App Development Software Java
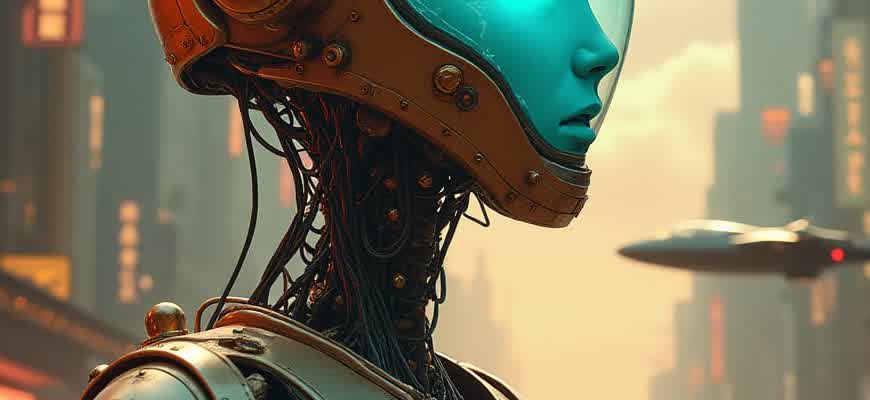
Java remains one of the most widely used programming languages for developing both mobile and web applications. It is known for its versatility, reliability, and cross-platform capabilities. The language is used by developers to create robust, scalable, and secure apps for various platforms, ranging from Android devices to enterprise-level web solutions.
Key features of Java in app development:
- Object-Oriented Structure for better code organization
- Automatic Memory Management via Garbage Collection
- Strong Multithreading Support
- Comprehensive APIs for quick integration of services
Java development tools are essential to streamline the coding process and improve productivity. Here are some of the most commonly used:
- Android Studio
- Eclipse IDE
- IntelliJ IDEA
- NetBeans
Java's "Write Once, Run Anywhere" capability enables developers to create applications that can run on any device, enhancing their reach and scalability.
Tool | Platform | Features |
---|---|---|
Android Studio | Android | Rich UI editor, Android-specific debugging tools |
Eclipse IDE | Cross-platform | Extensive plugin ecosystem, great for large projects |
IntelliJ IDEA | Cross-platform | Smart code completion, integrated version control |
Choosing the Ideal Java Framework for Application Development
When developing Java applications, selecting the right framework is crucial for ensuring scalability, security, and maintainability. With numerous options available, it’s essential to consider the specific needs of your project. A well-chosen framework can significantly reduce development time, improve code quality, and enhance performance. It’s important to evaluate the nature of your app and how the framework supports your development goals.
Different frameworks offer various advantages depending on the complexity of the application. For instance, some frameworks are ideal for large enterprise applications, while others are better suited for lightweight or microservices-based architectures. The choice also depends on factors like team familiarity, community support, and long-term project sustainability.
Key Criteria for Framework Selection
- Project Requirements: Understand the project’s scope and architecture.
- Performance: Assess whether the framework can handle expected load and speed requirements.
- Scalability: Ensure the framework can support future growth in terms of features and traffic.
- Security: Consider built-in security features such as authentication and encryption.
- Community and Support: A robust community can help with troubleshooting and continuous updates.
Popular Java Frameworks for App Development
- Spring Framework: Well-suited for enterprise applications, offering flexibility and scalability.
- Hibernate: Ideal for database management, simplifies object-relational mapping (ORM).
- JavaServer Faces (JSF): Focuses on simplifying UI development and integrates well with other Java technologies.
- Play Framework: A lightweight option for developing scalable web applications with a focus on speed.
Important: Always assess the specific needs of your application before settling on a framework. Even though a popular framework like Spring is widely used, it may not be the best choice for a small, microservice-based application.
Comparing Java Frameworks
Framework | Primary Use | Strengths | Weaknesses |
---|---|---|---|
Spring | Enterprise, Web Apps | Flexibility, wide community | Steep learning curve |
Hibernate | Database ORM | Simplifies database interactions | Can be slow for simple queries |
JSF | UI Development | Built-in components, good for Java EE apps | Limited outside Java EE environments |
Play | Web Apps, Microservices | Asynchronous, easy-to-use | Smaller ecosystem |
Key Features of Java Development Software for Mobile Applications
When developing mobile applications using Java, developers often rely on specialized tools and software that offer specific features designed to streamline the app creation process. These tools not only enhance productivity but also ensure that applications are efficient, responsive, and compatible with various mobile platforms. Understanding the key features of Java development software is essential for developers aiming to create high-performance mobile applications.
