Ios App Development Login
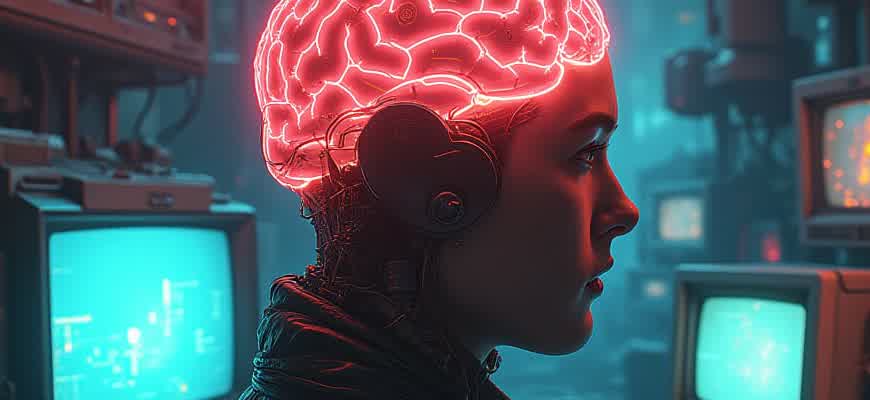
The user authentication process is a crucial component of any iOS application, ensuring secure access to the app’s features. Implementing a login system requires careful attention to both user experience and security protocols. One of the first steps in this process is selecting an authentication method, which can vary depending on the app's requirements and the level of security needed.
When designing the login interface, it is essential to consider both ease of use and robustness. Below are some key aspects to consider:
- Authentication Methods: Choose between traditional username/password, biometric authentication, or social login options.
- Security Protocols: Implement encryption protocols like HTTPS and data hashing to protect user credentials.
- User Feedback: Provide clear error messages and visual feedback for a better user experience.
Here is an overview of a basic login workflow:
- User enters login credentials (username/password).
- The app verifies the credentials with a backend server.
- If credentials are valid, access is granted. If invalid, the user is prompted to try again or reset their password.
Important: Never store passwords in plaintext. Use secure methods like bcrypt or PBKDF2 for hashing passwords.
For a more sophisticated implementation, consider adding multi-factor authentication (MFA) to further enhance security.
Authentication Method | Advantages | Challenges |
---|---|---|
Username/Password | Simple and widely used | Vulnerable to breaches if not handled securely |
Biometric Authentication | Convenient and highly secure | Requires hardware support and can raise privacy concerns |
Social Login | Easy for users with existing accounts | Depends on third-party services |
Comprehensive Guide to iOS App Development Login Features
When developing an iOS application, implementing a secure and user-friendly login system is critical for providing a seamless user experience. The login process must not only be intuitive but also secure, ensuring user data is protected. In this guide, we will explore essential aspects of login features in iOS apps, focusing on authentication methods, best practices, and how to implement these features efficiently.
For developers, understanding the available tools and techniques is key to creating a reliable and scalable login system. From integrating third-party authentication providers to customizing the login interface, there are various options to consider. Below, we discuss the core components and strategies for implementing an effective login flow in iOS applications.
Key Authentication Methods in iOS Apps
- Email and Password Authentication: The most common method for securing user accounts, often paired with password hashing for improved security.
- Social Media Authentication: Allows users to log in via Facebook, Google, or Apple ID, streamlining the login process and reducing friction.
- Two-Factor Authentication (2FA): Adds an extra layer of security, requiring users to verify their identity through an additional method, such as an SMS code.
- Biometric Authentication: Utilizing Face ID or Touch ID for a seamless and secure login experience.
Best Practices for Implementing Login Features
- Secure Password Storage: Always store passwords using secure hashing algorithms like bcrypt or PBKDF2 to prevent data breaches.
- Token-based Authentication: Use JWT (JSON Web Tokens) for managing user sessions instead of traditional session-based cookies.
- Input Validation: Ensure proper validation of user input to prevent injection attacks and improve the overall security of the app.
