Cross Platform Mobile App Development Tutorial
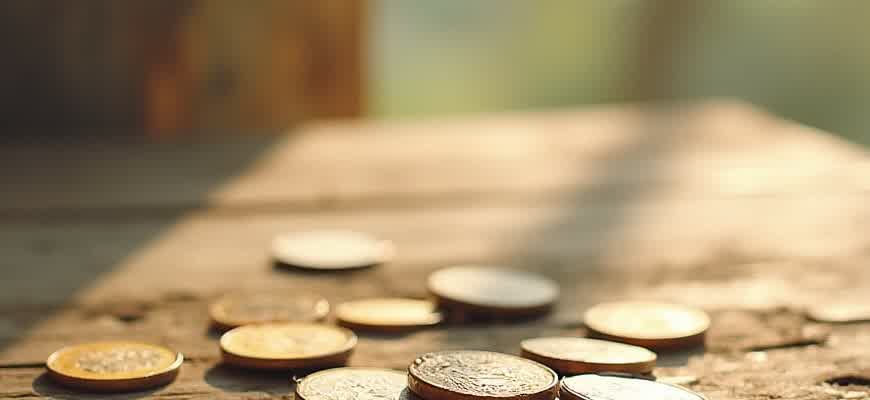
Creating mobile applications that work seamlessly across different platforms is essential for developers looking to reach a wider audience. With numerous tools available, developers can now build applications for both Android and iOS from a single codebase. In this guide, we'll explore the process of cross-platform development, including the advantages, challenges, and best practices.
Key Benefits of Cross-Platform Development
- Reduced development cost
- Faster time to market
- Consistency in user experience
- Easy maintenance and updates
"By developing once and deploying across platforms, developers can save time and resources, all while ensuring a consistent product experience."
Popular Frameworks for Cross-Platform Development
- React Native
- Flutter
- Xamarin
- Ionic
Comparison of Cross-Platform Frameworks
Framework | Language | Performance | Popularity |
---|---|---|---|
React Native | JavaScript | High | Very Popular |
Flutter | Dart | High | Increasing |
Xamarin | C# | Good | Moderate |
Ionic | HTML/CSS/JavaScript | Moderate | Decent |
Selecting the Ideal Framework for Cross-Platform Development
When working on a cross-platform mobile application, choosing the right framework is crucial to ensure the project’s success. A well-suited framework should allow seamless integration with both iOS and Android, providing consistency and minimizing development time. Several factors play a role in making this decision, including performance, community support, and the specific features needed for your app. Evaluating the pros and cons of each available framework will guide developers toward the best option for their project.
The development environment and the team’s familiarity with certain tools also influence the decision. With a range of frameworks offering various capabilities, it's important to prioritize compatibility with the app's intended functionality. Whether it’s using a hybrid framework for quick development or opting for a more robust one that delivers near-native performance, each choice carries distinct trade-offs.
Key Considerations for Framework Selection
- Performance: Some frameworks offer near-native performance, while others may suffer from slower execution due to abstraction layers.
- Development Speed: Frameworks like React Native or Flutter provide rapid development capabilities, which is beneficial for projects with tight deadlines.
- Community and Ecosystem: A strong community ensures plenty of resources, plugins, and troubleshooting support, which can reduce development challenges.
- Platform-Specific Features: Certain frameworks allow deep integration with platform-specific features, such as advanced UI elements or access to device hardware.
Popular Cross-Platform Frameworks
Framework | Strengths | Weaknesses |
---|---|---|
React Native |
|
|
Flutter |
|
|
Xamarin |
|
|
Important: The ideal framework depends on your team’s expertise, the specific needs of your app, and your target audience. Assess all available options before committing to ensure the framework aligns with your project’s long-term goals.
Setting Up Your Development Environment for Multiple Platforms
When building a cross-platform mobile application, the first step is configuring a development environment that supports all target platforms. This involves selecting the right tools, setting up dependencies, and ensuring compatibility across iOS, Android, and potentially other systems like Windows or macOS. A solid setup will streamline your workflow and minimize the risk of errors during development.
The key to an efficient environment is choosing a suitable IDE (Integrated Development Environment), configuring platform-specific SDKs (Software Development Kits), and setting up device emulators or simulators for testing. Below is a guide to get you started with the setup process for both iOS and Android platforms using popular cross-platform frameworks like React Native or Flutter.
