How to Build an Android App From Scratch
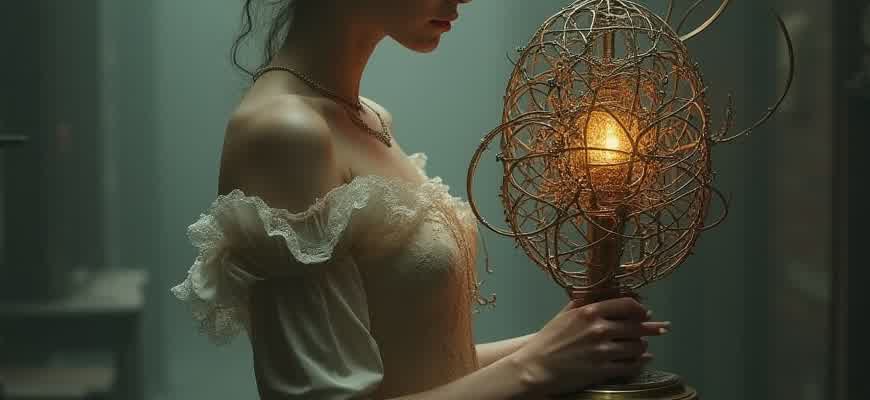
Building an Android application requires a solid understanding of Java or Kotlin, the Android SDK, and the development environment. The first step is setting up Android Studio, the official IDE for Android development. This platform provides all the tools and resources necessary for creating, testing, and deploying Android apps.
Here’s a structured approach to creating your first Android app:
- Set Up Development Environment: Install Android Studio and ensure you have the necessary SDKs and dependencies.
- Plan Your App: Define the core features and layout before starting coding.
- Start Coding: Create your app’s user interface (UI) and implement the business logic.
- Test the App: Test your app using an emulator or a physical device to fix any bugs.
- Deploy: Once the app is stable, you can publish it to the Google Play Store or distribute it directly.
Each of these steps involves specific tasks. Let’s dive deeper into the details:
- Set Up Your Development Environment: Install Android Studio and configure your SDK. Make sure to enable virtualization support to run emulators efficiently.
- Design Your App’s Architecture: Consider using MVVM (Model-View-ViewModel) or MVC (Model-View-Controller) for better scalability and maintainability.
- Build UI and Handle Events: Create activities, layouts, and views. Use XML for static layouts and Kotlin/Java for dynamic UI behaviors.
Important: Always keep in mind that the user interface should be intuitive. Make sure the app is easy to navigate and visually appealing.
After completing the initial setup and coding phases, you’ll need to ensure your app runs on different devices with various screen sizes and configurations. Testing on real devices is essential to confirm performance and resolve issues that may not appear in the emulator.
Step | Task |
---|---|
1 | Set up Android Studio |
2 | Design app architecture and user interface |
3 | Implement features and logic |
4 | Test and debug |
5 | Deploy to Play Store or distribute |
Choosing the Right Development Environment for Your Android App
When starting to build an Android app, selecting the appropriate development environment is crucial for a smooth and efficient workflow. The right tools not only enhance productivity but also ensure the app is optimized for performance, functionality, and user experience. There are various options to consider, each with its own advantages and limitations depending on the scope and complexity of your project.
Android development involves a mix of coding, debugging, and testing, so it's essential to pick an environment that supports these tasks seamlessly. Among the most popular choices, you'll find comprehensive integrated development environments (IDEs) as well as more lightweight alternatives tailored for specific needs. Below, we explore some of the most commonly used tools for Android development.
Key Development Tools for Android
- Android Studio: The official IDE for Android app development, providing robust features such as code completion, real-time error checking, and an emulator for testing your app on different devices.
- Visual Studio with Xamarin: A cross-platform tool that allows for app development for both Android and iOS, using C# and .NET.
- Eclipse: Once the go-to tool for Android development, now less popular but still a viable option for developers familiar with it.
