Ios App Development Vscode
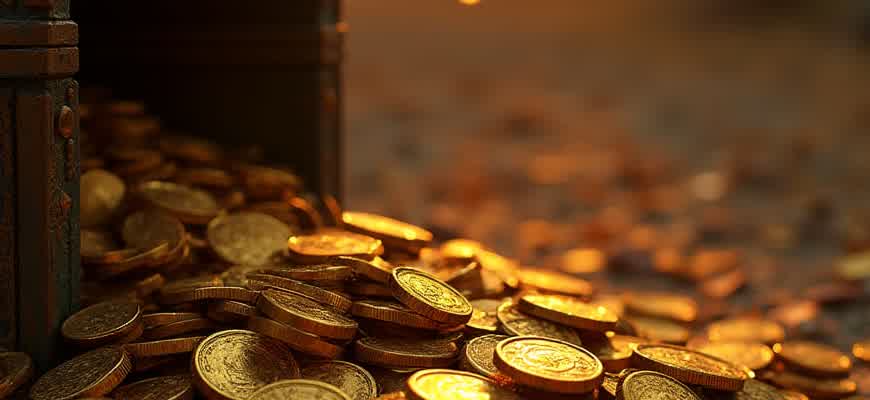
Visual Studio Code (VS Code) has emerged as a popular lightweight code editor, particularly among developers seeking an alternative to Xcode for iOS app development. Although Xcode is the standard IDE for iOS, VS Code offers flexibility and a fast development cycle, making it an attractive choice for many developers.
To set up VS Code for iOS development, follow these key steps:
- Install Xcode and set up Xcode Command Line Tools
- Install Node.js and npm to manage dependencies
- Set up VS Code extensions such as Swift and React Native Tools
- Configure your development environment for simulators and real device testing
Here's a breakdown of the development environment:
Tool | Description |
---|---|
Xcode | Required for building iOS applications, as it includes necessary SDKs and simulators. |
VS Code | Lightweight code editor ideal for editing Swift, Objective-C, or cross-platform code. |
Node.js | Essential for managing JavaScript-based projects or React Native applications. |
Note: While VS Code is highly flexible, some advanced features like Interface Builder and real-time preview are not natively supported.
iOS App Development Using VSCode: A Step-by-Step Approach
Developing iOS apps traditionally requires Xcode, but VSCode offers a lightweight alternative that can streamline your workflow, especially for those who prefer more control over their development environment. With the right setup and extensions, Visual Studio Code can be turned into a powerful tool for building iOS applications.
While Xcode is designed specifically for iOS development, VSCode allows developers to use a variety of programming languages and frameworks. By leveraging tools like Swift and Flutter, developers can work on iOS apps without the hefty setup of Xcode. This guide will walk you through the essential steps to get started with iOS development using VSCode.
Essential Tools and Setup
- VSCode Installation: Download and install Visual Studio Code from its official website.
- Swift Setup: Ensure you have the latest version of Swift installed on your machine.
- Xcode Command Line Tools: Install Xcode command line tools for building apps on your Mac.
- VSCode Extensions: Add essential extensions like "Swift for Visual Studio Code" and "Flutter" for better syntax support and debugging capabilities.
Building an iOS App in VSCode
- Create a New Project: Start by creating a new Swift or Flutter project in VSCode using the integrated terminal.
- Code Your App: Write your app's code using Swift for native development or Flutter for cross-platform solutions.
- Run Your App: Use the integrated terminal and Xcode command-line tools to compile and launch your app on the iOS simulator or an actual device.
Important: Although VSCode does not come with a built-in iOS simulator, you can use Xcode’s simulator and command-line tools to test your app during development.
Debugging and Testing
VSCode offers a range of debugging tools, including breakpoints and variable inspection. However, for full debugging functionality on iOS, you will still rely on Xcode's tools. Setting up remote debugging in VSCode with a Swift-based app may require some additional configuration, such as connecting to an iOS device via USB or using a network for remote debugging.
