolbar, select a simulator device (e.g., iPhone 14).
- Click the Play button (or press Command + R) to build and run your app.
- Wait for the simulator to launch, and you should see your app running on the virtual device.
Tip: Always test your app on the simulator before running it on a physical device. This helps you identify errors early in the development process.
Summary of Key Steps
Step | Description |
---|---|
Download Xcode | Install Xcode from the Mac App Store. |
Create a New Project | Start a new project using the App template. |
Run the App | Test your app on the iOS simulator. |
Following these steps will allow you to set up Xcode and start your first iOS app project. Don’t forget to explore the vast features Xcode offers to enhance your development experience.
Designing Effective User Interfaces: Key Principles for Aspiring iOS Developers
Creating user-friendly interfaces is a critical skill for new iOS developers. A well-designed app can greatly enhance user experience, while a poorly designed one can drive users away. When building interfaces for iOS apps, developers should focus on clarity, simplicity, and intuitive navigation. These principles should guide the layout, controls, and interactions throughout the app.
To help you navigate the design process, it's important to follow a set of best practices that align with iOS's Human Interface Guidelines. These guidelines are specifically created to ensure consistency across apps and improve usability. Below are some essential tips that can serve as a foundation for your design journey.
1. Simplify Layouts and Navigation
Effective layout design is crucial to user experience. Keep these points in mind:
- Minimize cognitive load: Avoid cluttered screens with too many elements. Stick to the necessary components to help users focus on the task at hand.
- Use consistent navigation: Users should easily understand where they are in the app. Utilize navigation bars, tabs, and swipe gestures to ensure smooth transitions.
- Follow platform standards: iOS apps should leverage standard navigation patterns like bottom tabs or side menus to maintain familiarity.
2. Design for Touch Interaction
When designing for iOS, it’s important to consider how users will interact with your app. Touch gestures, such as taps, swipes, and pinches, should feel natural. To optimize for touch interactions:
- Make interactive elements large enough for easy tapping.
- Ensure sufficient spacing between buttons and controls to prevent accidental taps.
- Use visual feedback like button highlighting or animations to show users their actions have been registered.
3. Use Consistent and Readable Typography
Typography is a key factor in ensuring readability and consistency within your app. Follow these guidelines:
Tip | Reason |
---|---|
Use standard fonts like San Francisco | It’s optimized for clarity and legibility across different screen sizes. |
Maintain appropriate font sizes | Ensure text is legible on all devices, especially for accessibility purposes. |
Tip: Keep headings large and clear to structure your content effectively. Also, provide contrast between text and background to enhance visibility.
Understanding Swift: Essential Concepts for iOS Development
Swift is Apple's primary programming language for developing iOS applications. It was introduced to replace Objective-C with a more modern, safer, and more efficient approach. Understanding Swift’s core concepts is essential for any beginner aiming to develop iOS apps. This guide focuses on the fundamental elements of Swift that will help you get started on your app development journey.
Before diving into complex app features, mastering basic syntax, data structures, and the language's core principles will set a strong foundation. Here are some key concepts you must familiarize yourself with to write effective Swift code.
Core Elements of Swift
- Variables and Constants: Variables hold values that can change, while constants store values that remain unchanged throughout the program.
- Data Types: Swift supports several built-in types like Int, String, Bool, and Double, each of which defines the kind of data a variable or constant can store.
- Control Flow: Conditional statements like if, else, and loops like for and while are crucial for controlling the execution of code based on certain conditions.
- Functions: Functions encapsulate reusable code. They can take inputs (parameters) and return outputs (return values).
Key Swift Features
Swift is designed with a strong emphasis on safety, performance, and expressiveness, which ensures fewer runtime errors and better readability.
- Optionals: Swift uses optionals to handle cases where a value might be absent. An optional can either hold a value or be nil.
- Closures: Closures are self-contained blocks of functionality that can be passed around and used in your code. They are similar to functions but can capture and store references to variables and constants.
- Protocols: A protocol defines a blueprint of methods and properties that can be adopted by classes, structures, and enums. This is used for creating reusable and flexible components.
Swift Data Structures
Data Structure | Description |
---|---|
Arrays | A collection of values ordered by index. |
Dictionaries | A collection of key-value pairs. |
Sets | An unordered collection of unique values. |
Grasping these key Swift features is crucial for building iOS applications effectively. Mastery over these concepts will lead to cleaner, safer, and more maintainable code in your projects.
Building Your First iOS App: Simple Projects to Build Confidence
Starting with iOS app development can feel overwhelming, but creating small, manageable projects is an excellent way to gain confidence. By focusing on simple tasks, you can gradually build up your skills without getting lost in complex frameworks and designs. These beginner projects offer hands-on experience with Swift and Xcode, allowing you to learn by doing. Whether you're creating a basic interface or integrating simple functionality, each step helps you grow as a developer.
When you're just getting started, choose projects that allow you to practice core concepts like UI layout, user input handling, and data management. The key is to pick projects that are simple enough to complete but challenging enough to push your limits. With each project, you'll improve your problem-solving skills and become more comfortable with the iOS development ecosystem.
