Learn Cross Platform App Development
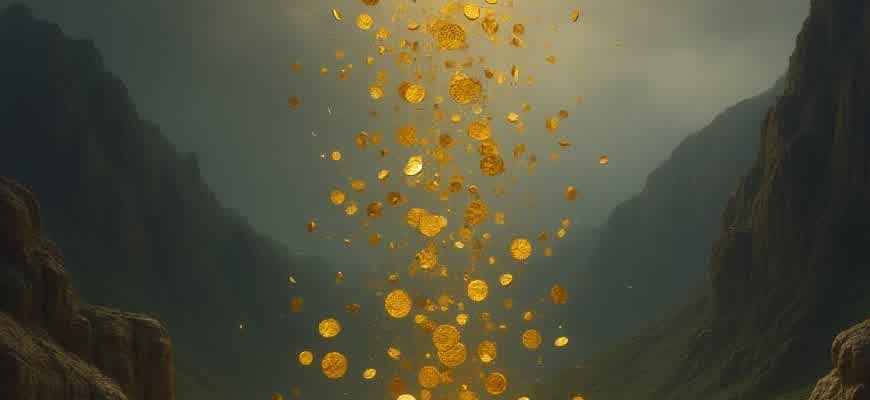
Developing mobile applications that run seamlessly on multiple platforms is a key skill for modern developers. Cross-platform development frameworks offer an efficient way to write a single codebase and deploy it on various operating systems, reducing both time and effort. Whether you're building for Android, iOS, or even web platforms, mastering this approach allows you to reach a wider audience.
Benefits of Cross-Platform Development:
- Code reusability across platforms.
- Faster development time and reduced costs.
- Consistent user experience across devices.
"The power of cross-platform development lies in its ability to streamline the process and eliminate repetitive coding tasks."
Popular Cross-Platform Frameworks:
- Flutter - Known for its fast performance and rich UI components.
- React Native - Offers native-like performance with JavaScript.
- Xamarin - A .NET-based framework, ideal for C# developers.
Framework Comparison:
Framework | Language | Platform Support |
---|---|---|
Flutter | Dart | iOS, Android, Web, Desktop |
React Native | JavaScript | iOS, Android |
Xamarin | C# | iOS, Android, Windows |
How to Choose the Right Framework for Cross Platform Development
Choosing the best framework for cross-platform development can significantly impact the efficiency, performance, and scalability of your mobile or web applications. Different frameworks offer distinct advantages based on project requirements, team skills, and the target audience. Some frameworks are better suited for fast development, while others provide more flexibility and control over the app’s behavior across platforms.
When making a decision, it’s important to evaluate your project’s specific needs, such as the desired level of native functionality, performance, and the availability of community support. By doing so, you can select a framework that aligns with your technical and business goals, ensuring long-term success and sustainability.
Factors to Consider
- Performance: Different frameworks use varying methods to render UI elements, and this can directly impact the app's performance. Ensure that the framework you select meets the performance standards for your application, especially if it requires real-time processing or heavy computations.
- Developer Experience: Choose a framework that aligns with your team’s expertise and available resources. If your team is more familiar with web technologies like JavaScript or TypeScript, frameworks like React Native or Ionic might be a better fit.
- Community and Ecosystem: A large and active community ensures that you have access to resources, tutorials, and plugins, making it easier to resolve issues and speed up development.
- Maintainability: Consider the long-term maintenance and scalability of the framework. A framework with a clear architecture and good documentation can make future updates and debugging easier.
Popular Frameworks Comparison
Framework | Programming Language | Performance | Popular Use Case |
---|---|---|---|
React Native | JavaScript/TypeScript | High | Mobile apps for both Android and iOS |
Flutter | Dart | Very High | Apps with rich UI and smooth animations |
Ionic | JavaScript/HTML/CSS | Moderate | Progressive Web Apps (PWAs) and mobile apps |
Xamarin | C# | High | Enterprise-level applications |
When selecting a framework, prioritize the one that offers the best balance between performance, developer productivity, and long-term support.
