Android App Development Using Java Course
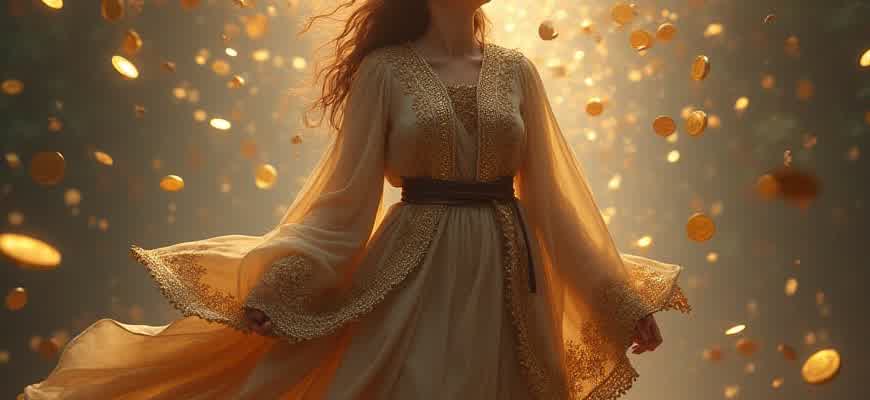
Java is one of the most widely used programming languages for Android app development. In this course, you will learn the fundamental and advanced concepts needed to build fully functional Android applications. The course is structured to provide both theoretical understanding and hands-on experience, enabling you to develop real-world applications.
Key topics covered include:
- Setting up the development environment with Android Studio
- Understanding Android components such as Activities, Services, and Broadcast Receivers
- Building user interfaces using XML and Java
- Working with databases and storing persistent data
- Integrating external APIs and services into your app
"This course is designed for developers who already have a basic understanding of Java and want to expand their skills into mobile app development."
The course structure includes a combination of theoretical lessons and practical coding exercises to ensure solid learning outcomes. Below is an overview of the course milestones:
Week | Topic | Key Concepts |
---|---|---|
1 | Introduction to Android Development | Setting up Android Studio, creating the first project |
2 | Understanding the Android Architecture | Activities, Views, and Layouts |
3 | User Interface Design | XML layouts, UI elements, event handling |
4 | Data Storage | SQLite, SharedPreferences, File I/O |
5 | Working with APIs | REST APIs, HTTP requests, JSON parsing |
Setting Up Android Studio for Java Development
To begin developing Android applications using Java, the first step is to correctly install and configure Android Studio. Android Studio is the official integrated development environment (IDE) for Android application development, and it supports Java as one of its primary programming languages. Below is a guide on how to set up Android Studio for Java-based Android app development.
Ensure that your system meets the minimum requirements for Android Studio. After that, you can proceed with the installation, configure the SDK, and get ready for development.
Steps to Install and Configure Android Studio
- Download Android Studio: Visit the official Android Studio website to download the latest version of the IDE for your operating system.
- Install Android Studio: Follow the installation prompts. On Windows, you can use the default settings, but on macOS or Linux, you may need to modify certain preferences based on your system.
- Configure Android SDK: During the installation process, Android Studio will ask you to install the Android Software Development Kit (SDK). This SDK is essential for building and running Android apps.
- Set up Java Development Kit (JDK): Android Studio requires JDK for Java-based projects. If it’s not installed on your system, download the JDK and link it with Android Studio through the IDE settings.
Important: Make sure you install the latest JDK version compatible with Android Studio, which is typically JDK 11 or newer for the best performance.
Configuring Android Studio for Java Projects
- Set Project Language to Java: When creating a new project, ensure you select Java as the primary language. Android Studio will automatically set up necessary files for a Java project.
- Update Gradle Files: Gradle is used to manage project dependencies. Verify the build.gradle files for correct dependencies, particularly those needed for Java development.
- Create a Virtual Device: You can test your Java-based Android app using Android Emulator. Create a virtual device for testing by using AVD Manager within Android Studio.
