Ios App Development Using Kotlin
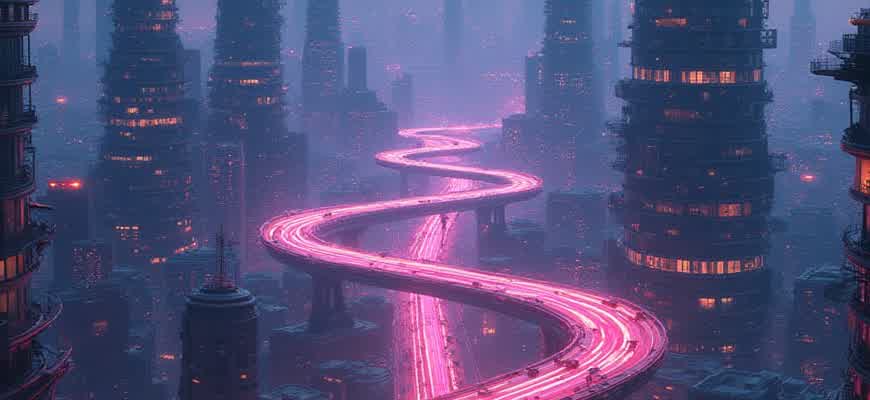
Kotlin is a modern programming language originally designed for Android development, but with the rise of cross-platform solutions, it can now also be used for creating iOS applications. Although iOS development is traditionally associated with Swift and Objective-C, Kotlin offers a flexible, concise, and interoperable alternative that can streamline the development process for apps targeting both Android and iOS platforms.
By leveraging Kotlin Multiplatform (KMP), developers can share a significant portion of code across both platforms, reducing redundancy and development time. Below is a comparison of the major benefits of using Kotlin for iOS development:
- Code Sharing: A large amount of the business logic can be shared between Android and iOS projects.
- Concise Syntax: Kotlin's syntax is simpler and less error-prone than older languages, which aids in faster development cycles.
- Strong Tooling Support: With integration into popular IDEs like IntelliJ IDEA and Android Studio, Kotlin provides solid development environments for iOS apps.
For developers interested in utilizing Kotlin for iOS, there are a few key steps to follow:
- Set up Kotlin Multiplatform Mobile (KMM) in your development environment.
- Create shared modules to handle business logic, networking, and database interactions.
- Write platform-specific code for UI and device features, as Kotlin does not provide direct access to iOS native frameworks.
Important: Kotlin Multiplatform for iOS is still evolving, and while it holds a lot of promise for cross-platform development, it may not fully replace native iOS development in certain cases requiring deep platform-specific functionality.
The following table outlines some common tools and libraries useful for Kotlin iOS development:
Tool/Library | Description |
---|---|
Kotlin Multiplatform Mobile (KMM) | Enables sharing business logic across Android and iOS applications. |
Ktor | A framework for making HTTP requests that works on both Android and iOS. |
SQLDelight | A database library that generates type-safe Kotlin code for interacting with databases on both platforms. |
Why Choose Kotlin for iOS Development Over Swift?
In recent years, Kotlin has emerged as a powerful tool for developing cross-platform applications, and its potential for iOS development is growing rapidly. While Swift has been the go-to language for iOS apps, Kotlin offers a unique set of advantages that make it an appealing choice for developers looking to streamline their workflows and maintain consistency across platforms.
Unlike Swift, which is iOS-specific, Kotlin allows developers to write shared code that can be used for both Android and iOS applications. This approach helps reduce development time and effort, making it easier to maintain a single codebase for multiple platforms.
Key Benefits of Kotlin for iOS Development
- Cross-Platform Development: Kotlin is part of the Kotlin Multiplatform project, enabling the use of the same codebase for both Android and iOS development. This drastically reduces the time needed to develop separate applications for different platforms.
- Interoperability with Swift: Kotlin can seamlessly interact with Swift and Objective-C, allowing developers to integrate Kotlin code into existing iOS projects without significant rewrites.
- Improved Developer Productivity: Kotlin's concise syntax and modern features help developers write cleaner, more maintainable code, leading to faster development cycles.
Kotlin's ability to share business logic and core functionality between platforms makes it an attractive option for businesses looking to save on development and maintenance costs.
Comparing Kotlin and Swift: A Quick Overview
Aspect | Kotlin | Swift |
---|---|---|
Cross-Platform Support | Yes (via Kotlin Multiplatform) | No (iOS-only) |
Integration with Existing Projects | High, can work with Java and Swift | Native iOS integration only |
Learning Curve | Lower for developers familiar with Java | Steeper for those unfamiliar with Apple's ecosystem |
While Swift is a powerful language designed specifically for Apple's ecosystem, Kotlin's flexibility, cross-platform capabilities, and ability to integrate with existing iOS codebases make it an appealing alternative for developers aiming for efficiency and code reusability across platforms.