Steps to Configure Your Environment
- Install the Necessary IDE: Choose an IDE that supports your framework. Popular options include:
- Visual Studio Code for React Native
- Android Studio for Flutter
- Install SDKs: Download and configure the Android SDK and iOS development tools.
- For Android: Install Android Studio and set up Android SDK.
- For iOS: Install Xcode for iOS SDK and other necessary tools.
- Configure Emulators/Simulators: Set up emulators for Android and simulators for iOS for testing your application across different devices.
- Android: Set up AVD (Android Virtual Device) in Android Studio.
- iOS: Use Xcode to set up iPhone or iPad simulators.
Key Tools for Cross-Platform Development
Tool | Description | Platform |
---|---|---|
Visual Studio Code | A lightweight code editor with powerful extensions for cross-platform development. | Windows, macOS, Linux |
Android Studio | Comprehensive IDE for Android development, includes emulators and debugging tools. | Windows, macOS, Linux |
Xcode | Essential for iOS development, including simulators and all necessary SDKs. | macOS |
Remember that every cross-platform framework might have specific setup instructions. Be sure to check the official documentation for your chosen framework to ensure compatibility and avoid common setup issues.
Building a Simple UI: Key Considerations for Cross-Platform Apps
When developing a cross-platform mobile application, creating a user interface (UI) that functions seamlessly across different devices and platforms is critical. Each platform, whether it's iOS or Android, comes with its own set of design guidelines, but it's essential to create a UI that feels native while still maintaining consistency across all devices. Understanding the key considerations can ensure that the app not only looks good but also performs well across multiple platforms.
For cross-platform app development, it's important to balance the use of native components with reusable code. This allows for efficient development while preserving the look and feel of each platform. Below are some key points to consider when designing a simple UI for cross-platform apps:
Key Considerations for Cross-Platform UI Design
- Responsive Layouts: Ensure the UI adapts to various screen sizes, orientations, and resolutions. This guarantees a consistent experience across all devices.
- Consistent Visuals: Use a consistent color scheme, typography, and iconography that aligns with both platforms' design guidelines.
- Platform-Specific Controls: Leverage platform-specific UI elements like buttons, sliders, and navigation bars to make the app feel native to each environment.
Remember, a good cross-platform UI should maintain consistency without sacrificing the user experience on each platform. Native-like performance and responsiveness are key to keeping users satisfied.
Design Guidelines Comparison
Aspect | iOS | Android |
---|---|---|
Navigation | Top navigation bar with back button | Bottom navigation bar with tabs |
Buttons | Flat, minimalistic with text labels | Raised buttons with text or icons |
Gestures | Swipe gestures for navigation | More varied gestures, including swiping and multi-finger actions |
By focusing on these points, developers can build a UI that is intuitive and consistent across platforms, improving the overall user experience.
Handling Device-Specific Features in Cross-Platform Applications
When developing cross-platform mobile apps, one of the biggest challenges is managing device-specific functionality. Different devices come with unique hardware, software, and platform capabilities, which can affect the behavior and performance of an application. Developers must find ways to access and optimize device features without sacrificing the advantages of cross-platform frameworks.
Effective handling of device-specific features requires understanding how to access platform-specific APIs and tools. Most cross-platform frameworks offer abstraction layers, but there are cases where developers need to write custom code to tap into native features. By using the right tools and conditional checks, it's possible to deliver a seamless experience on both iOS and Android without compromising performance or user experience.
Strategies for Accessing Device-Specific Features
- Platform-Specific Code: Many cross-platform frameworks, like Flutter and React Native, allow you to implement platform-specific logic. This lets you write platform-dependent code where necessary, while still using shared components for the rest of the app.
- Plugins and Libraries: Utilize third-party plugins and libraries that provide access to device hardware or features (such as camera, GPS, or sensors). These tools abstract the complexity of direct interaction with native code.
- Conditional Compilation: Implementing conditional compilation helps in separating platform-specific code from shared logic. This ensures that platform-specific behavior is only executed on the intended platform, maintaining performance across both.
Handling Platform-Specific UI Components
- Custom Views: For certain UI elements that behave differently on Android and iOS, creating custom views tailored to each platform ensures consistency.
- Native Navigation: In some cases, navigating between screens may require platform-specific adjustments, such as using iOS-style navigation on Apple devices and Material Design on Android.
