React Native Drag and Drop App Builder
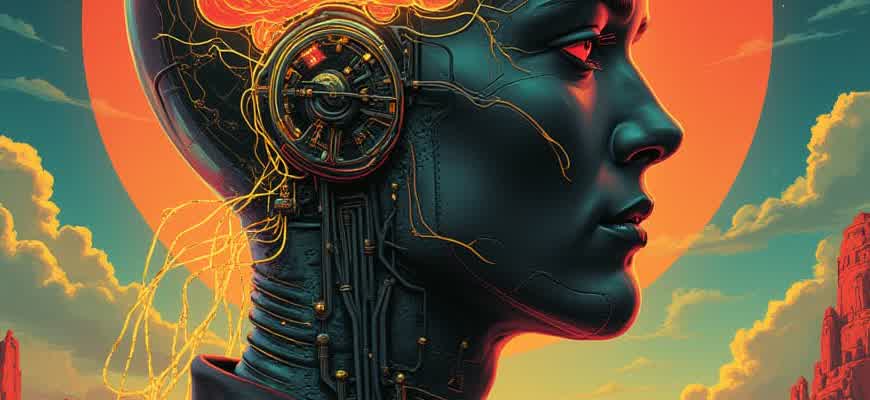
Creating interactive and intuitive mobile applications often requires dynamic user interfaces that enable drag-and-drop functionality. React Native provides an efficient platform for developers to implement such features with minimal effort. The drag-and-drop functionality can be achieved through various libraries and components that integrate seamlessly with React Native, offering flexibility and ease of use for mobile app development.
To build a successful drag-and-drop application, developers need to focus on key aspects such as event handling, component reordering, and performance optimization. The following points outline the core components of a drag-and-drop system:
- Drag source: The element that the user can drag.
- Drop target: The area or component where the dragged item can be dropped.
- Drag events: Handle the start, move, and end of a drag operation.
- State management: Maintain the state of the items being dragged and dropped.
React Native supports multiple libraries, such as react-native-gesture-handler and react-native-reanimated, which help developers manage gestures and animations efficiently during the drag-and-drop interaction. These tools enable smooth transitions and a responsive user experience.
React Native simplifies the process of creating sophisticated drag-and-drop features, empowering developers to focus on functionality rather than complex implementations.
When designing a drag-and-drop interface, it's crucial to maintain a balance between ease of use and visual clarity. Consider the following practices when structuring your layout:
- Ensure clear visual cues for draggable items.
- Implement smooth animations to enhance the user experience.
- Test performance on various devices to ensure responsiveness.
Component | Description | Library |
---|---|---|
Drag Source | Item that can be dragged. | react-native-gesture-handler |
Drop Target | Area where the dragged item is dropped. | react-native-reanimated |
Drag Events | Handles start, move, and end of drag action. | react-native-gesture-handler |
Why Opt for React Native for Drag-and-Drop Features in Mobile Applications?
When developing mobile applications that require drag-and-drop functionality, choosing the right framework is essential for creating a smooth user experience. React Native stands out for its ability to efficiently manage complex interactions and animations, all while maintaining performance across both iOS and Android platforms. The framework enables developers to use a single codebase, reducing both development time and costs without compromising the application's usability or performance.
React Native provides numerous libraries and components that simplify implementing drag-and-drop behavior. By leveraging native performance and modern JavaScript libraries, developers can create intuitive, responsive applications without sacrificing quality. Additionally, React Native’s flexibility ensures compatibility with third-party drag-and-drop libraries, enabling customization to meet specific project needs.
Key Advantages of React Native for Drag-and-Drop Implementation
- Cross-platform development: Write once, deploy on both iOS and Android, ensuring the same functionality on different devices.
- Native performance: React Native bridges JavaScript with native code, providing optimal performance for drag-and-drop gestures and transitions.
- Reusability of components: Reusable components in React Native speed up the development process, making it easier to manage complex drag-and-drop UI elements.
- Active community support: With React Native's large and growing community, developers have access to extensive documentation, tutorials, and reusable libraries for implementing drag-and-drop features.
Popular Libraries for Drag-and-Drop in React Native
- React Native Gesture Handler: A powerful library for handling gestures, providing smoother drag-and-drop interactions.
- React Native Draggable: Allows developers to implement draggable elements with ease, enabling intuitive UIs.
- React Native DnD: This library simplifies creating complex drag-and-drop interfaces with flexible features.
Performance and User Experience Considerations
Factor | Benefit of React Native |
---|---|
Responsiveness | Optimized performance across multiple devices with smooth interactions, minimizing lag. |
Flexibility | Easy to adapt and extend with custom animations and transitions for drag-and-drop. |
Development Speed | Reusable components and a unified codebase for both platforms speed up implementation. |
React Native's ability to integrate directly with native APIs ensures that drag-and-drop functionality feels fluid, while keeping the development process streamlined and efficient.
