Build Real Time Web App
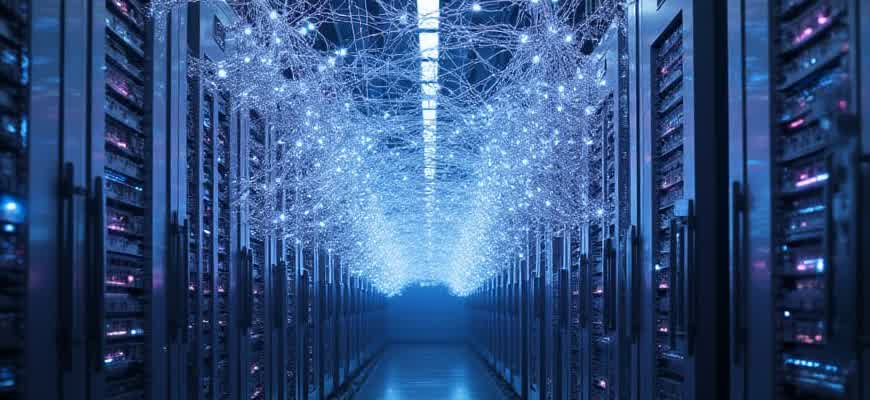
Real-time web applications allow users to interact with content instantly, with updates happening in the background without the need for manual refresh. These apps are crucial in fields like messaging, gaming, and live data feeds.
To develop a functional real-time web app, developers need to focus on the following key technologies:
- WebSockets for two-way communication
- Server-sent events (SSE) for one-way updates from server to client
- Long polling for maintaining a persistent connection in environments where WebSockets are unavailable
Key Steps:
- Set up a server capable of handling real-time connections (e.g., Node.js with Socket.io)
- Implement client-side JavaScript to manage incoming and outgoing messages
- Optimize server performance to handle multiple simultaneous connections
Real-time web apps require constant data flow between the server and client, ensuring seamless user interaction without delays.
Data Flow Example:
Component | Function |
---|---|
Client | Sends requests for updates and processes data received in real-time |
Server | Handles incoming connections, processes requests, and pushes updates to clients |
Building Real-Time Web Applications: A Step-by-Step Approach
Real-time web applications are designed to interact with users instantly, providing immediate updates or feedback without requiring a page refresh. To develop such applications, you'll need to implement mechanisms that allow continuous, bidirectional communication between clients and servers. This guide will walk you through key components and technologies that power real-time apps.
Real-time features are often powered by technologies such as WebSockets, Server-Sent Events (SSE), or long polling. Choosing the right protocol is crucial depending on your app's needs, the amount of data being transmitted, and the expected user interaction.
Key Components of Real-Time Web Applications
- WebSockets: A communication protocol that provides full-duplex communication channels over a single, long-lived connection.
- Server-Sent Events (SSE): A simpler alternative to WebSockets, used for server-to-client one-way communication.
- Push Notifications: Sending updates from the server to clients even when the application is not open, commonly used in mobile apps.
- Real-time Databases: Databases that allow changes to be reflected in real time, such as Firebase or MongoDB with change streams.
Steps to Develop a Real-Time Web App
- Set up the server: Choose your server framework (Node.js, Python with Flask/Django, etc.) and ensure it supports WebSockets or SSE for real-time data exchange.
- Implement real-time communication: Use libraries like Socket.io (for WebSockets) or EventSource (for SSE) to enable bidirectional or server-to-client communication.
- Use a real-time database: If your app requires live data syncing (like in chat apps or collaborative tools), integrate a real-time database such as Firebase or MongoDB.
- Deploy and scale: Ensure your infrastructure can handle multiple concurrent users by using load balancing and cloud services such as AWS or Azure for scalability.
Considerations for Optimizing Real-Time Web Apps
Consideration | Solution |
---|---|
Latency | Optimize server and network speed, use CDN for faster content delivery. |
Scalability | Use cloud-based solutions, containerization (Docker), and horizontal scaling. |
Security | Implement encryption (TLS), secure WebSocket connections, and user authentication. |
Real-time apps are challenging but incredibly rewarding. The best results come when your architecture is well-designed for scalability, security, and performance.
Choosing the Ideal Technology Stack for Real-Time Web Applications
When developing real-time applications, choosing the right technology stack is crucial for ensuring performance, scalability, and seamless user experience. Real-time systems demand low latency, high availability, and the ability to manage large volumes of concurrent connections. The stack should be chosen based on the nature of the application, whether it's chat, live streaming, or collaborative tools.
Several key factors influence the decision-making process, such as programming languages, frameworks, databases, and communication protocols. Each component of the stack needs to be carefully evaluated for its suitability in handling real-time data flow and maintaining smooth interaction between the client and server.
