Android App Development Tutorial 2024
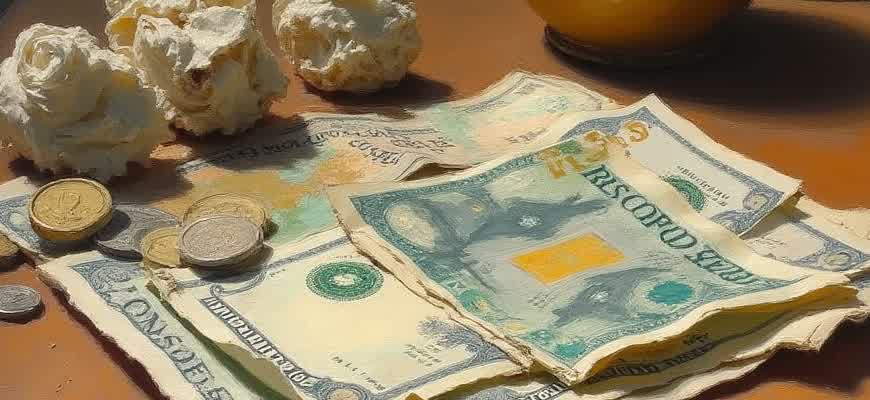
Building Android applications has evolved rapidly, with new tools and frameworks making the process faster and more efficient. In 2024, developers have access to a range of modern libraries and SDKs that help streamline app creation and improve performance. Whether you're a beginner or an experienced developer, understanding the core concepts is crucial for success in the Android ecosystem.
Here is a brief overview of the essential tools you will need to get started:
- Android Studio: The official IDE for Android development, offering a robust suite of features for building, testing, and debugging apps.
- Java/Kotlin: Kotlin is now the preferred language for Android development, offering cleaner syntax and improved productivity compared to Java.
- SDK Tools: The Android Software Development Kit provides the necessary components to create apps, including libraries and emulator tools.
Key Steps to Follow:
- Set up Android Studio and configure the development environment.
- Create a new project with appropriate settings (language, target API level, etc.).
- Design the user interface using XML or Jetpack Compose.
- Write business logic and handle app behavior in Kotlin or Java.
- Test the app on a physical or virtual device.
- Publish the app on the Google Play Store once satisfied with its performance.
Note: Android development in 2024 emphasizes the use of Jetpack libraries, which provide pre-built solutions for common app functionalities, improving code efficiency and maintainability.
Here is a table outlining the major Android development tools:
Tool | Purpose |
---|---|
Android Studio | Integrated Development Environment (IDE) for coding, debugging, and testing. |
Kotlin | Preferred programming language for Android, offering concise syntax and enhanced features. |
Jetpack | Set of libraries to help developers avoid boilerplate code and implement best practices. |
Configuring Android Studio for Your First Application
To begin developing your first Android application, it's crucial to properly set up Android Studio. This IDE (Integrated Development Environment) offers all the necessary tools to create, test, and debug Android apps. Before you start writing code, you'll need to ensure your system is prepared with the right installations.
Follow these steps to configure Android Studio and get it ready for your first app development:
Steps to Install and Set Up Android Studio
- Download the latest version of Android Studio from the official site.
- Run the installer and follow the on-screen instructions to complete the installation.
- Launch Android Studio and let it download any necessary SDK components.
- Once set up, open Android Studio and complete the first-time configuration by selecting the appropriate system settings.
Setting Up the Development Environment
- Ensure that your system meets the minimum hardware and software requirements for Android Studio.
- Install the latest version of the Android SDK (Software Development Kit).
- Enable the use of virtual devices (AVD) for testing, or connect a physical device for real-world debugging.
- Configure the correct project structure within Android Studio (choose an appropriate template and programming language such as Java or Kotlin).
Tip: It's highly recommended to use the latest stable version of Android Studio to avoid compatibility issues.