Step-by-Step Process to Integrate Drag and Drop into Your App
Incorporating drag and drop functionality into a mobile app can significantly enhance the user experience, especially in interactive applications like builders or editors. The process involves a series of steps that range from setting up the environment to configuring the drag-and-drop behavior. Here’s a guide to help you get started with integrating this feature into your React Native application.
To successfully implement drag-and-drop, it is crucial to choose the right tools and libraries. React Native provides several options, such as the "react-native-gesture-handler" library, which allows seamless interaction for draggable components. Below is a step-by-step process to integrate this functionality.
1. Install Required Dependencies
- First, you need to install the necessary libraries using npm or yarn:
- Run
npm install react-native-gesture-handler
to install the gesture handler. - Run
npm install react-native-reanimated
for animation support.
2. Set Up Gesture Handler
Once the dependencies are installed, it’s time to integrate the gesture handler into your app.
- Import the necessary components:
- Wrap the components you want to make draggable inside a
PanGestureHandler
.
import { PanGestureHandler } from 'react-native-gesture-handler';
3. Handle Gesture Events
Now, handle the gestures such as dragging by using the gesture handler's events.
- Define a
onGestureEvent
function to track the movement of the draggable item. - Use
Animated.Value
to animate the position of the item based on user interaction.
4. Example Code for Dragging
import React from 'react';
import { View, Text, Animated } from 'react-native';
import { PanGestureHandler } from 'react-native-gesture-handler';
const DraggableComponent = () => {
const translateX = new Animated.Value(0);
const translateY = new Animated.Value(0);
const onGestureEvent = Animated.event(
[{ nativeEvent: { translationX: translateX, translationY: translateY } }],
{ useNativeDriver: true }
);
return (
Drag Me!
);
};
export default DraggableComponent;
5. Testing and Optimization
Once the implementation is complete, test your app on different devices and platforms to ensure the drag-and-drop functionality works as expected. Make sure to optimize the performance, especially when dragging multiple components simultaneously.
Important: Always ensure smooth animations for better user experience. For performance optimization, use React Native's
useNativeDriver
to offload animations to native threads.
6. Troubleshooting
Issue | Solution |
---|---|
Item not moving smoothly | Ensure that useNativeDriver is set to true for all animations. |
Gestures are not being detected | Check if the PanGestureHandler is properly wrapped around the draggable item. |
Customizing Drag and Drop Components for Unique User Experiences
When designing a Drag and Drop application in React Native, the ability to customize components can significantly enhance the user experience. Custom components allow you to tailor the interaction flow, making it intuitive and engaging for users. By adjusting elements such as drag handles, drop zones, and feedback animations, you can create an environment that is not only functional but also visually appealing. These customizations help make the app feel more personalized and responsive to the user’s actions.
There are several strategies for personalizing the drag-and-drop components to suit specific app requirements. Below, we explore some techniques to implement these changes, focusing on visual design, user feedback, and interactive elements that contribute to a better experience.
Visual Customization Options
- Drag Handles: Customizing the look and feel of the drag handles can make the dragging process smoother and more intuitive. For example, changing the icon, size, or color of the handle based on its state (e.g., active or inactive) can improve the visibility of draggable items.
- Drop Zones: Adjusting the appearance of drop zones can provide users with visual cues. Different background colors or shadows can indicate where the dragged item will be dropped, making the interaction clearer.
- Interactive Animations: Adding animations when dragging or dropping items can enhance the visual feedback, making the app feel more dynamic and engaging.
Providing Interactive Feedback
- Hover Effects: Implementing hover effects on both draggable items and drop zones can visually signal when an item is ready to be moved or dropped.
- Snap-to-Grid: Enabling a snap-to-grid feature can help users align items more easily, ensuring a tidy and organized layout.
- Drag Ghosts: Changing the appearance of the item being dragged (e.g., a translucent copy) can give users a better sense of what’s being moved.
Key Considerations for Customization
Effective customization should focus on enhancing usability and reducing cognitive load. Avoid overwhelming the user with too many visual elements. Instead, aim for subtlety and clarity in your designs.
Table of Customization Elements
Customization Element | Description |
---|---|
Drag Handle Style | Adjusting size, color, and icon for better visibility and ease of use |
Drop Zone Design | Changing background color, borders, and shadows to indicate a valid drop area |
Interactive Animations | Adding movement or visual effects during drag and drop to enhance feedback |
Snap-to-Grid | Automatically aligning items to a grid after dropping for organized layouts |
Optimizing Performance of Drag and Drop in React Native Apps
Performance optimization in drag-and-drop interactions within React Native apps is crucial for ensuring a smooth user experience, especially on mobile devices with varying hardware capabilities. Efficiently handling drag events, animations, and rendering is key to minimizing jank and delays, which can negatively affect the app's responsiveness.
To achieve better performance, developers need to consider various strategies, from improving the layout rendering to using appropriate libraries and tools. In this guide, we'll explore several techniques that can significantly boost the performance of drag-and-drop interactions in React Native applications.