Core Components to Consider
- Server-Side Technology: A fast and scalable server environment is essential. Node.js is popular for its non-blocking, event-driven architecture, perfect for handling real-time interactions.
- Client-Side Frameworks: Front-end frameworks like React or Vue.js are great choices due to their ability to quickly update the UI without refreshing the page.
- Database: NoSQL databases like MongoDB and Redis offer flexibility in managing real-time data with low-latency operations, essential for quick read/write cycles.
- WebSockets: WebSockets provide a persistent, bidirectional communication channel between client and server, making it an ideal protocol for real-time applications.
Popular Tech Stacks
Technology | Use Case |
---|---|
Node.js + Socket.io | Real-time chat applications, messaging systems |
React + Redux + WebSockets | Interactive web applications with live updates |
Vue.js + Firebase | Real-time collaboration tools, real-time dashboards |
Tip: When choosing a stack, always prioritize technologies that allow for asynchronous communication and high throughput to handle multiple simultaneous connections.
Setting Up WebSockets for Bidirectional Communication
WebSockets provide a full-duplex communication channel over a single TCP connection, allowing for efficient, real-time data exchange between the client and server. Unlike traditional HTTP requests, which are client-initiated, WebSockets enable both the client and server to send data at any time, offering low-latency interaction ideal for applications like messaging, gaming, and live updates.
To establish a WebSocket connection, both the server and client need to implement specific protocols. WebSockets operate on top of the HTTP protocol and require an initial handshake, followed by an upgrade to the WebSocket protocol for continuous bidirectional communication.
Setting Up the Server
On the server side, you need to choose a WebSocket library that supports the protocol. Below is a basic example for setting up a WebSocket server using Node.js and the `ws` library:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
console.log('received: %s', message);
});
ws.send('Hello from server');
});
Configuring the Client
On the client side, creating a WebSocket connection is straightforward using JavaScript. The following example shows how to establish a connection and send/receive messages:
const socket = new WebSocket('ws://localhost:8080');
socket.onopen = () => {
socket.send('Hello from client');
};
socket.onmessage = (event) => {
console.log('Message from server: ', event.data);
};
Key Considerations
- Connection Management: WebSockets remain open until explicitly closed, so proper handling of connection establishment, message exchange, and closure is essential for preventing memory leaks.
- Security: Ensure that WebSocket communication is encrypted using `wss://` for secure connections.
- Error Handling: Implement error events to handle unexpected disconnections and retries.
Possible Challenges
WebSocket connections are not ideal for all use cases, particularly in environments with strict firewalls or proxies that block non-HTTP traffic.
Performance Considerations
WebSocket offers significant performance benefits for applications requiring continuous communication. For large-scale systems, consider load balancing and clustering strategies to handle multiple concurrent WebSocket connections efficiently.
Feature | HTTP | WebSocket |
---|---|---|
Communication Direction | Client to Server | Bidirectional |
Connection Type | Request-Response | Persistent |
Protocol | HTTP/HTTPS | WebSocket (ws/wss) |
Handling Real-Time Data Synchronization Across Multiple Clients
When developing real-time web applications, ensuring that data is consistently synchronized across multiple users or devices is a critical challenge. Real-time synchronization involves updating data on all connected clients immediately after any change is made, ensuring seamless interaction between users. This task requires efficient data transfer protocols, low-latency communication, and strategies for resolving conflicts when multiple clients attempt to update the same data simultaneously.
To achieve smooth synchronization, developers often rely on technologies such as WebSockets, server-sent events, and specialized frameworks like Firebase or Socket.io. These tools provide bi-directional communication channels, allowing the server to push updates to clients instantly without the need for the clients to continuously request data. However, keeping data consistent and conflict-free is a crucial consideration when building scalable real-time systems.
Key Strategies for Synchronizing Data in Real-Time
- Event-Driven Architecture: Using an event-driven approach helps propagate changes to multiple clients. Clients subscribe to specific data streams and automatically update when an event occurs.
- Versioning and Timestamps: Implementing versioning systems or using timestamps ensures that the most recent changes are applied correctly, helping to avoid conflicts when two users update the same data.
- Conflict Resolution: Techniques like operational transformation (OT) or conflict-free replicated data types (CRDTs) are commonly used to resolve simultaneous changes made by different clients without losing data integrity.
Common Technologies for Real-Time Synchronization
- WebSockets: A protocol that enables two-way communication over a single, long-lived connection, perfect for real-time applications.
- Server-Sent Events (SSE): An efficient way to push data from server to client, where the client maintains an open connection to receive updates.
