Android App Development in Visual Studio 2022
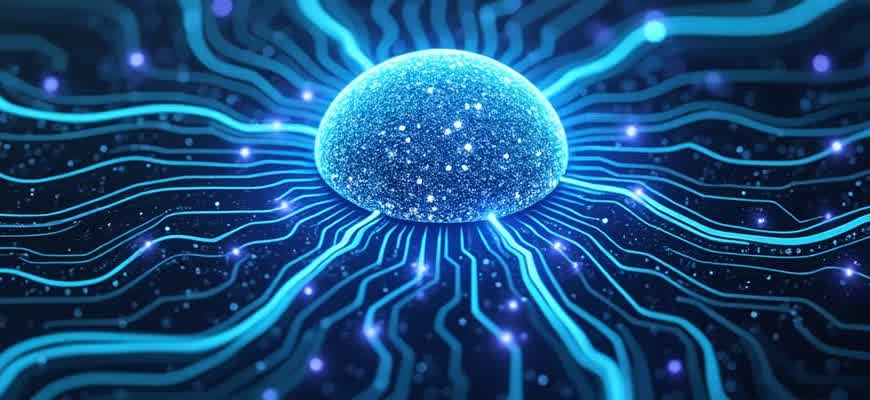
Visual Studio 2022 provides a robust environment for building Android applications. With the integration of Xamarin, developers can write cross-platform apps for Android and iOS using C#. This approach not only streamlines the development process but also ensures code sharing across different platforms, significantly reducing the overall development time.
To start building Android apps in Visual Studio 2022, you need to set up the necessary tools. Follow the steps below to ensure that your development environment is properly configured:
- Download and install Visual Studio 2022 from the official website.
- During installation, select the "Mobile development with .NET" workload.
- Ensure that Android SDKs and NDKs are installed, along with emulators for testing.
Once the environment is set up, developers can create new Android projects using Xamarin. Xamarin provides an efficient way to write Android-specific code in C#, while also enabling access to native Android APIs.
"Xamarin allows developers to build fully native Android apps while sharing significant code between platforms, leading to faster time-to-market."
Here is a quick overview of the main components involved in Android development within Visual Studio:
Component | Description |
---|---|
Xamarin | A framework for cross-platform app development using C# and .NET. |
Android SDK | Software Development Kit for building and testing Android applications. |
Emulator | A tool for testing apps on a simulated Android device. |
Setting Up Visual Studio 2022 for Android Development
To start developing Android applications in Visual Studio 2022, you need to ensure that your environment is properly configured. This involves installing the required components such as the Android SDK, Java Development Kit (JDK), and configuring Visual Studio to support mobile development. Once the environment is set up, you can begin creating, building, and testing Android apps directly from within the IDE.
Follow the steps outlined below to configure Visual Studio 2022 for Android development. It is important to ensure that all necessary components are installed and configured correctly to avoid issues during development.
Installation Steps for Android Development
- Download Visual Studio 2022: Begin by downloading the Visual Studio installer from the official website. Make sure to select the version that supports mobile development during installation.
- Install Android SDK and NDK: During the setup, Visual Studio provides an option to install the Android SDK and NDK (Native Development Kit), which are required for building Android applications.
- Configure JDK: Ensure that the correct version of the Java Development Kit (JDK) is installed. This is essential for Android development as it compiles Java code used in Android apps.
- Enable Xamarin and Mobile Development Features: Check the box for Xamarin in the installation wizard. Xamarin is necessary for building cross-platform mobile applications within Visual Studio.
Verification and Additional Configuration
After completing the installation, you can verify that everything is set up correctly by opening Visual Studio 2022 and creating a new Android project. If the project template for Android is available, your environment is configured properly.
Important: Ensure that your Android device or emulator is running to test your application. You may need to install additional components like the Android Emulator, which can be done from the Visual Studio Installer.
Required Tools
Tool | Purpose |
---|---|
Android SDK | Necessary for building Android apps and managing Android-specific dependencies. |
Java Development Kit (JDK) | Required for compiling Java-based code used in Android apps. |
Xamarin | Allows you to build cross-platform apps for Android and iOS from within Visual Studio. |
With these tools and configurations in place, you're ready to begin developing Android applications in Visual Studio 2022. Ensure that your environment is kept up to date to take advantage of the latest features and improvements.
