Ios App Development Kit
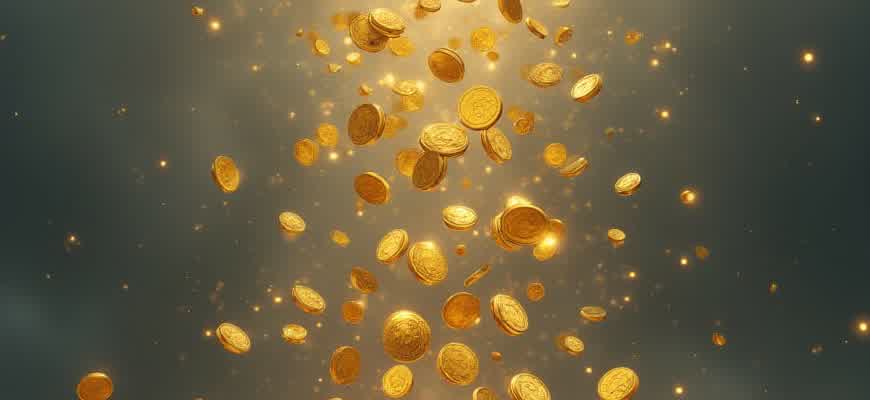
When developing applications for iOS, having the right set of tools is crucial to streamline the process and ensure a high-quality product. The iOS development ecosystem is built around a number of core components that provide developers with the necessary resources to create robust and user-friendly apps.
Key Components of an iOS Development Kit:
- Xcode: The primary Integrated Development Environment (IDE) for iOS app development, providing a suite of tools like Interface Builder, a debugger, and performance analyzers.
- Swift: The preferred programming language for iOS applications, known for its speed, safety, and expressiveness.
- UIKit: A framework that allows developers to create user interfaces with rich visual elements and touch-based interactions.
- Simulator: A tool for testing and debugging applications on various iOS devices without needing the physical hardware.
Important Note: Xcode also includes simulators for different Apple devices, allowing developers to test how their app will function across a wide range of devices and screen sizes.
Development Process Overview:
- Setup and configuration of the Xcode environment.
- Development of the app’s UI using Interface Builder or programmatically via UIKit.
- Writing logic and business rules using Swift.
- Testing the app on different devices using the simulator and physical devices.
- Debugging and optimization before the final submission to the App Store.
Frameworks and Libraries: The iOS SDK includes various other frameworks that help speed up the development process. Here's a brief look at some of the most useful:
Framework | Purpose |
---|---|
CoreData | Used for managing data models and persistent data storage. |
CoreAnimation | Facilitates smooth animations and visual effects. |
CloudKit | Enables seamless cloud integration for data syncing and storage. |
Building Robust Applications with an iOS Development Kit: A Comprehensive Guide
When embarking on the journey of developing iOS applications, the first step is understanding the tools that will shape the app's performance and user experience. The iOS Development Kit provides developers with a powerful suite of frameworks, libraries, and software development tools to create robust, feature-rich mobile applications. The key to mastering iOS app development lies in knowing how to effectively use the kit's components, from Xcode to various APIs and testing utilities.
To build high-performing apps, developers must be equipped with essential knowledge of the development environment, tools, and best practices. By leveraging the iOS development toolkit, you can streamline the coding process, ensure scalability, and deliver polished apps. This guide walks you through the crucial elements needed to harness the full potential of the iOS development ecosystem.
Key Tools in the iOS Development Kit
The iOS Development Kit provides several components to assist developers in creating reliable apps. Here are the primary tools involved:
- Xcode: The main integrated development environment (IDE) for iOS development. It includes a code editor, a simulator, debugging tools, and a performance analyzer.
- Swift: The programming language recommended for iOS development due to its speed, safety, and modern features.
- UIKit & SwiftUI: Frameworks used to design the user interface. While UIKit is the traditional approach, SwiftUI is the modern, declarative framework.
- Core Data: A framework for managing the app's model layer, helping store and retrieve data efficiently.
Key Steps to Build a Robust iOS Application
To develop a reliable and scalable iOS app, follow these essential steps:
- Set Up Your Development Environment: Download and install Xcode. Familiarize yourself with the IDE and its features.
- Design the User Interface: Use SwiftUI or UIKit to create intuitive and responsive interfaces for the app.
- Implement Business Logic: Write the necessary code using Swift to handle the app’s functionality.
- Test the Application: Use XCTest to ensure that your code works as expected. Don't forget to use the iOS Simulator for testing across multiple devices.
- Optimize Performance: Use tools like Instruments to identify performance bottlenecks and improve the app's speed.
