Flutter Build Responsive App
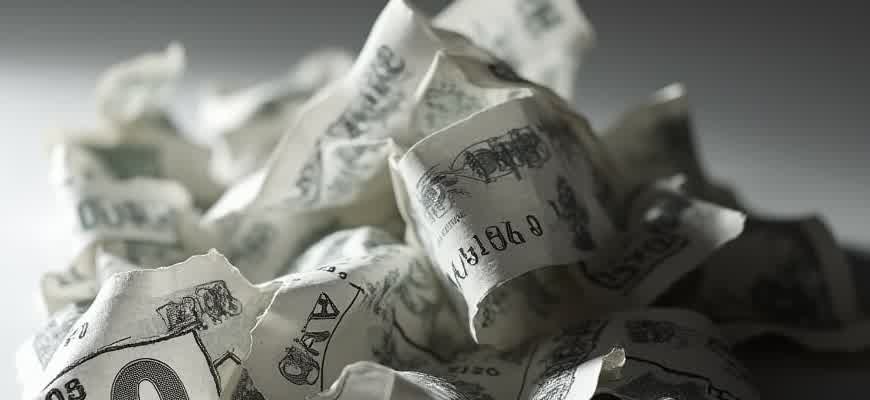
Creating responsive apps in Flutter involves adjusting your layout and UI elements to fit different screen sizes and orientations. Flutter offers several tools and techniques to help developers design applications that adapt seamlessly across various devices.
To build a responsive app in Flutter, consider the following principles:
- Use flexible widgets such as Flexible and Expanded to ensure dynamic sizing of elements.
- Leverage the MediaQuery class to get the screen size and adjust layouts accordingly.
- Implement LayoutBuilder for more control over the widget's size based on the available space.
Important: Always test your app on multiple screen sizes to ensure it behaves as expected across all devices.
One effective approach to make your Flutter app responsive is by using a GridView layout, which automatically adjusts the number of columns based on screen width. This ensures that your content looks great on both large tablets and smaller phones.
To optimize responsiveness, consider the following layout techniques:
- Define breakpoints for different screen sizes.
- Use AspectRatio to maintain consistent proportions.
- Adjust font sizes with TextScaleFactor for readability.
Lastly, you can use a Table widget to create structured layouts that automatically adjust based on available space. This allows your app to remain functional and visually appealing on any device.
Device | Breakpoint |
---|---|
Mobile | 320-600px |
Tablet | 600-900px |
Desktop | 900px+ |
Building a Responsive Application with Flutter
Creating responsive applications in Flutter requires careful handling of various screen sizes and orientations. One of the core principles is to ensure the UI adapts seamlessly to different devices, from mobile phones to tablets and desktops. Flutter provides a set of tools that allow developers to manage layouts and elements dynamically based on screen dimensions.
To build a responsive app, it's important to focus on flexible layouts, scaling elements, and making sure the app responds efficiently to device changes. Flutter's built-in widgets and features like MediaQuery, LayoutBuilder, and the flexible GridView play a significant role in achieving responsiveness.
Key Approaches for Responsiveness
- Use MediaQuery to get device screen size and orientation.
- Leverage LayoutBuilder to build layouts based on parent widget constraints.
- Apply Flexbox or Column and Row for scalable layouts.
- Utilize AspectRatio to maintain proportions of elements.
"Responsiveness in Flutter is not about fixing layouts, but about adapting them to various screen sizes and ensuring a consistent user experience."
Design Principles for Responsiveness
- Fluid Layouts: Build layouts that stretch and contract according to screen size.
- Scalable Text: Ensure that text sizes scale properly using
MediaQuery.of(context).textScaleFactor
. - Dynamic Content: Use flexible widgets that adjust content dynamically.
