Android App Development Hello World
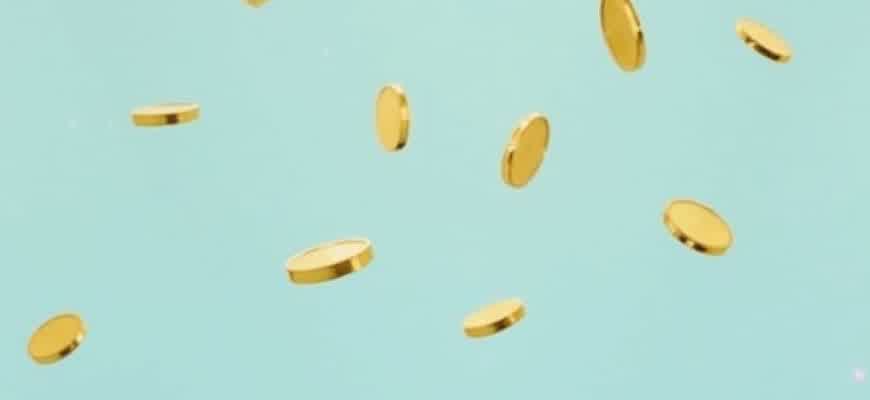
Developing a simple Android application starts with creating a "Hello World" app. This serves as the foundation for understanding how Android applications are structured and how to interact with basic UI components. Let's dive into the core steps involved in setting up your first Android project.
Steps for building a basic Android app:
- Install Android Studio, the official IDE for Android development.
- Create a new project and select a "Basic Activity" template.
- Navigate to the activity_main.xml file to define the layout of the app.
- Update the MainActivity.java file to include logic for displaying text.
- Run the app on an emulator or a physical device.
Important: Always check for Android SDK updates and ensure your project is using the correct API level to avoid compatibility issues.
The most fundamental component of an Android app is the user interface (UI). A basic UI can be built using XML in the layout files, where you can define buttons, text views, and other elements. Below is a simple example of a layout for the "Hello World" app:
File | Content |
---|---|
activity_main.xml |
|
Android Application Development: Getting Started with Your First App
When you begin your journey with Android app development, creating a simple "Hello World" app is an excellent starting point. This app will serve as a foundation to understand how to structure an Android project and run it on your device or emulator. You'll also get familiar with Android Studio, the official Integrated Development Environment (IDE) for Android development.
In this guide, we'll walk through the basic steps to create a "Hello World" application using Android Studio, starting from setting up your development environment to writing the first lines of code that display the classic greeting message on your screen.
Setting Up Your Development Environment
- Download and install Android Studio from the official website.
- Launch Android Studio and configure the IDE according to the setup instructions.
- Create a new project and choose "Empty Activity" template.
Writing Your First App
After setting up the project, you need to modify two important files: the layout file and the main activity file.
- Open res/layout/activity_main.xml and add the following XML code to set up a TextView component:
<TextView android:id="@+id/hello_world_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" />
- Next, open MainActivity.java and ensure that the code includes the necessary logic to load the layout:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); }
Tip: Always remember to test your app on either a physical device or an emulator to ensure proper functionality.
Output and Running the App
After completing the code, you can run your application by clicking the "Run" button in Android Studio. You should see the "Hello World!" message displayed on your device or emulator.
Step | Description |
---|---|
1 | Install Android Studio |
2 | Create New Project |
3 | Edit XML Layout |
4 | Edit Java Activity |
5 | Run and Test |
Setting Up Your Development Environment for Android
Before diving into Android app development, it's crucial to set up a proper environment. This will ensure that you have all the tools necessary to write, test, and deploy your applications efficiently. To start, you'll need to install Android Studio, the official IDE, along with the necessary SDKs and emulators. The following steps will guide you through the process.
The setup process can be broken down into several key components. First, you will need to download and install Android Studio, then configure the Android SDK and ensure your system is ready for running Android emulators or physical devices. Here’s a breakdown of the essential steps:
Installation Steps
- Download the latest version of Android Studio from the official website.