Advantages of Using VSCode for iOS Development
Advantages | Details |
---|---|
Lightweight Environment | VSCode is fast and uses fewer resources compared to Xcode. |
Customizability | You can extend VSCode with a variety of extensions tailored to your workflow. |
Cross-Platform | Works across different operating systems (Windows, macOS, Linux). |
Setting Up Visual Studio Code for iOS Application Development
To begin developing iOS apps in Visual Studio Code, several steps must be followed to ensure that the IDE is correctly configured. While Xcode is the primary tool for building iOS apps, VSCode can be an excellent alternative for writing code, especially when paired with the right extensions and configurations. By optimizing VSCode for iOS development, developers can take advantage of a lightweight and flexible code editor while maintaining efficiency.
In this guide, we will outline the steps to set up your environment, including essential tools and extensions. With the proper configuration, you can streamline your iOS development workflow in VSCode while still leveraging the power of the Apple ecosystem.
Prerequisites
- Install Xcode and Xcode Command Line Tools.
- Install Homebrew (for managing dependencies).
- Install Node.js (required for JavaScript-based tooling).
- Install CocoaPods (for managing iOS dependencies).
Steps to Set Up VSCode
- Download and install Visual Studio Code from the official website.
- Install the necessary extensions for iOS development:
- Swift - Provides syntax highlighting and code completion.
- Xcode Integration - Useful for bridging VSCode with Xcode projects.
- CocoaPods - Helps in managing dependencies for iOS projects.
- iOS Simulator - Integrates iOS simulator functionalities into VSCode.
- Configure the workspace settings to use the correct build tools and environment paths.
- Install CocoaPods by running sudo gem install cocoapods in the terminal.
- Ensure that the iOS simulator is correctly set up and that the project is recognized by Xcode.
Remember, Xcode is still necessary to build and deploy the app to the iOS simulator or device. VSCode functions as an editor, but you need Xcode for compilation and testing.
Workspace Configuration Example
Tool | Installation Command |
---|---|
Homebrew | bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)" |
Node.js | brew install node |
CocoaPods | sudo gem install cocoapods |
Installing Essential Plugins for Efficient iOS App Development
When setting up your development environment for iOS app creation in Visual Studio Code, it's important to install the right plugins to enhance your workflow. These tools not only increase productivity but also ensure smoother integration with iOS-specific tools and frameworks. Using the right extensions can significantly reduce time spent on repetitive tasks and streamline the overall coding process.
VS Code offers a variety of plugins that cater to different aspects of iOS app development, from code editing to debugging. Below, you’ll find a list of must-have extensions for building iOS applications effectively.
Key Plugins for iOS Development
- Swift Language Support: Adds syntax highlighting, IntelliSense, and code completion for Swift files.
- iOS Simulator Integration: Helps simulate the iOS environment right from VS Code, streamlining testing without needing Xcode's interface.
- Cocoapods Extension: Simplifies managing dependencies within your project.
- Debugger for Swift: Offers debugging capabilities for Swift code directly within VS Code.
Installation Steps
- Open VS Code and go to the Extensions view by pressing Ctrl+Shift+X.
- Search for the desired plugin (e.g., "Swift" or "iOS Simulator").
- Click on the install button for each extension.
- After installation, restart VS Code to ensure all changes take effect.
Note: After installation, make sure to configure each plugin according to your specific project setup for optimal performance.
Comparison of Popular iOS Development Extensions
Plugin | Features | Use Case |
---|---|---|
Swift | Syntax highlighting, code completion, linting | For writing and managing Swift code |
iOS Simulator | Simulate iPhone, iPad environments | For testing apps without using Xcode |
Cocoapods | Dependency management | For integrating third-party libraries |
Integrating Xcode with VSCode for Enhanced Debugging
Developing iOS applications often requires a seamless workflow between different development tools. One powerful combination is using Visual Studio Code (VSCode) in conjunction with Xcode. VSCode provides a lightweight and customizable code editor, while Xcode offers a robust suite of tools for building and debugging iOS apps. By integrating the two, developers can improve their debugging capabilities, enhancing productivity and reducing development time.
