Xcode Ecommerce App
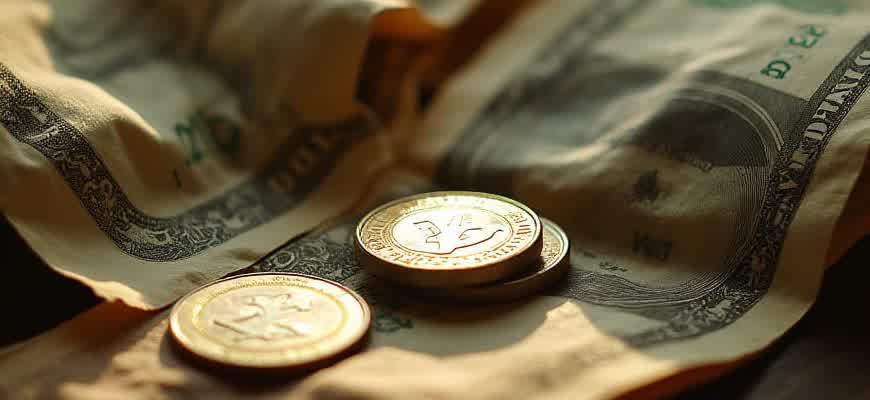
Xcode provides a comprehensive set of tools for developing iOS applications, including those focused on e-commerce. When creating an e-commerce app, developers leverage Xcode's powerful features like Swift, UIKit, and CoreData to build seamless user experiences and efficient back-end operations. The process typically involves integrating product catalogs, secure payment gateways, and user authentication systems.
Key Features of an E-commerce App Built with Xcode:
- Customizable product pages with high-quality images and detailed descriptions.
- Secure payment processing using Apple Pay or third-party services.
- Real-time order tracking and notifications for users.
- User account management, including registration, login, and password recovery.
Development Steps Overview:
- Setting up the project in Xcode and configuring necessary dependencies.
- Designing the app interface with Interface Builder for a responsive layout.
- Implementing core functionality like product listing, user authentication, and checkout processes.
- Testing and debugging the app for performance and security issues.
It's crucial to focus on the user experience (UX) to ensure that the app is intuitive and efficient, encouraging users to complete their purchases with ease.
Component | Description | Tools Used |
---|---|---|
Product Catalog | Display and categorize products for easy browsing. | CoreData, SwiftUI |
Payment Gateway | Securely process transactions via Apple Pay or other services. | Stripe SDK, Apple Pay API |
Order Management | Track user orders and update their statuses. | Firebase, CoreData |
Integrating Payment Gateways: Step-by-Step with Xcode
When building an e-commerce app in Xcode, integrating a payment gateway is a crucial step for enabling users to make transactions. A seamless payment integration ensures both security and usability, which are essential for a positive user experience. By utilizing iOS SDKs or third-party services like Stripe or PayPal, developers can add payment functionality to their apps efficiently.
In this guide, we will walk you through the process of integrating a payment system into your Xcode project. The integration process involves setting up the payment SDK, handling user input securely, and managing the transaction lifecycle.
Steps to Integrate Payment Gateway in Xcode
- Step 1: Choose a Payment Gateway
- Select a payment service provider (e.g., Stripe, PayPal, or Square) based on your needs.
- Ensure the provider supports iOS integration.
- Step 2: Install the SDK
- For Stripe, use CocoaPods to add the Stripe SDK to your project.
- For PayPal, install the PayPal iOS SDK via CocoaPods or Carthage.
- Step 3: Set Up API Keys
- Obtain API keys from the provider's dashboard and configure them in your app’s settings.
- Step 4: Implement Payment UI
- Design the user interface with text fields for card details and buttons for payment submission.
- Ensure the UI is simple and user-friendly to minimize errors during payment processing.
- Step 5: Handle Transactions
- Use the SDK’s API to send payment details securely to the gateway.
- Implement transaction callbacks to confirm successful payments and handle errors.
Important: Always use secure connections (HTTPS) to handle sensitive data such as credit card numbers.
Transaction Flow in a Payment Integration
Step | Action |
---|---|
Step 1 | Collect payment information securely from the user. |
Step 2 | Send payment data to the gateway API for processing. |
Step 3 | Receive payment status and update the app's interface. |
Tip: Test your payment gateway integration thoroughly using sandbox environments provided by the payment services.
Implementing Secure User Authentication in Your Xcode Ecommerce App
For any ecommerce app, user authentication plays a critical role in ensuring security and protecting sensitive data. In Xcode, there are several strategies to implement secure authentication that will keep your app safe from unauthorized access. These methods include integrating with authentication services like Firebase, implementing two-factor authentication (2FA), and securely storing user credentials using the iOS Keychain.
Here’s a step-by-step guide to implementing secure user authentication in your Xcode ecommerce app, focusing on best practices and security considerations.
1. Use a Secure Authentication Service
- Integrate with Firebase Authentication or OAuth for handling user login and registration securely.