"Leveraging frameworks such as Core Data and integrating testing tools early in development ensures that your app remains scalable, secure, and ready for production."
Essential Frameworks and APIs for iOS Development
These frameworks and APIs provide the backbone for most iOS applications:
Framework | Description |
---|---|
Core Animation | Used for creating smooth animations and effects in iOS applications. |
Core Location | Handles the location-based services, allowing apps to access GPS and other location data. |
MapKit | Used for integrating maps into your app and adding features like pinning locations and displaying routes. |
AVFoundation | Helps with audio and video processing, allowing apps to play, record, or edit media. |
Choosing the Right Tools for iOS App Development
When developing an iOS app, selecting the right tools is critical for creating a seamless and high-quality user experience. The tools you choose directly influence your productivity, the app's performance, and your ability to implement advanced features. Some tools are ideal for specific types of projects, while others offer flexibility across various use cases. Understanding the purpose of each tool and how it fits into your development pipeline will ensure that you are using the best solutions for your needs.
Below, we explore some of the most commonly used tools for iOS development. From programming languages to testing frameworks, it's essential to make informed decisions early in the development process. The following sections outline the core tools that can be considered when developing an iOS application.
Core Tools for iOS App Development
- Swift - The modern, fast, and safe programming language for iOS app development. It is easy to learn and offers powerful features that improve app performance.
- Objective-C - Older, but still relevant, especially for maintaining legacy applications. It offers strong compatibility with Apple's ecosystem.
- Xcode - The official integrated development environment (IDE) for building iOS apps. It includes tools for code writing, UI design, and testing.
- UIKit - Framework for building graphical elements and user interfaces within iOS apps. It’s highly customizable and works with Swift and Objective-C.
- Core Data - A framework for managing data models and persistence within your app.
Popular Testing and Debugging Tools
- XCTest - The testing framework provided by Apple, ideal for unit and UI testing.
- TestFlight - A service for beta testing your app with real users before launch.
- Instruments - A set of performance and debugging tools integrated within Xcode to optimize app performance.
Comparison of iOS Development Tools
Tool | Primary Use | Languages Supported | Key Feature |
---|---|---|---|
Xcode | IDE for iOS development | Swift, Objective-C | Interface Builder, Debugger |
Swift | Programming language | Swift | Safety and Performance |
TestFlight | Beta testing | All iOS apps | Beta Distribution |
Choosing the right development tools not only saves time but also significantly reduces the potential for errors, leading to a smoother app development process.
Preparing Your Development Setup for iOS Applications
Before diving into building iOS applications, it’s essential to configure a robust and functional development environment. This process ensures you have all necessary tools and frameworks in place, allowing for seamless development and testing. The foundation of iOS development lies in macOS and Xcode, Apple's official integrated development environment (IDE) for iOS apps.
Here are the steps involved in setting up your development environment:
1. Install macOS and Xcode
To start iOS app development, you must first have macOS installed, as Xcode is only available for macOS. Xcode provides everything needed for creating iOS apps, from design and coding to testing and debugging. Follow these steps:
- Download macOS from the official Apple website or App Store if not already installed.
- Install Xcode via the Mac App Store. The installation can take some time depending on your internet speed.
- Once installed, launch Xcode and sign in with your Apple ID to access the development tools.
Make sure you have the latest version of Xcode to ensure compatibility with the newest iOS features.
2. Set Up Command Line Tools
In addition to the Xcode IDE, Apple provides command-line tools that are essential for various tasks, such as installing dependencies and using Git for version control.
- Open Xcode and go to Preferences > Locations.
- Under "Command Line Tools," select the appropriate version of Xcode.
3. Install CocoaPods or Swift Package Manager
Managing dependencies is an important part of iOS development. To make this process easier, you can use CocoaPods or Swift Package Manager (SPM).
Package Manager | Purpose |
---|---|
CocoaPods | Helps manage third-party libraries for your app. |
Swift Package Manager | Integrated directly into Xcode and Swift, allowing for easy dependency management. |
Both CocoaPods and Swift Package Manager are widely used for dependency management in iOS apps, but Swift Package Manager has become increasingly popular due to its integration with Xcode.
Integrating Swift into Your iOS Development Environment
Swift is the primary programming language for iOS development, offering performance and safety improvements over Objective-C. By integrating Swift into your iOS App Development Kit (ADK), you unlock a range of features and tools that enhance the app development process, from debugging to efficient memory management. Swift's modern syntax and robust error handling make it a reliable choice for building high-performance applications.
To successfully integrate Swift, you need to ensure that the iOS ADK includes the necessary frameworks and libraries that support Swift. This involves understanding how to configure your development environment, set up the correct dependencies, and use Swift with tools like Xcode for code compilation and debugging. Additionally, the integration process involves managing compatibility between Swift and Objective-C when combining legacy code with modern Swift applications.