Setting Up a Kotlin-based iOS Development Environment
Developing iOS applications using Kotlin requires a specific setup that involves both native iOS tools and Kotlin-specific frameworks. To enable Kotlin support for iOS, developers use Kotlin Multiplatform Mobile (KMM), which allows the use of Kotlin code across both iOS and Android. Setting up this environment requires configuring multiple tools and dependencies to ensure smooth development.
To get started, you will need a macOS machine, as iOS development relies on Xcode, which is exclusive to macOS. Additionally, you must install the necessary Kotlin tooling, such as Kotlin Multiplatform and the required plugins for integration with Xcode. Below is a step-by-step guide to setting up your environment.
Steps to Set Up Kotlin for iOS Development
- Install Xcode: Download the latest version of Xcode from the Mac App Store. It provides all the necessary tools to compile iOS applications.
- Install Homebrew: Homebrew is a package manager for macOS that simplifies the installation of development tools. Run the following command in your terminal to install it:
- Install Kotlin Multiplatform: Kotlin Multiplatform Mobile (KMM) is essential for sharing code between iOS and Android. Use the following command to install it:
- Set Up Android Studio: Although you’re developing for iOS, Android Studio is essential for creating and managing the Kotlin project. You can download it from the official Android website.
- Install KMM Plugin for Android Studio: This plugin helps manage Kotlin-based iOS development. Install it directly through Android Studio by navigating to Preferences > Plugins and searching for "Kotlin Multiplatform Mobile".
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
brew install kotlin
Configuring Xcode for Kotlin Development
After setting up Kotlin Multiplatform and Android Studio, configure Xcode to work with the Kotlin codebase. You'll need to link your iOS target with the Kotlin code and ensure that the build process runs smoothly. Below is an essential checklist for configuring Xcode for Kotlin:
- Make sure that you have a valid Apple Developer account for building and testing iOS apps.
- Verify that Xcode is properly linked with your Kotlin Multiplatform project. This will be done via a shared framework that connects Kotlin with iOS code.
- Set up the necessary build configurations, such as specifying the iOS deployment target and choosing the correct architecture.
Key Tools and Requirements
Tool | Description |
---|---|
Xcode | Apple's IDE for iOS and macOS development. Required to compile and run iOS applications. |
Kotlin Multiplatform | A framework allowing Kotlin to be used across both Android and iOS platforms. |
Android Studio | Primary IDE for Kotlin development. Required for managing Kotlin Multiplatform projects. |
Homebrew | Package manager for macOS used to install development tools like Kotlin. |
Important: Ensure that all tools are updated to the latest versions to avoid compatibility issues during development.
Integrating Kotlin Multiplatform for Cross-Platform Mobile Development
Kotlin Multiplatform (KMP) is a powerful tool that allows developers to share code between Android and iOS applications. By using KMP, developers can write business logic once and reuse it across multiple platforms, significantly reducing development time and improving maintainability. This approach offers a unified experience for both iOS and Android without needing to completely rewrite or duplicate code for each platform.
To implement Kotlin Multiplatform in a mobile app, developers need to follow several key steps to ensure both compatibility and performance. The platform supports shared code for core business logic, data models, and networking, while also allowing platform-specific implementations when necessary. Below is an overview of how to effectively integrate KMP in cross-platform mobile apps.
Steps for Integrating Kotlin Multiplatform
- Set up the Kotlin Multiplatform Project: Create a new KMP project or convert an existing Android project to support iOS. This involves configuring the shared code module and defining platform-specific targets (Android, iOS).
- Share Business Logic: Write the common business logic that can be shared across both platforms. This includes tasks like handling network requests, managing data models, and business rules.
- Implement Platform-Specific Code: For features that are unique to each platform (e.g., UI components, device capabilities), use expect/actual declarations to define platform-specific implementations.
- Test and Debug: Ensure the shared code works on both Android and iOS. This may involve running tests on each platform and debugging platform-specific issues.
Key Advantages of Kotlin Multiplatform
- Reduced Development Time: Share significant portions of the codebase, leading to faster feature releases.
- Improved Code Consistency: Business logic remains consistent across platforms, reducing bugs related to discrepancies between Android and iOS versions.
- Platform Flexibility: Developers can still utilize platform-specific features and APIs while sharing core functionality.
Important: While Kotlin Multiplatform is powerful, it's not a one-size-fits-all solution. Some app features, especially complex UIs, may require more platform-specific customization.