Setting Up SDKs and Dependencies for Android Development
When starting an Android project in Visual Studio 2022, one of the first tasks is to ensure that all necessary SDKs and dependencies are properly installed. This step is crucial for enabling the development of Android applications and ensuring compatibility with various Android versions. The Android SDK provides essential tools such as the platform tools, build tools, and emulators, which are required for building, testing, and debugging Android apps.
Visual Studio simplifies the process of setting up the development environment by allowing integration with the Android SDK. However, there are specific dependencies and SDK components that must be installed and configured. Below is a guide on how to install and configure these components in Visual Studio 2022.
Installing SDKs and Required Tools
To start working with Android in Visual Studio 2022, you need to install the Android SDK, Java Development Kit (JDK), and other dependencies. Follow these steps to ensure everything is set up correctly:
- Download and Install the Android SDK: Visual Studio includes an installer for the Android SDK, but you need to confirm that the SDK is up-to-date.
- Install the Java Development Kit (JDK): The JDK is necessary for compiling Java-based Android code. Make sure to install the correct version, compatible with your Android SDK.
- Configure the SDK Manager: Use the SDK Manager to install the required Android platforms, build tools, and other libraries needed for your project.
- Install Android Emulator: If you plan to test your app on an emulator, install the necessary system images for different Android versions.
Note: Make sure to update the SDK and tools regularly to avoid compatibility issues with new Android features.
Required SDK Components
The following SDK components are typically needed for Android projects:
Component | Description |
---|---|
Android SDK Platform | Contains the core libraries and tools required for building Android apps for specific versions of Android. |
Build Tools | Includes tools required to compile and package your app. |
Android Emulator | Provides a virtual device to test and debug your Android applications without needing a physical device. |
Android Support Libraries | Provides backward-compatible versions of Android APIs, which is essential for supporting older Android versions. |
After completing these steps, you’ll be ready to start developing Android apps in Visual Studio 2022. Make sure all dependencies are properly installed and configured before you begin coding. Regular updates to both Visual Studio and the Android SDK will ensure smooth development and compatibility with the latest features and Android versions.
Creating Your First Android Project in Visual Studio
Developing Android applications in Visual Studio provides a rich, powerful development environment with a wide array of tools. The IDE integrates features such as debugging, testing, and UI design, making it easier to bring your ideas to life. This guide will help you get started with your first Android project in Visual Studio 2022.
To begin, you must have the necessary setup in place, including installing the Android development workload. Once that's done, you can create a new Android project, configure it for your specific needs, and start building your app. Below are the essential steps to follow.
Steps to Create Your First Android Project
- Install Required Tools: Ensure that you have the Android development workload installed in Visual Studio. This includes the Android SDK, Emulator, and Xamarin tools.
- Create a New Project: Go to File > New > Project. Select "Android" from the project templates.
- Choose a Template: Select a project template that fits your needs, such as "Blank App" for a minimal starting point or "Master-Detail" for more complex apps.
- Configure Project Settings: Enter a name, choose a target Android version, and set the project location.
- Build the Project: Once the project is set up, build the solution to ensure that all components are properly configured.
- Start the Emulator: Launch an Android emulator or connect a physical device to test the application.
Important Considerations
Make sure that your Android SDK and emulator are up to date. Regular updates ensure that your development environment remains compatible with the latest Android features.
Project Configuration Details
Setting | Description |
---|---|
Target Framework | Specify the version of Android that the app will support. |
Package Name | Define a unique identifier for your app, which is important for deploying on the Play Store. |
Minimum Android Version | Set the lowest version of Android that your app will run on. |
Using Xamarin for Cross-Platform Mobile App Development
When building mobile applications that target multiple platforms, Xamarin provides a powerful framework for developing cross-platform apps with a shared codebase. By leveraging C# and .NET, developers can create native-like experiences on both Android and iOS. Xamarin simplifies the development process by allowing code reuse across platforms, reducing time and resources needed for app creation.
Xamarin integrates well with Visual Studio 2022, offering developers an environment that supports debugging, testing, and deployment in a seamless manner. This makes it an attractive option for those looking to build efficient mobile applications without the need to manage separate codebases for different platforms.
Key Features of Xamarin
- Cross-platform Development: Write one codebase in C# and run it on both Android and iOS devices.