- Run the installer and follow the on-screen instructions to complete the installation.
- Launch Android Studio and let it download the necessary SDK components.
- Set up an Android Virtual Device (AVD) for testing purposes.
Important: Ensure that your system meets the minimum requirements for Android Studio. This includes having sufficient RAM, disk space, and the proper version of Java installed.
Configuring the SDK and Emulator
Once Android Studio is installed, you will need to configure the Android Software Development Kit (SDK) and an Android Emulator or a physical device for testing purposes.
Tip: To avoid performance issues, use an Intel-based system with hardware acceleration enabled for the Android Emulator.
- Open Android Studio and navigate to the SDK Manager.
- Select the SDK platforms and tools you wish to install, such as the latest Android version and Google APIs.
- Set up an AVD using the AVD Manager, or connect a physical device via USB for testing.
System Requirements
Component | Minimum Requirement |
---|---|
OS | Windows 7/8/10, macOS, or Linux |
RAM | 4 GB minimum (8 GB recommended) |
Disk Space | 2 GB free space (4 GB recommended) |
Java | JDK 8 or newer |
Creating a New Project in Android Studio
When starting a new project in Android Studio, it’s essential to properly configure the development environment. Android Studio provides a straightforward wizard to guide you through the process of creating a new Android application. This process includes specifying the project name, application name, and package name, as well as choosing the type of activity to use as a template.
Follow the steps outlined below to create your first Android project in Android Studio:
- Open Android Studio: Launch Android Studio and select "Start a new Android Studio project".
- Configure your project: Choose the project template (e.g., Empty Activity) and click "Next".
- Name your project: Enter the "Name", "Package Name", "Save location", and "Language" (Java or Kotlin). Then, click "Finish".
- Build the Project: Android Studio will start building the project, which can take a few minutes depending on your system.
Important: Make sure to select the appropriate language and SDK version to avoid compatibility issues in your project.
Once the project is created, Android Studio will open the project files in the editor. The project structure includes the following key components:
Component | Description |
---|---|
app/src/main/java | Contains the Java or Kotlin code files for your application. |
app/src/main/res | Contains resources such as layouts, strings, and images. |
AndroidManifest.xml | Defines the application configuration, including activities and permissions. |
Writing Your First "Hello World" App in Android
Creating your first Android application is an exciting experience. In this example, we will walk through the process of building a simple "Hello World" app using Java or Kotlin. The goal is to get a basic understanding of how to set up a project and implement your first lines of code that interact with the Android system.
Android Studio is the most common tool for Android development. The integrated environment simplifies creating, testing, and running Android apps. Whether you're using Java or Kotlin, the structure and steps are fairly similar, with some syntax differences between the two languages.
Steps for Writing the Code
- Set up a new project in Android Studio, choosing either Java or Kotlin as the language.
- Create the Activity by selecting "Empty Activity" template. This will set up the main screen of your app.
- Edit the XML Layout to define the UI. In the
res/layout/activity_main.xml
, modify theTextView
to display "Hello World". - Write the Java or Kotlin code in the
MainActivity
class to handle any interaction or simply display the message when the app starts.
Example Code
Language | Code Example |
---|---|
Java |
package com.example.helloworld; import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } } |
Kotlin |
package com.example.helloworld import android.os.Bundle import androidx.appcompat.app.AppCompatActivity class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } } |
Note: If you are using Kotlin, make sure your Android Studio project is set up with Kotlin support. This might require installing Kotlin plugin if it's not already included.
Run the Application
Once you've completed the setup, you can build and run the app. You'll see a simple screen with "Hello World" displayed, confirming that everything works as expected. With this foundation, you can now start experimenting with other components of Android development!
Understanding the Lifecycle of MainActivity in Android
The MainActivity in an Android application is a core component responsible for interacting with the user. It is the entry point when an app is launched and plays a critical role in managing the application's user interface. Understanding its lifecycle is essential for developing responsive and efficient Android applications. The lifecycle consists of several key states, such as creation, running, paused, and destroyed, each offering opportunities to manage resources effectively and ensure smooth user interactions.