Java development software offers a range of functionalities that are indispensable for mobile app development. These tools provide support for both Android and cross-platform app development, allowing developers to efficiently write, test, and deploy mobile apps. Here are the key features to consider when choosing Java development tools for mobile applications:
Key Features
- Cross-Platform Development: Java-based tools allow for the creation of applications that run on both Android and iOS with minimal changes to the code.
- Code Optimization Tools: These features help developers improve the performance of the application by detecting bottlenecks and providing optimization suggestions.
- Real-time Debugging and Testing: Java development environments provide powerful debugging tools that allow real-time testing and fixing of issues, ensuring smooth performance across devices.
- Integration with External APIs: Java development platforms support the integration of third-party services and APIs, adding functionality such as payments, social media sharing, and data synchronization.
- Robust Libraries and Frameworks: Java tools often come with extensive libraries and frameworks, including Android SDK and JavaFX, for efficient app development.
Real-time testing and debugging can significantly reduce the development time and improve the overall user experience by ensuring that the app functions seamlessly across various devices.
Benefits of Using Java Development Software for Mobile Apps
Feature | Benefit |
---|---|
Cross-Platform Compatibility | Helps developers write code once and deploy it on multiple platforms, reducing time and costs. |
Efficient Debugging Tools | Speeds up the development process by allowing real-time issue resolution. |
API Integration | Expands the functionality of mobile apps by easily connecting them with third-party services. |
Integrating APIs with Java Development Tools
When developing Java applications, incorporating external data and services through APIs is a common and essential task. APIs allow developers to extend their applications with additional functionality, such as accessing third-party services, databases, or web platforms. Integrating these APIs into Java projects involves using appropriate libraries, tools, and understanding how to handle requests and responses efficiently. In this process, developers rely on tools like IntelliJ IDEA, Eclipse, and NetBeans to simplify the integration tasks and manage dependencies.
Java offers a range of libraries and frameworks to streamline API integration. Libraries such as Apache HttpClient, OkHttp, and Spring RestTemplate provide convenient ways to send HTTP requests, manage authentication, and process responses. These tools also support modern features like asynchronous processing, error handling, and integration with JSON or XML data formats. Understanding how to use these libraries is key to seamless API communication and enhancing the overall development workflow.
Steps to Integrate an API in Java
- Choose the Right Library: Start by selecting a suitable library that will handle HTTP requests. Common choices include:
- Apache HttpClient
- OkHttp
- Spring RestTemplate
- Set Up Dependencies: Use build tools like Maven or Gradle to include the necessary libraries in your project. This step ensures that all dependencies are correctly resolved and accessible.
For Maven, add the following dependency to the pom.xml file:
<dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>4.5.13</version> </dependency>
- Send API Requests: Once the setup is complete, create methods to send requests to the API and handle responses.
Example of sending a GET request using HttpClient:
CloseableHttpClient client = HttpClients.createDefault(); HttpGet request = new HttpGet("https://api.example.com/data"); CloseableHttpResponse response = client.execute(request);
- Process Responses: After receiving the API response, parse and handle the data appropriately. This often involves converting JSON or XML data into Java objects.
- Use libraries like Jackson or Gson for JSON parsing.
- For XML, consider using JAXB or DOM parsing methods.
API Integration Example in Java
Step | Action | Tools/Libraries |
---|---|---|
1 | Set up API request | Apache HttpClient, OkHttp |
2 | Send request to the API endpoint | HttpGet, HttpPost, or RestTemplate |
3 | Parse the response | Jackson, Gson, JAXB |
Optimizing Mobile App Performance with Java Development Tools
When developing mobile applications using Java, optimizing performance is a critical aspect to ensure that the app runs smoothly and efficiently. Java development platforms offer a range of tools and techniques that help address performance challenges by reducing memory consumption, enhancing responsiveness, and improving battery life. By leveraging the capabilities of these platforms, developers can build faster and more scalable applications.
Effective optimization requires a deep understanding of the development environment and how Java handles key operations, such as memory management and threading. Developers must identify potential bottlenecks and apply best practices tailored to the Java ecosystem. The use of robust frameworks and libraries available on Java development platforms can significantly reduce development time and enhance performance.
Key Techniques for Optimizing Java-Based Mobile Applications
- Memory Management – Optimize garbage collection and avoid memory leaks.