Simple Projects to Get Started With
- To-Do List App - A great first project for learning how to manage user input and display dynamic content. You'll work with table views, basic data storage, and user interaction.
- Weather App - This project will help you integrate APIs and handle asynchronous data. You'll also practice working with UITableViews and displaying data in a user-friendly way.
- Simple Calculator - A straightforward project that introduces basic UI components and event handling, making it a perfect choice for beginners.
Key Concepts to Learn
- UI Elements - Master how to work with buttons, labels, text fields, and other common interface components.
- View Controllers - Understand how to manage different screens within your app and navigate between them.
- Swift Fundamentals - Grasp core programming concepts like variables, functions, and conditionals in Swift.
Helpful Resources for Beginners
Resource | Purpose |
---|---|
Apple Developer Documentation | Comprehensive guide for everything related to iOS development, from Swift syntax to advanced topics. |
Swift Playgrounds | An interactive learning environment that allows you to experiment with Swift code in a fun and engaging way. |
Udemy/iOS Development Courses | Structured courses with step-by-step guidance on creating iOS apps from scratch. |
"The journey of building your first app can be challenging, but it's also incredibly rewarding. Stick with it, and don't be afraid to ask for help along the way."
How to Test Your iOS Application on Real Devices: A Step-by-Step Guide for Beginners
Testing your iOS app on real devices is crucial for understanding how it performs in a real-world environment. While using the iOS Simulator is helpful, testing on physical devices ensures that your app runs smoothly across different hardware configurations. This guide will walk you through the process of testing your app on an actual iPhone or iPad.
There are multiple ways to deploy your app on a physical device. The most common method involves using Xcode, Apple's integrated development environment (IDE). In this guide, we'll focus on how to set up and run your app on a device for the first time, ensuring you get a realistic sense of performance, user experience, and device-specific behavior.
Steps to Test Your App on a Physical Device
- Enroll in the Apple Developer Program
- Before testing on a real device, you need to be a part of the Apple Developer Program. This allows you to sign apps and run them on physical devices.
- The membership costs $99 per year.
- Connect Your Device to Xcode
- Use a USB cable to connect your iPhone or iPad to your Mac.
- In Xcode, select your device from the device list in the top toolbar.
- Trust the Developer Certificate on Your Device
- Go to the Settings app on your device, then navigate to General > Device Management.
- Tap on your developer certificate and trust it to allow your app to run.
- Build and Run the App
- In Xcode, click on the "Run" button to deploy your app to the connected device.
- Once the app installs, you can start testing it immediately on your phone or tablet.
Things to Keep in Mind During Testing
Aspect | Consideration |
---|---|
App Performance | Check for any slowdowns or lag when using your app on a physical device compared to the simulator. |
Device-Specific Features | Test features like camera access, location services, and push notifications to ensure they work as expected on real hardware. |
Battery Usage | Monitor your app's impact on battery life to avoid draining the device too quickly. |
Tip: Always test on different devices (e.g., iPhone 12, iPhone SE, etc.) to ensure compatibility across various screen sizes and hardware configurations.
Deploying Your First iOS App to the App Store: Key Considerations
Releasing your first iOS app on the App Store is an exciting milestone, but it's important to understand the deployment process to ensure a smooth launch. Before submitting your app, there are a few steps you need to follow, including ensuring your app meets the App Store's guidelines, preparing necessary assets, and configuring the right settings in Xcode. Only after meeting all these requirements can you begin the submission process. Below is a guide to walk you through the essential stages.
Once your app is ready for release, you will need to set up your App Store Connect account. This platform will allow you to manage your app's details, monitor analytics, and perform app updates. It's also important to ensure that your app follows Apple’s review guidelines and contains no violations that could lead to rejection. Keep reading to understand the major steps involved in this process.
Steps for App Store Submission
- Prepare Your App for Release
- Ensure your app is free of bugs and optimized for performance.
- Update your app’s version number and build number in Xcode.
- Test your app on multiple devices and OS versions to ensure compatibility.
- Setup in App Store Connect
- Create a new app listing with detailed metadata such as title, description, and keywords.
- Upload app screenshots, icons, and other media assets required for App Store visibility.
- Submit for Review
- Upload your app via Xcode to App Store Connect.
- Fill in additional information such as app privacy policies and terms of use.
- Monitor the Review Process
- Be ready to address feedback or issues pointed out by Apple’s review team.
- In some cases, you may need to fix bugs or provide additional information for approval.
- App Approval and Launch
- Once your app is approved, you can release it on the App Store or schedule its release date.
- Monitor app performance and user feedback after launch.
Important: Make sure you carefully read Apple’s App Store Review Guidelines to avoid common rejection reasons like insufficient app functionality or violations of privacy policies.
Common Submission Issues
Issue | Solution |
---|---|
App Crashes on Launch | Test on real devices and fix crashes before submission. |
Missing App Metadata | Ensure all fields like app description, screenshots, and keywords are filled in. |
Rejected for Violations | Review Apple's guidelines and make necessary adjustments to comply with policies. |