Each phase of the MainActivity lifecycle corresponds to specific methods called by the system. These methods allow developers to handle initialization, state saving, resource management, and cleanup. In this section, we will explore the lifecycle in detail and highlight important methods and best practices for each stage of the activity's existence.
Key Lifecycle Methods
- onCreate() - Called when the activity is first created. Used for setting up the initial state of the activity, such as UI components and binding data.
- onStart() - Called after
onCreate()
or when the activity becomes visible to the user. - onResume() - Called when the activity is ready to interact with the user after being paused or stopped.
- onPause() - Triggered when the activity is partially obscured or not interacting with the user.
- onStop() - Called when the activity is no longer visible to the user.
- onDestroy() - Called before the activity is destroyed. Typically used to release resources.
Lifecycle Diagram
Method | Phase | Purpose |
---|---|---|
onCreate() | Initial creation | Initial setup and resource binding |
onStart() | Activity visible | Prepares the activity to become interactive |
onResume() | Active | Activity becomes interactive |
onPause() | Inactive | Activity partially obscured, save temporary state |
onStop() | Stopped | Activity no longer visible, clean up resources |
onDestroy() | Destroyed | Final cleanup before activity is destroyed |
Important: Each lifecycle method provides an opportunity to handle specific actions, such as saving the state of the activity, releasing resources, or updating the user interface. Failing to manage these transitions correctly can lead to performance issues or unresponsive applications.
Running Your First Android Application on an Emulator or Real Device
Once you’ve set up your development environment and created your first Android application, the next step is to run it on either an emulator or a real device. Testing on an emulator is convenient for quickly checking how your app works in a simulated environment, while running the app on a physical device provides a more accurate reflection of real-world performance and behavior.
To begin testing your app, you must configure the emulator or connect a device. Below are the steps for both options:
Running on an Emulator
- Open Android Studio and select your project.
- Click on the green "Run" button or use the shortcut Shift + F10.
- In the "Select Deployment Target" dialog, choose a pre-configured virtual device or create a new one.
- Click "OK" to start the emulator. Your app will now launch in the emulator window.
Running on a Physical Device
- Enable "Developer Options" and "USB Debugging" on your Android device.
- Connect your device to your computer using a USB cable.
- In Android Studio, click on the "Run" button or press Shift + F10.
- Select your device from the available list and click "OK". The app will be deployed and run directly on your device.
Note: Ensure that you have the necessary USB drivers installed on your computer if you're using a physical device.
Key Differences Between Emulator and Device Testing
Emulator | Device |
---|---|
Simulates Android devices with various configurations. | Represents real-world performance and behavior. |
Faster to set up, but might not reflect real performance. | Provides accurate performance and behavior testing. |
Useful for early development stages and basic testing. | Recommended for final testing before release. |
Debugging and Fixing Common Issues in "Hello World" Applications
When creating a basic Android "Hello World" app, developers may encounter several common issues. Understanding how to troubleshoot these problems is essential for smooth development. Whether it's a syntax error, incorrect resource reference, or misconfigured environment, identifying the cause of the issue early saves valuable time during the app development process.
In the following sections, we will explore typical problems faced during the creation of a simple Android "Hello World" app, along with potential solutions and debugging steps.
Common Issues and Solutions
- Missing or Incorrect Layout Files
- Ensure that the XML layout file is correctly placed in the
res/layout
folder. - Verify that the root element of the XML file is properly defined (e.g.,
RelativeLayout
orLinearLayout
).
- Ensure that the XML layout file is correctly placed in the
- Resource Reference Errors
- Check if the strings used in the layout (e.g.,
@string/hello_world
) are correctly defined in theres/values/strings.xml
file. - If the app crashes due to resource issues, ensure that the resource IDs are not misspelled and the corresponding XML files are properly saved.
