Ios App Development Guidelines
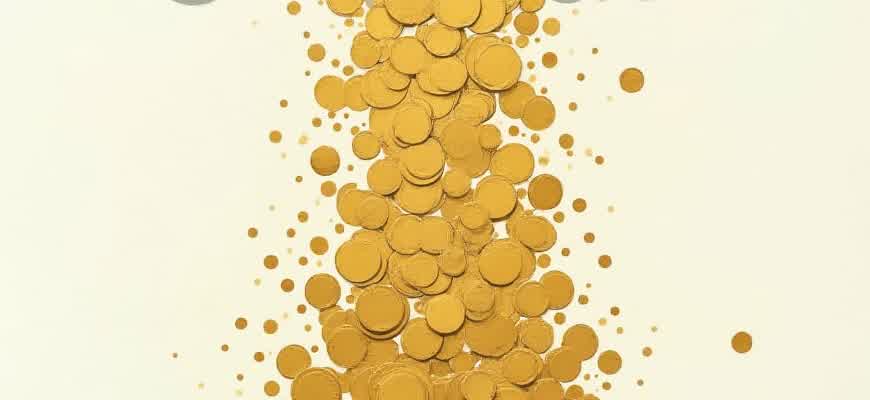
Developing a high-quality iOS app involves adhering to a set of structured guidelines to ensure functionality, usability, and compatibility with Apple's ecosystem. Below are key aspects of iOS app development:
- Interface Design - Focus on simplicity and intuitive user interaction.
- Performance Optimization - Ensure the app runs smoothly across a variety of devices.
- Security - Prioritize secure data handling and privacy.
The following table summarizes the core principles for building an effective iOS app:
Principle | Explanation |
---|---|
Consistency | Ensure UI elements follow Apple's Human Interface Guidelines for a seamless user experience. |
Efficiency | Optimize resource usage to prevent lag and excessive battery consumption. |
Security | Implement encryption, secure authentication, and protect sensitive user data. |
"Design should never be just about looks; it should enhance usability and provide meaningful experiences."
iOS Application Development Standards
Developing an iOS application requires a deep understanding of Apple's design principles, usability standards, and technical requirements. By adhering to these guidelines, developers ensure that their apps provide a seamless and consistent experience for users across various devices. This includes the user interface design, navigation flow, and technical constraints specific to iOS systems.
Following the official iOS app development guidelines is essential for creating high-quality apps that align with Apple’s expectations. This encompasses the use of standard controls, efficient coding practices, and proper implementation of system functionalities like notifications and location services.
Key Principles in iOS App Design
- Simplicity: Keep the user interface simple and intuitive, reducing complexity wherever possible.
- Consistency: Ensure that design elements are consistent across the app and with the overall iOS ecosystem.
- Feedback: Provide clear feedback to users for their actions, including visual cues, sounds, or vibrations.
- Accessibility: Make the app usable for all users, including those with disabilities, by integrating accessibility features.
Core Development Guidelines
- Performance Optimization: Ensure that the app is fast and responsive, optimizing resource usage like memory and CPU.
- Security: Implement strong encryption for sensitive data and follow best practices for authentication and authorization.
- Battery Efficiency: Design the app to minimize battery consumption, using background tasks and network calls efficiently.
Important Notes for iOS Development
"Always follow Apple’s Human Interface Guidelines to ensure your app provides the best possible user experience and meets Apple’s design standards."
Device-Specific Considerations
Device | Resolution | Screen Size |
---|---|---|
iPhone 13 | 2532 x 1170 | 6.1 inches |
iPad Pro 12.9 | 2732 x 2048 | 12.9 inches |
Designing User-Friendly Interfaces for iOS Applications
Creating an intuitive interface is crucial for providing a smooth user experience in iOS applications. An easy-to-navigate design ensures that users can quickly understand and engage with the app without unnecessary confusion. A well-designed UI should not only look aesthetically pleasing but also enhance usability by aligning with common user expectations and platform conventions.
When building an iOS app, it’s essential to focus on the interaction flow, accessibility, and responsiveness of the interface. Users should feel comfortable navigating through the app, regardless of their level of expertise with mobile devices. Adhering to Apple’s Human Interface Guidelines is a good starting point, but understanding the key elements of a clean design can elevate the user experience to the next level.
Key Principles for Designing Intuitive iOS UIs
- Consistency: Ensure the app behaves predictably. Keep design patterns consistent across different screens to avoid confusion.
- Clarity: Provide clear visual cues and minimize unnecessary complexity in the layout. Text should be legible, and icons should be recognizable.
- Responsiveness: Design interfaces that respond quickly to user input and adapt to various screen sizes and orientations.