This integration allows developers to leverage the strengths of both platforms. VSCode's versatility as a code editor can complement Xcode's debugging tools. By setting up proper configurations, it becomes possible to debug iOS apps directly within VSCode while still utilizing the full power of Xcode's simulator and other debugging features.
Setting Up the Integration
- Install the necessary extensions in VSCode, such as "Swift" and "Xcode" related plugins.
- Ensure that Xcode is correctly installed and accessible from the command line.
- Configure the "launch.json" file in VSCode to connect with Xcode's simulator for running the app.
Benefits of Debugging with Both Tools
- Real-time error tracking: Debugging in VSCode allows you to quickly identify syntax and runtime errors while maintaining the ability to step through your code in Xcode's simulator.
- Seamless workflow: Switching between VSCode and Xcode without interrupting the development process increases efficiency and focus.
- Customizable debugging: VSCode's extensive plugin ecosystem allows you to tailor your debugging environment to your specific needs, such as adding custom linting or logging tools.
Key Configuration Considerations
Configuration | Action |
---|---|
Xcode Command Line Tools | Make sure to install Xcode's command line tools via Xcode preferences. |
Launch Configuration | Set up the appropriate "launch.json" file in VSCode to specify the iOS target and simulator. |
Debugging Output | Ensure that output from the app is visible in both VSCode's integrated terminal and Xcode's debug console. |
Important: Always test the debugging setup with a small app first to ensure smooth communication between Xcode and VSCode before integrating into larger projects.
Writing Swift Code in VSCode: Best Practices
When working with Swift in Visual Studio Code, it's essential to establish a workflow that maximizes productivity and minimizes common pitfalls. VSCode is a powerful, lightweight IDE that offers flexibility, but it requires a specific setup to efficiently handle Swift code. Leveraging proper extensions, organizing code structure, and following best practices can make Swift development much smoother in this environment.
Although Xcode is the official IDE for iOS development, VSCode is an excellent alternative for developers seeking a lightweight setup or those working on cross-platform applications. This guide will provide insights into the best practices for writing Swift code in VSCode, covering key aspects like configuration, code organization, and tooling to enhance your workflow.
Essential Setup for Swift Development in VSCode
- Install the Swift Language Extension: First and foremost, ensure you have the Swift extension installed in VSCode. It provides syntax highlighting, auto-completion, and error checking.
- Install the Swift Toolchain: Set up the Swift toolchain on your system. This is necessary for compiling Swift code and ensuring the Swift environment works as intended.
- Enable Auto-formatting: Use SwiftFormat or SwiftLint to auto-format your code. This helps maintain consistent code style across your project and ensures readability.
Writing Swift Code Effectively
- Follow Swift's Naming Conventions: Adhere to standard naming conventions in Swift to ensure your code is clean and maintainable. This includes using camelCase for variables and functions, and CapitalizedCamelCase for types.
- Break Down Complex Functions: Avoid long, complicated functions. Break them into smaller, reusable components. This improves testability and readability.
- Use Optionals Wisely: Always unwrap optionals safely using guard or if-let statements. This avoids runtime crashes and improves code safety.
"Swift's optionals are a powerful feature, but they need to be handled with care to avoid unexpected crashes in your application."
Code Organization and Structure
Proper organization of your code is crucial for maintainability, especially when working with larger projects. Use the following structure to keep your Swift codebase clean:
Folder | Description |
---|---|
Sources | Contains all the Swift files for the project logic. |
Resources | Holds asset files like images, JSON files, and storyboards. |
Tests | Holds unit tests to ensure code reliability. |
By following these best practices and setting up your environment appropriately, you can streamline your Swift development in VSCode and focus on building robust, clean applications.