- Persistent Login: Consider implementing the option for users to stay logged in across app sessions while maintaining security through encrypted tokens.
Considerations for Customizing the Login Interface
Feature | Description |
---|---|
Login Form | Ensure a clean and simple form, avoiding unnecessary fields while providing clear error messages for invalid input. |
Forgot Password | Offer users a simple method to recover their password via email or SMS, with secure validation steps. |
Social Login Buttons | Provide clear, easily accessible social login buttons for users who prefer not to create a new account. |
Important: Always prioritize user privacy and data protection when implementing login features. Comply with regulations such as GDPR or CCPA if applicable to your app’s user base.
Key Considerations When Designing a Secure iOS Login Interface
When designing a secure login system for an iOS app, the goal is to ensure that users can authenticate easily while keeping their data safe. A well-designed login screen is not just user-friendly, but also resistant to common threats such as data interception, brute force attacks, and unauthorized access. Security should be at the forefront of every design decision, from how credentials are collected to how sensitive data is stored and transmitted.
In addition to protecting user credentials, an effective login interface also prioritizes accessibility and usability. While security measures like encryption and multi-factor authentication are crucial, the user experience should not be compromised. Striking the right balance between these elements is key to building a secure and functional authentication flow.
Critical Aspects for a Secure Login Interface
- Password Protection: Ensure that passwords are encrypted before they are transmitted over the network. Use secure hash functions like bcrypt or PBKDF2 to prevent password theft during transmission.
- Multi-Factor Authentication (MFA): Implementing MFA, such as SMS or email verification, adds an extra layer of protection, making it harder for attackers to gain unauthorized access.
- Secure Data Storage: Never store sensitive information like passwords in plaintext. Use iOS's keychain services to securely store authentication tokens.
Recommended Design Practices
- Use HTTPS: Always ensure that login credentials are transmitted via HTTPS to prevent eavesdropping and man-in-the-middle attacks.
- Account Lockout: After a certain number of failed login attempts, temporarily lock the account or introduce a delay to prevent brute force attacks.
- Password Visibility Toggle: Allow users to toggle the visibility of their password, which helps them ensure they’ve entered it correctly without compromising security.
“Security is not just a technical challenge, but a design challenge. A login interface should be both secure and easy to use, ensuring that users don't have to sacrifice one for the other.”
Important Data Security Measures
Security Feature | Implementation Details |
---|---|
Secure Socket Layer (SSL) | Always use SSL/TLS for encrypting data during transmission. |
Password Hashing | Use algorithms like bcrypt to hash and store passwords securely. |
Two-Factor Authentication (2FA) | Encourage users to enable 2FA for an added layer of security during login. |
Step-by-Step Process of Integrating Authentication APIs into Your iOS App
Integrating authentication into your iOS application is a crucial step to ensure secure access to users' personal data. By connecting your app with authentication APIs, you can easily manage user logins, sign-ups, and data protection. This process can vary depending on the authentication service provider (such as Firebase, OAuth, or a custom API), but the general workflow remains consistent.
Here is a detailed guide on how to incorporate an authentication API into your iOS application, along with some important considerations to keep in mind for a seamless user experience.
1. Set Up the Authentication API
The first step is to choose the right authentication provider and integrate its SDK into your app. Below is the process of setting up a service like Firebase Authentication:
- Register your app with the chosen provider (e.g., Firebase).
- Download and install the provider’s SDK using Swift Package Manager or CocoaPods.
- Configure the SDK within your app by initializing it in the AppDelegate or SceneDelegate.
- Verify the API keys and set up any necessary permissions in your app’s settings.
2. Implement the Login UI
Designing a user-friendly login interface is essential. Here’s how to implement a basic login screen:
- Create UI elements like text fields for the email and password, and buttons for login and sign-up.
- Use delegates to handle user input and validate the entered credentials.
- Handle edge cases such as invalid email format or weak passwords with appropriate feedback.