Tip: Prioritize touch targets that are large enough to tap easily, ideally with at least 44px of space around each tap area to improve accessibility.
Creating Effective Layouts
Use layouts that are simple yet functional. When structuring content, break down complex actions into digestible, manageable steps. This helps users stay focused and improves their overall experience. A clean and minimalistic approach can often be more effective than overwhelming the user with too much information.
- Hierarchy: Use visual hierarchy to emphasize key elements, ensuring important features stand out.
- White Space: Don’t overcrowd the screen. Ample white space helps make the app feel less cluttered and improves readability.
- Navigation: Keep navigation simple. Clear buttons and predictable gestures make for easier access to the app’s core functionality.
Interface Component Best Practices
Component | Best Practice |
---|---|
Buttons | Use clear labels and distinguish them visually. Avoid ambiguous or generic text like "Submit". |
Icons | Ensure icons are intuitive, easily recognizable, and consistent across the app. |
Forms | Group related fields together and use visual cues to indicate mandatory fields. |
Optimizing App Performance for a Seamless User Experience
Ensuring smooth performance is critical to providing a seamless experience in iOS applications. Users expect apps to run quickly and efficiently, with minimal delays or interruptions. Slow load times or lagging interfaces can lead to frustration and potentially drive users away. Therefore, optimizing app performance should be a priority during development to meet these high expectations.
In order to achieve optimal performance, developers must focus on several key areas such as efficient memory management, reducing CPU usage, and leveraging platform-specific features for better hardware integration. Following best practices for app optimization not only enhances the user experience but also contributes to the overall success of the app in the market.
Key Performance Optimization Strategies
- Memory Management: Properly managing memory usage helps prevent app crashes or slowdowns due to memory leaks. Use tools like Instruments to identify memory hotspots and implement automatic reference counting (ARC) to handle memory allocation efficiently.
- Efficient Rendering: Avoid rendering unnecessary elements by optimizing views and layouts. Use techniques such as lazy loading to load only the necessary content on-screen, minimizing rendering overhead.
- Background Task Handling: Offload heavy tasks to background threads to ensure the main thread remains responsive. Use Grand Central Dispatch (GCD) or Operation Queues to handle background tasks smoothly.
Tip: Always test your app's performance under real-world conditions. Use profiling tools to assess how your app behaves with different devices and network conditions.
Best Practices for Reducing Latency
- Reduce Network Calls: Minimize the number of network requests by caching data and using efficient APIs to reduce latency.
- Optimize Image Sizes: Compress and resize images before displaying them to avoid unnecessary loading times. Use formats like WebP for better compression.
- App Initialization: Avoid performing heavy tasks during app launch. Instead, load essential resources first and defer non-critical operations.
Performance Testing and Monitoring
Monitoring performance regularly is key to maintaining a smooth user experience throughout the app lifecycle. Make use of the following tools:
Tool | Use Case |
---|---|
Instruments | Detect memory leaks, CPU usage, and performance bottlenecks. |
Xcode Profiler | Profile app performance and measure execution times for functions. |
TestFlight | Collect user feedback on performance during beta testing. |
Implementing Seamless Navigation in iOS Applications
Navigation within an iOS application is crucial to delivering a smooth and intuitive user experience. To ensure users can effortlessly move through different views and features, it's important to follow well-established guidelines and adopt best practices in navigation design. A seamless navigation system enhances usability, reduces cognitive load, and provides users with clear paths to their destinations within the app.
To create a navigation system that feels natural and responsive, developers should prioritize simplicity and consistency. This includes organizing the app's structure into easily understandable navigation elements and ensuring that the flow is intuitive. Below are key elements and strategies for implementing seamless navigation in iOS applications.
Key Principles for Effective Navigation
- Consistency: Ensure navigation elements appear in predictable locations across the app.
- Minimalism: Avoid overcrowding screens with too many navigation options at once.
- Responsiveness: Ensure smooth transitions and quick loading times when switching between views.
- Feedback: Provide visual cues such as highlighted buttons or animations to indicate the current navigation state.
Navigation Components and Their Use Cases
- Tab Bar Navigation: Ideal for apps with a small number of primary sections. It provides quick access to core app functionalities.
- Navigation Controllers: Best used for hierarchical navigation, such as moving from a list of items to detailed item views.
- Modal Views: Suitable for presenting tasks that require the user’s full attention, such as forms or confirmation screens.
Ensure that each navigation flow respects the user's mental model. This means considering how users expect to interact with the app based on common patterns and behaviors they encounter in other applications.
