Multi Platform App Builder
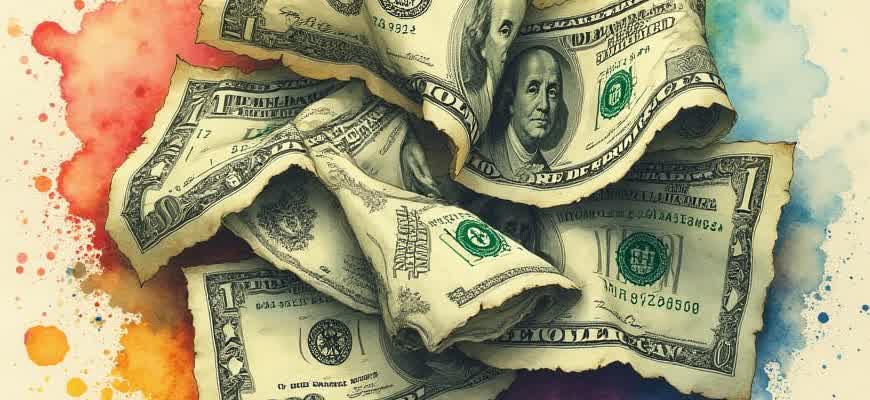
The demand for cross-platform mobile applications has surged in recent years. This approach allows developers to create a single codebase that runs seamlessly across various operating systems, such as iOS, Android, and even the web. Such tools enable businesses to maximize reach without having to manage multiple codebases or invest in platform-specific development.
One of the key advantages of using cross-platform development tools is the ability to:
- Reduce development time and cost
- Ensure consistency across platforms
- Reach a wider audience with minimal effort
Key takeaway: By utilizing cross-platform frameworks, companies can maintain a unified user experience across all devices while optimizing development resources.
Different solutions provide various features and capabilities. Here's a comparison of some of the most popular frameworks:
Framework | Supported Platforms | Language | Performance |
---|---|---|---|
React Native | iOS, Android | JavaScript | High |
Flutter | iOS, Android, Web | Dart | Very High |
Xamarin | iOS, Android, Windows | C# | High |
Ensuring Compatibility and Consistent User Experience Across Platforms
When developing applications for multiple platforms, one of the key challenges is ensuring that the app works seamlessly across different environments while providing a consistent user experience. The variability in operating systems, screen sizes, and hardware configurations means that developers must adopt strategies to address these differences effectively.
Achieving cross-platform compatibility requires a deep understanding of the unique capabilities and limitations of each platform. This includes considering the user interface (UI) design, performance optimization, and the way each platform handles device features like GPS, camera, or sensors. By focusing on these areas, developers can create a cohesive experience that feels native to each platform.
Key Strategies for Ensuring Cross-Platform Functionality
- Code Reusability: Use shared codebases and frameworks that support multiple platforms, such as React Native or Flutter. This reduces development time and ensures consistency across platforms.
- Responsive UI Design: Implement flexible layouts that adapt to different screen sizes and resolutions. Use relative units (like percentages) instead of fixed ones (like pixels) to ensure the UI scales correctly.
- Platform-Specific Customization: Customize the UI and features where necessary to match the conventions and guidelines of each platform while maintaining core functionality.
Testing Across Multiple Environments
Proper testing is crucial for identifying potential issues early in development. Conducting tests on real devices, simulators, and emulators is essential to ensuring your app performs well across platforms.
- Test the app on various devices with different screen sizes and hardware capabilities.
- Use automated testing tools to run tests on multiple platforms simultaneously.
- Perform manual testing to assess the user experience on each platform individually.
Important: Testing should go beyond functional performance; ensure that the design remains intuitive and consistent across platforms, adhering to user expectations specific to each operating system.
Platform-Specific Features and Integration
While maintaining cross-platform consistency, it is also essential to integrate platform-specific features that enhance the overall experience. Features like push notifications, local storage, and device-specific hardware access (such as fingerprint scanning or camera functionality) should be incorporated seamlessly, utilizing platform-specific APIs when necessary.
Platform | Key Feature | Integration Strategy |
---|---|---|
iOS | Touch ID/Face ID | Use the iOS Keychain and integrate with native authentication libraries. |
Android | Fingerprint Scanner | Leverage Android’s FingerprintManager API to authenticate users securely. |
Web | Push Notifications | Utilize browser push notification services (e.g., Firebase) for web users. |
Maximizing Performance and Speed in Your Multi Platform App
In the development of cross-platform applications, optimizing both performance and speed is crucial for delivering a seamless user experience across different devices. Developers must ensure that their applications run smoothly on various platforms while maintaining minimal load times and responsiveness. This requires strategic planning, a deep understanding of platform-specific constraints, and the application of performance-enhancing techniques.
Achieving optimal performance involves a combination of code optimization, resource management, and ensuring that platform-specific APIs are used efficiently. In this context, selecting the right framework and applying performance best practices is essential to meet user expectations and achieve faster app load times on both mobile and desktop devices.
Key Approaches to Enhance App Speed
- Code Minimization: Reduce the size of your app's code by removing unused libraries and functions. Less code means fewer resources consumed and faster loading.
