Android App Development Topics
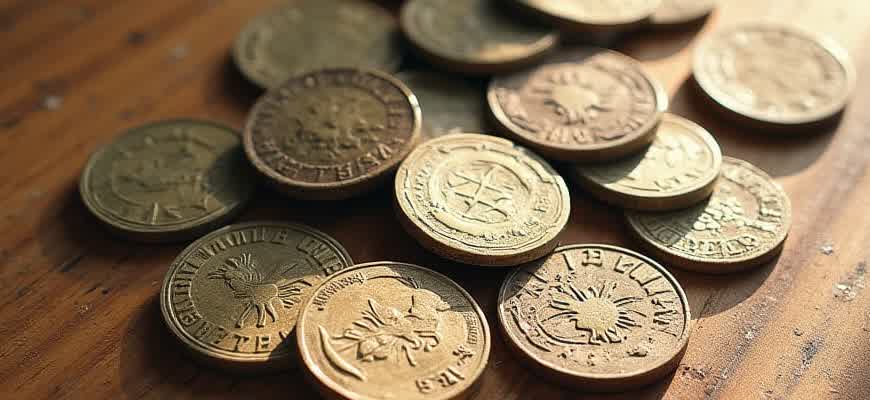
Developing an Android application involves various critical components, from designing the user interface to optimizing performance. Understanding the essential topics related to Android development is crucial for building high-quality apps.
1. User Interface Design
UI design plays a pivotal role in creating an intuitive user experience. Developers must focus on responsiveness and navigation flow to ensure users interact effortlessly with the app.
- Material Design principles
- XML layout files
- Responsive layouts for different screen sizes
2. Data Management and Storage
Effective data management is vital for Android applications. Understanding various storage options ensures seamless data handling for both online and offline scenarios.
- SharedPreferences for simple data
- SQLite for local databases
- Firebase for cloud storage
3. Performance Optimization
Performance is crucial for retaining users. Optimizing memory usage, reducing battery consumption, and minimizing load times are key considerations.
Optimization Area | Best Practices |
---|---|
Memory Usage | Use efficient data structures |
Battery Consumption | Minimize background processes |
Load Time | Asynchronous loading of data |
"Performance tuning is as important as the app's functionality in ensuring a satisfying user experience."
Choosing the Best Framework for Android App Development
When it comes to building Android apps, selecting the right framework can significantly impact development speed, code maintainability, and performance. Developers are often faced with numerous options, each with its own strengths and weaknesses. Understanding the specific needs of the app and the technical expertise of the team is key to making an informed decision. This choice will affect the long-term scalability, user experience, and integration with other tools and platforms.
There are many factors to consider when deciding on a framework, such as the app's functionality, the target audience, available resources, and the desired level of customization. Some frameworks excel in performance, while others offer rapid development with built-in features. Let's explore a few of the most popular options for Android development.
Top Frameworks for Android Development
- Flutter: Known for its speed and cross-platform capabilities, Flutter enables developers to create beautiful, high-performance apps using a single codebase for both Android and iOS.
- React Native: A widely-used JavaScript framework, React Native allows for the building of mobile applications using React. Its key advantage is its large community and reusable code between platforms.
- Jetpack Compose: A modern toolkit for native UI development in Android, Jetpack Compose streamlines UI creation with a declarative approach that reduces the need for boilerplate code.
Key Considerations When Choosing a Framework
- Performance: If high performance is a priority, native Android development with Kotlin or Java might be the best choice. Flutter and React Native offer a compromise between performance and development speed.
- Development Time: Cross-platform frameworks like Flutter and React Native tend to reduce the amount of time spent on app development, especially when targeting multiple platforms.
- Community Support: A strong community ensures regular updates, bug fixes, and access to a wide range of libraries. React Native, for instance, has a massive community behind it, which is a considerable advantage for solving issues quickly.
Keep in mind that while cross-platform frameworks offer speed and cost efficiency, they might come with limitations in terms of accessing specific platform features or performance. Always weigh the pros and cons based on the app’s requirements.
