Android App Development Using Python
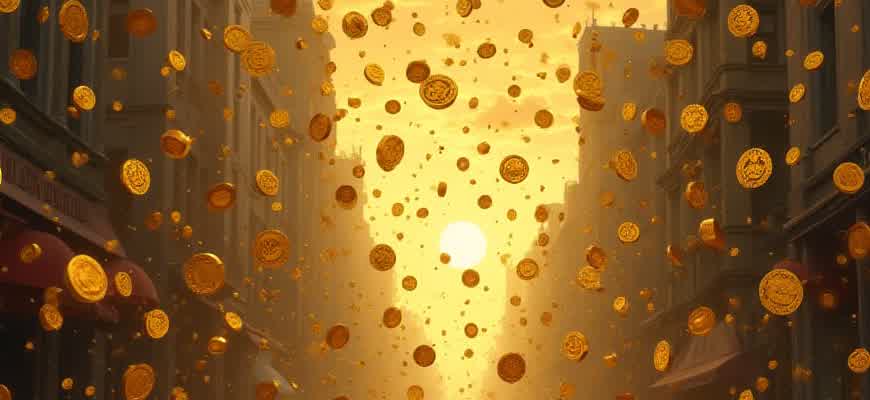
Building Android applications using Python has become increasingly popular due to the accessibility of various tools and frameworks. While Android development traditionally relies on Java or Kotlin, Python offers an alternative for those familiar with its syntax. The primary tools for Python-based Android development include Kivy, BeeWare, and PySide. These frameworks allow developers to write cross-platform apps that can run on both Android and other operating systems.
Key Tools for Python Android Development:
- Kivy: A popular library for rapid development of multi-touch applications, supporting a wide range of platforms.
- BeeWare: A set of tools and libraries for building native user interfaces on various platforms, including Android.
- PySide: A Python binding for the Qt application framework, suitable for creating desktop and mobile applications.
Advantages of Using Python for Android App Development:
- Familiarity for Python developers: If you already know Python, transitioning to mobile development becomes easier.
- Cross-platform support: Many frameworks allow you to build apps that work not only on Android but also on iOS and other platforms.
- Rapid prototyping: Python’s syntax is simple, making it easier to quickly prototype and iterate on app features.
Python, when combined with powerful frameworks like Kivy and BeeWare, provides a robust environment for creating Android applications without the steep learning curve associated with Java or Kotlin.
Development Process Overview:
Step | Description |
---|---|
Setup Environment | Install necessary tools like Python, Kivy, or BeeWare along with Android SDK. |
Code Your Application | Write the core functionality using Python and the chosen framework. |
Test Your App | Test on Android emulator or device to ensure compatibility and functionality. |
Setting Up the Python Environment for Android App Development
To begin developing Android applications with Python, it is essential to properly set up your development environment. Unlike traditional Android development using Java or Kotlin, Python requires specific tools and frameworks to work within the Android ecosystem. These tools will bridge the gap between Python and Android's native libraries, allowing developers to create fully functional apps using Python code.
One of the most popular approaches for this purpose is using Kivy, BeeWare, or Chaquopy, which provide the necessary framework to run Python applications on Android. Before starting, ensure that your system is ready by installing a few key tools and dependencies.
Key Steps for Python Environment Setup
- Install Python: Ensure that Python is installed on your system. It is recommended to use Python 3.8 or higher for compatibility with most Android development frameworks.
- Install Java Development Kit (JDK): The Android build system requires the JDK. Make sure you have the latest version of JDK installed.
- Install Android Studio: Android Studio is the official IDE for Android development. It is crucial for compiling and building apps, even if you're using Python as the primary language.
Setting Up Kivy for Android Development
- Install Kivy using pip:
pip install kivy
- Install Buildozer, which is used to compile Python applications for Android:
pip install buildozer
- Create a Buildozer configuration file to specify Android-specific settings:
buildozer init
Important: When using Kivy for Android development, remember that you may need to set up a virtual environment to avoid conflicts with other Python libraries. You can do this by using venv or conda.