Factors to Consider
- Platform Compatibility: Make sure the environment is compatible with your operating system (Windows, macOS, or Linux) and can run smoothly on your machine.
- Features and Functionality: Consider built-in tools such as debuggers, emulators, or performance monitors that can save you time during development.
- Community Support: A tool with strong community support can be helpful when you run into issues, as you can find answers, plugins, and tutorials easily.
Tip: If you’re new to Android development, starting with Android Studio is often the best choice due to its extensive features and official support from Google.
Comparison of Development Environments
IDE | Supported Platforms | Primary Language | Cross-Platform Support |
---|---|---|---|
Android Studio | Windows, macOS, Linux | Java, Kotlin | No |
Visual Studio | Windows, macOS | C#, F# | Yes (with Xamarin) |
Eclipse | Windows, macOS, Linux | Java | No |
Setting Up Android Studio for Your First Project
Before diving into Android development, it's crucial to prepare your development environment. The first step is to download and install Android Studio, which is the official IDE for Android app development. Once installed, you'll be able to create and manage Android projects with ease, as the IDE offers comprehensive tools like code editors, emulators, and debugging utilities.
Here’s a step-by-step guide to get you started with Android Studio, setting up everything you need for a smooth experience during your first Android development journey.
Installation of Android Studio
To begin, follow these steps to install Android Studio:
- Visit the official Android Developer website to download Android Studio for your operating system.
- Run the installer and follow the on-screen instructions to complete the installation process.
- Once installed, launch Android Studio and proceed with the initial setup. This may involve downloading additional components, like Android SDK and required plugins.
Configuring Android Studio for Your Project
After installing Android Studio, configure it for optimal performance:
- Ensure that you have the latest version of the Android SDK and necessary tools installed through the SDK Manager.
- Configure an emulator or connect a physical device for testing your app.
- Set up a new project with the appropriate SDK version based on your app requirements.
Important: Always update Android Studio and the SDK regularly to access new features, tools, and bug fixes.
Creating Your First Android Project
Once the IDE is set up, you’re ready to create your first Android project. Here’s how:
- Open Android Studio and click on "Start a new Android Studio project."
- Choose a project template (e.g., "Empty Activity") based on the type of app you're building.
- Enter your project name, package name, and set the save location.
- Choose the language (Java or Kotlin) and set the minimum API level for your app.
- Click “Finish” to create the project and open the main activity file.
Table: Key Elements in Android Studio Setup
Component | Purpose |
---|---|
SDK Manager | Download and manage SDK versions and other development tools. |
AVD Manager | Configure and run Android emulators for testing your app. |
Gradle | Manage dependencies and build configurations for your project. |
Understanding the Structure of an Android Project and Its Files
When creating an Android app, understanding the structure of the project is essential for efficient development. An Android project consists of various directories and files that serve different purposes. These files can be categorized into several components, each playing a specific role in the overall functionality of the app. Knowing where each file is located and how it interacts with the rest of the project is key to managing the project effectively.
The main structure of an Android project is organized in a way that separates code, resources, and configuration files. By understanding this structure, you can navigate the project more easily, add new features, and troubleshoot any issues that may arise during development. Below is an overview of the typical files and directories you will encounter.
Key Directories and Files in an Android Project
- app/ - This is the main module of the project where most of your code, resources, and configuration files are stored.
- src/ - Contains all Java or Kotlin files that define the functionality of the app.
- res/ - The resources directory holds XML files for layouts, drawables, and other assets such as images and strings.
- manifests/ - Includes the AndroidManifest.xml file, which contains essential information about the app, like permissions and activities.
- gradle/ - Contains configuration files for Gradle, which handles the build and dependency management processes.
Important Files to Know
File | Description |
---|---|
AndroidManifest.xml | Defines essential app details like permissions, activities, and components. |
build.gradle | Contains project-level and app-level configuration for Gradle, including dependencies and build variants. |
strings.xml | Stores all the text-based resources used in the app, such as labels, buttons, and error messages. |
activity_main.xml | The default layout file for the main activity, defining the UI elements on the screen. |
Understanding the purpose of each directory and file within an Android project helps streamline the development process, ensuring that the app remains organized and maintainable throughout its lifecycle.