Managing Dependencies with CocoaPods and VSCode
When developing iOS applications using Visual Studio Code, managing third-party libraries and dependencies becomes crucial for maintaining a clean and scalable project structure. CocoaPods is one of the most popular tools used for dependency management in iOS development, helping developers easily integrate external libraries into their projects.
To efficiently manage dependencies within VSCode, you need to set up CocoaPods in your Xcode project and integrate it with the code editor. Once configured, you can automate the installation and management of dependencies directly from the terminal, reducing the complexity of handling libraries manually.
Steps to Integrate CocoaPods with VSCode
- Open your iOS project directory in the terminal.
- Run the command pod init to create a Podfile in the project.
- Add the required dependencies in the Podfile under target 'YourApp' do.
- Execute pod install to install the dependencies.
- Open the generated YourApp.xcworkspace file in Xcode to continue your development.
Important: Always use .xcworkspace instead of .xcodeproj to open your project after installing pods. This ensures that all CocoaPods dependencies are correctly linked to your project.
Handling CocoaPods with VSCode
Although VSCode doesn't provide built-in support for managing CocoaPods, you can still run terminal commands within the editor. Here's how to effectively use VSCode for dependency management:
- Install the Terminal extension to run shell commands directly inside VSCode.
- Open the terminal in VSCode and navigate to your iOS project directory.
- Execute pod install to update or add new dependencies.
- Ensure your project is linked to Xcode by opening the .xcworkspace file for further development.
Dependencies Table
Library | Purpose | Installation Command |
---|---|---|
Alamofire | Networking library for iOS | pod 'Alamofire' |
SnapKit | Autolayout framework | pod 'SnapKit' |
Realm | Mobile database | pod 'Realm' |
Running iOS Apps Locally Using VSCode Terminal
For developers working with iOS applications, Visual Studio Code (VSCode) provides a versatile environment, enabling both code editing and terminal operations within a single interface. The integrated terminal in VSCode allows you to run and manage various tasks related to iOS app development directly from the editor, which can improve productivity and streamline the development workflow.
When it comes to testing and running iOS apps locally, the VSCode terminal is an invaluable tool. It simplifies interactions with the underlying development tools, such as Xcode’s command line utilities, allowing you to manage builds, install dependencies, and execute the app on a local simulator or device.
Steps to Run an iOS App from VSCode Terminal
- Install Dependencies: Make sure you have all the necessary tools installed, including Xcode and CocoaPods for managing project dependencies. You can install CocoaPods via the terminal with the following command:
sudo gem install cocoapods
- Navigate to the Project Directory: Use the terminal to move to your iOS app project folder:
cd /path/to/your/project
- Install Project Dependencies: If you are using CocoaPods, you need to install the required dependencies for the project:
pod install
- Build the App: Use the Xcode command line tool to build the app:
xcodebuild -scheme YourAppScheme -sdk iphonesimulator
- Run on Simulator: Launch the app on the iOS simulator with the following command:
xcrun simctl boot 'iPhone 12'
- Deploy on Device (Optional): For running the app on a physical device, use the following command:
xcodebuild -destination 'platform=iOS,id=
'
Key Considerations
- Simulator vs Physical Device: Always ensure that you are targeting the correct device or simulator based on your testing needs.
- Dependencies: Make sure all dependencies are installed and up to date to avoid build issues.
- App Signing: Ensure that your app is properly signed if you plan to deploy it to a physical device.
Common Commands for iOS App Development in VSCode Terminal
Command | Description |
---|---|
pod install | Installs CocoaPods dependencies for the project. |
xcodebuild | Builds the app using Xcode command-line tools. |
xcrun simctl | Manages the iOS simulator, including booting devices and running apps. |
Implementing Code Suggestions and Syntax Highlighting in VSCode
When developing iOS applications in VSCode, enhancing the coding experience with features like auto-completion and syntax highlighting is crucial for productivity. These features help developers write code more efficiently by offering suggestions and visually distinguishing different code elements. Integrating these features into VSCode requires configuring specific tools and extensions that enable the editor to understand the syntax and structure of the iOS development languages, such as Swift or Objective-C.