Example: Creating a Simple Responsive Grid
Widget | Description |
---|---|
GridView |
Used to create a responsive grid layout that adjusts based on screen size. |
Flexible |
Allows widgets to resize within a parent layout, making the app more responsive. |
Choosing the Right Layout for Responsive Design in Flutter
When developing a responsive application in Flutter, selecting an appropriate layout is critical for ensuring the app adapts seamlessly to different screen sizes. Flutter offers several layout options that help developers achieve responsive designs by adjusting the UI components based on available screen space. Choosing the right layout widget can simplify the process of building an app that looks great on both small and large screens.
One of the most important considerations is how the layout scales across different devices. Developers can use flexible and adaptive widgets to make sure the design doesn’t break when transitioning from one screen size to another. Flutter provides a variety of layout options, each designed to address specific responsiveness needs, making it easier to create a fluid user experience.
Popular Layout Widgets for Responsiveness
- Column & Row: These widgets are great for stacking elements vertically or horizontally. They allow flexibility in how elements are spaced and aligned, making them ideal for smaller screens.
- Flex: Flex enables more control over how elements are distributed. It’s perfect when you need to create more complex layouts with dynamic resizing of child elements.
- Wrap: A flexible alternative to Row and Column, Wrap arranges children widgets in a horizontal or vertical sequence that automatically wraps when there’s not enough space.
- GridView: This layout is ideal for displaying a grid of items, such as images or text blocks, that can adapt to various screen sizes.
Layout Decision Process
- Identify Screen Size Requirements: Understand the screen sizes you’re targeting (e.g., mobile, tablet, desktop) and the kind of responsiveness needed.
- Use Flexible Widgets: Make use of widgets like Flexible, Expanded, and MediaQuery to allow elements to resize according to screen dimensions.
- Consider Screen Orientation: Adapt the layout for both portrait and landscape orientations to ensure a consistent experience across devices.
Note: Flutter’s built-in tools like MediaQuery and LayoutBuilder can provide real-time information about the screen size, which helps in determining the best layout for responsiveness.
Grid-Based Layouts
For more complex, multi-item layouts, grid-based systems are often the best choice. GridView in Flutter can be used to display multiple items in a grid-like structure that automatically adapts based on available space. This ensures that the app looks good regardless of the screen's aspect ratio or resolution.
Layout Type | Best For | When to Use |
---|---|---|
Column | Vertical stacking | When elements need to be stacked vertically with equal or flexible spacing |
Row | Horizontal stacking | When elements need to be aligned horizontally |
Wrap | Wrapping elements | When elements need to wrap around when space is limited |
GridView | Multiple items in a grid | When displaying a collection of items in a grid-like layout |
Adapting to Different Screen Sizes with Media Queries in Flutter
Flutter provides a flexible framework for building apps that can adjust to different screen sizes, orientations, and resolutions. One of the key techniques to achieve this is by using media queries, which allow you to query the device's characteristics such as width, height, pixel density, and orientation. By leveraging these queries, developers can create applications that adapt dynamically to various screen configurations, ensuring a seamless user experience across different devices.
In Flutter, media queries are implemented through the `MediaQuery` class, which provides access to detailed information about the current screen. Using these data points, you can conditionally alter the layout, font sizes, and component visibility, optimizing the app's design for different screen sizes without needing to duplicate code or use fixed dimensions.
Using MediaQuery for Responsive Layouts
To implement media queries, you can access `MediaQuery.of(context)` to retrieve the device’s screen properties. Here’s an example of how you can use this to adjust widget sizes based on the screen's width:
double screenWidth = MediaQuery.of(context).size.width; double screenHeight = MediaQuery.of(context).size.height; if (screenWidth > 600) { // Adjust layout for larger screens } else { // Adjust layout for smaller screens }
The following properties can be accessed using `MediaQuery`:
- size - Returns the screen size as a
Size
object (width and height). - orientation - Identifies whether the device is in portrait or landscape mode.
- devicePixelRatio - Indicates the pixel density of the device.
- textScaleFactor - Defines the scale factor for text, which is important for accessibility.