- Firebase Realtime Database: A cloud-hosted NoSQL database designed for real-time data synchronization with automatic conflict resolution mechanisms.
Important: Real-time synchronization must consider data consistency, reliability, and network issues to ensure the best user experience, especially in high-traffic applications.
Data Synchronization Strategies Comparison
Technology | Pros | Cons |
---|---|---|
WebSockets | Low latency, full-duplex communication, widely supported | Requires persistent connections, complexity in managing connections |
Server-Sent Events | Simple, one-way communication, ideal for live updates | Limited to server-to-client communication, no client-to-server |
Firebase | Automatic synchronization, built-in conflict resolution | Dependency on third-party service, limited control over infrastructure |
Optimizing Server Infrastructure for Low Latency Connections
In a real-time web application, achieving low latency is critical for providing a seamless user experience. Server infrastructure plays a vital role in reducing the delay between user interactions and server responses. By focusing on strategic configurations and performance-enhancing techniques, developers can ensure the application delivers fast and reliable responses across various network conditions.
To minimize latency, it is essential to carefully evaluate and optimize key aspects of the server infrastructure, such as network configuration, server location, and load balancing mechanisms. Below are several strategies to help reduce latency in a real-time web application.
Key Optimization Strategies
- Edge Computing Deployment: Utilize edge servers closer to end-users to reduce the physical distance data needs to travel, improving response times.
- TCP Optimizations: Fine-tune the Transmission Control Protocol (TCP) stack to minimize connection setup time and reduce packet loss.
- HTTP/2 or HTTP/3 Protocols: Switching to modern HTTP protocols enhances performance by allowing multiplexed connections and faster resource loading.
- Compression Algorithms: Implementing efficient compression reduces the size of data transmitted between the client and server, which speeds up transmission time.
Server and Network Configurations
- Data Center Selection: Choose data centers located in regions with high internet speed and low latency to serve users efficiently.
- Load Balancing Techniques: Implement intelligent load balancing strategies to distribute traffic evenly across multiple servers, preventing any single server from becoming overwhelmed.
- Persistent Connections: Maintain long-lived TCP connections, reducing the overhead associated with repeatedly opening and closing connections.
Important: The use of content delivery networks (CDNs) and optimized DNS resolution can significantly reduce latency by serving static assets from locations closer to the user.
Performance Monitoring
Once optimizations are in place, continuous monitoring is essential to ensure the infrastructure maintains low latency over time. Regularly analyzing network traffic, response times, and server performance metrics helps to identify bottlenecks or areas for improvement.
Optimization Technique | Impact on Latency |
---|---|
Edge Computing | Significant reduction in response time by serving data from geographically closer locations. |
TCP Optimizations | Reduces latency through faster handshakes and improved packet loss handling. |
Compression | Decreases data transfer time by reducing the amount of data sent over the network. |
Managing User Authentication in Real-Time Applications
In real-time web applications, managing user authentication effectively is crucial to ensure both security and seamless user experience. Unlike traditional apps where authentication is often a one-time process, real-time apps require continuous session management, which adds complexity. Real-time user interaction, such as in chat apps or collaborative tools, demands that authentication systems are robust and responsive while handling multiple users simultaneously.
Additionally, maintaining a secure environment in a dynamic, constantly updating system requires technologies that enable session persistence, token management, and real-time user validation. Below are essential strategies for managing user authentication in these environments.
Key Authentication Strategies
- Token-based Authentication: Using JWT (JSON Web Tokens) or similar methods ensures that users' authentication states can persist across multiple requests. Tokens can be stored securely in local storage or cookies and verified on each interaction.
- Real-Time Session Handling: In real-time systems, it's important to manage user sessions continuously. A system should be able to detect if a user has logged out or their session has expired and terminate active connections.
- WebSockets Integration: WebSockets provide a bi-directional communication channel that is essential for real-time applications. Authentication should be enforced as part of the WebSocket handshake to ensure only authorized users can establish connections.
Important: Authentication tokens should never be stored in local storage for sensitive applications. Consider using secure HTTP-only cookies to mitigate potential attacks like XSS (Cross-site Scripting).
Example Flow of Authentication
- The user logs in using credentials, which are validated by the backend.
- The backend responds with a JWT or an access token, which the frontend stores.
- On each subsequent request, the frontend includes the token to authenticate the user.
- For real-time apps, the token is sent during the WebSocket handshake, ensuring secure and authorized communication.
- If a token is invalid or expired, the server rejects the request and prompts for re-authentication.