Designing for Efficient Navigation Flow
The goal of any navigation system is to provide users with an efficient and uninterrupted path to their desired actions. It’s important to create a logical structure that is easy to follow. When designing an app’s architecture, consider the following best practices:
Navigation Type | Use Case | Recommended Components |
---|---|---|
Simple Navigation | Small apps with few screens | Tab Bar, Navigation Controller |
Complex Navigation | Apps with multiple features or settings | Split View Controller, Custom Navigation Flow |
Task-Specific Navigation | Apps with isolated tasks or processes | Modal Views, Popovers |
Ensuring App Security with Best Coding Practices
To create a secure iOS application, developers must focus on writing clean, maintainable code that protects both user data and system integrity. Proper handling of sensitive information, including passwords and API keys, is a critical first step. This can be achieved by following robust encryption protocols and limiting data exposure to only essential components.
Security practices should be embedded in the development process from the very beginning. Code reviews, automated security testing, and adherence to platform-specific security guidelines are essential to identifying vulnerabilities early. By applying best coding practices, developers reduce the risk of attacks and ensure that the application remains trustworthy throughout its lifecycle.
Key Security Practices for Secure iOS Code
- Input Validation: Always validate inputs from external sources to prevent injection attacks such as SQL injection or cross-site scripting (XSS).
- Data Encryption: Use AES or RSA encryption for storing sensitive data. Never store unencrypted passwords or keys.
- Secure API Communication: Use HTTPS for API requests to ensure data is encrypted in transit.
- Code Obfuscation: Obfuscate sensitive code to make reverse engineering more difficult.
Important Tips for Secure Code Practices
- Use Keychain Services for storing passwords or tokens securely.
- Utilize Apple’s App Transport Security (ATS) to enforce secure connections.
- Leverage Static Analysis Tools to find vulnerabilities early.
- Regularly Update Dependencies to patch known security flaws in libraries and frameworks.
"Security is not a feature, it's a fundamental principle in every aspect of your app's development."
Common Security Mistakes to Avoid
Security Issue | Consequences | Prevention |
---|---|---|
Storing sensitive data in plain text | Data breach, identity theft | Always use encryption methods for storing sensitive data |
Weak authentication | Unauthorized access to app data | Implement strong multi-factor authentication |
Ignoring platform-specific security features | Increased vulnerability to attacks | Follow platform guidelines such as iOS’s ATS and keychain |
Integrating iOS Features: Push Notifications and In-App Purchases
When developing an iOS app, integrating native features such as push notifications and in-app purchases is crucial to enhance user engagement and generate revenue. These features are key components for offering a personalized experience and monetization opportunities. Both are supported by iOS frameworks and require specific implementation steps to ensure smooth functionality within the app's ecosystem.
Push notifications enable apps to send messages directly to users, even when the app is not in the foreground. This keeps users informed and encourages them to return to the app. On the other hand, in-app purchases provide a way for developers to monetize apps by allowing users to buy additional content or features within the app itself.
Push Notifications
To implement push notifications, developers need to use the Apple Push Notification Service (APNs). Below are the key steps for integrating push notifications into an iOS app:
- Set up an APNs certificate in the Apple Developer account.
- Configure the app to request permission to send notifications from users.
- Handle notification delivery and actions within the app.
- Test the push notifications on physical devices.
Ensure that push notifications are well-targeted and not overly frequent to avoid user annoyance and uninstalls.
In-App Purchases
In-app purchases (IAP) are used to sell content or subscriptions directly within the app. To add IAP to an iOS app, the following steps are necessary:
- Create an IAP product in App Store Connect.
- Implement the StoreKit framework to handle purchase transactions.
- Manage products, receipts, and purchase validation in the backend.
- Test purchases using the sandbox environment before going live.
Always provide a clear and straightforward way for users to restore previous purchases, especially for subscription-based apps.
Comparison of Push Notifications and In-App Purchases
Feature | Push Notifications | In-App Purchases |
---|---|---|
Purpose | Engagement and communication with users | Monetization through app content or features |
Implementation | APNs, local notifications | StoreKit, App Store Connect |
User Interaction | Background updates, alert notifications | Purchase flow within the app |
Optimizing Apps for Various iPhone and iPad Models
When developing an app for iOS, it is crucial to ensure that it performs seamlessly across different iPhone and iPad models. Each device has unique screen sizes, resolutions, and hardware capabilities, making optimization a key factor in delivering a smooth user experience. Proper adaptation of your app can prevent performance issues and visual inconsistencies, especially when dealing with older devices alongside the latest releases.
In order to provide a consistent experience, developers must consider various strategies for adapting layouts, performance, and user interface elements based on the device's specifications. Efficient use of device features like the screen's pixel density, processing power, and storage will significantly impact the success of the app. Below are some essential tips and recommendations for ensuring optimal app performance on different iOS devices.