- Responsive Layouts: Design the app’s layout to adapt to different screen sizes and resolutions using responsive design techniques, ensuring a consistent experience across devices.
Handling Native Features Using Plugins
Feature | iOS Plugin | Android Plugin |
---|---|---|
Camera | camera_plugin_ios | camera_plugin_android |
GPS | geolocation_ios | geolocation_android |
"Cross-platform frameworks can abstract many device features, but developers often need to rely on native plugins or platform-specific APIs for certain advanced capabilities."
Integrating Third-Party Libraries and SDKs in Your Cross-Platform App
When developing a cross-platform mobile application, integrating third-party libraries and software development kits (SDKs) is often necessary to extend your app's functionality. These integrations provide developers with ready-made solutions to implement complex features such as payments, analytics, or social media sharing, without having to build them from scratch. Using third-party resources can significantly speed up development time, but it is important to handle integration carefully to ensure stability and compatibility across different platforms.
While integrating external libraries, it’s essential to follow a structured approach to avoid issues like dependency conflicts or security vulnerabilities. Each platform (iOS and Android) may have its own specific requirements for integrating SDKs, which can require different approaches. Understanding these nuances and adhering to best practices will ensure a smoother experience when combining different resources in your app.
Steps for Integrating Libraries and SDKs
- Choose Compatible Libraries - Ensure the SDK or library is compatible with the frameworks and tools you are using, such as Flutter, React Native, or Xamarin.
- Install the Library - Use package managers like npm, CocoaPods, or Gradle to install the necessary dependencies.
- Configure Your Project - Modify configuration files and set up necessary permissions to enable the SDK's features.
- Handle Platform-Specific Code - For some libraries, you may need to add platform-specific code or settings (e.g., AndroidManifest.xml for Android, Info.plist for iOS).
- Test the Integration - Test the SDK’s functionality on both platforms to ensure consistency and to troubleshoot potential issues.
Important Considerations
Always check for updates to third-party libraries. Outdated versions can introduce bugs or security flaws that might not be compatible with newer versions of mobile operating systems.
Managing updates for integrated SDKs is crucial for maintaining app stability and performance. Regularly monitor the library’s repository for updates and document any changes to keep your app functioning optimally. Below is a table showing common SDKs and the platforms they support:
SDK/Library | Supported Platforms | Use Case |
---|---|---|
Firebase | Android, iOS, Web | Analytics, Notifications, Authentication |
Stripe | Android, iOS, Web | Payments Integration |
React Native Camera | Android, iOS | Camera Access |
Testing Your Cross-Platform Application on Multiple Devices
Ensuring that your cross-platform application performs consistently across various devices is crucial for delivering a seamless user experience. Testing on different devices helps identify platform-specific issues, such as differences in screen sizes, resolutions, and hardware capabilities. The goal is to make sure the app operates flawlessly whether it's running on Android, iOS, or other platforms.
To perform effective testing, you should focus on both functionality and performance aspects. This means verifying the app’s features, user interface, and speed on a range of devices with different operating system versions. A structured testing approach will help you detect and fix issues before the app is released to users.
Testing Strategy and Devices
Start by testing on a variety of devices that represent your target audience. You should include both physical devices and simulators/emulators for different platforms. Here are the key steps:
- Test on real devices: This helps identify real-world issues like performance problems and hardware-related bugs.
- Utilize emulators and simulators: These tools allow for testing on different screen sizes and configurations without needing physical devices for every scenario.
- Include multiple OS versions: Test across different versions of iOS and Android to ensure compatibility with older and newer versions.
Checklist for Cross-Platform App Testing
Use the following checklist to ensure all critical aspects are covered:
- UI Consistency: Ensure the user interface looks consistent on different screen sizes.
- Performance: Measure the app's speed, memory usage, and responsiveness on various devices.
- Device-Specific Features: Test features like GPS, camera, and sensors across devices.
- Network Conditions: Simulate various network conditions (3G, Wi-Fi, etc.) to test app behavior under different bandwidths.
Testing Tools
Several tools can help automate and simplify cross-platform app testing:
Tool | Description |
---|---|
Appium | Open-source tool for automating mobile apps on both Android and iOS. |
BrowserStack | Cloud-based tool to test mobile apps on real devices and various OS versions. |
Firebase Test Lab | Google’s cloud-based service for testing apps on real devices in various environments. |
Remember, effective testing isn't just about finding bugs; it’s about understanding how your app performs in diverse environments and ensuring the best user experience across all platforms.