Steps to Integrate Swift with Your iOS ADK
- Ensure that your project is set up to support Swift by configuring the build settings in Xcode.
- Enable Swift compatibility in your project by adding a Swift bridging header when mixing Swift with Objective-C code.
- Set up the Swift version within your project’s settings to align with the version of Xcode you are using.
- Use Swift’s error handling system (try-catch) to prevent runtime crashes and improve code stability.
- Test the integration by running your project on multiple devices and simulators to ensure compatibility.
Important Considerations When Using Swift
Performance optimization: Swift provides significant performance improvements compared to older languages, but it is important to analyze performance using Xcode’s built-in tools such as the profiler to identify bottlenecks.
Key Swift Libraries for iOS Development
Library | Description |
---|---|
UIKit | Used for creating user interfaces, handling touch events, and managing views in iOS applications. |
Core Data | Provides data management solutions, such as object graph management and persistent storage capabilities. |
SwiftUI | Declarative framework for building user interfaces using Swift, streamlining UI development with reactive programming. |
Final Thoughts
Integrating Swift into your iOS App Development Kit is a seamless process when you take advantage of Xcode’s tools and properly configure your project. Swift’s features allow for better performance, maintainability, and code clarity. Be sure to follow the outlined steps to ensure a smooth integration and leverage Swift’s modern capabilities for developing high-quality apps.
How to Leverage Xcode for Efficient iOS App Development
Xcode is an essential tool for iOS app development, providing a comprehensive environment for designing, coding, and testing apps. With its vast array of features, developers can streamline their workflow and accelerate the development process. By taking advantage of Xcode’s built-in tools, such as Interface Builder, debugging tools, and simulators, developers can efficiently create high-performance applications.
This guide will focus on how to use key Xcode functionalities to optimize the app development process. Xcode’s features not only aid in code writing but also help with user interface design, performance monitoring, and app testing. Below are some techniques that can be used to make iOS development more efficient and seamless.
Key Features for Streamlining Development
- Interface Builder – Xcode’s Interface Builder allows developers to design user interfaces visually, reducing the need for manual coding of UI elements.
- Integrated Debugging Tools – Xcode’s debugging tools help developers quickly identify and fix issues, reducing time spent on troubleshooting.
- Simulators – Xcode includes multiple device simulators, enabling developers to test apps across different screen sizes and iOS versions without needing physical devices.
- Code Completion & Syntax Highlighting – These features help increase coding speed and minimize errors by suggesting code snippets and highlighting syntax issues.
Steps to Streamline Your Workflow
- Create a New Project – Start by selecting the appropriate template based on your app type, such as a Single View App or a Tabbed App, to set up the foundation.
- Design the Interface – Use Interface Builder to drag and drop UI elements. Customize the elements with properties and connect them to your code.
- Write the Code – Utilize Xcode’s code editor with features like autocomplete, syntax checking, and error highlighting to write clean, efficient code.
- Test on Simulators – Run your app in various simulators to ensure compatibility across different devices and iOS versions.
- Debugging – Use breakpoints and the console to quickly detect and resolve issues in your code.
“Using Xcode’s suite of tools, developers can rapidly prototype, test, and refine apps, making the overall development process faster and more efficient.”
Helpful Xcode Tools and Functions
Tool | Purpose |
---|---|
Interface Builder | Design the user interface visually with drag-and-drop functionality. |
Swift Playgrounds | Experiment with Swift code and instantly see the results. |
Simulator | Test the app on various virtual devices without needing a physical device. |
Instruments | Analyze app performance and identify memory leaks or other performance bottlenecks. |
Designing Custom User Interfaces with Interface Builder
Interface Builder is an essential tool in iOS development that allows developers to visually design user interfaces. Using a drag-and-drop interface, developers can quickly lay out components, set properties, and bind UI elements to underlying code, significantly speeding up the design process. This tool is integrated within Xcode and enables a seamless design experience for creating custom, dynamic interfaces for iPhone and iPad apps.
When designing custom interfaces, Interface Builder provides an intuitive way to create complex layouts without needing to write large amounts of code. Developers can easily use predefined UI components, customize them, and ensure they adapt to various screen sizes using Auto Layout. Below are some of the fundamental features and steps involved in creating custom user interfaces.
Steps to Create Custom Interfaces in Interface Builder
- Start by opening your Xcode project and selecting a storyboard or XIB file where you want to design your UI.
- Drag UI components (such as buttons, labels, or text fields) from the object library to the canvas.
- Set properties for each component (e.g., text, colors, font styles) in the Attributes Inspector.