Additional Tools and Considerations
Tool | Description |
---|---|
BeeWare | A Python toolkit for building native apps, including Android. It provides a comprehensive set of libraries and tools. |
Chaquopy | A plugin for integrating Python with Android Studio, allowing you to write both Java and Python code in the same project. |
Choosing the Best Framework for Android App Development: Kivy vs BeeWare
When it comes to developing Android applications using Python, selecting the right framework is critical to the success of the project. Two of the most widely discussed frameworks are Kivy and BeeWare, both offering a set of unique features and trade-offs. Understanding the strengths and weaknesses of each is essential for making an informed decision based on the specific requirements of the application.
Both Kivy and BeeWare allow Python developers to build cross-platform mobile apps, but they cater to slightly different needs. Kivy is highly focused on providing a simple, interactive user interface for applications, whereas BeeWare aims to provide a more native feel by integrating with platform-specific APIs. To help you make the right choice, let's take a closer look at their key differences.
Key Features Comparison
Aspect | Kivy | BeeWare |
---|---|---|
Ease of Use | Relatively easy to set up and use, with good documentation. | More complex, requires additional configuration for native look and feel. |
Native Look and Feel | Less emphasis on native appearance; has its own UI toolkit. | Focuses on creating truly native applications using platform-specific widgets. |
Performance | Good performance for basic applications, but can struggle with complex graphics. | Performance varies depending on the platform, with an emphasis on stability over raw power. |
Cross-Platform Support | Supports Android, iOS, Linux, Windows, macOS, and Raspberry Pi. | Supports Android, iOS, macOS, Windows, and Linux but is still under development for mobile platforms. |
Considerations for Selecting the Right Framework
- Project Complexity: Kivy may be a better choice for projects that require rapid development with simple user interfaces.
- Native User Experience: BeeWare excels if your goal is to create applications with a native feel on each platform.
- Community and Support: Kivy has a larger and more established community, providing easier access to support and resources.
- Performance Needs: If your application demands high performance, Kivy might be preferable for its optimization capabilities.
Note: While Kivy is often chosen for its simplicity and flexibility, BeeWare is a better option when native integration is a top priority.
Building a Basic Android Application with Kivy
Developing Android applications with Python has become easier thanks to frameworks like Kivy. This open-source Python library allows developers to create cross-platform mobile applications, including Android apps, with a single codebase. Kivy is highly customizable, offering a wide range of built-in widgets and tools to create interactive UIs. In this guide, we’ll walk through the process of building a simple Android app using Kivy, from setting up the environment to running the app on an Android device.
To begin with, you'll need a few essential tools and libraries installed. Kivy supports Android development through the use of Buildozer, a tool that automates the process of packaging your Python application for Android. Once everything is set up, you can start developing your app using Kivy's Python API.
Steps to Build an Android App with Kivy
The following steps outline how to create a simple app:
- Install Dependencies: First, install Python, Kivy, and Buildozer. You can install Kivy using pip:
- pip install kivy
- Create a Python Script for Your App: Write the Python code for your app using Kivy's widgets. Here's a basic example:
- Package the App for Android: Use Buildozer to package the app into an APK file. The following command will initiate the process:
- buildozer android debug
- Install the APK: Once Buildozer completes the build, you can install the APK on your Android device.
from kivy.app import App from kivy.uix.button import Button class MyApp(App): def build(self): return Button(text='Hello, Android!') if name == 'main': MyApp().run()
Remember to ensure your device is connected via USB and debugging mode is enabled to install the APK.
Key Components of Kivy
To understand how Kivy works, here are some important elements that you’ll use:
Component | Description |
---|---|
App | Core class that manages your application. It is the entry point to the app. |
Widgets | Elements like buttons, labels, and text inputs that make up the UI. |
Layouts | Used to arrange widgets in a structured manner. |
Integrating Android APIs with Python: A Step-by-Step Approach
Integrating Android APIs with Python involves connecting Python code to various Android features and services that are typically accessed via Java or Kotlin. This can be done through tools and frameworks that allow Python to communicate with Android's native capabilities. The process opens up opportunities to build Android apps with the simplicity and flexibility of Python while leveraging Android’s robust API ecosystem.
