Build a Crm with React
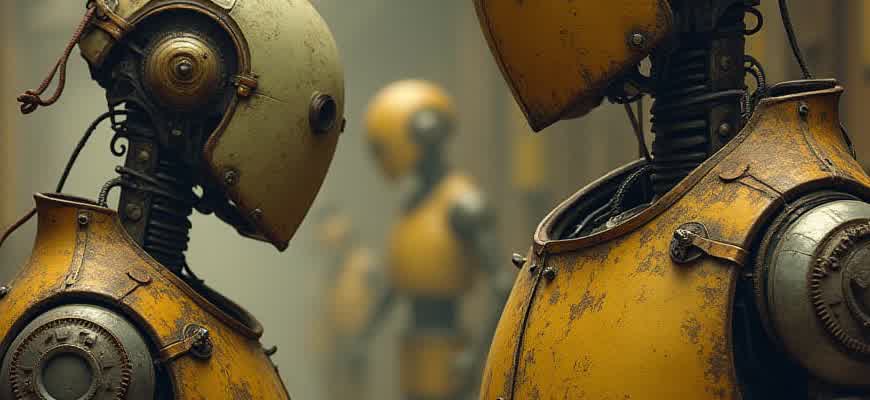
Creating a Customer Relationship Management (CRM) system with React involves designing an interactive and dynamic interface that allows businesses to manage customer interactions effectively. The key components of the application include user management, data visualization, and seamless communication features. By leveraging React's component-based architecture, you can create reusable elements that make the development process more efficient.
To begin, it’s important to understand the structure of a basic CRM system. The primary functions usually include:
- Storing customer data such as contact information, purchase history, and preferences.
- Providing tools for managing customer communications like emails, phone calls, and messages.
- Tracking customer interactions and engagement through a dashboard.
Tip: Focus on creating a scalable and modular architecture to ensure easy maintenance and future updates.
As you start to build the CRM, you'll need to integrate a variety of technologies alongside React. One of the essential tools is a database to store and retrieve customer information. Typically, developers use back-end technologies like Node.js or Python with a database like MongoDB or PostgreSQL. Here’s an overview of the steps involved:
- Set up the backend and connect it to a database to store the CRM data.
- Develop the React components for the front-end interface, such as forms for adding new customers or editing existing data.
- Integrate API calls to fetch or update customer information in real-time.
Component | Purpose |
---|---|
Customer List | Displays a list of all customers stored in the database. |
Customer Form | Allows adding or updating customer data. |
Dashboard | Shows metrics like total number of customers, engagement stats, etc. |
Building a CRM Application with React: A Practical Approach
Creating a CRM system with React offers developers a powerful way to manage customer data while leveraging the flexibility and performance that React provides. This guide will cover the essential components of building a CRM, including creating a user interface, managing state, and ensuring a smooth user experience. With the combination of React's reactivity and component-based structure, building a CRM becomes both scalable and maintainable.
By breaking down the process into smaller tasks, we can tackle the development of a CRM step-by-step. From setting up the project structure to integrating essential functionalities like contact management, lead tracking, and analytics, React allows developers to create efficient solutions for real-world business needs. The use of React hooks, context, and modern JavaScript features further enhance the development process.
Key Features to Implement in Your CRM
- Contact management: Create, update, and delete customer profiles.
- Lead tracking: Track potential customers through the sales pipeline.
- Analytics dashboard: Provide insights into business performance.
- User authentication and authorization: Secure access to different levels of CRM features.
Step-by-Step Development Process
- Set up the project with Create React App or another boilerplate.
- Create reusable components for the UI, such as contact forms and tables.
- Implement state management using React hooks or context API for shared state.
- Integrate backend services (e.g., Firebase or Node.js) for storing customer data.
- Add routing for different views like contact lists, lead pipelines, and reports.
- Test the app thoroughly, focusing on performance and security.
Example of a Simple CRM Table
Customer Name | Phone | Status | |
---|---|---|---|
John Doe | [email protected] | +1234567890 | Lead |
Jane Smith | [email protected] | +0987654321 | Customer |
Tip: Keep your components small and reusable to improve maintainability and reduce complexity as your CRM grows.
Setting Up the Project: Create a React App from Scratch
To begin building a CRM with React, it's essential to set up the project correctly. The first step is to create a React application from the ground up. React provides an efficient way to manage UI components, and setting up the project ensures you can easily scale your application as the requirements grow.