- Use Auto Layout to define constraints and make the interface responsive to different screen sizes.
- Connect UI elements to code through IBOutlets and IBActions to handle user interactions.
Key UI Components and Properties
Component | Description |
---|---|
UILabel | Used for displaying text. Can be customized for font, color, and alignment. |
UIButton | Allows users to trigger actions. Text and style can be modified. |
UITextField | Allows users to enter text. It supports custom borders and placeholder text. |
Remember, Auto Layout is crucial for ensuring your custom interface works across different device sizes, especially with the variety of iPhone and iPad screen resolutions.
Final Considerations
Creating custom interfaces with Interface Builder not only simplifies the design process but also improves the flexibility of your app’s UI. By leveraging Auto Layout and connecting interface elements to your code, you can build responsive and interactive applications efficiently. Whether you're working on a simple app or a more complex one, Interface Builder allows you to quickly iterate on design ideas and test them directly on the device or simulator.
Optimizing iOS Application Efficiency Using Built-in Tools
When developing iOS applications, ensuring high performance is essential for providing users with a seamless experience. Fortunately, Apple's development ecosystem offers a variety of built-in frameworks designed to enhance application efficiency. These frameworks help streamline common tasks such as memory management, graphics rendering, and network communication, contributing to faster and more responsive apps.
Leveraging these built-in tools can significantly reduce the time spent on performance-related optimizations, allowing developers to focus on features and user experience. The integration of frameworks such as Core Data, Metal, and Grand Central Dispatch ensures efficient management of system resources while maximizing application performance.
Key Built-in Frameworks for Performance Improvement
- Core Data: A powerful framework for managing object graphs and persistent data. It allows developers to handle large datasets efficiently by minimizing memory usage and simplifying data retrieval operations.
- Metal: A high-performance graphics framework that provides direct access to the GPU, enabling the development of graphics-intensive applications such as games or image processing tools.
- Grand Central Dispatch (GCD): GCD simplifies multi-threading, allowing for better management of concurrent tasks and ensuring that the application remains responsive, even during intensive background operations.
- SwiftUI: While primarily a UI framework, SwiftUI optimizes rendering performance by updating only necessary parts of the view hierarchy, which reduces unnecessary computation and redraws.
"Optimizing performance isn't just about making an app faster–it's about using the right tools to manage resources effectively, ensuring a smoother experience for users across different devices."
Performance Metrics and Tools for Monitoring
To identify areas that need optimization, developers can utilize several built-in tools provided by Xcode. These tools help monitor memory usage, CPU usage, and other critical performance metrics during development and testing.
Tool | Purpose |
---|---|
Instruments | Provides detailed insights into performance, memory usage, and energy impact. Essential for identifying performance bottlenecks. |
Time Profiler | Monitors the time spent on various methods, helping to pinpoint time-consuming tasks within the app. |
Memory Graph Debugger | Visualizes memory usage and helps detect memory leaks and retain cycles, preventing crashes and slowdowns. |
"By actively using performance monitoring tools, developers can make data-driven decisions to refine app performance and provide a more responsive user experience."
Testing iOS Applications on Simulators and Physical Devices
When developing iOS applications, it is crucial to test the app's functionality across different environments to ensure its reliability and performance. Two primary testing options are available: simulators and real devices. Both have their advantages and limitations, which developers must understand to optimize their testing strategy.
The iOS Simulator, provided by Xcode, is a tool that mimics various iPhone and iPad models on your Mac. It allows developers to quickly run and debug their apps without needing an actual device. However, it cannot replicate certain device-specific features such as accelerometer or GPS performance.
Testing with Simulators
Simulators are ideal for basic testing and debugging, especially when dealing with UI layout, app flow, and general functionality. They allow developers to simulate a variety of devices and iOS versions without physical hardware.
- Advantages: Quick testing, access to multiple device types, easy debugging
- Disadvantages: Cannot fully replicate device performance, lacks some hardware capabilities (e.g., sensors, camera)
Testing on Real Devices
Testing on real devices ensures that the app performs as expected in real-world conditions. This is critical for assessing performance metrics such as battery usage, network latency, and device-specific behaviors.
- Advantages: Accurate performance metrics, access to full hardware features, real-world testing environment
- Disadvantages: Time-consuming setup, requires multiple devices for comprehensive testing
Testing on real devices is essential for performance and usability, as simulators cannot capture the nuances of real-world use.
Device Testing Matrix
Device Type | Simulator | Real Device |
---|---|---|
iPhone | Available for various models | Must be connected physically |
iPad | Available for various models | Must be connected physically |
Sensor Testing (e.g., Accelerometer) | Not supported | Fully supported |