Setting Up Your Development Environment for Cross-Platform Apps
Before diving into cross-platform app development, it's crucial to establish a solid development environment. The right setup ensures smooth workflow, compatibility, and efficiency across different platforms. This involves choosing appropriate frameworks, installing necessary software tools, and configuring the environment according to your project's requirements.
Whether you're developing with React Native, Flutter, or Xamarin, setting up a unified environment is the first step towards building seamless apps that work across multiple operating systems. Below are the key steps to consider when preparing your development environment.
Required Tools for Cross-Platform Development
The choice of tools depends on the framework you're working with. Here’s a quick comparison of the most popular tools:
Framework | IDE/Editor | Dependencies |
---|---|---|
React Native | VS Code, WebStorm | Node.js, npm, Expo CLI |
Flutter | Android Studio, VS Code | Flutter SDK, Dart |
Xamarin | Visual Studio | .NET SDK |
Steps to Set Up the Environment
- Install the necessary IDE or text editor: Choose an Integrated Development Environment (IDE) like VS Code or Android Studio, depending on your framework.
- Download platform-specific SDKs: For Android, install Android Studio or set up Xcode for iOS development.
- Set up command-line tools: Install necessary CLI tools like Node.js (for React Native) or Flutter CLI for easier app management.
- Verify dependencies: Ensure that all dependencies like Dart (for Flutter) or .NET SDK (for Xamarin) are installed properly.
Important: Always make sure that the SDK versions are compatible with each other and your project's requirements to avoid conflicts and errors during development.
Testing Your Setup
- Run initial tests: Create a simple "Hello World" app in your framework to test if the setup is working correctly.
- Check for updates: Regularly update your development environment to stay aligned with new releases and improvements in the frameworks.
- Use emulators or real devices: Set up emulators for iOS and Android, or connect real devices for more accurate testing.
Key Challenges in Cross Platform Mobile App Development
Developing mobile applications that work seamlessly across different platforms can save time and resources. However, the process is not without its challenges. Developers often face difficulties due to the inherent differences in platform requirements and expectations. Despite the promise of a unified codebase, achieving native performance, appearance, and functionality remains a challenge for many teams working on cross-platform projects.
While the idea of "write once, run anywhere" is appealing, there are several factors that must be addressed to create a high-quality cross-platform app. These challenges can range from dealing with platform-specific issues to optimizing the app’s performance and user interface. Below are the key challenges that developers commonly encounter in cross-platform development.
1. Platform-Specific Behavior and UI
One of the major hurdles in cross-platform development is handling the unique design patterns, behavior, and interactions of each mobile platform. Different platforms (iOS, Android) come with their own UI components, design guidelines, and interaction models that may not always align with the universal approach of cross-platform frameworks.
"Achieving a native look and feel across all platforms is often harder than anticipated, even with frameworks like Flutter or React Native."
- iOS and Android have distinct approaches to UI components.
- Native elements (e.g., navigation bars, buttons) may behave differently on each platform.
- Ensuring consistent performance across devices with varying specifications is tricky.
2. Performance Optimization
Performance is another significant issue in cross-platform app development. While cross-platform tools like React Native or Xamarin provide the flexibility of a shared codebase, they often don't offer the same level of performance as native apps. Complex animations, advanced graphics, or large-scale data processing can be slower compared to native development.
- Cross-platform frameworks may introduce unnecessary overhead.
- Performance issues arise from bridging code between native and cross-platform layers.
- Memory management can be more complicated in cross-platform environments.
3. Integration with Device-Specific Features
Many mobile applications need to interact with platform-specific features such as GPS, camera, sensors, or push notifications. Cross-platform tools might not always provide full access to these features, and developers often need to implement custom native modules to fill in the gaps.
Feature | Common Issue |
---|---|
Camera | Inconsistent performance or limited access across platforms. |
Push Notifications | Platform-specific configurations and behavior. |
GPS | Differences in accuracy and latency across platforms. |
Optimizing App Performance Across Multiple Platforms
When developing cross-platform applications, maintaining optimal performance across different platforms is crucial. The challenge lies in creating a seamless user experience while adapting to the unique requirements of each platform. Whether you are using frameworks like Flutter, React Native, or Xamarin, there are several strategies that can be implemented to ensure that your app runs efficiently across Android, iOS, and web environments.