- Native Performance: Xamarin compiles to native code, providing high performance and access to platform-specific APIs.
- UI Consistency: Xamarin allows developers to create consistent user interfaces that match the design principles of each platform.
- Extensive Libraries: Utilize .NET libraries and third-party packages to enhance functionality across both platforms.
Advantages of Xamarin
- Reduced Development Time: A single codebase minimizes the need for writing separate logic for Android and iOS.
- Cost Efficiency: Maintaining one codebase means fewer resources for development and maintenance.
- Access to Native Features: Xamarin provides access to device-specific APIs, enabling deep integration with the mobile platform.
Xamarin’s ability to bridge the gap between native development and cross-platform solutions allows developers to create apps that are both performant and efficient.
Comparison of Xamarin with Other Frameworks
Feature | Xamarin | React Native |
---|---|---|
Programming Language | C# | JavaScript |
Performance | Native | Near-Native |
UI Customization | Platform-specific | Component-based |
Code Reusability | High (C# codebase) | High (JS components) |
Debugging Android Applications in Visual Studio 2022
Debugging plays a crucial role in the development of Android applications, ensuring that developers can identify and fix issues efficiently. Visual Studio 2022 provides a rich set of tools to help developers troubleshoot their apps, offering features such as breakpoints, logs, and real-time inspection. By integrating with Android emulators or physical devices, developers can perform thorough testing and debugging directly from the IDE, ensuring high-quality performance in their applications.
In Visual Studio 2022, the debugging process is streamlined through various built-in features. Developers can run apps in debug mode, where they can pause execution at specific breakpoints, inspect variable values, and examine the flow of the application. The debugging interface also supports monitoring system performance, logging errors, and reviewing crash reports, all of which are essential for delivering a polished Android application.
Key Debugging Features
- Breakpoints: These allow developers to pause the execution of the app at specific points, enabling inspection of the app’s state.
- Logcat: Provides detailed logs, including stack traces and error messages, helping developers identify problems during runtime.
- Live Reload: Automatically refreshes the app with code changes, speeding up the development process and debugging cycle.
Steps to Debug an Android App
- Set Breakpoints: Place breakpoints at critical parts of the code where you want to pause execution.
- Launch Debugger: Start the debugger either on an emulator or connected device.
- Inspect Values: Once the app pauses at a breakpoint, inspect the values of variables, method calls, and the call stack.
- Step Through Code: Use step-over, step-in, or step-out to navigate through the code to find issues.
- View Logs: Use the Logcat window to view real-time log messages, warnings, and errors from the device.
Tip: You can enable remote debugging for apps running on real devices by connecting via Wi-Fi, allowing you to debug apps without a USB connection.
Device/Emulator Debugging Settings
Device Type | Settings |
---|---|
Emulator | Ensure AVD (Android Virtual Device) is properly configured with the desired system image and Android version. |
Physical Device | Enable "Developer Options" and "USB Debugging" on the device. Connect it via USB or Wi-Fi for remote debugging. |
Managing Android Emulators for Testing on Different Devices
Testing Android applications on multiple devices is a crucial step to ensure compatibility and performance across various screen sizes, resolutions, and hardware configurations. Using Android emulators in Visual Studio 2022 allows developers to simulate different Android devices without needing to own each one physically. These emulators can be configured to mimic a wide range of devices, enabling efficient testing for different Android versions and configurations.
To set up and manage these emulators effectively, it’s important to understand how to create and configure virtual devices. This can be done through the Android Device Manager in Visual Studio, which offers various options for customizing device profiles, such as screen size, resolution, and Android API level. It is also essential to optimize the performance of emulators, as running multiple virtual devices simultaneously can be resource-intensive.
Creating and Managing Emulators
- Access the Android Device Manager – This tool is integrated into Visual Studio and allows you to create and manage virtual devices.
- Select Device Configuration – Choose a predefined device or create a custom one by specifying screen size, resolution, and other hardware attributes.
- Set Android API Level – Ensure that the selected API version aligns with the version you plan to target for your application.
- Performance Settings – Configure the emulator's hardware acceleration settings to optimize its performance for smooth testing.
Key Steps to Optimizing Emulator Performance
- Enable Hardware Acceleration – Make sure that the Android Emulator is using hardware acceleration (e.g., Hyper-V on Windows or HAXM on macOS) to improve speed.