3. Handle Authentication Flow
Once the UI is set up, implement the authentication logic. Depending on the API, this usually involves the following:
Important: Always ensure that sensitive data like passwords or tokens are stored securely, preferably in the iOS Keychain.
Step | Action |
---|---|
Login | Use the authentication API to verify user credentials and retrieve a token. |
Sign-Up | Collect the user’s email and password, then register the new account with the API. |
Logout | Call the API to log out the user and clear the session data. |
4. Handle Errors and Edge Cases
Make sure your app handles errors such as incorrect credentials, network issues, or authentication timeouts gracefully. Provide clear error messages to the user so they understand what went wrong and how to resolve it.
How to Implement Biometric Authentication for Seamless User Login
Integrating biometric authentication into your iOS app provides users with a quick and secure login experience. It enhances user engagement by eliminating the need for passwords, offering a smoother and safer alternative. This feature takes advantage of technologies such as Face ID and Touch ID, which are supported natively on iOS devices, making it easier to implement without external libraries.
To implement biometric authentication, you need to leverage the Local Authentication framework, which provides access to biometric sensors. It allows your app to request authentication via Face ID, Touch ID, or even the device passcode when biometrics are unavailable or disabled.
Steps to Set Up Biometric Authentication
- Import the Local Authentication Framework: Start by adding the necessary imports to your project.
- Check Device Compatibility: Before triggering authentication, ensure the device supports biometrics and that the user has set it up.
- Request Authentication: Use the `LAContext` class to initiate the biometric authentication process.
- Handle Success or Failure: Implement callbacks to handle the result, whether successful or if an error occurs.
Important: Always provide an alternative login method (like a passcode) in case biometric authentication fails or is not available on the device.
Code Example
import LocalAuthentication let context = LAContext() var error: NSError? if context.canEvaluatePolicy(.deviceOwnerAuthenticationWithBiometrics, error: &error) { context.evaluatePolicy(.deviceOwnerAuthenticationWithBiometrics, localizedReason: "Log in using your fingerprint or face") { success, authenticationError in if success { // Proceed to authenticated area } else { // Handle error } } } else { // Handle biometric unavailability }
Biometric Authentication vs. Traditional Login
Feature | Biometric Authentication | Traditional Login |
---|---|---|
Security | Highly secure, based on unique physical traits | Depends on password strength, vulnerable to hacking |
Speed | Instant authentication with face or fingerprint recognition | Time-consuming, requires remembering and typing passwords |
User Experience | Seamless, minimal effort from user | Can be frustrating, especially if passwords are forgotten |
By incorporating biometric authentication, you offer users a modern, fast, and secure login experience that aligns with current trends in app security. Always ensure to test thoroughly across devices and handle error cases gracefully to maintain a high level of user satisfaction.
Ensuring Data Privacy and Secure Storage for Login Credentials in iOS Apps
When developing an iOS app with user authentication, it is crucial to protect users' login information from unauthorized access. Safeguarding login credentials requires using secure storage mechanisms and employing best practices to prevent potential data breaches. With the increasing importance of privacy regulations like GDPR and CCPA, developers must integrate robust solutions to ensure that sensitive information is never exposed or compromised.
To maintain high levels of security, iOS offers several built-in tools and guidelines, such as Keychain and Secure Enclaves, which enable secure storage of passwords and tokens. However, simply relying on these tools is not enough; developers must also follow industry standards and implement strong encryption methods for data transmission and access controls.
Secure Storage Practices
- Keychain Services: Use the iOS Keychain to store sensitive data, such as passwords or authentication tokens. This system provides encryption and access control mechanisms.
- Encrypted Storage: Implement AES or RSA encryption algorithms to further protect data at rest within the app.
- Secure Enclave: Leverage the Secure Enclave to store highly sensitive data like biometric data (Face ID, Touch ID) or cryptographic keys.
Best Practices for Data Handling
- Data Encryption: Always encrypt data both at rest and in transit. Use HTTPS and TLS for secure communication between the app and server.
