Android App Development Setup
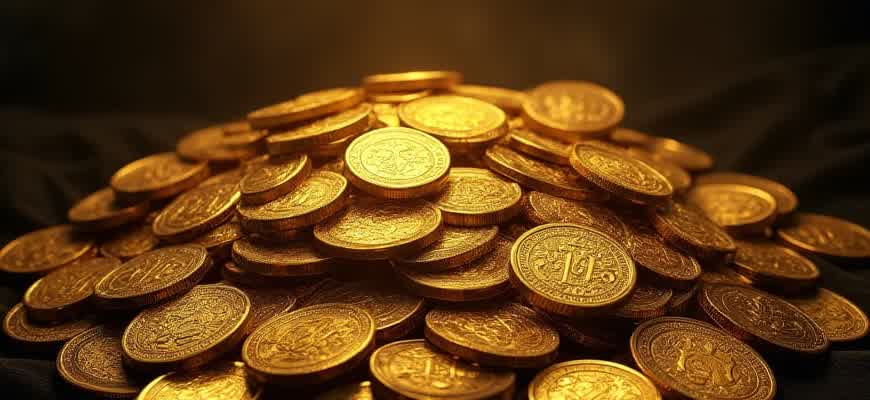
To start building Android applications, you need to establish the proper development environment. Here are the essential steps to get up and running:
- Install Android Studio – Android Studio is the official integrated development environment (IDE) for Android. It provides all the tools needed to create, test, and debug your app.
- Set Up Android SDK – The Software Development Kit (SDK) includes necessary libraries and tools for building Android apps. Make sure to download and install the latest version.
- Configure Emulator or Device – You can run your app either on an Android emulator or a physical Android device. Ensure that your device is set up for development by enabling developer options and USB debugging.
Make sure to keep your Android Studio and SDK updated to avoid compatibility issues with the latest Android versions.
Here is a table summarizing the tools needed for Android development:
Tool | Description | Installation Link |
---|---|---|
Android Studio | IDE for Android development, includes everything you need to build and test apps. | Download |
Android SDK | Library and toolset required for building Android apps. | Download |
Android Emulator | Software that mimics Android devices on your computer. | Setup Guide |
Choosing the Right Development Environment for Android
When it comes to developing Android applications, selecting the appropriate development environment is crucial for a smooth and efficient workflow. The right tools and platform can significantly enhance productivity and minimize development challenges. There are multiple options available, but understanding the core features and limitations of each can help developers make an informed choice.
Two main development environments dominate Android app creation: Android Studio and alternative lightweight editors. While Android Studio provides an all-in-one solution with integrated tools for building, testing, and deploying apps, other editors like IntelliJ IDEA and Visual Studio Code offer flexibility and less overhead. The choice largely depends on the complexity of the project, performance needs, and personal preference.
Key Development Environment Options
- Android Studio: The official IDE for Android development. Offers extensive tools, including emulators, performance analyzers, and UI designers.
- IntelliJ IDEA: A full-featured IDE that supports Android development with a focus on Java and Kotlin. Known for its deep code analysis and integrations.
- Visual Studio Code: A lightweight code editor, often preferred for smaller projects or developers looking for a minimalist approach. Offers Android development via plugins.
Advantages and Disadvantages
Environment | Advantages | Disadvantages |
---|---|---|
Android Studio | Comprehensive tools, official support, great for large projects. | Heavier on system resources, may be overkill for small projects. |
IntelliJ IDEA | Highly efficient, powerful for Java and Kotlin, good performance. | Can be complex for beginners, limited Android-specific tools compared to Android Studio. |
Visual Studio Code | Fast, minimal setup, flexible, and great for smaller apps. | Lacks native Android tools, less integrated for large-scale development. |
Choosing the right IDE should be aligned with the project requirements. If you need extensive Android-specific features and official support, Android Studio is the clear winner. For smaller or more experimental projects, lightweight editors can save you time and resources.
