Build Social Media App React
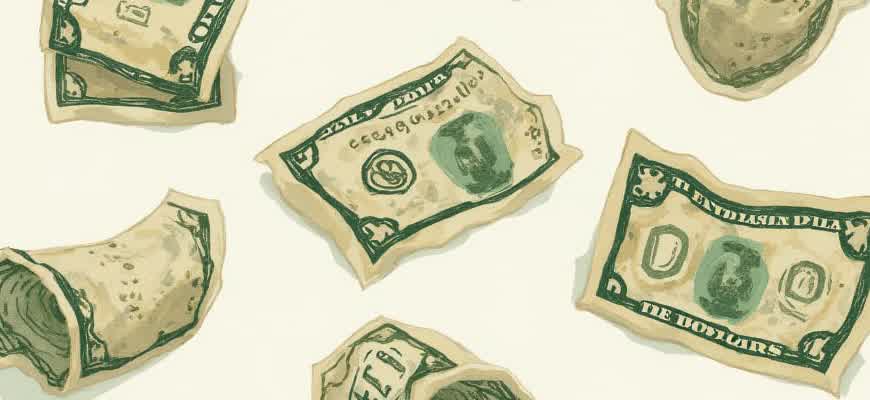
Creating a social media platform using React involves several key steps, from setting up the environment to implementing core features like user authentication, content management, and interactive elements. React's component-based architecture makes it easy to build scalable and dynamic user interfaces, essential for handling large amounts of real-time data in social apps.
First, you'll need to structure your application to manage the state and data flow effectively. Below is a general approach for building a basic social media platform:
- Set up the project using tools like Create React App or Next.js for server-side rendering.
- Implement user authentication and authorization with Firebase or JWT tokens.
- Build reusable components for posts, comments, and user profiles.
- Handle data storage and retrieval with a backend solution like Firebase or Node.js with Express.
- Optimize performance using React’s hooks and Context API for global state management.
Additionally, one of the crucial parts is managing user-generated content effectively. Here’s an outline of how you can design the database for posts and comments:
Entity | Fields | Description |
---|---|---|
Post | ID, User, Content, Timestamp | Each post is associated with a user and has content along with a timestamp for creation. |
Comment | ID, Post ID, User, Content, Timestamp | Comments are tied to a specific post and include the content of the comment and timestamp. |
Note: You should ensure that your application scales well by implementing proper data pagination and efficient API calls for handling large amounts of data.
Creating a Social Media Application Using React: A Hands-On Approach
Building a social media platform with React requires a solid understanding of both the framework itself and the architecture of social networking apps. The main challenge lies in handling dynamic content, user interactions, and real-time updates. With React’s component-based structure, this task becomes manageable and modular, allowing for efficient management of states and UI rendering.
In this practical guide, we will go through the steps to create a simple social media app. We will focus on the core features that most platforms share: user profiles, posts, and interactions. This guide assumes you have basic knowledge of React, but we will break down the steps clearly to ensure anyone can follow along.
Key Features to Implement
- User authentication and login system
- Post creation and display
- Commenting and liking posts
- User profiles with editable information
- Real-time updates (optional)
Step-by-Step Guide
- Set up the React environment: Install Node.js, create a new React app using Create React App, and set up the necessary dependencies such as React Router for navigation and Axios for making HTTP requests.
- Build components: Start by creating reusable components like Header, Post, Profile, and Comment. Each component should be modular and focus on a specific part of the UI.
- Manage state: Use React’s useState and useEffect hooks to manage state across your app, ensuring that user data, posts, and comments are updated efficiently.
- Integrate with a backend: Set up a backend API (Node.js, Express) to handle requests for fetching and posting data. You can use Firebase or a custom backend solution.
- Testing: Ensure your app works smoothly by testing user interactions, component rendering, and API calls.
Tip: Keep your app scalable. As the app grows, consider breaking down components further and using state management libraries like Redux for better data flow.
Table of Common React Concepts
Concept | Description |
---|---|
State | Stores data for a component, triggering a re-render when updated. |
Props | Allow data to be passed from a parent component to a child component. |
Hooks | Functions like useState and useEffect that allow you to use state and side effects in functional components. |
Choosing the Optimal Architecture for Your Social Media App
When developing a social media platform using React, selecting the right architecture is crucial for scalability, performance, and maintainability. A well-structured architecture helps ensure that your app can handle large amounts of data, manage user interactions effectively, and grow seamlessly over time. This decision should consider both front-end and back-end frameworks, data flow, and how various components will communicate with each other.
The architecture must also align with your app's long-term vision, from basic features to advanced functionalities. With React’s component-based design, the front-end architecture often revolves around a state management system and reusable components, while the back-end requires efficient database management and APIs for handling data requests.