Useful Tools in Android Studio
Tool | Description |
---|---|
Emulator | A virtual device that simulates Android devices on your computer for testing. |
Logcat | Shows system messages, including stack traces and log output, helpful for debugging. |
Android Device Monitor | A comprehensive tool to inspect your app’s performance and track various system stats. |
Understanding the Structure and Files in an Android Project
When developing an Android application, it’s important to understand the structure of the project and the role of each file within it. The Android project is organized in a way that helps developers manage resources, code, and configurations efficiently. It also allows for seamless integration with Android Studio, ensuring that the development process is streamlined and manageable. Below is an overview of the key components and directories you will encounter in an Android project.
The structure of an Android project includes various folders and files, each serving a specific purpose. The most important folders include src, res, and manifests. These directories contain the core elements of the app, such as source code, resources (images, layouts, strings), and configuration files. Understanding where each type of file is located and what it is responsible for is crucial for effective app development.
Key Folders and Files
- src/ - This folder contains the Java or Kotlin code for the application. It is divided into packages based on functionality.
- res/ - This directory holds all the resources for the app, such as images, layout files, strings, and colors.
- manifests/ - The AndroidManifest.xml file is located here, and it contains important configuration details, such as app permissions and activities.
- build.gradle - This file is used for build configurations and dependencies. It defines the version of the app and libraries used during development.
- libs/ - Holds external libraries used by the app.
Important: The structure of the Android project might vary slightly depending on whether you are using Java or Kotlin, but the core components remain consistent.
File Overview
File/Folder | Description |
---|---|
AndroidManifest.xml | Contains the app's metadata, permissions, and main activity declarations. |
build.gradle | Manages project dependencies, SDK versions, and build configurations. |
res/ | Stores resources like layouts, images, strings, and other UI elements. |
src/ | Contains Java or Kotlin code, organized into packages. |
Note: The res/ folder is further divided into subfolders such as drawable/, layout/, values/, and others for better organization of resources.
Building Your First Interface with XML Layouts
Designing a user interface (UI) for an Android app involves creating layouts using XML files. These layouts define the arrangement of UI components such as buttons, text fields, and images. XML is a markup language that provides a straightforward and structured way to build UI elements. In this section, we will cover the basics of creating a simple XML layout and how to implement it within an Android app.
When you begin developing a UI, you work with different types of layout managers, such as LinearLayout, RelativeLayout, and ConstraintLayout. These layouts help organize how elements are displayed on the screen. To start, you'll need to define the layout structure in an XML file located in the res/layout directory of your project.
Basic Structure of XML Layout
Here’s an example of a basic XML layout file:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Welcome to My App" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
</LinearLayout>
This layout contains a TextView and a Button, arranged vertically using LinearLayout.
Essential Layout Components
- TextView: Displays text on the screen.
- Button: Provides a clickable interface element.
- ImageView: Displays images.
- EditText: Accepts user input.
These basic components can be arranged and customized according to your app’s needs.
Important Notes
Make sure to always set layout_width and layout_height attributes for every UI element. Common values include match_parent and wrap_content.
For complex UIs, you may want to organize elements using RelativeLayout or ConstraintLayout, which offer more flexibility compared to LinearLayout.
XML Layout Example Table
Component | Attribute | Explanation |
---|---|---|
TextView | android:text | Sets the text displayed in the TextView. |
Button | android:onClick | Specifies the method to be called when the button is clicked. |
Handling User Inputs in Android with Java and Kotlin
Processing user input efficiently is crucial for creating interactive and responsive Android applications. Whether you are using Java or Kotlin, both languages offer various tools and methods for handling different types of user interactions, such as touch events, text inputs, and button clicks. Understanding how to manage these inputs ensures that your app provides a seamless experience.
In Android development, user inputs are typically captured through UI elements like buttons, text fields, checkboxes, and radio buttons. Both Java and Kotlin provide similar functionalities for interacting with these elements, though Kotlin tends to offer a more concise syntax. This guide will walk you through the basic techniques for handling user inputs in both languages.