Tools and Resources for Kotlin Multiplatform
Tool | Purpose |
---|---|
Kotlin Plugin for IntelliJ IDEA | Helps in writing and managing Kotlin code for both Android and iOS targets. |
Apple's Xcode | Required for building iOS apps. Used in combination with the Kotlin/Native compiler for iOS targets. |
Gradle | Manages dependencies, builds, and the overall project structure for KMP. |
Challenges in Adopting Kotlin for iOS App Development
While Kotlin has become a popular language for Android app development, its application for iOS platforms is still relatively new and comes with several challenges. Despite the advantages of Kotlin's modern features, developers face significant obstacles when using it for building iOS applications. These challenges are mainly related to compatibility issues, limited ecosystem support, and performance concerns, making the development process more complex compared to using Swift or Objective-C, which are natively supported by iOS.
One of the key reasons for these challenges is the cross-platform nature of Kotlin Multiplatform (KMP). While KMP allows developers to write shared code for both iOS and Android, it is not always seamless. Developers often need to use additional frameworks or tools, which introduces complexity. Understanding these hurdles is crucial for anyone looking to adopt Kotlin for iOS development.
Common Issues in Kotlin-based iOS Development
- Limited Native Integration: Although Kotlin Multiplatform allows code sharing, it does not have full native integration with iOS features like Swift does.
- Tooling Support: Development tools and IDEs like Xcode are not fully optimized for Kotlin, which may lead to an inefficient development environment.
- Performance Overhead: The use of Kotlin for iOS may lead to slower performance compared to using native Swift, especially in resource-intensive applications.
Technical Limitations and Workarounds
Integration with iOS Frameworks: Kotlin lacks direct support for iOS's native APIs, which can lead to compatibility issues. Developers must rely on wrappers or additional libraries, which may not always be up to date or as efficient as native Swift libraries.
Cross-Platform Tools: Using Kotlin requires the integration of additional tools like CocoaPods or Gradle for managing dependencies, adding an extra layer of complexity. These tools often need manual configurations to work smoothly with iOS-specific dependencies.
“Despite its potential, Kotlin on iOS is still in an experimental phase, and developers need to weigh the pros and cons before making it their primary development language for iOS apps.”
Summary of Key Issues
Challenge | Description |
---|---|
Native Integration | Limited support for iOS-specific APIs and features, resulting in additional coding effort for developers. |
Tooling Support | IDE and debugging tools like Xcode are not optimized for Kotlin, which can lead to slower development cycles. |
Performance | Kotlin-based applications may suffer from performance issues when compared to native Swift apps, especially for complex use cases. |
Testing and Debugging iOS Applications Developed with Kotlin
When developing iOS applications using Kotlin, ensuring the quality of the code through rigorous testing and debugging is crucial. Testing plays an essential role in detecting bugs early in the development process, preventing issues that may affect user experience or performance. In Kotlin, developers can leverage both native tools and frameworks designed specifically for iOS to conduct efficient testing. Debugging tools, on the other hand, help identify and fix issues within the code, ensuring that the app performs as expected across different devices and iOS versions.
Effective debugging and testing in Kotlin for iOS development involve a combination of automated tests, manual checks, and efficient use of debugging tools. The integration of tools like Xcode and IntelliJ IDEA makes it easier to simulate real-world scenarios, track down errors, and verify that code changes do not introduce new problems. Below are the key practices and tools used for this process.
Key Practices for Testing and Debugging
- Unit Testing: Writing unit tests ensures that individual functions or components behave as expected. This can be done using frameworks such as JUnit or Spek integrated with Kotlin.
- UI Testing: Automated UI tests are essential for validating the user interface. Tools like XCUITest allow testing UI elements and user interactions.
- Integration Testing: This ensures that different components of the app work together as intended. It can be done using tools like MockK to simulate dependencies.
Debugging Techniques
- Using Breakpoints: Set breakpoints in the code to pause execution and inspect variables, call stacks, and other details in real-time.
- Console Logs: Utilizing println or Log.d statements helps track the app's behavior and outputs during execution.
- Memory and Performance Profiling: Tools like the Xcode Instruments suite help identify memory leaks, performance bottlenecks, and other optimization opportunities.
Tip: Always test on multiple devices and iOS versions to ensure compatibility and performance consistency across different environments.