One popular way to integrate Android APIs with Python is by using frameworks like Kivy or BeeWare. These tools allow Python developers to write mobile applications that can run on Android, making it easier to incorporate Android-specific features like GPS, camera, and sensors. The integration, however, requires a detailed understanding of both the Android API and the framework used to bridge Python with Android.
Step-by-Step Guide
- Set up the development environment: Install necessary Python libraries and Android SDK. Frameworks like Kivy or BeeWare provide all the tools required to develop mobile apps in Python.
- Create a new project: Begin by setting up a new Android project using your chosen framework (e.g., Kivy). This typically involves initializing the project structure and configuring dependencies.
- Access Android APIs: Use the Android API calls through the framework. For example, Kivy allows Python code to interact with Android’s Java-based API via Pyjnius, a tool that bridges the gap between Python and Java.
- Integrate Android features: Implement features like location services, camera, and sensors by calling Android’s built-in methods via Python code. Ensure permissions are correctly set in your Android manifest file.
- Test and debug: Test your app on an emulator or physical device, ensuring that all integrated APIs work as expected. Use logging to troubleshoot issues and refine the app functionality.
Note: While Python simplifies development, you might need to dig into Java or Kotlin for complex Android-specific features that are not directly accessible from Python.
Common Android API Integrations
Feature | Python Integration |
---|---|
Location Services | Use Kivy’s GPS module or Pyjnius to access Android's location APIs. |
Camera | Access Android camera API through Pyjnius for capturing photos or video. |
Push Notifications | Leverage Firebase Cloud Messaging with Python bindings for sending notifications. |
Debugging Android Apps Written in Python: Tools and Techniques
Debugging is a crucial part of the development process, especially when working with Android apps written in Python. Since Android is primarily designed to work with Java and Kotlin, Python developers face unique challenges. However, with the right tools and techniques, these challenges can be overcome efficiently.
For Python-based Android development, the key is understanding the integration of Python with Android frameworks, such as Kivy, BeeWare, or Chaquopy. Debugging often involves both native Android code and Python code, requiring a mix of tools designed for both environments. Effective debugging not only ensures app stability but also improves performance and user experience.
Common Tools for Debugging Python Android Apps
- PyCharm or Visual Studio Code: Both of these IDEs offer powerful debugging features such as breakpoints, variable inspection, and step-through code execution. They are essential for catching logical errors and managing Python code behavior effectively.
- Android Studio with Python Plugin (Chaquopy): Android Studio is primarily for Java and Kotlin, but with the Chaquopy plugin, Python code can be integrated and debugged directly within the Android Studio environment.
- Logcat: A built-in tool in Android Studio, Logcat allows for tracking errors, warnings, and logs from both the native Android and Python components. This is particularly helpful for debugging runtime errors and viewing logs in real time.
Effective Debugging Techniques
- Use Breakpoints: Set breakpoints in both your Python code and Android components. This allows you to stop execution at key points and inspect variables, helping to identify issues in the app flow.
- Check Log Outputs: Regularly monitor log outputs, especially when debugging Python-to-Java interactions. Logcat will display detailed error messages that can provide insight into where the problem originates.
- Unit Testing: Create unit tests for both the Python code and the Android interface. This will help isolate issues and ensure that individual components of the app are functioning correctly before integrating them into the full application.
Helpful Information
Tip: When debugging Android apps written in Python, focus on areas where Python interacts with native Android APIs. Pay attention to issues related to memory management and threading, as Python's performance on Android can sometimes differ from typical desktop environments.
Performance Monitoring
In addition to standard debugging tools, performance profiling is essential for ensuring that the Python code runs efficiently on Android devices. Python apps, especially when using frameworks like Kivy, may not always provide the same performance as native Java/Kotlin apps. Using tools like Android Profiler (available in Android Studio) can help detect CPU and memory usage spikes that may arise from inefficient Python code.