Example: Adjusting Layout Based on Screen Width
By querying the screen width and adjusting widget properties dynamically, you can ensure that your app’s layout is optimized for both small and large devices, improving usability and aesthetics.
Consider the following approach when designing for both mobile and tablet devices:
Screen Size | Layout Adjustments |
---|---|
Small (Phone) | Single-column layout, compact elements |
Large (Tablet) | Multi-column layout, larger padding and margins |
By using media queries in combination with flexible layouts such as `LayoutBuilder` and `Flexible`, you can further enhance the responsiveness of your app.
Using Flexbox and Expanded Widgets for Fluid Layouts in Flutter
In Flutter, creating flexible layouts that adapt seamlessly to different screen sizes is essential for building responsive applications. Two powerful tools for achieving fluid layouts are the Flexbox layout system and the Expanded widget. The Flexbox model is particularly useful for designing user interfaces that automatically adjust based on available space. Combined with the Expanded widget, developers can efficiently distribute space between various UI elements.
The Flexbox system in Flutter works with the Row and Column widgets. These widgets allow for dynamic alignment and distribution of child elements within the available space. The Expanded widget, when wrapped around a child widget, ensures that it occupies any remaining space within its parent. This provides the flexibility needed for responsive designs.
Flexbox Layout with Row and Column
- Use Row for horizontal arrangements and Column for vertical layouts.
- Both Row and Column widgets support the Flex property, enabling custom proportions for children.
- The Expanded widget can be used to fill the remaining space in both Row and Column layouts.
Using Expanded Widget
The Expanded widget is used to create flexible layouts by distributing space across child widgets. When a widget is wrapped inside an Expanded widget, it takes up the remaining space in the main axis of the parent (Row or Column). This behavior is essential for creating layouts that respond to varying screen sizes without fixed dimensions.
Note: Using Expanded can result in more readable code and maintainable layouts when working with complex UI elements.
Example Layout
Widget | Use Case |
---|---|
Row | Horizontal layout |
Column | Vertical layout |
Expanded | Fills remaining space within Row or Column |
Best Practices
- Always test layouts on different screen sizes to ensure proper responsiveness.
- Use Expanded only when necessary, as it can cause child widgets to stretch unnaturally if overused.
- Combine Flexbox with other layout widgets such as Spacer or Align for more complex designs.
Optimizing Flutter Widgets for Multiple Screen Sizes and Orientations
When developing applications using Flutter, one of the primary challenges is ensuring that the interface adapts seamlessly to different screen sizes and orientations. Flutter offers a variety of tools and techniques to make widgets responsive and flexible across multiple devices, but proper implementation requires careful planning and testing. The goal is to provide an optimal experience regardless of whether the app is viewed on a small phone, large tablet, or rotated screen.
In this context, understanding how to adjust the layout and functionality of widgets becomes essential. Several strategies can be applied to make widgets more responsive, ensuring they behave consistently across varying device specifications.
Key Techniques for Responsiveness
- MediaQuery: This provides information about the current screen size, orientation, and pixel density, allowing developers to adjust widget dimensions and layouts accordingly.
- LayoutBuilder: A widget that builds itself based on the parent widget's constraints, making it useful for creating responsive designs that adapt to available space.
- Flexible and Expanded: These widgets allow elements to take up proportional space, making the layout more adaptable to screen sizes.
To ensure the best user experience, it’s important to account for both small screens (like phones) and larger devices (like tablets and desktops) while maintaining performance.
Orientation Considerations
In addition to screen size, orientation plays a significant role in widget optimization. Flutter allows developers to listen for orientation changes and adjust the layout dynamically. Below are some methods for managing orientation changes:
- Use MediaQuery.of(context).orientation to determine the orientation and adjust layouts accordingly.
- Leverage Flexible widgets to ensure that content scales properly when switching between portrait and landscape modes.
- Consider providing different layouts for portrait and landscape orientations to take full advantage of the screen real estate.