Key Optimization Strategies
- Use of Reanimated for Smooth Animations: The React Native Reanimated library allows for smoother animations by offloading animation calculations to the native thread, reducing performance bottlenecks associated with JavaScript thread.
- Efficient Rendering with FlatList: When handling large lists of draggable items, using FlatList over ScrollView ensures that only the visible items are rendered, preventing unnecessary re-renders.
- Memoization and PureComponent: Memoizing components with React’s React.memo and using PureComponent can prevent unnecessary re-renders during drag operations, improving the app's performance.
Reducing Re-renders and Event Overhead
- Minimize State Updates: Frequent state updates can lead to re-renders. Try to reduce the number of state changes during drag events or use useRef for non-state-related values.
- Throttle Drag Events: Throttling the drag event listeners ensures that they are not fired too frequently, which can lead to performance issues. Consider using requestAnimationFrame or a debouncing mechanism.
Use native driver for animations whenever possible to ensure smooth transitions. This minimizes the load on JavaScript thread and allows for a much more fluid drag-and-drop experience.
Best Practices for Optimized Drag-and-Drop Components
Technique | Impact |
---|---|
Native Driver for Animations | Offloads animation work to the native thread for smoother performance. |
Virtualized List Rendering | Reduces memory usage and unnecessary rendering by showing only the visible items. |
Efficient Drag Event Handling | Improves responsiveness by reducing the frequency of drag event listeners. |
Troubleshooting Common Issues in React Native Drag and Drop Development
When developing a drag-and-drop application in React Native, developers often face various challenges related to touch handling, performance, and integration with other components. Addressing these issues effectively requires a clear understanding of the core concepts and common pitfalls. Below are some of the typical problems and their solutions to improve the user experience and the app's performance.
One of the most frequent issues developers encounter is touch event handling, which can sometimes result in non-responsive drag actions. Another challenge is managing the positioning of draggable elements during interactions. These problems can typically be resolved with proper configuration and optimization techniques.
Common Problems and Solutions
- Touch Handling Delays – Delays in touch response can occur when the drag event is not properly debounced or throttled. This can be fixed by using optimized event listeners.
- Unintended Item Movement – If draggable items move unexpectedly, it may be due to incorrect calculations of their initial position. Ensure that the starting coordinates are correctly set based on the layout of the element.
- Performance Issues on Low-End Devices – Complex drag-and-drop interactions can slow down the app, especially on older devices. Use optimized libraries and reduce the amount of DOM manipulation during drag actions to mitigate this.
Debugging Tips
- Check Coordinates – Ensure the correct calculation of drag positions by inspecting the coordinates with console logs.
- Use Native Modules – For smoother performance, consider integrating native code using libraries like react-native-gesture-handler to handle touch events more efficiently.
- Test Across Devices – Always test on multiple devices and screen sizes to ensure consistent behavior of drag-and-drop features.
Example Troubleshooting Table
Issue | Possible Cause | Solution |
---|---|---|
Touch Event Not Firing | Incorrect touch event listener or binding | Ensure touch events are bound correctly to the target component |
Item Overlapping | CSS positioning errors | Verify that draggable items have absolute or relative positioning set |
App Lag on Drag | Too many re-renders during the drag operation | Optimize the component render cycle, or use memoization techniques |
Tip: For improved performance, use the useNativeDriver flag in animations to offload computations to the native layer, minimizing JS thread load.
Testing Drag and Drop Functionality in React Native Apps
Ensuring that drag-and-drop interactions work seamlessly in React Native applications is critical for providing an intuitive user experience. To test this functionality effectively, you need to focus on different aspects such as touch events, component rendering, and state management. The goal is to ensure that items can be dragged and dropped within the app without any glitches or unexpected behavior.
Testing drag-and-drop behavior requires both manual testing and automated tools. A combination of unit tests, integration tests, and end-to-end testing will give you a comprehensive understanding of how the feature performs across different devices and scenarios.
Manual Testing Process
Begin by testing the basic interactions on physical devices to identify any device-specific issues. Use a range of screen sizes and orientations to check responsiveness.
- Drag and drop items in different areas of the screen.
- Test with different numbers of draggable components.
- Verify that items maintain their position after being dropped.
Important: Always check the functionality on both iOS and Android as there might be platform-specific issues affecting behavior.
Automated Testing
Automated tests can be written using testing frameworks like Jest, along with libraries like React Testing Library or Enzyme. Focus on testing the following:
- State updates when dragging and dropping.
- Interactions between components before and after the drop event.
- Handling drag-and-drop events in edge cases (e.g., slow or fast gestures).
Automated tests help identify regressions in drag-and-drop functionality, saving time in manual testing while ensuring reliability during development.
Common Testing Tools
Consider using the following tools to assist in testing:
Tool | Description |
---|---|
Jest | Used for unit and integration testing of JavaScript code, including React Native apps. |
React Testing Library | Provides utilities to test React components and their behavior. |
Detox | An end-to-end testing framework for React Native apps, useful for testing drag-and-drop on actual devices. |