Essential Configuration Table
Configuration | Action |
---|---|
SDK Installation | Install Android SDK during setup |
JDK Version | Ensure JDK 11 or later is installed |
Android Emulator | Configure and run a virtual device using AVD Manager |
Understanding Android Architecture for Java Developers
For Java developers transitioning to Android development, it’s essential to understand the core structure and components of the Android platform. Android applications are built on a layered architecture, where each layer has distinct responsibilities and operates independently. This separation allows for greater flexibility, scalability, and maintainability in app development. Knowing how to navigate through these layers is crucial for effective app development and debugging.
The Android operating system is composed of several key components that work together to provide a responsive and seamless user experience. These components are arranged in a stack, with each layer building upon the one below it. Java developers need to familiarize themselves with these layers to optimize performance, manage resources efficiently, and ensure that their applications are scalable and robust.
Key Layers in Android Architecture
- Linux Kernel: The foundation of Android, handling hardware abstraction, drivers, and system resources.
- Android Runtime (ART): Provides the environment for running Java-based applications, managing memory and performance.
- Application Framework: A collection of reusable components and services for app development, such as UI elements and location services.
- Applications: The top layer where end-user apps are created and executed, relying on the lower layers for functionality.
Android Components for App Development
Within the Android framework, key components play a central role in developing Android apps. These include:
- Activities: Represent a single screen of interaction within an app.
- Services: Run in the background to perform long-running operations without UI interaction.
- Broadcast Receivers: Handle system-wide or app-specific messages from other apps or the system itself.
- Content Providers: Facilitate data sharing between different apps, ensuring that data is accessible across the system.
Important: Understanding these components helps Java developers map their existing knowledge of object-oriented programming and event-driven systems onto the Android framework, making the transition smoother.
Android Development Flow
Stage | Description |
---|---|
Design | Developing the UI and user interaction elements using XML layouts and defining the flow of activities. |
Implementation | Writing Java code to handle the app’s logic and interactions with Android services and components. |
Testing | Using Android-specific tools to test app functionality and ensure compatibility across devices. |
Deployment | Building the APK and deploying it to the Google Play Store or distributing it privately. |
Building Your First Android App with Java: A Step-by-Step Guide
Creating your first Android app can be an exciting and rewarding experience. With Java as your primary programming language, you can develop a wide range of mobile applications. In this guide, we'll walk you through the process of building a simple Android app using Java and Android Studio.
The goal of this tutorial is to help you understand the basic components of an Android app, including activities, layouts, and user interfaces. By the end of this step-by-step guide, you'll have a functional app ready to run on an Android device or emulator.
Step 1: Set Up Your Development Environment
Before you can start building your app, you need to set up your development environment. Here’s how you can do that:
- Download and install Android Studio from the official website.
- Open Android Studio and set up a new project.
- Select Java as the programming language and choose a template for your app (e.g., "Empty Activity").
- Ensure you have the Android SDK installed and updated.
Important: Android Studio will handle most of the setup automatically, but make sure your system meets the necessary requirements for smooth operation.
Step 2: Create a Basic User Interface
Next, you will design the user interface (UI) for your app. The UI is defined using XML files in Android, which specify how elements are arranged on the screen. Here's how to create a simple UI:
- Open the "activity_main.xml" file, located in the "res/layout" folder.
- Use the XML tags to add elements like buttons, text fields, and labels. For example, to add a button, you can use:
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me!" />
Once you've added the necessary elements to your layout, you can link them to your Java code to make the app interactive.
Step 3: Add Functionality with Java
Now that your UI is set up, it's time to add functionality using Java. In this step, you'll define what happens when a user interacts with your app, such as when they click a button.
- Open the "MainActivity.java" file, which is where the logic of your app is implemented.
- Find the method onCreate(), which is called when the app starts. Inside this method, you can set up your button click listener.
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getApplicationContext(), "Button Clicked!", Toast.LENGTH_SHORT).show();
}
});
In this example, when the button is clicked, a toast message will appear on the screen.
Step 4: Test Your App
Once you have written the code, it's time to test your app. You can run your app on an Android emulator or a physical device:
- Click on the "Run" button in Android Studio to launch your app.