Important Considerations for Performance
Aspect | Recommendation |
---|---|
Complex Layouts | Minimize nested widgets and use simpler layouts for better performance on smaller devices. |
Images | Use responsive image sizes to avoid loading unnecessarily large images on smaller screens. |
Animation | Ensure animations are smooth across devices by using the Flutter performance tools to monitor and optimize. |
Creating Adaptive Navigation Bars for Mobile and Tablet Devices
When developing mobile and tablet applications, the navigation bar is one of the key components that needs to be adjusted according to the device's screen size and orientation. The primary goal is to provide users with an intuitive and efficient navigation experience while optimizing the available screen real estate. By using responsive design techniques, developers can ensure that the navigation bar is appropriately displayed across various devices.
Adaptive navigation bars should automatically adjust their layout to accommodate smaller screens on mobile devices and larger displays on tablets. This can be achieved by utilizing Flutter's built-in widgets, such as `Drawer`, `BottomNavigationBar`, and `AppBar`, combined with media queries to detect screen size and orientation. These elements allow developers to create a seamless navigation experience, with the flexibility to switch between different navigation styles based on the platform.
Mobile Navigation
On mobile devices, space is limited, so the navigation bar needs to be compact and accessible. A common approach is to use a bottom navigation bar, which takes up less screen space while still providing easy access to key sections of the app. Here's an example structure:
- Home
- Search
- Profile
- Settings
Tablet Navigation
For tablets, the larger screen allows for a more complex navigation system. Developers often use a drawer menu or side navigation bar for a wider range of options. This layout can provide better organization and visibility of content. Consider this example:
- Dashboard
- Notifications
- Messages
- Help
Note: It is important to test navigation bars on multiple devices to ensure they function properly in both portrait and landscape modes.
Comparison Table: Mobile vs Tablet Navigation
Device | Navigation Type | Optimal Layout |
---|---|---|
Mobile | Bottom Navigation | Compact with 3-5 icons |
Tablet | Drawer or Side Navigation | Expanded menu with icons and text |
Managing Font Sizes and Text Scaling for Diverse Screen Sizes in Flutter
When developing responsive applications in Flutter, one of the critical aspects to address is handling text and font scaling across a variety of devices. Given the wide range of screen sizes and resolutions, ensuring that the text is both readable and visually appealing on all devices is crucial. Flutter provides several ways to handle font scaling, allowing developers to fine-tune how text adjusts based on device characteristics. The key challenge is making sure that text is scalable without compromising the layout or user experience.
Flutter offers built-in tools like the MediaQuery
class and TextScaleFactor
to help manage text sizes dynamically. These allow the font size to adjust based on the screen's pixel density or the user's device settings. Below are some strategies for optimizing text and font size handling in Flutter apps:
Strategies for Handling Text Scaling
- Use of MediaQuery: MediaQuery provides information about the size of the screen and the device's pixel density. This can be used to scale text appropriately based on these factors.
- TextScaleFactor: This widget allows developers to scale the text based on the user’s accessibility settings, ensuring the app respects user preferences for larger or smaller text.
- Flexible Font Sizes: By using relative font sizes, you can make sure that your text scales dynamically. For example, using
em
units instead of fixed pixel sizes allows the font to adjust according to the screen’s properties.
Key Considerations for Font Scaling
When implementing text scaling, always test your app on different screen sizes to ensure the text remains readable and the layout doesn't break. It’s important to account for different font rendering behaviors on various devices.
Additionally, it is essential to consider the following when working with text scaling:
- Ensure font sizes are appropriate for all devices without overflowing or truncating text.
- Respect system-wide settings for text size (e.g., larger accessibility settings) to improve accessibility.
- Avoid hardcoded font sizes that may not adapt well to different screen resolutions.