Designing User Interfaces with XML Layouts
Creating an intuitive user interface (UI) is a critical part of mobile app development. In Android, the layout of the app’s interface is built using XML, which allows developers to design visually appealing and responsive screens. The XML layouts provide a clear separation between the logic of the app and its visual structure, enabling easier maintenance and updates.
XML layouts are structured hierarchically, using various UI components to create the desired screen. Developers can include buttons, text fields, images, and other widgets within these layouts. The key to designing a smooth UI is to balance usability with performance by carefully selecting the appropriate layout components.
Elements of an XML Layout
There are several components available in XML to help build the UI. Some of the most commonly used are:
- LinearLayout: Organizes child elements either vertically or horizontally.
- RelativeLayout: Positions elements relative to each other or to the parent container.
- ConstraintLayout: Offers flexible and efficient positioning of UI elements with constraints.
- TextView: Displays text on the screen.
- Button: A clickable UI element for user interaction.
The following table outlines some key attributes used in XML layouts:
Attribute | Description |
---|---|
layout_width | Defines the width of the element (e.g., match_parent, wrap_content, or a specific value). |
layout_height | Specifies the height of the element (e.g., match_parent, wrap_content, or a specific value). |
padding | Sets the space around the content of the element. |
gravity | Aligns the content inside the element (e.g., center, start, end). |
Remember to test the UI design on various screen sizes to ensure it adapts correctly to different devices. XML layouts can scale using `dp` (density-independent pixels) and `sp` (scaled pixels) units for better responsiveness.
Writing Code in Java/Kotlin for App Features
When developing the core functionality of an Android app, the choice between Java and Kotlin becomes crucial. Both languages have their advantages, but the selection depends on the specific needs of your project. Java has been the primary language for Android development for years, while Kotlin, introduced by Google in 2017, has quickly become the preferred language for many developers due to its modern features and concise syntax.
The process of writing code for app functionality involves creating methods, defining variables, and implementing logic that connects your UI to backend processes. Whether you are developing an app from scratch or adding new features to an existing one, the quality of your code impacts performance, scalability, and maintainability.
Writing Java/Kotlin Code for App Logic
To implement functionality, you will typically follow these steps:
- Define user inputs and interactions.
- Write methods to handle these inputs and update the UI accordingly.
- Integrate external APIs or databases if needed.
- Test and debug your app to ensure smooth performance.
For example, handling a button click in Android can be done like this:
Button myButton = findViewById(R.id.my_button); myButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Do something when the button is clicked } });
In Kotlin, the code would be simplified:
myButton.setOnClickListener { // Do something when the button is clicked }
Important: Kotlin is fully interoperable with Java, so you can use both languages in the same project, but the best practice is to stick to one language for consistency.
Working with Data and External Libraries
Android apps often rely on external libraries and APIs to perform certain tasks like fetching data from the internet or accessing databases. Both Java and Kotlin support these integrations, but Kotlin’s modern syntax can make it easier to work with libraries such as Retrofit, Gson, or Room for database management.
- Choose a library based on the task (e.g., use Retrofit for network calls).
- Add the required dependencies in the build.gradle file.
- Implement the necessary classes and functions to handle the data.
Here’s an example of integrating Retrofit for network calls in Kotlin:
val retrofit = Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .build() val service = retrofit.create(ApiService::class.java) val call = service.getData() call.enqueue(object : Callback{ override fun onResponse(call: Call , response: Response ) { // Handle successful response } override fun onFailure(call: Call , t: Throwable) { // Handle failure } })
Below is a simple comparison of Java and Kotlin code for Retrofit integration:
Language | Code Example |
---|---|
Java | Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .build(); ApiService service = retrofit.create(ApiService.class); Call |
Kotlin | val retrofit = Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .build() val service = retrofit.create(ApiService::class.java) val call = service.getData() |
Implementing Navigation and Transitions Between Screens in Android Apps
Building a smooth and intuitive navigation system is a fundamental aspect of creating a successful Android application. The way users transition between screens, known as activities, directly impacts the user experience. Understanding how to implement effective navigation and seamless activity transitions is crucial for ensuring users have an enjoyable interaction with your app.