- Reduce the Number of Active Emulators – Limit the number of emulators running simultaneously to conserve system resources and improve testing efficiency.
- Adjust Emulator Settings – Tweak settings like memory allocation and the number of processor cores dedicated to the emulator for better performance.
Device Compatibility Table
Device Type | Screen Size | API Level |
---|---|---|
Pixel 5 | 6.0 inches | 30 |
Galaxy S21 | 6.2 inches | 29 |
Nexus 5X | 5.2 inches | 28 |
Note: Testing on multiple devices helps identify layout issues, performance bottlenecks, and functional discrepancies across different Android versions and screen sizes.
Optimizing Build Process for Faster Android App Compiling
Efficient Android app development requires reducing build times to ensure a smoother and more productive workflow. With Visual Studio 2022, developers have several techniques available to optimize the build process, minimizing delays and improving overall performance. The build process can be time-consuming, especially for large projects, but with a few strategic adjustments, you can significantly speed things up.
Several optimization techniques can be applied to reduce compile times. Among the most effective are reducing resource usage, enabling parallel builds, and leveraging incremental compilation. These methods help streamline the process, enabling quicker iteration and more effective debugging.
Effective Strategies for Speeding Up the Build Process
- Incremental Compilation: Make use of Android's incremental build system, which only rebuilds the parts of your app that have changed, instead of recompiling everything from scratch.
- Parallel Builds: Enable parallel builds for faster execution. Visual Studio 2022 supports building multiple projects simultaneously, significantly reducing total compile time.
- Reduce Resource Usage: Minimize the number and size of resources like images, layouts, and XML files to lower the amount of data that needs to be processed during each build.
- Optimize Gradle Settings: Adjust Gradle settings such as enabling daemon and configuring build cache to improve performance during the build process.
Key Build Configuration Tips
- Enable Parallel Execution in Gradle: In the gradle.properties file, add the line org.gradle.parallel=true to allow for parallel execution of independent tasks.
- Use Build Caching: Build caching allows Gradle to avoid rebuilding unchanged parts of the project, resulting in faster builds. Enable caching by adding org.gradle.caching=true to gradle.properties.
- Optimize Dependencies: Review and minimize dependencies to prevent unnecessary processing. Remove unused or redundant libraries that add extra overhead to the build process.
"A significant improvement can be achieved by avoiding excessive dependency management during the build. Try to maintain a clean and lean project structure to benefit from faster builds."
Build Performance Metrics
Optimization Strategy | Impact on Build Time |
---|---|
Incremental Compilation | Reduces time by rebuilding only modified files |
Parallel Build | Can reduce total compile time by up to 50% |
Gradle Build Cache | Reduces rebuild times for unchanged code |
Publishing Android Apps Directly from Visual Studio
Visual Studio 2022 offers a streamlined process for developers to publish Android applications directly from the IDE. By integrating Android development tools and providing a set of features, the platform significantly simplifies the deployment process. This allows developers to avoid using separate tools or complex configurations when pushing apps to stores or devices.
The process involves configuring the app's build settings, signing, and preparing it for release, which can be easily done within the Visual Studio environment. Once the app is ready, developers can instantly deploy it to various Android stores, such as the Google Play Store, or directly to physical devices for testing purposes.
Key Steps for Publishing Android Apps
- Configure App Settings: Before publishing, ensure your app’s settings are correctly configured, including versioning, permissions, and supported screen sizes.
- Generate a Signed APK or AAB: In Visual Studio, you can easily generate a signed APK or Android App Bundle (AAB) required for release.
- Test Your Application: Make sure to run the app on different devices to verify compatibility and performance.
- Upload to Store: Once the app is packaged, it can be uploaded to Google Play or any other platform directly from Visual Studio.
"Publishing Android applications directly from Visual Studio eliminates the need for complex third-party tools and provides a seamless development-to-deployment workflow."
Essential Tools and Requirements
Tool | Description |
---|---|
Google Play Console | Required for uploading the app to the Play Store and managing its release. |
Key Store | Used for signing the APK or AAB to ensure authenticity. |
Android SDK | Necessary for compiling and building the app for Android devices. |
By following these steps, developers can easily navigate the Android app publishing process directly from within Visual Studio 2022, ensuring a smooth transition from development to production.