Key Debugging Resources
Resource | Description |
---|---|
PyCharm Debugger | Offers advanced debugging features for Python development, including visual debugging and runtime variable tracking. |
Android Studio Logcat | Essential for viewing logs and debugging both Python and Android code in a single platform. |
Chaquopy | A plugin that enables seamless integration of Python in Android Studio, allowing for a unified debugging process. |
How to Enhance the Performance of Python-Based Android Applications
Developing Android applications using Python can be a rewarding experience, but ensuring optimal performance requires careful consideration of multiple factors. Python is known for its simplicity, but it may not always be the most efficient language when compared to Java or Kotlin, especially when targeting resource-constrained devices like smartphones. Optimizing performance involves fine-tuning both the Python code and the Android-specific configurations. Below are some key strategies to improve the efficiency of Python-based Android apps.
To get the best performance, developers need to make use of optimized libraries, limit the use of heavy computations, and manage memory effectively. Also, leveraging the right tools for compiling Python code into machine code can significantly boost execution speed. It's essential to adopt a holistic approach to app optimization by addressing both the Python environment and the Android system.
Key Optimization Strategies
- Use Pyjnius and Kivy for Native Components: When working with Android APIs, Pyjnius allows access to Java classes, while Kivy offers highly optimized UI elements. Both tools help bridge the gap between Python and Android's native performance.
- Reduce CPU Usage with Efficient Algorithms: Avoid using complex or nested loops. Focus on algorithmic efficiency and utilize libraries like NumPy for mathematical operations to minimize the CPU load.
- Optimize Memory Usage: Python’s memory management can be a concern on mobile devices. Use garbage collection and consider memory pools to handle objects efficiently.
Important Considerations
Keep in mind that Python apps often run slower than native Android apps written in Java or Kotlin. Although Python is flexible, it lacks direct access to Android’s low-level functionalities, which can result in slower performance.
Tools for Code Optimization
- Cython: A superset of Python that compiles code into C, improving execution speed and reducing memory usage.
- Chaquopy: A plugin that allows mixing Python code with Java, enabling more control over performance-critical operations.
- Pydroid: A Python IDE for Android that can help test and optimize Python-based applications on actual devices.
Performance Comparison Table
Approach | Performance Impact | Usage |
---|---|---|
Native Android Development (Java/Kotlin) | High | Best for performance-critical applications |
Python (with Kivy/Pyjnius) | Moderate | Good for rapid development, moderate performance needs |
Python (via Cython) | Improved | Suitable for computational-heavy tasks |
Packaging and Deploying Python-Based Android Apps to the Google Play Store
Deploying a Python-based Android app requires several steps to ensure the app functions properly and meets all the criteria set by Google Play. This process includes converting the Python code into a format that Android devices can execute, optimizing the app for performance, and preparing it for publication. The most common approach is using tools like Kivy, BeeWare, or Chaquopy to compile the app into a native APK (Android Package) file.
Once the app is packaged, developers need to go through the deployment process. This involves signing the APK, creating a developer account on the Google Play Console, and uploading the APK for distribution. Below are the key steps for packaging and deploying an app built with Python:
Steps for Packaging and Deploying the Python Android App
- Prepare the Development Environment: Install necessary tools such as Python, Kivy, Android SDK, and Java Development Kit (JDK).
- Package the App: Use Kivy or another framework to compile the app into an APK file. This involves creating a build configuration and running the build command.
- Sign the APK: Sign the APK with a valid key to ensure its authenticity and security before submitting to the Play Store.
- Create a Google Play Developer Account: Register as a developer on the Google Play Console, which requires a one-time registration fee.
- Upload the APK: Go to the Google Play Console and upload the APK file. Fill in necessary app details like description, screenshots, and category.
- Publish the App: Once all details are complete and the app is reviewed, click the "Publish" button to make the app available on the Play Store.
Important Considerations for Deployment
Always ensure that your app adheres to Google Play's policies regarding privacy, security, and content before submission. Non-compliance may result in the app being rejected or removed from the store.
After the app is published, developers should monitor its performance, respond to user feedback, and release updates as needed. Optimizing the app for various Android versions and screen sizes is essential for providing the best user experience.
Packaging Tools Comparison
Tool | Advantages | Disadvantages |
---|---|---|
Kivy |
|
|
BeeWare |
|
|
Chaquopy |
|
|