We'll use the React toolchain and the Node.js environment to get started. Make sure you have Node.js installed on your machine before proceeding with the setup.
Steps to Initialize the React Project
- Install Node.js: If you haven't already, download and install the latest version of Node.js from the official website.
- Use Create React App: This tool sets up a new React project with a good default configuration.
- Create the Application: Run the following command in your terminal:
npx create-react-app crm-app
- Navigate to the Project Directory: After the app is created, move into the directory:
cd crm-app
- Start the Development Server: Begin working on the app by running:
npm start
Note: If you prefer a custom setup, you can skip Create React App and configure Webpack and Babel manually, but using the React CLI tool is faster and more efficient for most projects.
Project Structure Overview
Once the app is created, the following basic structure will be generated:
Folder/Files | Description |
---|---|
public/ |
Contains the static files like index.html and icons. |
src/ |
Where all your React components and logic will reside. |
package.json |
Contains project metadata, dependencies, and scripts. |
node_modules/ |
Stores all the installed dependencies. |
Important: Keep your
src/
folder organized as the project grows, especially with components and services for better maintainability.
Choosing the Right State Management for Your CRM
When building a CRM with React, the choice of state management is crucial to ensure smooth performance and easy maintainability. A CRM application typically involves managing complex data structures such as contacts, tasks, and notes. Therefore, selecting the appropriate state management solution becomes essential to handle data flow efficiently, ensure synchronization, and prevent unnecessary re-renders.
There are multiple options available for state management in React, each with its own strengths and weaknesses. It is important to assess the complexity of your CRM, the team’s familiarity with specific tools, and the scale of the application before making a decision. Below are some of the most popular approaches.
State Management Options
- React Context API: Suitable for simpler applications where the global state does not change frequently. This solution avoids the overhead of setting up external libraries.
- Redux: Provides a more scalable solution for larger applications. It offers a centralized store and middleware for side-effect management, making it ideal for complex CRM systems that require extensive state manipulation.
- Zustand: A lighter alternative to Redux, Zustang is easier to integrate and can be a good choice for small to medium CRM applications.
Key Considerations
- Complexity: If your CRM is going to handle multiple components with intricate state dependencies, solutions like Redux or Zustand are more appropriate due to their middleware capabilities.
- Performance: React Context API can cause unnecessary re-renders in large applications. This can be mitigated by using techniques like memoization, but for larger CRMs, Redux or Zustand would be better suited for performance optimization.
- Learning Curve: Redux has a steeper learning curve, while Context API is more intuitive and easier to implement, especially for smaller apps.
Important: Always consider the scalability of your application. A simple CRM may benefit from Context API, but as your application grows, you might find yourself needing the added capabilities of Redux or other advanced state management tools.
State Management Comparison
Solution | Complexity | Performance | Ease of Use |
---|---|---|---|
React Context API | Low | Medium | High |
Redux | High | High | Low |
Zustand | Medium | High | Medium |
Designing a Scalable Data Structure for CRM Contacts
When building a CRM system, designing a data structure to efficiently store and manage contact information is crucial. A scalable design ensures that as the number of contacts increases, the system remains performant and easy to maintain. The contact data structure should be flexible enough to accommodate various types of information, such as personal details, communication history, and relationship status, while also allowing for future growth and changes in requirements.
One key consideration when designing a contact data structure is to ensure it is optimized for both read and write operations. Efficient querying is important for accessing contact details, while writing updates and adding new entries should be quick. The design should also allow for easy modifications as business needs evolve, such as adding new fields or relationships between contacts and other entities in the system.
Key Elements of a Scalable CRM Contact Structure
- Contact ID: A unique identifier for each contact to ensure data integrity.
- Name and Basic Information: Fields for first name, last name, phone number, email, and address.
- Communication History: A log of interactions such as emails, calls, meetings, or support tickets.
- Relationship Data: Information about the contact’s role in the organization or their relationship with the company.
- Tags and Labels: Categories that help organize contacts based on their characteristics or behavior.
- Custom Fields: Fields for additional data that may vary based on business needs.
Schema Example
Field | Type | Description |
---|---|---|
Contact ID | String/UUID | Unique identifier for the contact. |
Name | String | Full name of the contact. |
String | Contact's email address. | |
Phone Number | String | Contact's primary phone number. |
Communication History | Array | List of past interactions. |
Tags | Array | Categories or labels assigned to the contact. |
Important: It's essential to design the data model with future scalability in mind. Adding new data fields or relationships should not require a complete overhaul of the structure.