Installing Android Studio and SDK Tools
To begin developing Android applications, you need to set up the necessary environment. The first step is installing Android Studio, which includes all the required SDK tools. Android Studio is the official IDE provided by Google, and it streamlines the development process with its built-in features, including code completion, debugging, and performance analysis.
The installation process is straightforward, but there are a few key steps to ensure everything is properly set up. In this section, we'll guide you through downloading and installing Android Studio along with the required SDK tools.
Downloading and Installing Android Studio
Follow these steps to install Android Studio on your system:
- Visit the official Android Studio website at developer.android.com/studio.
- Select the appropriate version for your operating system (Windows, macOS, or Linux).
- Download the installer and run the setup file.
- Follow the on-screen instructions to complete the installation.
- Launch Android Studio after installation is complete.
Setting Up SDK Tools
During the installation of Android Studio, the SDK tools are also installed automatically. However, you might need to configure or update them manually in certain cases.
Note: If the Android SDK is not installed, you can set it up through the SDK Manager in Android Studio.
- Open Android Studio and click on "SDK Manager" located in the toolbar.
- Check that the latest version of the Android SDK and the required tools are installed, such as Android SDK Platform-Tools and Android SDK Build-Tools.
- If any tools are missing, use the SDK Manager to download and install them.
Additional Information
Component | Description |
---|---|
Android Studio | Official Integrated Development Environment (IDE) for Android development. |
SDK Tools | Includes Android SDK, Platform Tools, Build Tools, and Emulator for testing apps. |
SDK Manager | Tool for managing Android SDK versions and components. |
Setting Up Android Emulator for Testing Your Apps
To test your Android applications efficiently, using the Android Emulator is essential. This tool allows you to run your app on a virtual device, mimicking a range of devices with different screen sizes, resolutions, and Android versions. Before you begin testing, you must first set up the emulator environment on your system. This ensures that your app behaves correctly on various devices without needing physical hardware.
The process involves installing Android Studio, configuring the Android Virtual Device (AVD), and ensuring that the emulator can run smoothly. Once everything is set up, you can easily test, debug, and optimize your application in various virtual environments without needing real devices for each configuration.
Installing the Android Emulator
The first step in setting up the emulator is installing Android Studio, which includes the necessary components for running virtual devices. Follow these steps:
- Download and install Android Studio from the official website.
- Open Android Studio and select the "SDK Manager" from the toolbar.
- Under the "SDK Tools" tab, check the box for "Android Emulator" and click "Apply".
Creating a Virtual Device (AVD)
Once the emulator is installed, you need to create a virtual device to run your app. Here's how you can do it:
- Go to "Tools" > "AVD Manager" in Android Studio.
- Click on "Create Virtual Device" and choose a hardware profile (e.g., Pixel 5, Nexus 6P).
- Select a system image based on the desired Android version.
- Configure the AVD with options like RAM, storage, and graphics acceleration.
- Click "Finish" to create the virtual device.
Running the Emulator
Once your AVD is set up, you can launch the emulator and run your app:
- Open your project in Android Studio.
- Click on the "Run" button and select the virtual device you just created.
- The emulator will start, and your app will be installed and launched on it.
Important: The emulator's performance can vary depending on your computer's hardware. Enabling hardware acceleration can improve the emulator's speed.
AVD Configuration Options
Here are some common configurations you can adjust when setting up your AVD:
Option | Description |
---|---|
RAM | Defines the amount of memory allocated to the virtual device. Higher RAM may improve performance but use more resources. |
Graphics | Choose between "Automatic", "Hardware", or "Software" for rendering the graphics in the emulator. |
Device Orientation | Set whether the virtual device starts in portrait or landscape mode. |
Configuring Java Development Kit (JDK) for Android Development
Setting up the Java Development Kit (JDK) is a crucial step for Android development. The JDK provides the necessary tools and libraries that allow developers to write, compile, and run Java applications, which are essential for Android app development. Without a properly configured JDK, the Android Studio IDE cannot compile Java code, and thus, the Android development process will be hindered.