Optimization requires careful attention to resource usage, code execution speed, and platform-specific constraints. Below are some key techniques and practices that help enhance app performance on multiple platforms.
1. Efficient Code Sharing and Platform-Specific Customization
- Minimize Redundant Code: Avoid duplicating code unnecessarily by using shared components for cross-platform logic. This reduces overhead and ensures consistency.
- Utilize Platform-Specific APIs: Take advantage of platform-specific features to fine-tune performance. For instance, iOS and Android may offer different optimization mechanisms for handling memory management.
- Conditional Rendering: Customize UI components to fit platform-specific guidelines. For example, use native design patterns for Android and iOS to avoid performance issues caused by layout mismatches.
2. Resource Management and Memory Usage
- Lazy Loading: Load resources only when needed to avoid overburdening the system. For example, load images and data asynchronously.
- Memory Optimization: Use memory profiling tools to identify memory leaks and optimize resource usage. This is essential when targeting multiple platforms with varying resource capacities.
- Efficient Data Handling: Compress large datasets and implement caching strategies to minimize the amount of data loaded at once.
3. Performance Monitoring Tools
Implementing performance monitoring tools helps in tracking app performance across different platforms and identifying areas that need optimization. Below is a comparison of commonly used tools:
Tool | Platform Support | Features |
---|---|---|
Firebase Performance Monitoring | iOS, Android, Web | Real-time performance metrics, network and app start-up tracing |
Flipper | iOS, Android | Debugging, performance monitoring, plugin support |
Sentry | iOS, Android, Web | Error tracking, performance profiling |
Consistent performance monitoring and timely updates are key to ensuring your app remains optimized across all platforms. Regularly check for platform-specific changes that might affect app performance.
Integrating Native Features in Cross-Platform Apps
One of the primary challenges when building cross-platform applications is integrating native features of each platform while maintaining code reusability. Cross-platform development frameworks, such as Flutter, React Native, and Xamarin, allow developers to write code once and deploy it on multiple platforms. However, sometimes these frameworks do not provide full access to platform-specific capabilities, which leads to the need for native code integration.
To address this, developers often rely on plugins, bridges, or platform channels that allow communication between the cross-platform code and native modules. These integrations enable the use of native APIs, such as device hardware access, camera functionality, or platform-specific UI elements, ensuring a more native experience for users.
Methods for Integrating Native Features
- Plugins and Packages: Many cross-platform frameworks provide plugins or packages that extend the functionality of native APIs. For example, React Native has a rich ecosystem of third-party libraries, while Flutter offers plugins like flutter_camera for camera access.
- Native Modules: In cases where no pre-built solution exists, developers can write custom native code for each platform. This involves creating platform-specific code (Java for Android, Swift for iOS) that is invoked from the shared codebase.
- Platform Channels: Frameworks like Flutter use platform channels to communicate between Dart code (the framework's language) and native code. This allows seamless data exchange and execution of platform-specific logic.
Tip: When integrating native features, always ensure to handle platform-specific behavior properly to avoid performance issues and ensure consistency across platforms.
Common Native Features and Integration Examples
Feature | Native Integration Method | Framework Example |
---|---|---|
Camera Access | Use of platform-specific APIs or third-party packages | React Native (react-native-camera), Flutter (camera plugin) |
Push Notifications | Utilize native notification services with custom configuration | React Native (react-native-push-notification), Flutter (firebase_messaging) |
Geolocation | Access location services directly through native code | React Native (react-native-geolocation), Flutter (geolocator) |
Effective Strategies for Debugging and Testing Cross-Platform Applications
When developing cross-platform applications, ensuring smooth functionality across different devices and platforms is crucial. Proper debugging and testing help identify and resolve potential issues early, improving both performance and user experience. As cross-platform frameworks often rely on a single codebase, debugging can be challenging due to the complexity of managing platform-specific differences. To address these challenges, developers should follow structured practices to streamline the debugging and testing process.