Handling Button Clicks
Buttons are one of the most common UI elements for triggering actions. Both Java and Kotlin allow you to set listeners to detect when a button is clicked.
Java:
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Handle button click
}
});
Kotlin:
val button: Button = findViewById(R.id.button)
button.setOnClickListener {
// Handle button click
}
Working with Text Inputs
Handling user input from text fields is essential for gathering information. Both Java and Kotlin offer methods for extracting data from EditText elements.
Java:
EditText editText = findViewById(R.id.editText);
String userInput = editText.getText().toString();
Kotlin:
val editText: EditText = findViewById(R.id.editText)
val userInput = editText.text.toString()
Checkboxes and Radio Buttons
To capture user choices, CheckBox and RadioButton are commonly used. Below is an example of how you can check if a box is checked.
Java:
CheckBox checkBox = findViewById(R.id.checkBox);
boolean isChecked = checkBox.isChecked();
Kotlin:
val checkBox: CheckBox = findViewById(R.id.checkBox)
val isChecked = checkBox.isChecked
Table of Common Input Methods
Input Type | Java Code | Kotlin Code |
---|---|---|
Button Click |
|
|
Text Input |
|
|
Checkbox State |
|
|
Remember, while Kotlin is more concise, Java can still offer more flexibility in certain scenarios. Both languages are powerful tools for managing user input in Android applications.
Integrating External Services and APIs in Android Apps
Modern Android applications often require communication with external servers or services to enhance functionality. This is typically achieved by integrating APIs (Application Programming Interfaces) and web services into the app. APIs provide the means for the app to send and receive data from remote servers, allowing it to display dynamic content, authenticate users, and access additional features like maps, social media, or weather data.
Integrating external APIs involves handling HTTP requests, processing JSON or XML responses, and ensuring smooth communication between the app and the server. With Android, developers can leverage libraries like Retrofit, Volley, or the native HTTPURLConnection to simplify API interactions. It's crucial to implement efficient error handling and security measures to protect user data and enhance the user experience.
Steps for Integrating an API
- Choose the appropriate API based on app requirements (e.g., weather, user authentication, etc.).
- Obtain the API key or credentials if needed for access.
- Integrate a library like Retrofit or Volley to handle API requests.
- Set up asynchronous requests to avoid blocking the main UI thread.
- Parse the API response (typically in JSON or XML format) and display the data in the app.
Common Techniques for API Communication
- GET requests: Used to fetch data from the server, often for displaying lists or data from external sources.
- POST requests: Used for sending data to the server, such as submitting form data or authenticating a user.
- PUT/PATCH requests: Used to update existing resources on the server.
- DELETE requests: Used to delete data or resources from the server.
Important Considerations
Aspect | Consideration |
---|---|
Security | Ensure sensitive data is encrypted using HTTPS and implement proper authentication mechanisms. |
Rate Limiting | Check the API documentation for rate limits to avoid hitting usage caps. |
Handling Errors | Implement error handling to gracefully manage failed requests, timeouts, and invalid responses. |
Tip: Always handle API responses on a background thread to avoid UI freezing and ensure a smooth user experience.
Effective Debugging of Android Apps with Android Studio
Debugging is a crucial step in the development process of any Android application. Android Studio provides a variety of tools to identify and resolve issues that may arise during development. These tools allow developers to track performance, identify bugs, and optimize the code in real time, making the debugging process efficient and manageable. By leveraging these tools, you can pinpoint the root causes of errors, test different scenarios, and ensure a smoother user experience.
In this tutorial, we will explore key debugging features in Android Studio, focusing on features such as Logcat, breakpoints, and the Android Debug Bridge (ADB). Understanding how to effectively use these tools will help streamline the development workflow and improve app performance significantly.