Key Considerations for Architecture
- Scalability: Plan for future growth in terms of both user base and features. Ensure that the app can handle increasing traffic and data without major rewrites.
- Performance: Use caching strategies, lazy loading, and code splitting to improve loading times and responsiveness.
- Maintainability: Organize code in a modular way so that updates and bug fixes can be made efficiently.
"The architecture you choose should be adaptable to the evolution of your app’s features, whether it’s for handling millions of users or integrating with new technologies."
Front-end and Back-end Considerations
- Component-Based Architecture: In React, build reusable components that manage their own state and can be composed into larger UI structures. This makes the app easy to scale and maintain.
- State Management: Use tools like Redux or Context API for managing app-wide state and facilitating communication between components.
- API Design: Implement RESTful or GraphQL APIs for fetching data from the server, ensuring smooth communication between front-end and back-end.
Database & Backend Architecture
Database | Considerations |
---|---|
NoSQL (e.g., MongoDB) | Flexible schema, ideal for handling large, dynamic datasets (e.g., user profiles, posts, and comments). |
SQL (e.g., PostgreSQL) | Structured data with strong relationships, ideal for complex queries and transactions. |
By making thoughtful decisions about architecture early in the development process, you set a strong foundation for your social media app to grow, remain responsive, and provide an optimal user experience.
Setting Up React Environment for Your Social Media Project
Before diving into building your social media app, it's crucial to set up a proper React development environment. This ensures smooth workflows and avoids unnecessary issues during development. The first step is installing Node.js and npm, which are essential for managing packages and dependencies. Once these are in place, you can initialize your React project with Create React App or configure Webpack and Babel manually for more control.
After the initial setup, configuring your project structure and dependencies is important. This involves organizing your files into components, services, and assets, making your codebase scalable. You will also need to install React Router for navigation, and libraries like Redux or Context API for state management, depending on your app's complexity.
Key Steps to Setup the React Development Environment
- Install Node.js and npm.
- Use Create React App to scaffold the project.
- Install React and ReactDOM dependencies.
- Set up your development server (Webpack or Create React App).
- Install additional libraries (React Router, Redux, Axios, etc.).
Note: Always ensure that your Node.js version is compatible with the latest version of React to avoid conflicts.
Dependencies and Tools You Might Need
Package | Description |
---|---|
react | Main React library for building UI components. |
react-dom | Enables DOM rendering for React components. |
react-router-dom | For handling navigation and routing in your app. |
redux | State management tool, useful for complex app states. |
axios | Library for making HTTP requests to external APIs. |
Designing User Authentication and Authorization Systems
When developing a social media platform, one of the most critical aspects is ensuring secure and efficient user authentication and authorization. These systems control who can access specific resources within the app and prevent unauthorized actions. Authentication is the process of verifying the user's identity, while authorization determines what actions or resources a user can access after they are authenticated.
To implement a robust authentication and authorization system, it’s important to understand the differences between authentication methods and access control mechanisms. This ensures that users are protected, and sensitive data is only accessible by those who have the proper permissions.
Authentication Techniques
Various authentication methods can be implemented depending on the security requirements of the application. The most common techniques are:
- Email and Password: Users enter their credentials to sign in. This method is simple but should be supplemented with security measures like hashing passwords.
- OAuth: Users authenticate through third-party providers (Google, Facebook, etc.), providing a quicker registration/login experience.
- Multi-Factor Authentication (MFA): A combination of methods like a password and an SMS code to provide an additional layer of security.
- Biometric Authentication: Uses facial recognition or fingerprint scanning for authentication, offering a higher level of convenience and security.
Authorization Strategies
Once authentication is complete, authorization determines what each user is allowed to do in the application. This can be managed through the following approaches:
- Role-Based Access Control (RBAC): Users are assigned roles (e.g., admin, user, guest), each having different levels of access and permissions.
- Attribute-Based Access Control (ABAC): Permissions are granted based on attributes like user location, device type, or time of access.
- Access Control Lists (ACLs): Each resource has a list of permissions that specify which users or groups can access the resource.
Important Considerations
Security is not just about protecting users’ passwords; it's about implementing layers of defense at every level of access.
When designing your authentication and authorization systems, ensure you also consider:
Factor | Considerations |
---|---|
Session Management | Ensure secure handling of user sessions, preventing session hijacking or fixation. |
Data Encryption | Encrypt sensitive data both in transit and at rest to safeguard against unauthorized access. |
Rate Limiting | Prevent brute-force attacks by limiting the number of authentication attempts within a set time frame. |
Implementing Real-Time Communication in React with WebSockets
In modern social media applications, providing a seamless, interactive experience often requires real-time communication features. Integrating WebSockets into a React app can enhance user engagement by enabling features like instant messaging, live notifications, or real-time updates. WebSockets allow for a persistent, open connection between the client and server, facilitating data exchange without the need to repeatedly make requests. This makes it ideal for building responsive and interactive social media platforms.