- Two-Factor Authentication (2FA): Implement 2FA to add an extra layer of security for user logins.
- Minimize Data Retention: Only store necessary user data and remove it from the system once it is no longer required.
"It is not enough to just use encryption–secure coding practices and thorough testing are essential to mitigate potential vulnerabilities."
Security Overview Comparison
Method | Description | Pros | Cons |
---|---|---|---|
Keychain | A secure storage solution for passwords and cryptographic keys. | Integrated with iOS, offers encryption, and is simple to implement. | Limited to iOS devices; not suitable for large-scale data storage. |
Secure Enclave | A hardware-based secure element designed to protect sensitive data. | Provides the highest level of security for sensitive data. | Device-dependent and more complex to implement. |
Handling Login Failures and Error Messages for User Clarity
When developing a login feature for an iOS app, handling authentication errors is crucial to ensure a smooth user experience. Users should be informed about the reason for their failed login attempt in a clear and helpful manner. This helps in preventing confusion and frustration, which can negatively affect user retention. Proper error handling goes beyond simply showing an error message; it requires understanding the nature of the issue and presenting it in an actionable way.
To enhance user understanding, error messages should be specific, concise, and easily understood. Generic messages like "Login failed" should be avoided. Instead, provide a clear explanation such as "Incorrect username or password" or "Account locked due to multiple failed login attempts." Additionally, error handling can be paired with visual cues and actionable steps to guide the user in resolving the issue efficiently.
Best Practices for Error Handling
- Be Specific: Instead of vague messages, specify the exact issue such as "Password incorrect" or "Email not registered."
- Provide Actionable Steps: If the user has forgotten their password, provide a link to reset it.
- Avoid Overloading with Information: Too much detail can overwhelm the user. Keep messages brief and to the point.
- Use Visual Cues: Highlight the specific input field that caused the error to make the process smoother for the user.
Example Error Handling Flow
- User attempts to log in with invalid credentials.
- The app displays a message: "The username or password you entered is incorrect."
- A link to reset the password is provided below the error message.
- If the user continues to fail, a warning is displayed: "Your account is temporarily locked. Please try again later."
Helpful Error Message Table
Error Type | Message | Next Step |
---|---|---|
Invalid Credentials | Incorrect username or password | Try again or reset your password |
Account Locked | Account locked due to multiple failed attempts | Wait 15 minutes or reset your password |
Email Not Found | The email address is not registered | Sign up for a new account |
Important: Providing a detailed error message helps users quickly resolve issues and get back to using the app. It also reduces the likelihood of user frustration.
Best Practices for Managing User Sessions and Token Expiration
Efficient management of user sessions and the handling of token expiration are critical elements in ensuring both security and user experience in iOS applications. Proper implementation ensures that users are not continuously prompted to log in while maintaining a secure environment. Token expiration, on the other hand, helps prevent unauthorized access in case a session is left open or a token is compromised.
To optimize the handling of these factors, it's crucial to use industry-standard protocols, such as OAuth 2.0, and implement secure token storage mechanisms. Additionally, automatic token renewal and proper session invalidation are key practices to improve both security and usability.
Key Strategies for Handling Sessions and Token Expiration
- Token Storage: Store tokens securely using Keychain for iOS apps to ensure sensitive information is encrypted.
- Token Refresh: Implement automatic token refresh mechanisms to renew tokens before they expire, minimizing the need for users to manually log in.
- Session Timeout: Set a session timeout value to automatically log out users after a period of inactivity, reducing the risk of unauthorized access.
Steps to Improve Token Management
- Check Expiration Before Making API Requests: Always validate token expiration before sending a request. If expired, initiate a refresh process.
- Refresh Tokens Using Secure Methods: Use a secure endpoint for refreshing tokens to ensure that the token renewal process is safe from interception.
- Invalidate Tokens After Logout: Ensure that tokens are completely invalidated when the user logs out to prevent session hijacking.