Comparison of Frameworks
Framework | Platform Support | Performance | Development Speed |
---|---|---|---|
Flutter | iOS, Android | High | Fast |
React Native | iOS, Android | Moderate | Fast |
Jetpack Compose | Android | High | Moderate |
Optimizing Performance: Managing Memory and CPU in Android Applications
Effective memory and CPU management is crucial for ensuring smooth performance in Android apps. Optimizing these resources not only improves responsiveness but also contributes to battery efficiency and user satisfaction. By identifying and addressing issues like memory leaks, inefficient CPU usage, and excessive background tasks, developers can significantly enhance app performance.
In Android app development, proper memory management includes handling object allocation, garbage collection, and reducing memory consumption. Similarly, CPU management involves optimizing threads, background services, and resource-heavy tasks that can cause lags or high battery usage. Below are key strategies to achieve these optimizations:
Memory Optimization Techniques
- Avoiding Memory Leaks: Ensure objects are properly dereferenced and are eligible for garbage collection when no longer in use.
- Using Efficient Data Structures: Opt for memory-efficient collections like SparseArray instead of HashMap.
- Bitmap Optimization: Scale bitmaps down to an appropriate size before displaying them to reduce memory usage.
CPU Management Strategies
- Thread Management: Utilize background threads for non-UI tasks and avoid blocking the main UI thread, which can cause unresponsiveness.
- Minimizing Background Processes: Limit the number of background services running at once, and use WorkManager for deferrable background tasks.
- Efficient Network Requests: Batch network calls to reduce the overhead of frequent requests.
Memory leaks and excessive CPU usage not only degrade performance but also lead to increased battery consumption and poor user experience.
Tools for Monitoring
Tool | Purpose |
---|---|
Android Profiler | Real-time monitoring of memory, CPU, and network usage. |
LeakCanary | Detects and helps fix memory leaks. |
Systrace | Tracks CPU usage and helps identify performance bottlenecks. |
Best Practices for API Integration in Android Applications
Integrating third-party APIs into Android applications is crucial for adding functionality such as accessing remote data, authentication, and much more. Proper handling of these connections ensures smooth user experiences and effective data exchange. However, mismanaged API integrations can lead to performance issues, errors, and security vulnerabilities. In this guide, we’ll explore key strategies to help developers build robust, efficient, and secure API connections in Android apps.
When working with APIs, developers must follow a set of best practices to ensure data is fetched efficiently and securely. These practices involve choosing the right libraries, managing network requests, handling responses, and maintaining security protocols throughout the process.
Efficient Data Fetching and Network Requests
When designing an API integration, it’s essential to optimize the network requests and handle responses in an efficient manner. This includes choosing a library for networking, like Retrofit or OkHttp, which can simplify handling HTTP requests. Additionally, asynchronous operations should be prioritized to avoid blocking the main UI thread.
- Use Retrofit for simplicity: It simplifies the process of sending HTTP requests and parsing JSON responses.
- Use OkHttp for custom requests: OkHttp gives more control over low-level request configurations like timeouts, interceptors, etc.
- Implement async tasks: Asynchronous requests prevent UI freezes by handling network tasks off the main thread.
Remember: Never perform network operations on the main UI thread. Always use background threads or async frameworks to keep the user interface responsive.
Managing API Responses and Error Handling
Handling responses efficiently is just as important as sending requests. Properly parsing and managing errors will ensure that your app reacts gracefully to API failures, timeouts, and other issues.
- Handle different HTTP response codes: For instance, 404 errors indicate missing resources, while 500 errors represent server-side issues.
- Implement proper error logging: Use a logging framework such as Timber for debugging network calls.
- Use try-catch blocks: Proper error handling ensures that unexpected issues don’t crash the app.
Security Considerations for API Integration
Ensuring the security of your API integrations is essential for protecting user data and app functionality. This involves using encryption and authentication techniques, like OAuth, and handling sensitive data appropriately.