Authentication Table Overview
Method | Description | Use Case |
---|---|---|
JWT (JSON Web Token) | Compact, URL-safe means of representing claims to be transferred between two parties. Typically used for stateless authentication. | Widely used in RESTful APIs and mobile apps. |
OAuth 2.0 | Authorization framework that allows third-party services to exchange web resources without exposing credentials. | Common in scenarios where users need to log in using external accounts (e.g., Google or Facebook). |
WebSocket Authentication | Authentication during the initial connection setup of a WebSocket. Ensures only authorized clients can maintain a persistent connection. | Ideal for real-time apps like chat services or collaborative tools. |
Integrating Push Notifications for Instant User Updates
Push notifications have become an essential feature for real-time applications, providing users with instant alerts and updates without the need to actively refresh the page. By integrating push notifications, web apps can offer a more engaging experience, ensuring users are always informed of critical events in real time. This is especially important for messaging platforms, social networks, and e-commerce sites, where timely communication can significantly impact user experience and engagement.
To implement push notifications effectively, web developers need to utilize technologies such as service workers, Web Push APIs, and background sync to ensure messages are delivered even when the app is not in the foreground. Moreover, it's crucial to design notifications that are both relevant and non-intrusive to enhance user satisfaction rather than disrupt their experience.
Steps to Implement Push Notifications
- Set up a service worker to handle background tasks and message delivery.
- Request the user's permission to send push notifications.
- Integrate a push notification service (e.g., Firebase Cloud Messaging, OneSignal) for easier management.
- Send push messages using the Web Push API and ensure compatibility with all browsers.
- Test and optimize the delivery, timing, and relevance of notifications.
Best Practices for Push Notification Design
- Timing: Notifications should be sent at the right time, when the user is likely to engage with them.
- Personalization: Tailor messages to the user's preferences or previous interactions.
- Clear Messaging: Be concise and ensure the message is actionable.
- Respect User Preferences: Allow users to customize notification settings to reduce unwanted interruptions.
When implemented properly, push notifications can significantly improve user engagement and retention. They serve as a powerful tool to keep users connected and informed in real-time.
Push Notification Service Comparison
Service | Features | Compatibility |
---|---|---|
Firebase Cloud Messaging | Cross-platform, reliable delivery, integration with other Google services | Chrome, Firefox, Safari, Edge |
OneSignal | Advanced segmentation, analytics, A/B testing | Chrome, Firefox, Safari, Edge, iOS, Android |
Pushwoosh | Real-time messaging, geo-targeting, multi-channel support | Chrome, Firefox, Safari, Edge, iOS, Android |
Scaling Real-Time Web Apps to Handle High Traffic
As real-time web applications grow in popularity, ensuring that they can handle large volumes of traffic becomes essential. Unlike traditional web apps, real-time apps often require lower latency, faster updates, and higher scalability to accommodate fluctuating user loads. Without careful planning and optimization, these apps may become slow, unreliable, or even crash under heavy usage. Scaling these applications efficiently can be complex, but there are proven strategies that can help meet the challenge.
To effectively scale real-time apps, it is necessary to focus on improving infrastructure, enhancing system design, and using advanced caching strategies. With the right approach, it’s possible to ensure that a real-time app remains responsive and reliable even during periods of peak traffic. Below are key practices for scaling these systems efficiently.
Key Strategies for Scaling
- Load Balancing: Distribute incoming traffic across multiple servers to prevent any single server from becoming overwhelmed.
- Horizontal Scaling: Add more servers to the pool as traffic increases, allowing for better load distribution and fault tolerance.
- Microservices Architecture: Break down the application into smaller, independent services that can be scaled independently according to demand.
- Event-Driven Design: Use an event-driven approach to handle requests asynchronously, which allows for faster processing and scalability.
Optimizing Communication Channels
In real-time apps, communication channels such as WebSockets or Server-Sent Events (SSE) need to be optimized for high traffic. When dealing with large volumes of concurrent connections, a robust system is necessary to manage message delivery and reduce latency.
Important: Use load balancing strategies for WebSockets and optimize message queuing to handle large numbers of simultaneous connections.
Recommended Tools for Scaling
Tool | Description |
---|---|
Redis | A high-performance in-memory data store used for caching, pub/sub messaging, and real-time data sharing. |
NGINX | A powerful reverse proxy server that can be used to load balance WebSocket traffic and handle concurrent requests. |
Kubernetes | A container orchestration platform that automates scaling and management of containerized applications. |
Conclusion
Scaling real-time web apps requires a combination of infrastructure improvements, system optimizations, and proper tool usage. By focusing on horizontal scaling, load balancing, and efficient communication channels, it is possible to maintain a highly responsive application even during peak traffic periods. The right tools and architecture choices can ensure the app remains stable and scalable in the face of high user demand.