Handling Expired Tokens
Scenario | Recommended Action |
---|---|
Token Expired | Attempt to refresh the token using the refresh token. If unsuccessful, prompt the user to log in again. |
Token Revoked | Immediately log out the user and ask them to log in again. |
Important: Always implement secure token storage and transmission protocols to prevent data leakage and potential security breaches.
The Role of OAuth in Simplifying Third-Party Login Integrations
OAuth is a protocol that plays a crucial role in streamlining the integration of third-party login services in mobile applications. It enables users to authenticate using their existing accounts from services like Google, Facebook, or Twitter, without the need for developers to handle sensitive login data. By using OAuth, developers can save time and effort in managing user authentication and security processes, as the authentication is delegated to trusted third-party providers.
OAuth allows users to grant access to their information securely, without sharing their passwords with third-party apps. This reduces the risk of data breaches and enhances the overall user experience by providing a quicker and more secure way to log in to applications. Below is a breakdown of the key benefits and functionality of OAuth in third-party login systems:
- Secure Authentication: OAuth ensures that passwords are never exposed to the application, reducing security risks.
- User Convenience: Users can quickly log in with their existing accounts, saving time and effort.
- Reduced Development Time: OAuth simplifies the process of integrating login systems, minimizing the need to build custom authentication mechanisms.
OAuth Workflow
OAuth works through a series of steps to authenticate users without exposing their credentials:
- Authorization Request: The application redirects the user to the third-party service's authorization page.
- User Authorization: The user grants or denies the requested permissions for the app.
- Authorization Code: If the user authorizes the request, the third-party service sends an authorization code to the app.
- Access Token: The app exchanges the authorization code for an access token, allowing access to the user's data.
OAuth allows developers to avoid handling sensitive login information, making the process both secure and efficient.
Comparison of OAuth and Traditional Login
Feature | OAuth | Traditional Login |
---|---|---|
Security | High (no password sharing) | Moderate (passwords handled directly) |
Ease of Use | Fast login with existing accounts | Users must create new credentials |
Development Effort | Low (leveraging existing services) | High (requires custom authentication) |
Testing and Debugging Login Functionality in iOS Applications
Testing and debugging login functionality is crucial for ensuring the security, usability, and performance of iOS applications. A seamless login process is key to user experience, and thorough testing is required to guarantee that all potential issues are addressed before release. The testing phase focuses not only on the correctness of the login but also on factors such as speed, data validation, and error handling.
Common errors during login testing can range from UI glitches to failed API calls or incorrect error messages. Debugging these issues often requires isolating specific problems within the login flow, which involves using various debugging tools and techniques to pinpoint the source of the malfunction.
Key Testing Areas
- Input Validation: Ensuring that all input fields (username, password) are validated correctly before submission.
- API Integration: Testing the communication with the server to ensure that the credentials are properly verified.
- Error Handling: Proper display of error messages for invalid inputs or connection issues.
- Performance: Checking that the login process runs smoothly without noticeable delays or crashes.
Steps to Debugging iOS Login Features
- Reproduce the Issue: Try to recreate the problem in different scenarios (incorrect credentials, no internet, etc.) to identify any patterns.
- Use Breakpoints: Set breakpoints in the code to analyze how data flows during the login process.
- Check Console Logs: Review the Xcode console logs for error messages and warnings that might indicate a specific issue.
- Monitor Network Requests: Use tools like Charles Proxy or the network debugger in Xcode to check if requests are being made correctly and responses are received as expected.
Effective debugging relies on narrowing down the source of the issue to a specific component in the login flow. Start with the most likely causes, such as input validation or network connectivity, before diving deeper into code logic.
Testing Tools
Tool | Purpose |
---|---|
Xcode Debugger | Used for setting breakpoints and examining the flow of data through the app. |
Charles Proxy | Helps in monitoring and analyzing network requests and responses. |
UI Testing Framework | Automates user interface testing to simulate various login scenarios. |