- Select an emulator or connected device to deploy the app.
- Ensure that your app functions as expected by testing all interactive elements.
Tip: Use Android Studio’s built-in tools to debug and test your app efficiently.
Conclusion
You've now built your first simple Android app with Java. As you gain more experience, you can start adding more features, such as data storage, network communication, and custom UI components. The possibilities are endless!
Working with Java-based UI Components in Android
In Android development, the user interface (UI) plays a critical role in providing users with a seamless experience. Java is one of the primary programming languages used to build interactive UIs in Android apps. Understanding how to utilize Java-based UI components is essential for creating responsive, visually appealing applications. Android provides a variety of UI elements, such as buttons, text fields, and lists, that can be customized and manipulated programmatically using Java.
UI components in Android are typically implemented in XML layout files and are accessed and modified through Java code. These components include basic elements like Buttons, TextViews, EditTexts, as well as more complex ones such as RecyclerViews and ImageViews. Java allows developers to manage interactions with these components through event listeners, handlers, and specific methods that manipulate their properties and behavior.
Basic UI Components in Android
Several fundamental UI components can be easily integrated into Android applications. Below is a list of common components and their purpose:
- Button: Triggers actions when clicked by the user.
- TextView: Displays text in the UI, typically used for labels or information.
- EditText: Allows the user to input text or data.
- ImageView: Displays images in the application interface.
These components can be configured using Java code in the Activity class or other UI-related classes. Below is a simple example of how a button can be set up and have a click event attached in Java:
Button myButton = findViewById(R.id.my_button);
myButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Action when button is clicked
}
});
Working with Lists and Grids
Android provides powerful components for displaying collections of data, such as ListView and RecyclerView. These components allow developers to display data in a scrollable list or grid format. The RecyclerView is particularly versatile and can be customized for complex layouts, improving performance compared to the older ListView.
Component | Usage | Key Advantage |
---|---|---|
ListView | Displays a vertically scrollable list of items. | Simple implementation, suitable for basic lists. |
RecyclerView | Displays a collection of items in various layouts (e.g., grid, linear). | Highly flexible, better performance with large data sets. |
Using Java, you can control the interaction with these components, such as updating the content dynamically or responding to user clicks. RecyclerView, in particular, requires the implementation of an adapter class that manages the display of data within each item of the list or grid.
Note: Always ensure to manage UI updates on the main thread to prevent performance issues in your Android applications.
Integrating APIs and Web Services in Your Java Android App
In modern Android app development, integrating external data and services through APIs and web services is a critical step for enhancing app functionality. Java provides several mechanisms for interacting with APIs, enabling developers to extend their applications with real-time data, cloud services, and third-party integrations. By connecting your app to external servers, you can fetch data, post updates, and leverage various services, such as payment gateways, maps, or social media platforms.
This process typically involves handling network requests, parsing responses, and updating the UI with the fetched data. Developers can make synchronous or asynchronous requests using libraries like Retrofit, OkHttp, or Volley. These tools simplify the process of handling HTTP requests, managing responses, and reducing boilerplate code.
Key Steps to Integrate APIs
- Choose an appropriate library for network communication (e.g., Retrofit, Volley).
- Obtain API credentials or access tokens from the service provider.
- Make requests to the API endpoints using the chosen library.
- Parse the JSON or XML responses into Java objects.
- Display the data in your app’s user interface.
Note: Always ensure proper handling of errors, such as network failures or invalid responses, to improve app stability.