Auto-completion and syntax highlighting are powered by language extensions that parse the code and provide real-time feedback. VSCode offers various ways to implement these features, primarily through the use of Language Server Protocol (LSP) and TextMate grammars. Developers can leverage existing extensions or create custom ones to fit their project needs.
Implementing Auto-Completion
Auto-completion in VSCode is achieved through language-specific extensions or custom Language Server Protocol (LSP) setups. These setups analyze the syntax of the code and predict the next words, methods, or variables based on the context.
- Install relevant iOS development extensions such as Swift or Objective-C.
- Configure LSP to enable auto-suggestions for these languages.
- Ensure that the extension is set up to provide real-time code suggestions while typing.
Key Benefits:
Auto-completion saves developers time by suggesting relevant code snippets, reducing the chance of errors and increasing development speed.
Syntax Highlighting Configuration
Syntax highlighting can be achieved by using predefined TextMate grammars that define how different parts of the code should be highlighted, such as keywords, variables, functions, and other constructs.
- Install a syntax highlighting extension for Swift or Objective-C.
- Ensure that the file type association is correct for the codebase.
- Customize TextMate grammars if needed to meet the specific needs of your project.
Tip: Syntax highlighting not only enhances readability but also helps in debugging by visually distinguishing errors or potential issues in the code.
Example Configuration Table
Extension | Feature | Setup Instructions |
---|---|---|
Swift for Visual Studio Code | Auto-completion, syntax highlighting | Install the extension via the VSCode marketplace and configure LSP. |
Objective-C | Syntax highlighting | Download the extension and associate Objective-C file types. |
Troubleshooting Common Issues in VSCode During iOS App Development
When working with VSCode for iOS app development, you may encounter a variety of issues that can disrupt your workflow. Addressing these problems quickly is essential for maintaining productivity. Common issues often involve configuration errors, missing dependencies, and issues related to the integration of Xcode with the VSCode environment. Below are some tips and solutions for resolving these problems effectively.
Before diving into specific problems, ensure that your development environment is properly set up. Make sure you have the correct versions of Xcode, VSCode, and all necessary extensions installed. If you encounter errors during the setup or while running the project, it's essential to troubleshoot each element systematically to resolve conflicts or misconfigurations.
Common Issues and Fixes
- Missing Dependencies - This is one of the most frequent issues when setting up a new project. Ensure all required libraries and packages are properly installed using
npm install
orpod install
. - Incorrect Build Settings - Often caused by incorrect configurations in build settings or missing environment variables. Double-check your
xcconfig
files and the VSCode workspace settings. - Code Signing Errors - These errors typically occur when the iOS app cannot be signed properly. Ensure that your Apple Developer account is correctly linked to Xcode and that the necessary provisioning profiles are in place.
Steps for Resolving Build Issues
- Ensure your macOS is up to date along with all necessary developer tools.
- Verify that all packages are correctly installed by running
npm install
andpod install
in the terminal. - Check if there are any conflicts between your Xcode project settings and VSCode settings.
- Run
flutter clean
orxcodebuild clean
to clear old build data. - If the error persists, consider resetting your VSCode workspace or recreating your Xcode project configuration.
Tip: Sometimes, simply restarting VSCode or cleaning the build folder can resolve many issues.
Common Errors in the Debugger
Error | Solution |
---|---|
Unable to Launch Simulator | Make sure the iOS simulator is installed via Xcode and properly configured. Restart both VSCode and the simulator. |
Debugger Not Attaching | Ensure that the appropriate debugging extensions, such as the Flutter or React Native extension, are installed and active. |