Security Measure | Description |
---|---|
HTTPS | Always use HTTPS to secure the communication between the app and the API server. |
OAuth | Use OAuth for safe user authentication and authorization to external services. |
Data Encryption | Encrypt sensitive data both in transit and at rest to protect user privacy. |
Managing User Data Securely: Android Storage Options and Techniques
When developing Android applications, handling sensitive user data requires careful attention to security. The Android operating system provides several storage options, each with its own use cases and security considerations. Understanding these options and how to implement them securely is crucial for maintaining user privacy and data integrity.
Secure data storage can be achieved by using Android's native storage solutions, such as SharedPreferences, internal storage, external storage, and databases, while also employing encryption and access control mechanisms to safeguard sensitive information.
Android Storage Solutions
Android provides several options for saving data locally on a device, with each option offering a different level of security:
- SharedPreferences: Ideal for storing small pieces of data such as user preferences or settings. It is essential to encrypt sensitive data stored here to prevent unauthorized access.
- Internal Storage: Data stored in internal storage is private to the application and is inaccessible to other apps. It is a secure option for storing files that should not be shared with other applications.
- External Storage: While external storage is useful for saving large files like media, it is less secure, as it is accessible by other apps and can be easily compromised. Encryption is strongly recommended for sensitive data.
- SQLite Databases: Used for structured data, such as records and tables. SQLite offers more complex querying capabilities and can be secured using encryption techniques.
Techniques for Securing Data
Once you've selected a storage solution, securing the data becomes the next priority. Some best practices for data security include:
- Encryption: Encrypt sensitive data both in transit and at rest. Android offers the Android Keystore system, which securely stores cryptographic keys.
- Access Control: Use permissions to restrict access to certain storage areas. For example, private files should only be accessible by the app that created them.
- Secure Backup: Implement a backup strategy that ensures data is encrypted during the backup process and protected when restored.
It is important to avoid storing sensitive information like passwords and payment details in plain text. Always use encryption and key management practices to safeguard user data.
Comparison of Storage Methods
Storage Option | Security Level | Use Case |
---|---|---|
SharedPreferences | Low (if unencrypted) | Small data like preferences |
Internal Storage | High | Private files specific to the app |
External Storage | Low (unless encrypted) | Large files like media |
SQLite Databases | High (with encryption) | Structured data with complex queries |
Creating Custom UI Components in Android Apps
Android development often requires building custom UI components to create unique user experiences. While standard UI elements are suitable for most use cases, custom components offer greater flexibility in terms of design, functionality, and user interaction. In this guide, we will discuss how to create and implement your own custom views and layouts for Android applications.
When implementing custom UI components, developers typically extend the View or ViewGroup classes to create unique elements. This process involves overriding key methods like onDraw()
for custom drawing and onLayout()
for handling layout positioning. Additionally, attributes can be customized through XML for easy usage within layouts.
Steps to Implement Custom Views
- Create a new class: Start by extending
View
orViewGroup
depending on whether you need a single view or a collection of views. - Override necessary methods: Implement
onDraw()
for drawing andonLayout()
for managing layout. - Define custom attributes: Use XML attributes to provide flexibility in customization and make it easier to use the component in layouts.
Example of a Custom View
Here's an example of a custom TextView that draws text in a custom font:
public class CustomTextView extends TextView {
public CustomTextView(Context context, AttributeSet attrs) {
super(context, attrs);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
// Custom drawing logic here
}
}
Customizing UI Components with XML
Incorporating custom UI components in Android layouts is straightforward with XML. You can define custom attributes and set properties directly in the layout file. For example:
Key Considerations
Aspect | Description |
---|---|
Performance | Custom views can be computationally expensive if not optimized properly. Avoid excessive drawing operations and complex layouts. |
Accessibility | Ensure your custom views are accessible to users with disabilities by providing appropriate content descriptions and touch feedback. |
Custom UI components enhance the user experience by offering tailored interactions and visual designs. However, developers must ensure that these components maintain performance and accessibility standards.