- Efficient Memory Management: Allocate memory efficiently and release unused resources promptly to avoid memory leaks that slow down app performance.
- Compression: Compress images, assets, and other data to reduce loading times, especially for mobile apps with limited bandwidth.
Platform-Specific Optimizations
- Mobile Platforms: Leverage platform-specific tools like Android's Proguard and iOS's LLVM optimizer to minify code and reduce runtime overhead.
- Desktop Platforms: Utilize native code optimizations and ensure that your app is leveraging the hardware acceleration capabilities of the platform.
- Cross-Platform Tools: If using a framework like Flutter or React Native, ensure you are familiar with their performance tips, such as using native modules and minimizing re-renders.
“Optimizing performance isn’t just about making the app faster; it’s about creating a smooth, efficient user experience that feels intuitive and responsive on every platform.”
Best Practices for Performance Monitoring
To ensure the app maintains its speed across all platforms, it is crucial to continuously monitor its performance. Some best practices include:
Practice | Description |
---|---|
Use Profiling Tools | Utilize platform-specific profiling tools like Xcode Instruments or Android Profiler to analyze memory usage and CPU performance. |
Measure Load Time | Implement loading time monitoring to track delays and identify potential bottlenecks during startup. |
Test on Real Devices | Test on a variety of physical devices to get a real-world sense of app performance under different network conditions and hardware capabilities. |
Integrating Third-Party Tools and APIs in a Multi-Platform Environment
Integrating external services and APIs in a multi-platform application requires careful consideration of platform-specific constraints and compatibility. Modern frameworks offer native support for connecting to various third-party tools, but developers must ensure that the integration is seamless across all devices and platforms. The complexity of dealing with different operating systems (iOS, Android, Web, etc.) can introduce challenges such as inconsistent API responses or platform-specific authentication methods.
Successful integration starts with choosing the right API and ensuring it supports the desired platforms. Common third-party integrations include payment gateways, social media login systems, mapping services, and analytics tools. These services often provide SDKs or client libraries tailored for different environments, which can simplify the integration process. However, developers must consider the potential impact on performance, security, and maintenance when incorporating these tools into their applications.
Steps for Effective Integration
- Choose compatible APIs: Ensure that the selected API provides SDKs or wrappers for all targeted platforms.
- Use platform-specific adapters: Implement abstraction layers to handle any platform-specific quirks or variations in the API responses.
- Test thoroughly: Test on all target platforms to ensure that data flows smoothly and that the application maintains consistent performance.
Key Considerations
- Authentication and Authorization: Different platforms may have unique authentication mechanisms, such as OAuth on mobile devices or API keys for web apps.
- Data synchronization: Ensure that data fetched from third-party services is synchronized correctly across platforms, taking into account caching, offline access, and consistency issues.
- API Rate Limits: Be aware of the rate limits imposed by third-party services and implement strategies such as exponential backoff or request throttling to avoid service disruptions.
Remember to monitor the performance and reliability of integrated services over time, as third-party APIs may evolve, resulting in deprecated features or unexpected changes.
Example API Integration: Payment Gateway
Platform | SDK/Library | Authentication Method |
---|---|---|
iOS | Stripe SDK | OAuth 2.0 |
Android | Stripe Android SDK | OAuth 2.0 |
Web | Stripe JavaScript Library | API Key |
How to Scale and Maintain Your App Built with a Multi-Platform Tool
Building a multi-platform application offers a streamlined approach to reach diverse user bases across various operating systems. However, as your app grows, it becomes crucial to address performance, scalability, and long-term maintenance. The flexibility of multi-platform tools allows for rapid development, but it’s essential to ensure that the app remains performant and easy to maintain as the user base and feature set expand.
Scaling and maintaining such applications requires a structured approach that focuses on optimizing code, managing dependencies, and leveraging platform-specific features. Understanding the balance between cross-platform functionality and platform-specific optimizations is key to avoiding common pitfalls as the project grows.
1. Optimize Code and Resources
Efficient codebase management plays a central role in both scalability and maintainability. Here are a few essential practices:
- Modularization: Break down your app into smaller, reusable components to improve code manageability.
- Efficient Resource Management: Reduce image sizes, minify JavaScript, and optimize assets to prevent performance bottlenecks.
- Memory Management: Pay attention to memory leaks and ensure the app frees up resources correctly, especially on mobile devices.
2. Leverage Platform-Specific Features
While multi-platform tools provide a unified codebase, some features are better optimized or only available natively on specific platforms. By utilizing platform-specific APIs, you can boost performance.
- For Android, use native tools like Jetpack for better UI and background task management.
- For iOS, leverage SwiftUI to get the most out of the system’s native user interface components.
- For desktop apps, consider utilizing the platform’s own UI frameworks (like Electron for cross-platform desktop apps).
3. Regular Maintenance and Updates
Continuous updates and maintenance are critical to keeping the app functional and user-friendly. Here's how to manage that:
Always track bug reports and performance issues through monitoring tools and prioritize updates based on user feedback.
Maintenance Task | Frequency | Tools |
---|---|---|
Bug Fixing | Weekly | Jira, Bugzilla |
Code Review | Bi-weekly | GitHub, Bitbucket |
Feature Updates | Monthly | CI/CD, GitLab |