Considerations for Device-Specific Adjustments
- Responsive Layouts: Use auto layout to adjust the interface according to different screen sizes and orientations.
- Retina Displays: Ensure assets are optimized for high-density screens with multiple resolution options.
- Adaptive UI Components: Incorporate UI elements that adjust to both small (iPhone SE) and large (iPhone 14 Plus) screens seamlessly.
- Performance Scaling: Optimize code and resources for lower-end devices while making use of advanced capabilities in newer models.
Key Performance Metrics and Device Variations
Device | Processor | RAM | Screen Resolution |
---|---|---|---|
iPhone 12 | A14 Bionic | 4 GB | 2532 x 1170 |
iPad Pro 12.9 (2021) | M1 Chip | 8 GB | 2732 x 2048 |
iPhone 7 | A10 Fusion | 2 GB | 1334 x 750 |
When targeting a wide range of devices, it's essential to balance between high-quality visuals and optimized performance to avoid app slowdowns, particularly on older models.
Testing iOS Applications Across Devices and OS Versions
Ensuring compatibility across various iOS devices and operating system versions is crucial for delivering a high-quality user experience. Testing an app across multiple configurations helps identify issues related to performance, layout, and functionality, which might not be evident on a single device or OS version. This process allows developers to optimize their app to work seamlessly for a wide range of users.
With multiple iPhone and iPad models in circulation, along with different iOS versions, testing becomes a multi-dimensional task. Developers must prioritize testing on the most commonly used devices and OS versions while ensuring they cover a broad range of configurations to minimize the risk of bugs and crashes.
Strategies for Effective Testing
- Device Variety: Test on devices with different screen sizes, hardware configurations, and performance capabilities to ensure optimal performance.
- OS Version Support: Ensure the app functions well on multiple iOS versions, especially the latest stable releases and older versions still in use.
- Beta Testing: Engage users in beta testing to gain real-world feedback on different devices and OS versions.
Recommended Testing Process
- Identify a list of devices and OS versions to test, prioritizing the most commonly used ones.
- Perform manual and automated tests on each configuration to identify compatibility issues.
- Use device farms and cloud testing services to test on devices that are not physically available.
- Analyze crash logs and user feedback to fix device-specific issues.
Tip: Make sure to test on both newer and older devices, as performance issues or UI bugs might arise differently on older hardware or with legacy iOS versions.
Sample Testing Matrix
Device | iOS Version | Test Focus |
---|---|---|
iPhone 14 | iOS 16 | Performance, UI responsiveness |
iPhone 8 | iOS 15 | App stability, UI scaling |
iPad Pro 11" | iOS 14 | Landscape mode, multitasking |
Submitting Your iOS App to the App Store: A Step-by-Step Guide
Once your iOS app has been developed, it's time to submit it to the App Store for review. This process involves several crucial steps that ensure your app meets Apple's guidelines and is ready for public use. Carefully following each step will help avoid delays and rejections, ensuring that your app reaches users smoothly and quickly.
In this guide, we will walk you through the essential stages of submitting your app, from preparing your app in Xcode to managing its availability on the App Store. This process requires attention to detail, especially when filling out necessary information, uploading assets, and addressing any issues that might arise during the review process.
Preparation and App Submission
Before submitting your app, make sure you have everything ready. This includes your app's icon, screenshots, and app description. Below is an overview of the key steps to follow:
- Sign Up for an Apple Developer Account - You need a registered Apple Developer account to submit your app to the App Store. This account gives you access to all necessary tools, including App Store Connect.
- Build and Archive the App - Use Xcode to compile and archive your app. Ensure that the app works correctly and passes all tests before you proceed to submission.
- Fill Out Metadata and Upload Screenshots - In App Store Connect, provide necessary details such as app name, description, keywords, and a minimum of one screenshot for each device type (iPhone, iPad, etc.).
- Submit for Review - After uploading your app's details and assets, submit it for Apple's review. This can take anywhere from a few days to a week.
Note: Ensure your app meets all of Apple's guidelines and passes all necessary tests, including functionality and design standards, before submitting.
Review Process and Final Steps
Once submitted, your app will undergo a review process by Apple’s team. During this time, your app will be checked for compliance with App Store policies. After the review, you will receive one of the following outcomes:
- Approval - If your app meets all requirements, it will be approved and published on the App Store.
- Rejection - If any issues are found, your app will be rejected with a list of required changes. You can make corrections and resubmit.
Step | Description |
---|---|
Submission | Submit your app for Apple's review through App Store Connect. |
Review | Apple’s review team checks your app for compliance with guidelines. |
Approval/Rejection | Receive feedback and either get your app published or make necessary changes. |