Key Debugging Tools in Android Studio
- Logcat: This is one of the most powerful tools for tracking real-time logs and error messages. You can filter and search logs to trace specific issues, view error stack traces, and gain detailed insights into your app's behavior.
- Breakpoints: Breakpoints allow you to pause the execution of your app at specific lines of code. This helps to examine variables, check the flow of execution, and step through your code line by line.
- Android Debug Bridge (ADB): ADB lets you communicate directly with an Android device or emulator, perform commands, and analyze app behavior without needing to run the entire app each time.
Steps to Debug Using Android Studio
- Setting up Breakpoints: To set a breakpoint, simply click in the left margin of the code editor next to the line where you want the app to pause. Once set, you can run your app in debug mode and Android Studio will stop execution at the breakpoint.
- Using Logcat: Open the Logcat window from the bottom toolbar. Select the desired device and filter the log messages by severity or tag. This will help you focus on specific errors or information.
- Inspecting Variables: While the app is paused at a breakpoint, use the "Variables" window to inspect the values of variables. This helps identify incorrect values that might be causing bugs.
- Utilizing ADB: Run commands like "adb logcat" to see the logs in real time from your terminal, or "adb shell" to access the device’s command shell for advanced debugging tasks.
Important Tips for Effective Debugging
Tip | Description |
---|---|
Use conditional breakpoints | Conditional breakpoints pause execution only when certain conditions are met, saving time during the debugging process. |
Leverage the Profiler | The Android Studio Profiler helps track memory usage, CPU usage, and network requests to pinpoint performance bottlenecks. |
Use Remote Debugging | If the issue is device-specific, remote debugging allows you to inspect and debug apps running on real devices. |
Remember, debugging is an iterative process. Regularly test your code, analyze logs, and use Android Studio’s powerful tools to identify and resolve issues promptly.
Testing Your Android Application on Real Devices and Emulators
When developing an Android application, it is essential to ensure that it works smoothly on a variety of devices and configurations. Testing on both real devices and emulators provides valuable insights into performance, user experience, and compatibility. Each method has its advantages and is crucial in identifying potential issues before releasing the app to the public.
Real devices offer a more accurate representation of how your app will perform in the hands of actual users, considering factors like hardware capabilities, screen size, and user interactions. On the other hand, emulators are convenient and provide a controlled environment for testing multiple Android versions and configurations without needing a physical device for each one.
Testing on Real Devices
Testing on physical devices is one of the most reliable ways to assess the performance and functionality of your Android app. Here are the main advantages:
- Authentic User Experience: Real devices simulate actual usage scenarios, including varying network conditions, battery levels, and hardware-specific behavior.
- Hardware-Specific Testing: Devices offer specific features like cameras, sensors, or GPS that emulators may not accurately reproduce.
- Performance Metrics: Testing on real devices helps you gauge performance in real-world conditions, such as device limitations on memory and processing power.
Testing on Emulators
Emulators are a useful tool for simulating different Android environments without requiring multiple physical devices. They offer flexibility and are highly customizable:
- Multi-Device Testing: Emulators allow testing on a variety of screen sizes, resolutions, and Android versions.
- Convenience: You don’t need physical devices for each configuration, which saves space and resources.
- Simulating Different Network Conditions: Emulators allow you to simulate different network speeds, ensuring that your app performs well even under adverse conditions.
Key Differences Between Real Devices and Emulators
Feature | Real Devices | Emulators |
---|---|---|
Performance | Realistic performance, with real-world limitations | Can be slower than real devices, depending on the system |
Hardware Access | Full access to hardware features (camera, sensors, etc.) | Limited hardware access, can simulate some features |
Convenience | Requires physical device for each configuration | Multiple configurations available without physical devices |
Network Simulation | Real network conditions | Can simulate various network speeds and conditions |
Important Note: Testing on both real devices and emulators is recommended for a thorough evaluation of your app's performance and compatibility.