Optimizing Mobile App Performance Across Multiple Platforms
When developing a mobile app for multiple platforms, ensuring optimal performance is essential for a smooth user experience. The challenge arises from the varying hardware capabilities, operating system constraints, and different user expectations across platforms. Effective performance optimization requires addressing these differences without sacrificing functionality or design.
To achieve the best performance on iOS, Android, and other platforms, developers need to employ strategies that streamline code execution, reduce memory usage, and minimize battery consumption. In this section, we will explore key techniques for optimizing cross-platform mobile app performance.
Key Strategies for Performance Optimization
- Efficient Code Execution: Utilize platform-specific APIs and libraries to access hardware acceleration, ensuring that the app runs smoothly on different devices.
- Memory Management: Minimize memory leaks by carefully managing memory allocation, especially in resource-intensive tasks such as media processing and real-time data fetching.
- Asynchronous Processing: Use asynchronous functions and background threads to avoid blocking the main UI thread, ensuring a responsive interface.
- Network Optimizations: Implement efficient network communication techniques like caching and data compression to reduce loading times and improve performance, especially on slower networks.
Platform-Specific Adjustments
Different platforms have distinct characteristics that can influence app performance. It is important to tailor the optimization strategies for each target operating system:
- iOS: Optimize memory usage by leveraging the native iOS memory management tools, and avoid using excessive third-party libraries that may cause bloat.
- Android: Take advantage of Android’s multi-threading capabilities, and optimize battery usage by managing background tasks effectively.
- Cross-Platform: For tools like Flutter or React Native, ensure the codebase is modular and reusable while focusing on keeping the UI fluid across both platforms.
Tip: Regular profiling on each platform is crucial to identify performance bottlenecks and ensure optimal user experience across devices.
Performance Comparison Table
Platform | Optimized Techniques | Challenges |
---|---|---|
iOS | Efficient memory management, use of native APIs, code optimizations | Hardware fragmentation |
Android | Multi-threading, battery usage optimization, reducing background tasks | Device diversity, inconsistent OS versions |
Cross-Platform | Reusable codebase, adaptive UI, platform-specific adjustments | Performance trade-offs between platforms |
Publishing Your Cross-Platform App on iOS and Android
After developing your mobile app using a cross-platform framework, the next crucial step is publishing it on both iOS and Android platforms. Each platform has its own set of requirements and processes, but understanding these steps can ensure your app reaches a wider audience with minimal complications. Both the Apple App Store and Google Play Store have specific guidelines, so following them carefully will help your app get approved without unnecessary delays.
In this guide, we'll walk through the essential steps involved in submitting your app for both platforms, from preparing the app for release to the final submission process. By following these instructions, you can simplify the deployment of your app to both stores.
Steps for Publishing on iOS
- Join the Apple Developer Program: To publish your app on iOS, you need to be part of the Apple Developer Program, which requires an annual membership fee.
- Prepare your app for submission: Ensure that your app meets Apple's guidelines, including proper app icon sizes, descriptions, and privacy policies.
- Test on multiple devices: Thoroughly test the app on various iPhone and iPad models using Xcode’s simulator or real devices.
- Submit via App Store Connect: Upload your app to App Store Connect, where you can set app details, pricing, and distribution options.
Steps for Publishing on Android
- Join Google Play Console: To submit your app on Google Play, you must sign up for a Google Play Console account, which requires a one-time registration fee.
- Prepare your app for release: Make sure your app follows Google's guidelines, such as providing correct metadata and required assets (screenshots, icons, etc.).
- Build the APK or AAB file: Compile your app as an APK or AAB file for submission. You can do this directly from your development environment like Android Studio.
- Submit on Google Play Console: Upload your APK or AAB file to Google Play Console and fill out the necessary information about your app before submitting for review.
Key Differences Between iOS and Android Publishing
Platform | Submission Process | Fee | Review Time |
---|---|---|---|
iOS | App Store Connect submission with Xcode upload | Annual membership fee ($99/year) | 1-3 days |
Android | Google Play Console submission with APK/AAB upload | One-time registration fee ($25) | 1-7 days |
Important: Make sure your app meets both platform's content and functionality guidelines to avoid rejection during the review process.