To ensure smooth Android app development, you must install the correct version of the JDK and configure it within your development environment. This process involves downloading the JDK, installing it, and linking it to Android Studio so that the Android SDK can use the Java tools effectively.
Steps to Configure JDK
- Download the JDK: Visit the official Oracle website or adopt OpenJDK for your platform.
- Install the JDK: Follow the installation steps based on your OS (Windows, macOS, or Linux).
- Set the JAVA_HOME Environment Variable: Define the JDK path in your system's environment variables.
- Link JDK to Android Studio: In Android Studio, set the JDK path in the settings to point to the installed JDK directory.
Configuring JAVA_HOME Variable
- Open System Properties (for Windows) or Terminal (for macOS/Linux).
- Navigate to Environment Variables and create a new variable called JAVA_HOME with the JDK installation path.
- For Windows, use:
C:\Program Files\Java\jdk-x.x.x
- For macOS/Linux, use:
/usr/lib/jvm/java-x-openjdk
Important Notes
Ensure that your JDK version matches the one required by the Android Gradle plugin to avoid compatibility issues.
JDK Versions and Compatibility
JDK Version | Supported Android Studio Version |
---|---|
JDK 8 | Android Studio 3.x and earlier |
JDK 11 | Android Studio 4.x and later |
Setting Up a Device for Debugging via USB or Wi-Fi
To begin debugging your Android app, it’s necessary to prepare your device for communication with Android Studio. This process involves enabling specific settings on your device to allow a successful connection, whether through USB or over a Wi-Fi network. Each method has its own steps, but both offer the same goal: to test your app on an actual Android device instead of an emulator.
There are two primary ways to connect your Android device for debugging: USB connection or Wi-Fi. Both methods require enabling Developer Options and USB debugging on the device, but the setup steps differ slightly for each connection method.
USB Debugging Setup
Follow these steps to enable USB debugging on your device:
- Open "Settings" on your Android device.
- Navigate to "About phone" and tap on it.
- Find "Build number" and tap it seven times to unlock Developer Options.
- Return to the main "Settings" screen and go to "Developer options".
- Toggle "USB debugging" on.
After enabling USB debugging, connect the device to your computer via USB. Ensure that Android Studio detects the device and appears in the device dropdown for testing.
Important: If this is your first time connecting the device, a prompt will appear asking for permission to allow USB debugging. Confirm this by tapping "OK."
Wi-Fi Debugging Setup
To debug your app wirelessly, follow these additional steps:
- Make sure both your device and computer are on the same Wi-Fi network.
- Enable USB debugging on your device (as shown in the previous section).
- Connect your device to the computer via USB initially.
- In Android Studio, open the "Terminal" window and enter the following command:
adb tcpip 5555
. - Disconnect the USB cable and find the IP address of your device in the "Settings" under "Wi-Fi" or "Network" settings.
- Enter the following command to connect via Wi-Fi:
adb connect
.:5555
Once connected, you can now debug the app wirelessly. This setup is particularly useful for testing apps without the limitation of cables.
Important: Wi-Fi debugging might be slower than a USB connection due to network latency. Ensure your network is stable for optimal performance.
Device Setup Comparison
Method | Steps | Connection Type |
---|---|---|
USB Debugging | Enable USB debugging, connect via USB cable | Wired |
Wi-Fi Debugging | Enable USB debugging, use ADB commands to switch to Wi-Fi | Wireless |
Integrating Firebase with Your Android Project
Firebase is a powerful platform that offers a variety of backend services for mobile applications. Integrating Firebase with an Android project can enhance functionality by enabling features such as authentication, real-time databases, cloud storage, and more. The process requires setting up Firebase in the Android Studio environment and configuring the necessary services in the Firebase Console.
Here is a step-by-step guide on how to integrate Firebase with your Android application:
Steps to Add Firebase to Your Android Project
- Go to the Firebase Console and create a new project.
- Link your Android project to the Firebase Console by registering your app.
- Download the google-services.json file and add it to your project’s app directory.