One of the key approaches is leveraging platform-specific tools and simulators to replicate real-world conditions. Additionally, automated testing tools can speed up the process by quickly identifying regressions and issues in different environments. Below are some best practices that will help you debug and test your cross-platform application effectively.
1. Use Platform-Specific Debugging Tools
Each platform (iOS, Android, etc.) comes with its own set of debugging tools. Leveraging these tools allows developers to quickly isolate platform-specific issues. Some examples include:
- Xcode Debugger for iOS apps
- Android Studio Profiler for Android apps
- React Native Debugger for React Native apps
These tools help monitor app performance, trace errors, and debug efficiently. It is recommended to use them in combination with common logging practices to gain deeper insights into application behavior across different platforms.
2. Automated Testing and Continuous Integration
Automated testing ensures that each part of the application functions as expected across platforms. Tools such as Appium, JUnit, or Flutter's integration testing can help automate tests for UI interactions, API calls, and other functional elements.
- Write unit tests for core functionality.
- Use integration tests to verify that all components work together.
- Set up continuous integration (CI) to run tests on every code change.
Automated testing helps prevent regressions, ensuring that new code changes do not negatively affect existing functionality.
3. Test on Real Devices
Simulators and emulators are helpful, but they cannot always replicate real-world conditions accurately. Testing on actual devices is crucial to understanding how the app performs under various conditions. Key areas to focus on include:
- Network performance (slow connections, dropouts)
- Battery usage and resource consumption
- Device-specific features (camera, sensors, etc.)
Testing on real devices ensures the app performs optimally across different screen sizes, resolutions, and hardware capabilities.
4. Use Cross-Platform Debugging Frameworks
Frameworks like Flutter DevTools or React Native Debugger offer cross-platform debugging support that can streamline the process. These tools allow developers to debug their apps on both iOS and Android with a unified interface.
Framework | Debugging Tool | Supported Platforms |
---|---|---|
Flutter | Flutter DevTools | iOS, Android, Web |
React Native | React Native Debugger | iOS, Android |
Monetizing Your Cross Platform Application
Monetizing a cross-platform mobile application can be a key factor in its success. Once your app is developed and ready for the market, the next step is figuring out how to generate revenue from it. There are several ways to achieve this, depending on your app's nature and target audience. The right monetization strategy can help you achieve a sustainable income while also offering value to your users.
Some of the most common methods include offering in-app purchases, subscriptions, and ad-based revenue. Each approach has its own advantages and drawbacks, and it's important to consider which model best fits your app's goals and user experience. Let’s explore these methods in more detail.
Monetization Strategies
- In-App Purchases: Selling virtual goods or premium content within the app, such as additional features, levels, or functionality.
- Subscription Model: Charging users a recurring fee for access to premium content, services, or features. This is often used for media, fitness, or SaaS apps.
- Advertisements: Displaying ads within the app, either as banner ads, video ads, or native ads. This model generates revenue based on user engagement.
- Freemium Model: Offering a free version of the app with limited features, while charging for access to advanced features or content.
- Paid App: Charging users a one-time fee to download your app from the app store.
Considerations for Effective Monetization
Tip: Choose a monetization strategy that complements your app’s functionality and user experience. Invasive ads or hidden charges can frustrate users and lead to a high uninstall rate.
To ensure long-term success, you must consider user retention and overall satisfaction. Offering value is key to getting users to spend money within your app. Below are some factors to keep in mind:
- Target Audience: Know who your users are and what they are willing to pay for.
- Pricing Structure: Whether it’s a one-time fee or a subscription, make sure the pricing is competitive and justifiable.
- Ad Placement: For ad-based monetization, ensure that the ads do not negatively impact the user experience. Place them in less intrusive locations.
- Market Research: Analyze competitor apps to understand what monetization models work best within your app's category.
Comparing Monetization Models
Monetization Model | Pros | Cons |
---|---|---|
In-App Purchases | Generates ongoing revenue, scalable | Requires constant content updates to keep users engaged |
Subscription Model | Reliable, recurring income | Users may cancel subscriptions if they don’t see enough value |
Advertisements | No need to charge users, passive income | May lead to user dissatisfaction if overused |
Freemium | Attracts a large user base | Conversion to paid users may be slow |