- Firebase provides pre-built UI components, so you can avoid creating your own authentication flow, reducing the potential for errors.
- Ensure that all authentication data is transmitted via HTTPS to prevent data leaks.
2. Securely Store User Data
Never store sensitive user information (such as passwords) in plain text. Instead, use the iOS Keychain to securely store authentication tokens and sensitive data.
Tip: The Keychain encrypts stored data and provides a secure way to persist authentication information even if the app is uninstalled and reinstalled.
3. Implement Two-Factor Authentication (2FA)
- Enable 2FA in your app to add an extra layer of security.
- Use SMS or email verification for the second factor.
- Ensure the second factor is time-sensitive to prevent unauthorized access.
4. Secure Network Communication
All user credentials should be transmitted over HTTPS, and SSL certificates should be properly validated to protect user data from man-in-the-middle attacks.
Security Feature | Implementation |
---|---|
HTTPS | Ensure all API calls are made over HTTPS for secure data transmission. |
SSL Pinning | Implement SSL pinning to verify the server’s identity and prevent connection to rogue servers. |
Optimizing Checkout Speed in Ecommerce Apps
In any ecommerce application, one of the most critical factors influencing conversion rates is the checkout process. A slow and cumbersome checkout can lead to frustrated customers abandoning their carts. In order to ensure that users have a smooth and fast checkout experience, it's essential to focus on optimizing app performance. With careful attention to loading times, data handling, and UI/UX, the app can offer a seamless checkout experience that boosts user retention and satisfaction.
One way to optimize the checkout process is by reducing the number of unnecessary requests and minimizing the payload. This can be achieved through data caching, optimizing API calls, and reducing image and asset sizes. Additionally, leveraging efficient algorithms for payment processing and order validation helps prevent bottlenecks during the checkout flow. Below are key strategies to implement for improving performance.
Key Strategies to Speed Up Checkout
- Reduce image and asset sizes: Compress images and use modern formats like WebP to minimize load times.
- Optimize API calls: Batch requests and ensure that data fetching only happens when necessary to avoid redundant calls.
- Use background processing: Offload time-consuming tasks, like payment processing, to run in the background while the user continues interacting with the app.
- Preload critical data: Cache frequently used data such as shipping addresses or payment methods, so users don’t have to re-enter them each time.
- Implement lazy loading: Delay loading of non-essential elements on the checkout page to ensure the main content loads quickly.
Important: A good rule of thumb is to aim for a page load time under 3 seconds. Anything longer can lead to a significant drop in conversion rates.
Recommended Techniques
- Use a Content Delivery Network (CDN) to serve assets from the closest server location, minimizing latency.
- Apply efficient state management to minimize re-renders, keeping the UI responsive even during complex data updates.
- Implement form validation as the user types to prevent delays when submitting data.
- Optimize payment gateways by choosing providers with faster response times and ensuring your app integrates with them properly.
Performance Benchmarks
Optimization Technique | Expected Impact |
---|---|
Image Compression | Reduces load times by up to 40% |
Lazy Loading | Improves page load by up to 30% |
API Call Optimization | Decreases response time by 50% |
Tip: Always test performance using tools like Xcode Instruments to track real-time performance during development.
Creating a Smooth Mobile Shopping Cart with Xcode
Building an efficient shopping cart for an e-commerce app involves both seamless functionality and a smooth user experience. Xcode provides all the necessary tools to develop an interactive and responsive cart system for iOS apps. The key components for success are good UI design, backend integration, and user-friendly features like item modification and real-time updates.
In this guide, we'll explore the core steps to implement a dynamic shopping cart using Xcode. From handling the user interface to managing the data flow, you'll see how to combine the power of Swift and Xcode’s Interface Builder to create a truly integrated experience.
Key Features to Implement in Your Shopping Cart
- Item Selection: Allow users to easily add, update, or remove items.
- Quantity Management: Provide controls for users to adjust quantities of selected items.
- Real-Time Price Updates: Ensure the cart displays updated pricing when changes are made.
- Order Summary: Show a clear breakdown of the selected products, including taxes and shipping costs.
Steps to Build the Cart Interface
- Create a Product Model to represent individual items, including details like name, price, and quantity.
- Use UITableView to list items in the cart, allowing users to scroll through their selections.
- Integrate a UIViewController to manage interactions, such as adding/removing items and updating quantities.
- Use Core Data or a similar framework for persistent data storage to remember cart contents across app sessions.
Backend Integration and Data Flow
The backend plays a critical role in syncing cart information with your server and processing orders. When users add or remove items, the app should update the cart both locally and on the server. It’s essential to handle both network errors and API responses to keep the cart state consistent.
Tip: Make sure to implement error handling and ensure that the cart state remains consistent even if the user loses connection during a session.
Example Cart Data Structure
Product Name | Price | Quantity | Total |
---|---|---|---|
Wireless Headphones | $100 | 2 | $200 |
Smartphone Case | $25 | 1 | $25 |