- Thread Management – Efficiently handle multi-threading to avoid blocking and improve responsiveness.
- UI/UX Optimization – Minimize unnecessary UI updates and leverage hardware acceleration when possible.
- Code Optimization – Use profiling tools to identify inefficient code and refactor where necessary.
Important Tip: Use Java’s built-in tools like Android Profiler or VisualVM to monitor resource consumption and pinpoint performance issues.
Tools for Performance Enhancement in Java
Tool | Purpose |
---|---|
Android Studio Profiler | Monitors CPU, memory, and network usage to optimize app performance. |
NetBeans | Analyzes code performance, detects memory leaks, and provides code suggestions. |
Eclim | Improves Java code execution time in real-time by integrating with Eclipse IDE. |
Note: Always prioritize optimizing for the most commonly used features in your app to achieve a tangible performance boost for end-users.
Testing Java Mobile Applications with Popular Tools
When developing Java-based mobile applications, thorough testing is crucial to ensure functionality, performance, and user experience. A variety of testing tools can help automate and streamline the process, allowing developers to detect bugs and optimize performance across different devices. Using the right testing tools not only saves time but also increases the overall quality of the app. Below are some widely used tools for testing Java mobile apps.
Testing Java mobile applications can be approached from multiple angles: functional testing, performance testing, and UI testing. Each type of testing requires specific tools and methods, ranging from unit testing to end-to-end system tests. Let’s explore some of the most popular testing frameworks and tools for Java-based mobile app development.
Key Testing Tools for Java Mobile Apps
- JUnit: A widely used framework for unit testing in Java. It allows developers to write repeatable tests, ensuring that individual units of code work as expected.
- Appium: A cross-platform tool for automating mobile apps. It supports both Android and iOS and provides testing capabilities for native, hybrid, and mobile web apps.
- Espresso: An Android-specific testing framework that helps automate UI testing by simulating user interactions with the app on the device.
- UIAutomator: Google’s testing tool for Android, focused on UI testing across multiple apps and user interactions.
Popular Testing Approaches
- Unit Testing: Focuses on individual components or functions of the app to ensure that each part works independently. JUnit is commonly used here.
- Integration Testing: Verifies that different components of the app interact as expected, including database connections and API interactions.
- UI Testing: Ensures that the user interface responds appropriately to user inputs and interactions. Espresso and UIAutomator are frequently used in this area.
- Performance Testing: Assesses the app’s stability and speed, helping to identify potential bottlenecks or crashes under different conditions.
Tip: When testing Java mobile apps, it's essential to test on a variety of real devices, as emulators do not always simulate real-world performance accurately.
Comparing Testing Tools
Tool | Platform | Primary Use | Features |
---|---|---|---|
JUnit | Android & Java | Unit Testing | Easy to integrate, supports test automation, widely adopted in Java |
Appium | Android & iOS | Cross-platform Testing | Supports multiple languages, cloud testing integration, real device testing |
Espresso | Android | UI Testing | Fast execution, easy setup, great for UI interaction testing |
UIAutomator | Android | UI Testing | Tests across apps, interacts with system apps, integrates with Android Studio |
Deploying Java-Based Mobile Apps to App Stores
Deploying a Java-based mobile application to the app stores requires a series of critical steps to ensure its smooth launch and availability to users. Whether you're aiming for the Google Play Store or the Apple App Store, the process involves preparing your app for distribution, meeting specific guidelines, and testing for compatibility with the target devices.
Before submitting your app, it's important to understand the unique requirements and submission guidelines for each platform. The following steps outline the key actions you need to take in order to deploy your Java-based mobile application to app stores successfully.
Key Steps for Deployment
- Prepare the App for Distribution: Ensure the app is fully functional and has passed all necessary tests. This includes testing the app for bugs, performance issues, and security vulnerabilities.
- Build a Release Version: Generate the release version of your Java-based application using appropriate tools like Gradle or Maven for Android or Xcode for iOS.
- Create Store Listings: Prepare promotional material such as screenshots, descriptions, and keywords to optimize app visibility in the stores.
- Set Up Developer Accounts: Create and configure developer accounts on the respective platforms (Google Play Console for Android, App Store Connect for iOS).
App Store Submission Process
- Google Play Store:
- Sign in to your Google Play Console account.
- Upload the APK or AAB file.
- Fill out the necessary app details (name, description, category, etc.).
- Submit the app for review and wait for approval.