Best Practices for Structuring Data
- Normalization: Avoid data redundancy by separating information into different tables or collections, ensuring efficient updates and queries.
- Indexing: Use indexes on frequently queried fields like email or phone number to speed up lookups.
- Data Consistency: Ensure that changes to contact data are handled properly to avoid data inconsistencies.
- Modular Design: Break down contact data into modular components, such as personal information, communication logs, and tags, to improve maintainability and extendability.
Integrating APIs to Fetch and Manage Customer Data
In a CRM system, integrating external APIs plays a crucial role in effectively managing and retrieving customer data. By using APIs, data can be pulled from various sources, allowing for a unified view of customer interactions and ensuring accurate and up-to-date information. React, with its efficient handling of state and components, is an ideal choice for integrating these APIs, enabling seamless data flow between the front-end and the back-end.
To integrate APIs in a React-based CRM, the first step is to connect to the relevant endpoints and manage the responses properly. This can be achieved using JavaScript libraries like Axios or the native fetch API, both of which allow easy interaction with RESTful services. The data received from the APIs can then be processed and displayed dynamically within the UI.
Key Steps for API Integration
- Set up the API client to handle requests and responses.
- Use React state management to store and update customer data.
- Display customer data dynamically in various components.
- Handle errors and loading states effectively to improve user experience.
Example of Fetching Customer Data
When integrating an API to fetch customer details, the process involves making a request to the server and updating the React state based on the response. Here is a basic example:
const fetchCustomerData = async () => {
try {
const response = await fetch('https://api.example.com/customers');
const data = await response.json();
setCustomers(data);
} catch (error) {
console.error('Error fetching customer data:', error);
}
}
This function makes a request to the API, parses the returned data, and stores it in the React state.
Managing Customer Data in a Table
Customer Name | Phone | |
---|---|---|
John Doe | [email protected] | (123) 456-7890 |
Jane Smith | [email protected] | (987) 654-3210 |
Important: Ensure proper error handling for API calls to provide feedback if the request fails or the data is not available.
Handling Data Updates
- Trigger updates via API calls when customer data changes.
- Synchronize the front-end state with back-end updates to ensure consistency.
- Utilize forms for editing customer data and make PUT/PATCH requests to update it on the server.
Building Authentication and User Roles in Your CRM
When developing a CRM system, user authentication and access control play a crucial role in ensuring that sensitive data is protected while allowing users to access the appropriate resources. This process typically involves implementing secure login mechanisms, user role assignment, and permission management. React, along with libraries like Firebase or Auth0, can make this process simpler and more efficient, providing built-in tools to handle user authentication.
Additionally, defining user roles is key to establishing a hierarchy of access within the system. For example, an admin may have access to all features, while a regular user can only view and update their own data. Implementing a robust role-based access control (RBAC) system ensures that users have the correct level of access, preventing unauthorized actions.
Authentication Mechanisms
- JWT (JSON Web Token): A popular method for securing APIs, ensuring that each user is authenticated and authorized for a specific action.
- OAuth 2.0: Commonly used for third-party authentication (e.g., Google, Facebook login), it provides a streamlined user experience while ensuring security.
- Session-based Authentication: Storing user session information on the server and linking it to a session ID on the client.
User Role Management
Important: Clearly define the roles and permissions for each user type during the planning phase to avoid unnecessary complexity during implementation.
- Admin: Full access to the CRM, including user management, reporting, and configuration.
- Manager: Can view and edit data within specific parameters but cannot modify user roles or access system-wide settings.
- User: Limited to personal data and basic interaction with the CRM.
Role-based Access Control (RBAC)
Role | Permissions |
---|---|
Admin | Full CRUD operations, user management, settings access |
Manager | View and edit data, generate reports, limited configuration |
User | View personal data, update own information |
Creating Dynamic Dashboards for Enhanced Customer Insights
To effectively manage customer data, building dynamic dashboards in a CRM system allows businesses to visualize key insights and track customer behavior in real-time. These dashboards provide an overview of important metrics, helping teams to stay informed and make data-driven decisions. By leveraging React, developers can create interactive and responsive dashboards that are both user-friendly and visually appealing.
Dynamic dashboards are essential for presenting complex data in an easily digestible format. With React, developers can build components that update automatically as new data comes in, allowing for a seamless user experience. This approach not only enhances user interaction but also enables the real-time tracking of customer engagement, sales performance, and more.