React, known for its component-based architecture, can easily accommodate WebSockets for real-time interactions. By establishing a WebSocket connection and managing its lifecycle within React components, developers can ensure that data flows continuously and efficiently. Below are steps and best practices to consider when integrating WebSockets with a React application.
Setting Up WebSockets in a React Application
- Install WebSocket client library, such as
socket.io-client
or nativeWebSocket
. - Initialize a WebSocket connection inside a React component's
useEffect
hook to ensure it's set up when the component mounts. - Use state management (via
useState
) to track data received from the WebSocket server. - Clean up the WebSocket connection in the
useEffect
cleanup function to prevent memory leaks.
Handling Real-Time Data in React
Real-time data flow is crucial for user engagement. Using React'suseEffect
anduseState
hooks allows developers to manage WebSocket connections and handle dynamic updates efficiently.
- Establish a connection to the WebSocket server and subscribe to events.
- On receiving new data, update the component's state to trigger a re-render and display the latest information.
- Handle edge cases, such as lost connections or reconnection attempts, to ensure robustness.
Example WebSocket Connection in React
Code Segment | Description |
---|---|
const socket = new WebSocket('ws://example.com/socket'); |
Creates a new WebSocket connection to the server. |
socket.onmessage = (event) => { setMessages(prev => [...prev, event.data]); }; |
Updates the state with the new message when received. |
useEffect(() => { return () => socket.close(); }, []); |
Closes the WebSocket connection when the component unmounts. |
Building Scalable Database Solutions for Social Media Data
Designing a database for a social media platform requires more than just storing user data. It’s essential to create a structure that can handle vast amounts of dynamic and evolving data. User interactions, posts, comments, and media files must be easily accessible and adaptable to scale as the platform grows. A well-designed database will allow seamless data retrieval, high availability, and efficient updates, even as millions of users engage simultaneously.
When scaling a database for social media, it’s critical to consider both the read and write operations that will occur frequently. Optimizing for performance while ensuring data consistency is key to preventing bottlenecks and downtime. Choosing the right database architecture, whether relational or NoSQL, can drastically affect the performance and scalability of the app.
Key Considerations for Scalability
- Data Partitioning: Dividing data into smaller chunks (sharding) enables faster queries and better load distribution across multiple servers.
- Read-Write Optimization: Using techniques like caching and load balancing helps manage heavy read or write operations without compromising speed.
- Replication: Creating multiple copies of data across different regions ensures high availability and disaster recovery.
Choosing the Right Database System
- Relational Databases: Good for structured data and complex queries, such as user profiles, relationships, and interactions. Example: PostgreSQL, MySQL.
- NoSQL Databases: Ideal for flexible, unstructured data like posts, comments, and multimedia. Examples: MongoDB, Cassandra.
- Graph Databases: Suitable for representing relationships between users and content. Example: Neo4j.
Performance Strategies
Strategy | Description |
---|---|
Data Caching | Temporarily storing frequent queries in memory to reduce database load. |
Indexing | Improves query performance by creating indexes on frequently searched fields. |
Horizontal Scaling | Adding more servers to distribute the load, enhancing performance during high traffic periods. |
Important: The choice of database system must align with the specific needs of your social media platform. Ensure the system you choose can support both high concurrency and large volumes of data without significant latency.
Building an Efficient Feed System with React
To develop an effective feed system in a social media application using React, it's essential to manage the flow of dynamic data and optimize rendering for performance. The feed often displays posts from various users, media content, and interactive elements like comments and reactions. React's component-based structure is particularly useful for this, as it allows you to break down the feed into smaller, reusable components.
The core of a successful feed system lies in the management of state and props. The feed must fetch data asynchronously and update based on user interactions or new content. React’s hooks, such as useState and useEffect, allow seamless handling of dynamic data and provide a simple way to control component updates. Additionally, using state management libraries like Redux can help in handling complex state logic across the feed's components.
Optimizing Rendering with Efficient Techniques
To prevent unnecessary re-renders and ensure the app performs efficiently, developers should implement strategies like lazy loading and virtualization. These techniques allow only the visible portion of the feed to be rendered, saving resources and improving the overall performance of the app.
- Lazy loading: Delays loading of media content and additional posts until the user scrolls near them.
- Virtualization: Renders only a small subset of the feed, dynamically adjusting as the user scrolls.
By combining React's rendering optimizations with effective data handling, developers can create a smooth, user-friendly feed that scales well with increasing content.