In Android, navigation can be achieved through intents, navigation components, or custom transitions. A robust implementation of these methods ensures that your app feels responsive and coherent, offering users a smooth flow as they move from one screen to another. Below is an overview of how to implement these features effectively.
Types of Navigation in Android Apps
- Intents: These are used to initiate a transition from one activity to another. Intents can carry data, allowing different parts of your app to communicate with each other.
- Navigation Component: This is part of Android Jetpack and simplifies app navigation by using NavController and NavGraph. It helps in managing fragment transactions and deep links.
- Custom Navigation: If you want more control over transitions and animations, you can implement custom navigation logic using fragments or activity transitions.
Managing Activity Transitions
Android offers several ways to handle the transition between activities, with or without animations. Each method has its own use case, and choosing the right one will enhance the experience for the user.
- Default Transition: By default, when transitioning between activities, Android applies a fade-in/fade-out effect. This is simple but may not always match your app's style.
- Custom Animations: You can define custom animations for enter, exit, and pop transitions using overridePendingTransition() method.
- Shared Element Transitions: For smoother transitions between activities, shared elements like images or text can be animated to appear as if they move from one screen to the other.
Important: Always ensure that transitions are not too long or distracting. Short and simple animations enhance the user experience without slowing down navigation.
Example Transition Using Custom Animations
Method | Description |
---|---|
Activity A to Activity B | Use overridePendingTransition() to define custom enter and exit animations. |
Shared Element Transition | Use ActivityOptions.makeSceneTransitionAnimation() to transition shared elements between activities. |
Testing Your Android Application on Emulators and Real Devices
Testing is an essential step when developing an Android application, as it ensures that your app runs smoothly on different devices and operating systems. There are two primary ways to test your app: using emulators and testing on physical devices. Each method has its advantages and limitations, making it important to use both during the development process.
Emulators are virtual devices that run on your computer, mimicking the behavior of real Android devices. They allow developers to test their apps without needing physical hardware. Physical devices, on the other hand, offer a more accurate testing environment since they simulate real-world usage and performance.
Emulator Testing
Emulators are widely used for testing applications because they provide a variety of configurations, such as different screen sizes, Android versions, and resolutions. The Android Studio provides a built-in emulator that allows developers to simulate various devices with ease. However, it's important to note that emulators can be slower and may not reflect all hardware features of real devices.
- Advantages:
- Convenient for quick testing during development.
- Supports multiple configurations of virtual devices.
- Useful for testing edge cases like different screen sizes and Android versions.
- Limitations:
- May not provide accurate performance feedback.
- Doesn’t replicate real-world hardware features like sensors, cameras, or accelerometers.
Physical Device Testing
Testing on physical devices is crucial for obtaining real-world performance data. By running the app on actual devices, developers can observe how the app behaves in various scenarios, such as battery consumption, responsiveness, and hardware interactions.
- Advantages:
- Accurate performance testing under real-world conditions.
- Ensures compatibility with actual device hardware features.
- Allows you to test touch interactions and real-time sensors.
- Limitations:
- Requires physical devices, which can be costly and time-consuming to acquire.
- Limited number of devices may restrict the range of testing.
Key Considerations for Testing
Testing on both emulators and physical devices is crucial to ensure that your app works seamlessly across different environments. Emulators help you catch most issues early, while physical devices ensure you account for real-world performance and user experience.
Aspect | Emulator | Physical Device |
---|---|---|
Performance | Slower | Real-time performance |
Hardware Access | Limited | Full access |
Testing Range | Multiple configurations | Actual device scenarios |