Managing Background Operations: Services and WorkManager in Android
In Android development, performing tasks in the background is crucial to improve app performance and responsiveness. Background tasks are typically long-running operations that need to continue even when the user is not directly interacting with the app. Handling these tasks efficiently is essential for building reliable and smooth experiences. Android provides different mechanisms to manage background processes, primarily through Services and WorkManager.
Services are one of the fundamental components used to run background tasks. However, for tasks that require more complex scheduling and management, the WorkManager API is preferred due to its ability to handle tasks that need to be deferred or executed periodically, even across app restarts. Understanding when and how to use each is key for optimizing background processing in Android apps.
Using Services for Background Work
Services are used for tasks that need to run continuously in the background. They can be either started by the app or bound to it. There are two main types of Services in Android:
- Started Service: Runs in the background once it is started and continues until the task is finished or the service is stopped.
- Bound Service: Allows components (e.g., Activities) to bind to it for communication during its lifetime.
When using Services, it's important to handle lifecycle management properly, especially since the system may stop them to free up resources. For tasks that need to persist even after the app is closed, you should consider using foreground services, which are less likely to be killed by the system.
Important: Always ensure that long-running background tasks are handled appropriately to prevent battery drain and memory issues.
WorkManager for Task Scheduling
WorkManager is a robust API designed for managing deferred, scheduled, and periodic background tasks. It is suitable for tasks like uploading data, performing database cleanup, or syncing content. WorkManager ensures that tasks are completed even if the app is terminated or the device is restarted.
Here are some key features of WorkManager:
- Guaranteed execution: Ensures tasks are completed even if the app is killed or the device is rebooted.
- Flexible scheduling: Supports constraints such as network availability, charging status, and device idle state.
- Support for periodic work: Enables periodic tasks such as syncing data every few hours.
When deciding between Services and WorkManager, consider the nature of the task and its scheduling needs. WorkManager is generally preferred for tasks that don't require immediate execution but need to be reliable and scheduled with specific conditions.
Comparison Between Services and WorkManager
Feature | Service | WorkManager |
---|---|---|
Execution Reliability | May be killed by the system | Ensures guaranteed execution |
Task Scheduling | Manual management | Automatic, with support for constraints |
Background Task Type | Continuous or one-time | Deferred, periodic, and scheduled |
Improving User Control through Android's Permission System
Android's permissions system plays a critical role in balancing user privacy and app functionality. It allows users to control the access of apps to sensitive data and device features such as location, camera, and microphone. However, improper handling of permissions can result in poor user experience or even security risks. Understanding how to manage these permissions effectively is essential for developers aiming to provide better user control while maintaining app functionality.
In recent Android versions, permissions are categorized into two primary groups: normal and dangerous. Normal permissions are granted automatically without user interaction, while dangerous permissions require explicit user approval. Developers need to adopt best practices for requesting, handling, and explaining permissions in a way that fosters trust and transparency with users.
Key Strategies for Managing Permissions
- Request Permissions at the Right Time: Ask for permissions only when necessary, and avoid requesting them during the app's startup. This avoids overwhelming the user.
- Provide Clear Explanations: Always explain why a specific permission is needed. Use simple language to ensure users understand how granting the permission enhances their experience.
- Respect User Decisions: If a user denies a permission request, don't continuously ask for it. Instead, provide alternatives or gracefully degrade features that rely on that permission.
Remember, asking for too many permissions or irrelevant ones can lead to user distrust, which can result in lower app ratings or uninstalls.
Handling Permissions Based on App Requirements
Depending on the functionality of the app, developers may need to request specific permissions. Here's a breakdown of common permissions and how to handle them:
Permission | Usage | Request Type |
---|---|---|
ACCESS_FINE_LOCATION | Used for GPS-based services | Dangerous |
CAMERA | Used for capturing photos or videos | Dangerous |
READ_EXTERNAL_STORAGE | Used for accessing external storage | Dangerous |
INTERNET | Used for network access | Normal |
Always tailor your app's permission requests to the needs of your users, and make sure to handle them in a transparent and user-friendly manner.