Key Features of Dynamic Dashboards
- Real-time Data Updates: Dashboards reflect live data, ensuring up-to-date information at all times.
- Customizable Widgets: Users can choose and arrange widgets to display the most relevant metrics.
- Data Filtering and Segmentation: Dashboards allow users to filter and segment data based on various parameters.
Types of Visual Elements in Dashboards
- Graphs and Charts: Visual representations of customer data, such as bar graphs and pie charts, make patterns easier to recognize.
- Tables: Tabular data displays offer a detailed breakdown of customer interactions and sales metrics.
- Maps: Geographic visualizations help track customer locations and sales distribution.
Interactive dashboards empower teams to make informed decisions quickly, improving overall customer satisfaction and engagement.
Example of a Data Table in a Dashboard
Customer Name | Last Interaction | Sales Amount | Status |
---|---|---|---|
John Doe | April 6, 2025 | $500 | Active |
Jane Smith | March 30, 2025 | $350 | Inactive |
Robert Johnson | April 2, 2025 | $1200 | Active |
Real-Time Notifications and Alerts Implementation in React
Incorporating real-time notifications in a CRM system is essential for providing users with immediate updates. To achieve this, React offers powerful tools like WebSockets and state management libraries such as Redux or Context API. WebSockets allow for a persistent connection between the client and server, enabling the delivery of notifications as soon as an event occurs on the server side. This ensures that users receive alerts in real-time, improving user experience and enhancing communication.
Setting up a notification system requires a well-structured approach to handle various events, such as incoming messages, task assignments, or status changes. The process involves subscribing to specific events on the backend, capturing these events, and dispatching them to the front end. Using React’s state management, we can trigger UI updates to notify the user about new events.
Steps to Implement Notifications
- Set up a WebSocket connection to listen for real-time updates from the server.
- Integrate state management to store incoming notifications in the global state.
- Display notifications using React components that update dynamically based on state changes.
- Implement notification sounds or visual indicators to alert users effectively.
Example Notification Structure
Notification Type | Description | Action |
---|---|---|
Message | New message from a colleague or client. | Open the message or mark as read. |
Task Update | Task assigned, updated, or completed. | View task details or change status. |
Alert | Important system or user-specific alert. | Take immediate action or dismiss. |
Tip: Use push notifications for critical alerts, especially when the user is inactive in the app.
Testing, Debugging, and Optimizing Your CRM Application
When developing a CRM application with React, it is essential to ensure the stability, efficiency, and overall performance of the system. Testing is a crucial part of the development process as it helps to identify and fix potential issues early on. Debugging involves isolating the root causes of errors and resolving them, while optimization ensures that the application runs smoothly even as it scales with increased usage. Together, these practices contribute to delivering a high-quality product that meets user expectations.
In this section, we will explore strategies to effectively test, debug, and optimize your CRM system. These steps will guide you in maintaining code quality and improving user experience, ensuring your application remains robust and performant in a production environment.
Testing Your CRM Application
Testing plays a pivotal role in the development of any React application. For a CRM, it’s important to cover different types of testing to ensure the integrity of the features and the overall application flow.
- Unit Testing: Test individual components to ensure they function correctly in isolation.
- Integration Testing: Validate the interaction between different components or services within your CRM.
- End-to-End Testing: Test the entire workflow, simulating real user behavior to catch issues in the full application flow.
Debugging Strategies
React provides powerful tools to help developers debug applications efficiently. Some best practices include:
- Use React Developer Tools: Utilize the React Developer Tools extension to inspect components, check the state, and debug re-renders.
- Console Log Statements: Insert log statements in critical areas of the code to understand the flow and identify issues.
- Error Boundaries: Implement error boundaries to catch JavaScript errors and display a fallback UI instead of crashing the application.
Optimizing Performance
Optimizing the performance of your CRM is vital for a smooth user experience. React provides several techniques to improve performance, especially as the application grows.
Optimization Technique | Description |
---|---|
Code Splitting | Split your app into smaller bundles so that only the necessary code is loaded for each page. |
Memoization | Use React.memo and useMemo to prevent unnecessary re-renders of components and expensive computations. |
Lazy Loading | Load components only when they are needed to improve initial load time. |
Tip: Always profile the performance of your CRM using React’s built-in Profiler to identify bottlenecks and areas for improvement.