Example of Text Scaling Implementation
Device | Text Scale Factor | Font Size |
---|---|---|
Phone (Standard) | 1.0 | 16px |
Tablet (Large Screen) | 1.2 | 18px |
Phone (Large Accessibility) | 1.5 | 24px |
By utilizing these strategies, developers can create more adaptable and user-friendly Flutter applications that scale text effectively across different devices, ensuring a seamless user experience regardless of screen size.
Testing Your Flutter Application on Multiple Devices and Screen Sizes
When building a responsive application with Flutter, it is crucial to test your app on various devices to ensure a seamless user experience. Different devices come with varying screen sizes, resolutions, and aspect ratios, which can significantly affect how your app appears and functions. This testing helps you identify issues such as layout distortions, pixel density inconsistencies, and interactions that may not work well on smaller or larger screens.
To efficiently test your app, you can take advantage of Flutter's built-in tools and external emulators. Testing across a wide range of devices ensures that your app provides optimal performance and usability, no matter the device your users are using. Below are some essential tips and methods for testing your Flutter app on different screen resolutions.
Testing Strategies
- Use the Flutter Device Preview package to simulate various screen sizes and resolutions directly within the IDE.
- Test your app on both real devices and emulators to account for hardware-specific performance issues.
- Take advantage of Flutter’s layout widgets like LayoutBuilder and MediaQuery to build adaptive UIs that adjust based on screen size.
- Consider using the Flutter Inspector tool to analyze your app’s UI and troubleshoot any layout problems.
Common Device Resolution Considerations
Testing your Flutter app requires awareness of the screen resolutions of popular devices. Below is a table showing common resolutions across different categories:
Device Type | Resolution | Aspect Ratio |
---|---|---|
Smartphones | 1080x1920 px | 16:9 |
Tablets | 2048x1536 px | 4:3 |
Laptops | 2560x1600 px | 16:10 |
High-Resolution Displays | 3840x2160 px (4K) | 16:9 |
Always test with both high and low resolution screens to catch any issues related to scaling, such as text rendering and image distortion.
Best Practices for Deploying a Responsive Flutter Application
When deploying a responsive Flutter application, ensuring compatibility across various devices and screen sizes is crucial. Flutter’s built-in tools, such as the `LayoutBuilder` and `MediaQuery`, allow developers to create flexible and adaptive layouts. However, the deployment process also requires careful attention to app performance and the optimization of assets to ensure a smooth user experience.
One of the key considerations is testing the app on multiple screen sizes and device orientations to avoid layout issues. Flutter offers the `flutter doctor` command to identify potential issues and the `flutter run` command to test the app on different simulators. Additionally, keeping an eye on the app's performance can prevent lags and other user experience issues.
Performance Tips for Flutter Deployment
- Optimize Asset Loading: Use `AssetImage` for images and pre-load assets in advance to reduce runtime overhead.
- Use `ListView` for Large Data Sets: Instead of rendering all items at once, utilize `ListView.builder` to load only the visible items.
- Minimize Rebuilds: Use the `const` constructor where possible and avoid unnecessary widget rebuilds to improve efficiency.
Remember to profile the app’s performance regularly using Flutter’s DevTools to detect memory leaks, janky animations, and CPU usage spikes before deploying it to production.
Best Practices for Asset Management
- Use Scalable Images: Leverage SVGs or responsive image formats to ensure assets are displayed correctly on different screen sizes.
- Compress Images: Use tools like `flutter_image_compress` to reduce the size of image assets and improve load times.
- Localize Assets: For international apps, use `flutter_localizations` to ensure proper asset loading based on the user’s locale.
Testing on Multiple Devices
- Test responsiveness on different screen sizes, including tablets and foldable devices.
- Use the Flutter device lab to test on multiple virtual and physical devices simultaneously.
- Ensure that touch targets are adequately sized for mobile and tablet devices.
Device Type | Recommended Screen Size |
---|---|
Smartphone | 320px - 600px |
Tablet | 600px - 900px |
Desktop | 900px and up |