Useful Debugging Tools
Tool | Purpose |
---|---|
Xcode Debugger | Helps with step-by-step execution and inspection of code to identify and fix bugs. |
Instruments (Xcode) | Used for profiling performance, checking memory usage, and identifying performance issues. |
Firebase Crashlytics | Real-time crash reporting tool that helps track app crashes and provides detailed logs for debugging. |
Utilizing Kotlin Coroutines for Efficient Asynchronous Handling in iOS Applications
Asynchronous tasks are a cornerstone of modern mobile applications, and Kotlin Coroutines have become a popular choice for managing these tasks in iOS development. By utilizing Kotlin's built-in support for coroutines, developers can simplify asynchronous programming and enhance the overall responsiveness of their applications. The combination of Kotlin and coroutines provides an efficient and effective way to manage background operations such as network requests, database queries, and UI updates without blocking the main thread.
With the increasing demand for smoother and more responsive user experiences, managing concurrency and asynchronous tasks is critical. Kotlin Coroutines provide a structured approach to handle asynchronous code in a more readable and maintainable manner. This approach is especially beneficial for iOS development, where responsiveness is essential for ensuring a seamless user interface and overall application performance.
Key Benefits of Kotlin Coroutines in iOS Development
- Structured Concurrency: Coroutines allow for better control over background tasks, making code easier to manage and debug.
- Reduced Boilerplate: Unlike traditional callback-based approaches, coroutines simplify the code and reduce the need for nested callbacks.
- Non-Blocking IO Operations: By executing tasks asynchronously, Kotlin Coroutines free up the main thread, allowing the app to remain responsive while processing time-consuming tasks.
How to Use Kotlin Coroutines for Asynchronous Tasks
Implementing coroutines for handling asynchronous tasks involves a few simple steps:
- Setup Coroutine Scope: Create a CoroutineScope tied to your app's lifecycle, such as the activity or view model, to ensure proper task management.
- Launch Coroutines: Use the
launch
function to initiate background operations that run concurrently with the UI thread. - Use suspend functions: Define functions that can be suspended, allowing the coroutine to pause and resume based on task completion.
- Handle Results: Use
withContext
to switch to the appropriate dispatcher for UI updates once background tasks are completed.
Example: Making an Asynchronous Network Call
Code | Description |
---|---|
CoroutineScope(Dispatchers.Main).launch { |
Launch a coroutine on the main thread. |
val result = withContext(Dispatchers.IO) { |
Switch to IO thread for network call. |
val data = fetchDataFromNetwork() |
Perform network request in background thread. |
updateUI(data) |
Update UI on the main thread with the result. |
} |
End coroutine block. |
By using Kotlin Coroutines in your iOS app, you ensure that background tasks are handled efficiently while maintaining a smooth user experience.
Building UI Components in Kotlin for iOS: Best Practices
When developing user interfaces for iOS applications using Kotlin, it's essential to adhere to specific guidelines to ensure a smooth, maintainable, and performant experience. Kotlin Multiplatform enables the creation of iOS apps with shared code, and building UI components is an integral part of this process. This requires leveraging Kotlin's interoperability with iOS-specific frameworks, while maintaining flexibility and efficiency. It’s crucial to follow best practices that align with both iOS design principles and Kotlin capabilities for optimal results.
To successfully build UI components for iOS in Kotlin, developers should focus on efficient code organization, adherence to UI patterns, and the effective use of available libraries and frameworks. Understanding the platform-specific nuances and using the right Kotlin tools can significantly enhance the user experience, ensure better performance, and reduce development time. Below are some recommended practices for building UI components with Kotlin for iOS applications.
Key Best Practices for UI Component Development
- Use Kotlin Multiplatform Mobile (KMM) for code sharing. This allows you to write shared UI logic that works seamlessly across both Android and iOS, minimizing duplication.
- Leverage SwiftUI for iOS UI Design when targeting iOS, as it offers native features and a declarative syntax that simplifies UI construction and enhances performance.
- Ensure Consistency with Platform Guidelines to maintain a native look and feel. Utilize UIKit for more complex iOS UI elements that are not easily accessible via KMM.
Structure of UI Components
- Modularize UI Components: Break down components into smaller, reusable parts. This promotes maintainability and scalability.
- Use SwiftUI Views in Kotlin: By using Kotlin's interoperability, developers can create SwiftUI views within the Kotlin codebase, allowing for better UI control.
- Properly Handle State Management: Always manage state using appropriate frameworks to ensure smooth user interactions and UI responsiveness.
Remember that Kotlin's seamless integration with iOS frameworks is essential for creating efficient and responsive UI components. Leveraging both platforms' strengths ensures a unified and high-performing user interface.
Performance Considerations
Practice | Description |
---|---|
Memory Management | Ensure proper memory handling when creating UI components to avoid leaks and performance degradation. |
Asynchronous UI Updates | Use async functions to prevent UI freezing, ensuring smoother interactions with the app. |
Optimize Rendering | Optimize view rendering processes by avoiding unnecessary redraws and reflows of UI elements. |