- Include the Firebase SDK in your project’s build.gradle files.
- Sync your project to ensure all dependencies are downloaded.
It is important to sync your project after making any changes to the gradle files to ensure the Firebase SDK is properly linked.
Essential Firebase Services for Android Apps
Service | Description |
---|---|
Firebase Authentication | Manages user authentication with email, social media logins, and more. |
Firebase Realtime Database | Provides a NoSQL cloud database for real-time syncing across devices. |
Firebase Cloud Storage | Stores and manages user-generated content, such as images and videos. |
Once the setup is complete, you can start integrating the desired Firebase services into your application. Firebase simplifies tasks like user management and data synchronization, making your app development process more efficient and scalable.
Setting Up Version Control with Git for Android Development
Version control is an essential tool for managing code changes and collaborating on Android projects. Git, as the most widely used version control system, provides the necessary features to track changes, manage branches, and merge work efficiently. Properly configuring Git within your Android development environment ensures that your project remains organized and that every team member can collaborate seamlessly.
To integrate Git into your Android development workflow, you will need to follow specific steps to ensure that both the local and remote repositories are correctly set up. This configuration will allow you to push changes to platforms like GitHub or GitLab and maintain a clean version history.
Steps to Configure Git for Your Android Project
- Install Git: Ensure that Git is installed on your local machine. You can download and install Git from here.
- Initialize Git Repository: Open your project in Android Studio and initialize a new Git repository by running the following command in the terminal:
git init
- Commit Initial Code: Add your project files to the staging area and commit them to the local repository:
git add .
git commit -m "Initial commit"
- Set Up Remote Repository: Create a new repository on GitHub, GitLab, or another platform and link it to your local repository:
git remote add origin
- Push Changes to Remote: Push your local commits to the remote repository:
git push -u origin master
Best Practices for Git in Android Projects
Ensure you commit changes frequently and with clear messages. This makes it easier to track progress and collaborate with other developers.
- Branching: Use branches for new features or bug fixes. This keeps the main branch stable while you develop new code.
git checkout -b feature/new-feature
- Git Ignore: Create a .gitignore file to avoid committing unnecessary files such as build outputs, logs, or local configurations. Android Studio automatically provides a default .gitignore template.
- Commit Messages: Write concise and meaningful commit messages. Use the format: "type: message". For example:
fix: resolved crash on startup
Git Configuration in Android Studio
Action | Command |
---|---|
Set user name | git config --global user.name "Your Name" |
Set user email | git config --global user.email "[email protected]" |
Building and Running Your First Android Application
Creating your first Android application involves several essential steps, starting from setting up the development environment to running the app on an emulator or real device. Once you have installed Android Studio and configured your project, it's time to start coding and testing your app. This guide will walk you through the key steps to successfully build and run your first Android project.
To begin with, Android Studio is the official Integrated Development Environment (IDE) for Android development. It provides all the necessary tools for building apps, including a code editor, emulator, and debugging features. After creating your project, Android Studio will generate default files and settings for your app. Let's look at how to compile and run it.
Steps to Build and Run Your First Android Application
- Launch Android Studio and create a new project.
- Choose an app template and configure the project name, package, and save location.
- Once the project is created, navigate to the MainActivity.java file and modify the code.
- In the res folder, customize the UI by editing activity_main.xml.
- Run your project on an emulator or physical device to test its functionality.
Important: Always check if the Android SDK and necessary tools are up to date before building your app. You can do this in the "SDK Manager" within Android Studio.
Running the Application
- Click on the "Run" button (green triangle) in the toolbar.
- Select a virtual device or connect a physical Android device via USB.
- Wait for the app to be built and deployed on the selected device.
- After successful deployment, the app will launch automatically, and you can interact with it.
Emulator | Physical Device |
---|---|
Requires setting up a virtual device in Android Studio. | Simply connect via USB and enable developer options. |
Ideal for testing without needing real hardware. | Provides real-world testing for performance and behavior. |