- Check if the strings used in the layout (e.g.,
- Incorrect Gradle Configuration
- Ensure that the
build.gradle
file is correctly configured with the appropriate SDK versions and dependencies. - Sync the project with Gradle after making changes to the Gradle files to avoid build issues.
- Ensure that the
Steps for Debugging
- Check the Logcat for error messages or stack traces. This provides detailed information about the crash or bug.
- Set breakpoints in your
MainActivity.java
file to pause execution and inspect variable values and flow of control. - Ensure the emulator or physical device has the correct Android version for compatibility with the app.
Tip: Always check for typos in both Java and XML files, as they are a common source of errors.
Common Logcat Errors and Fixes
Error | Solution |
---|---|
ResourceNotFoundException | Verify the resource IDs in the XML and Java files are correct and match. |
NullPointerException | Ensure that all objects are initialized before use, especially UI elements like TextView or Button . |
ActivityNotFoundException | Check that the AndroidManifest.xml file includes the correct Activity declaration. |
Exploring Basic UI Components in Android Development
When developing an Android app, understanding the fundamental UI elements is crucial for creating user-friendly interfaces. Android offers a wide variety of UI components that you can use to build your application, ranging from simple buttons to complex input forms. Each of these elements is designed to provide a specific function, enhancing the user experience by making the app more interactive and intuitive.
Getting familiar with the most commonly used UI components will help you create a solid foundation for your app. These components allow users to interact with the app and input data, navigate through screens, and receive feedback. Below is an overview of some basic UI elements in Android development.
Common UI Elements
- Button: A clickable element that performs an action when pressed.
- TextView: Displays text to the user, often used for static information.
- EditText: An editable field where users can input text.
- ImageView: Displays images on the screen.
- CheckBox: A box that can be checked or unchecked to indicate a binary option.
UI Layouts
- LinearLayout: Arranges child elements in a single row or column.
- RelativeLayout: Positions child elements relative to each other.
- ConstraintLayout: A more flexible layout that allows precise positioning of elements with constraints.
Key Points to Remember
"Mastering these basic UI components and layouts is the first step in developing a successful Android app."
UI Elements Comparison
Element | Usage | Example |
---|---|---|
Button | Triggers actions | Submit button |
TextView | Displays text | Welcome message |
EditText | Allows text input | Username field |
Building and Packaging Your App for Distribution
Once you have created your Android app, the next step is to prepare it for distribution. This process involves building the final version of your application, ensuring it's optimized for different devices, and then packaging it for distribution through various channels, such as the Google Play Store or other platforms. The final output is an APK or AAB file, which is the format used for installation on Android devices.
Before distribution, you need to properly configure your project for release. This includes setting the correct version numbers, signing the app with a release key, and ensuring that all necessary resources are included. Once these steps are completed, your app is ready to be packaged and distributed.
Steps to Build and Package Your Android App
- Ensure the project is free of errors and warnings.
- Update the versioning in the build.gradle file.
- Configure the app for release by adjusting build settings for performance and size optimization.
- Sign the app with a secure key before packaging for distribution.
- Build the APK or AAB file using Android Studio.
- Test the app thoroughly on various devices to ensure compatibility.
Packaging Your App
- Generate the APK or AAB: In Android Studio, navigate to the "Build" menu, then select "Generate Signed Bundle / APK".
- Choose Build Variant: Select either APK or AAB depending on your distribution method. AAB is recommended for Google Play.
- Sign the App: Provide the necessary keystore and credentials for signing the app.
- Build and Export: After completing the signing process, export the final APK/AAB file ready for distribution.
Note: It's essential to keep your keystore and credentials secure, as they are needed to update the app in the future.
App Distribution
After packaging your app, you can distribute it through different channels. The most common method is via the Google Play Store, but you can also distribute the APK directly or through other platforms like Amazon Appstore.
Distribution Method | Details |
---|---|
Google Play Store | Requires an AAB file, automatic updates, and app analytics. |
Direct APK Distribution | Users manually download and install the app, but updates need to be managed manually. |
Other Platforms | Includes stores like Amazon Appstore; may require additional packaging. |