Important: Always monitor the feed's performance using tools like React DevTools to detect performance bottlenecks and optimize the app further.
Handling Dynamic Content and User Interaction
The feed must also handle various types of dynamic content, such as posts with images, text, videos, and interactive elements like like buttons or comment sections. Each of these elements can be represented by different components in React. Ensuring these components are responsive and can efficiently handle updates is key to providing a seamless user experience.
- Use state management to update the content of the feed when new posts are added.
- Incorporate interactive elements that allow users to engage with posts in real-time.
- Apply caching techniques to reduce the need for repeated API calls.
Component | Purpose | Optimization Method |
---|---|---|
Feed Container | Holds the entire list of posts | Use memoization to avoid unnecessary re-renders |
Post | Represents individual posts | Lazy load images/videos |
Comments | Displays comments for each post | Load comments in batches |
Optimizing Media Uploads and Streaming in React-Based Applications
Handling media files efficiently is crucial for React applications that support user-generated content, such as social media platforms. Optimizing file uploads and streaming ensures a smooth user experience and minimizes performance bottlenecks. For React apps, this involves dealing with various media types, handling large files, and managing network resources effectively.
One of the primary challenges is ensuring that media files, whether images, videos, or audio, are uploaded quickly and reliably. Proper optimization techniques can reduce server load, improve upload speed, and enhance the overall performance of the app.
Strategies for Efficient Media Uploads
- Chunked Uploads: Splitting files into smaller chunks before uploading helps prevent timeouts and allows for resumable uploads in case of connection issues.
- File Compression: Compressing images and videos before upload reduces the amount of data being transferred, which speeds up the process and saves bandwidth.
- Progressive Uploads: Implementing progress bars or indicators gives users real-time feedback on upload status and improves the user experience.
Best Practices for Streaming Media
- Adaptive Bitrate Streaming: This technique adjusts the quality of the video stream based on the user’s internet speed, ensuring smooth playback even on slow networks.
- Lazy Loading: Load media content only when it's about to be viewed. This reduces initial page load time and saves bandwidth.
- Content Delivery Networks (CDNs): CDNs cache media content on multiple servers worldwide, reducing latency and improving streaming performance.
Table of Common Media Formats and Their Optimizations
Media Type | Recommended Optimization |
---|---|
Images | Compression (JPEG, WebP), Responsive Images (srcset) |
Videos | Adaptive Streaming (HLS, DASH), Compression (H.264) |
Audio | Compression (MP3, OGG), Streaming (HLS, AAC) |
"By leveraging proper file handling strategies, React apps can deliver seamless media experiences for users without compromising on performance."
Deploying Your Social Media Application: Key Tools and Best Practices
Once you've built a social media app with React, deploying it efficiently is critical for ensuring performance, scalability, and a smooth user experience. The deployment phase involves choosing the right platform, optimizing your app, and monitoring its performance post-launch. Below are some best practices and essential tools that can make the deployment process easier and more effective.
Choosing the right tools and platforms can greatly influence the success of your app in a production environment. Below are the recommended steps for deployment, as well as tools that help in each stage.
Key Tools for Deployment
- Vercel - An excellent platform for React apps, offering seamless integration with GitHub and automated deployments.
- Netlify - A great choice for React-based static apps, with built-in Continuous Deployment and scalability options.
- Heroku - Ideal for backend-heavy applications that require server-side logic along with frontend deployment.
- AWS Amplify - A comprehensive tool for full-stack apps with serverless architecture, perfect for scaling social media applications.
Best Practices for Deployment
- Optimize your React build: Before deploying, ensure your app is production-ready by running build optimizations. This includes tree-shaking, minification, and code-splitting.
- Set up Continuous Integration (CI): Utilize CI tools like GitHub Actions or CircleCI to automate the deployment pipeline and ensure consistency across all environments.
- Configure Environment Variables: Manage environment variables securely through services like AWS Secrets Manager or Dotenv to prevent hardcoding sensitive data.
- Use a Content Delivery Network (CDN): A CDN will help reduce latency and speed up the loading time of static assets like images, videos, and stylesheets.
- Enable HTTPS: Secure your application with SSL certificates to ensure data privacy and security for your users.
"Efficient deployment not only improves the speed of the app but also ensures that users have a safe and seamless experience across different devices."
Post-Deployment Monitoring
Once the app is live, constant monitoring is essential to maintain optimal performance. Utilize tools such as:
Tool | Description |
---|---|
New Relic | Real-time application monitoring for performance issues and user behavior. |
Google Analytics | Track user engagement and app performance metrics. |
Datadog | Monitor infrastructure, logs, and errors to catch issues early. |
By leveraging these tools and following best practices, you ensure your social media app is scalable, secure, and ready for the next wave of users.