- Apple App Store:
- Sign in to App Store Connect.
- Upload the IPA file via Xcode.
- Provide necessary app details and metadata.
- Submit the app for review and approval.
Important Considerations
Make sure your app complies with all platform-specific policies and requirements before submission to avoid rejection.
Platform | File Format | Developer Account |
---|---|---|
Google Play Store | APK / AAB | Google Play Console |
Apple App Store | IPA | App Store Connect |
Security Considerations for Java-Based Application Development
When developing Java applications, securing the software throughout its lifecycle is crucial to protect sensitive data and ensure the integrity of the application. Java's widespread usage in enterprise environments makes it a prime target for potential security threats, requiring developers to adopt best practices and take proactive measures. The focus should be on securing both the development environment and the deployed application.
To achieve a secure Java application, developers must consider various aspects, including secure coding techniques, secure communication, and vulnerability management. This requires constant awareness of emerging threats and the implementation of defensive mechanisms to safeguard against attacks.
Key Security Measures in Java Application Development
- Input Validation – Ensure that all user inputs are validated to prevent injection attacks (e.g., SQL injection, XML injection). Use robust libraries like Java’s PreparedStatement to mitigate risks.
- Authentication and Authorization – Implement strong authentication mechanisms (e.g., OAuth, JWT) and enforce role-based access control (RBAC) to limit access to sensitive data.
- Encryption – Encrypt sensitive data both in transit and at rest using industry-standard algorithms like AES and RSA.
- Exception Handling – Avoid exposing stack traces to users, as they can provide attackers with valuable information. Properly handle exceptions and log errors securely.
Important Security Best Practices
“Never trust user input, always validate and sanitize it thoroughly.”
- Use Java’s built-in security features, such as Java Security Manager and JCE (Java Cryptography Extension).
- Keep third-party libraries and dependencies updated to minimize vulnerabilities.
- Enable secure coding guidelines such as the OWASP Top 10 when designing the application architecture.
- Regularly perform penetration testing and code reviews to identify potential vulnerabilities.
Common Vulnerabilities in Java Applications
Vulnerability | Risk | Mitigation |
---|---|---|
Cross-Site Scripting (XSS) | Injection of malicious scripts into web applications. | Use output encoding and proper input sanitization. |
SQL Injection | Unauthorized database access or data manipulation. | Use PreparedStatement and avoid dynamic SQL queries. |
Insecure Deserialization | Execution of malicious code during deserialization. | Validate and sanitize data before deserializing, use safe libraries. |
Maintaining and Updating Java Apps After Release
After deploying a Java-based application, the work doesn't end. It is essential to focus on keeping the app secure, functional, and up-to-date. This involves ongoing support, addressing bugs, implementing new features, and ensuring compatibility with updated Java versions. Failure to properly maintain your app can lead to performance issues and security vulnerabilities, which may negatively affect the user experience and your app's reputation.
Effective post-launch maintenance includes monitoring the app’s performance, troubleshooting errors, and releasing updates regularly. It is crucial to have a structured process for handling feedback and introducing improvements. Below is a detailed approach to post-launch app maintenance.
Key Aspects of Java App Maintenance
- Bug Fixes: Regularly check for bugs reported by users or identified in error logs. Implement patches as soon as possible to maintain stability.
- Performance Optimization: Continuously monitor app performance. Refactor code to ensure it runs efficiently, and address any scalability issues.
- Security Updates: Keep the app secure by patching vulnerabilities as they arise. Update dependencies to the latest versions and apply security best practices.
- Feature Enhancements: Plan and implement new features to keep the app relevant and competitive in the market.
Best Practices for Post-Launch Updates
- Monitor User Feedback: Actively listen to users and prioritize improvements based on their feedback. This helps in refining user experience.
- Automate Testing: Implement automated testing to catch potential issues before they affect the end users. This ensures faster, more reliable updates.
- Version Control: Use version control systems to manage code changes effectively, ensuring smooth collaboration and rollback options when needed.
Common Tools for Maintenance
Tool | Purpose |
---|---|
Jenkins | Automates the build and deployment process, ensuring continuous integration and delivery. |
SonarQube | Helps analyze code quality, identify bugs, and maintain coding standards throughout the development lifecycle. |
JProfiler | Performance profiling tool to monitor Java applications and optimize resource usage. |
Effective maintenance ensures that your Java application remains secure, fast, and aligned with users' needs, which ultimately contributes to its long-term success.