Example of API Integration with Retrofit
- Define a Retrofit instance with a base URL:
- Create an interface for defining the API endpoints:
- Make the API request and handle the response asynchronously:
Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .build();
public interface ApiService { @GET("data") CallgetData(); }
ApiService apiService = retrofit.create(ApiService.class); Callcall = apiService.getData(); call.enqueue(new Callback () { @Override public void onResponse(Call call, Response response) { if (response.isSuccessful()) { // Update UI with response data } } typescriptEdit @Override public void onFailure(Call call, Throwable t) { // Handle failure } });
Common Challenges and Solutions
Challenge | Solution |
---|---|
Handling slow network responses | Use background threads or AsyncTask to avoid blocking the main UI thread. |
Parsing complex JSON data | Use libraries like Gson or Moshi to simplify the conversion of JSON to Java objects. |
Dealing with authentication | Use OAuth 2.0 or API tokens for secure authentication methods. |
Debugging and Testing Java Android Applications
When developing Android applications using Java, debugging and testing are critical steps to ensure that your app works as intended. These processes help identify and fix errors, improve app performance, and enhance user experience. Effective debugging requires understanding the common issues that may arise, such as incorrect logic, memory leaks, or resource mismanagement. Testing ensures that the app functions correctly under different conditions and on various devices, which is crucial for user satisfaction and app reliability.
Debugging and testing Java Android applications require a systematic approach. Android Studio provides a range of tools to assist with these tasks. The built-in debugger helps to track down issues by setting breakpoints, inspecting variables, and stepping through code. Additionally, testing frameworks like JUnit and Espresso allow developers to perform unit and UI tests, ensuring that individual components and the overall user interface are functional.
Debugging Tools and Techniques
- Logcat: A powerful logging tool that helps developers view log messages from the app while it's running, providing insights into app behavior and errors.
- Breakpoints: Set breakpoints in the code to pause execution and inspect variables at runtime.
- Stack Traces: Use stack traces to identify the source of crashes and errors in the application.
Testing Strategies
- Unit Testing: Writing unit tests for individual functions or methods to ensure they behave correctly.
- UI Testing: Using tools like Espresso to test the app’s user interface to make sure it responds appropriately to user actions.
- Integration Testing: Verifying that different modules of the app work together as expected.
Remember to test your app on multiple devices and Android versions to account for different screen sizes, performance levels, and OS behaviors.
Common Debugging and Testing Mistakes
Issue | Solution |
---|---|
Not using breakpoints effectively | Use breakpoints to pause code at key points and inspect variable values to better understand the app's state. |
Overlooking edge cases in testing | Ensure tests cover various input scenarios and edge cases, such as invalid data or network failures. |
Improving Efficiency in Java-Based Android Applications
Performance optimization is crucial in developing Android apps, especially when using Java. Ensuring smooth user experience requires understanding both the hardware limitations of devices and the software intricacies of Android. By addressing common performance bottlenecks and applying targeted solutions, developers can create responsive and efficient applications that consume fewer resources, enhancing both speed and user satisfaction.
Effective performance optimization includes memory management, reducing CPU usage, and optimizing network calls. Below are some important techniques and considerations for improving the performance of Java-based Android applications.
Memory Management and Efficient Data Handling
In Android, memory is a limited resource, and efficient memory management is key to maintaining app performance. Developers should ensure that memory usage is minimized by eliminating unnecessary objects, using memory-efficient data structures, and properly managing memory allocation and deallocation. Some approaches to optimize memory include:
- Use Object Pooling to avoid frequent allocation and deallocation of objects.
- Minimize the use of large objects in memory, especially during UI updates.
- Leverage Weak References for objects that can be garbage collected when not in use.
Efficient memory management ensures that your app runs smoothly even on devices with lower RAM.
Reducing CPU Load and Improving Responsiveness
Minimizing the CPU load is another critical aspect of performance optimization. Excessive CPU usage can lead to slow app performance and battery drain. Developers should consider the following practices:
- AsyncTask or Threads for heavy background operations to avoid blocking the main UI thread.
- Optimize algorithms to reduce their time complexity, such as using more efficient sorting or searching algorithms.
- Avoid unnecessary background services that consume CPU cycles.
Optimizing Network Operations
Network calls often impact app performance, especially when dealing with slow or unreliable connections. Optimizing network usage is crucial to ensure smooth user experiences. Some approaches include:
Optimization Method | Description |
---|---|
Compression | Compress data before sending it over the network to reduce transfer time. |
Caching | Use local caching mechanisms to avoid repeated network requests for the same data. |
Batching Requests | Combine multiple network requests into a single request to minimize network overhead. |
Network optimizations can significantly reduce the latency and improve the perceived responsiveness of your app.