App Vue Template
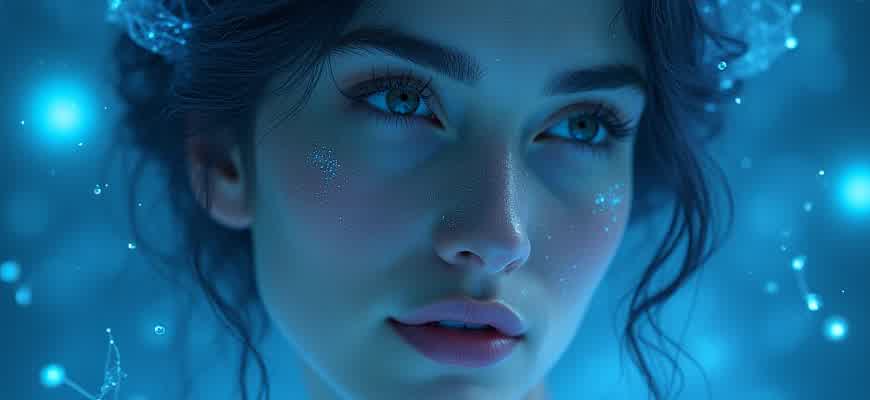
The Vue.js framework provides a flexible and intuitive way to build user interfaces by using templates. These templates serve as a blueprint, combining HTML and Vue-specific syntax to create dynamic, reactive web applications.
In a typical Vue.js template, you will find various sections for structuring components, which include:
- Template Section: Contains the HTML structure of the component.
- Script Section: Holds the logic and data for the component.
- Style Section: Defines the component’s CSS styles.
"A well-organized template improves maintainability and scalability of the application."
Below is an example illustrating how these sections interact within a basic Vue.js template structure:
- Define a template: The HTML markup that forms the UI.
- Add a script: This is where the logic, such as data binding and methods, is placed.
- Apply styles: Use scoped styles to ensure that the component’s appearance is encapsulated.
Section | Description |
---|---|
Template | Defines the HTML structure and uses Vue directives for dynamic behavior. |
Script | Holds component logic, data, and methods. |
Style | Defines styles scoped to the component to prevent conflicts. |
Why Opt for a Vue.js Template for Your Next Project
When starting a new web application, time and efficiency are critical factors in ensuring the project's success. Vue.js templates offer a pre-configured structure that helps developers focus on creating the core features instead of building the foundation from scratch. These templates often come with optimized components, tools, and features tailored to specific use cases, such as dashboards or e-commerce platforms.
Moreover, Vue.js templates follow best practices and allow seamless integration with other tools and libraries. This reduces development time and increases the likelihood of building a scalable and maintainable application. Below are the main reasons why choosing a Vue.js template can be a smart decision for your next project.
Key Benefits of Using a Vue.js Template
- Pre-Built Structure: Templates come with an organized file structure that enables faster project setup, minimizing the need for initial configuration.
- Reusable Components: Many templates include ready-made components that can be reused across multiple parts of your app, saving time on design and functionality.
- Responsive Design: Most Vue.js templates are designed to be mobile-first, ensuring your app looks good on any device.
- Customizable: Templates provide flexibility for developers to customize the look and feel of the app to meet specific requirements.
"Using a Vue.js template accelerates development while ensuring the app is built on a solid, maintainable foundation."
Considerations When Choosing a Template
- Compatibility: Ensure the template supports the latest version of Vue.js and is compatible with other technologies you plan to use.
- Community Support: Templates with active community support often have regular updates and bug fixes, providing a more stable foundation.
- Performance: Check if the template is optimized for performance, as slow loading times can affect user experience and SEO.
Template Comparison Table
Template Name | Features | Price |
---|---|---|
Vue Paper Dashboard | Customizable components, Material Design, fully responsive | Free |
Vuexy | Multiple layouts, RTL support, dark mode | Paid |
Vue Admin | Pre-built dashboard, multi-language support | Free |
Customizing Your Vue Template: A Step-by-Step Guide
Vue.js templates are a powerful tool for building dynamic user interfaces. They allow developers to easily structure and manage application layouts. Customizing these templates to fit your specific project requirements is essential for creating a streamlined and efficient user experience. In this guide, we will walk through key steps to personalize a Vue template to your needs.
Whether you are starting from a base template or working on a more complex project, understanding how to adjust the template structure is crucial. From modifying layouts to adding custom components, this guide provides a clear roadmap to help you get started.
Understanding the Template Structure
A Vue template is essentially the view layer of your application, where HTML and Vue-specific syntax meet. To begin customizing, it’s important to understand the basic elements that make up a Vue template:
- Data Binding: The template connects with Vue’s reactive data system through interpolation (e.g., {{ message }}) and directive-based binding (e.g., v-bind, v-model).
- Directives: These are special attributes prefixed with "v-" (e.g., v-for, v-if) that provide additional functionality to elements in the template.
- Components: Vue allows the creation of reusable components, which can be customized and nested within your template.
Step-by-Step Customization Process
To effectively customize your Vue template, follow this structured approach:
- Step 1: Define Your Layout - Start by organizing your layout structure. Consider using Vue’s
router-view
for dynamic page rendering. - Step 2: Modify Template Tags - Update the default HTML tags in the template to fit your design needs. For example, change default divs into custom components or adjust classes and IDs.
- Step 3: Integrate Vue Directives - Enhance interactivity by using directives such as
v-show
for conditional rendering orv-for
for list rendering. - Step 4: Add Custom Components - Break down your layout into smaller, reusable Vue components to keep your code clean and maintainable.
Important Tips for Customization
Remember to keep your templates modular and reusable. This will not only make your code cleaner but also improve the scalability of your application as it grows.
Useful Resources
For further assistance, consider exploring Vue's official documentation and community-driven tutorials. Here's a helpful table of resources:
Resource | Link |
---|---|
Vue Documentation | vuejs.org |
Vue Component Guide | Components Guide |
Optimizing Your Application's Performance with Vue Templates
When developing applications using Vue, it's crucial to ensure that the template rendering process remains efficient to maintain smooth performance. Vue templates are compiled into virtual DOM trees that help manage the DOM structure more effectively. However, improper handling of these templates can lead to unnecessary re-renders, slow load times, and overall performance degradation. Optimizing the template structure and the way data is handled is essential to creating responsive, fast-loading applications.
Vue provides a set of tools and best practices for streamlining template rendering, and understanding how to use them effectively can make a significant difference. Leveraging computed properties, avoiding unnecessary watchers, and minimizing the use of dynamic bindings can greatly enhance the efficiency of your Vue application. Below are key strategies for optimizing Vue templates.
Key Optimization Strategies
- Use of Computed Properties: These are cached based on their dependencies, so they are recalculated only when necessary, reducing redundant computations.
- Limit Re-renders: Preventing unnecessary re-renders by using keys on elements and ensuring that only the parts of the UI that need to change are updated.
- Lazy Loading of Components: Implement lazy loading for components that are not required immediately, reducing the initial load time.
- Minimize the Use of v-for: Avoid using v-for with large datasets directly in templates. Instead, use pagination or virtual scrolling for better performance.
"Efficient template design is the foundation for a fast and scalable Vue application. Prioritize performance from the start to avoid costly re-renders and unnecessary computations."
Template Optimization Best Practices
- Ensure that bindings are minimal and only used when necessary. Excessive binding can lead to performance issues.
- Use functional components for simple, stateless templates. These have a smaller memory footprint and faster render times.
- Implement proper key management in lists to prevent Vue from unnecessarily re-rendering entire lists when only a few elements have changed.
Performance Comparison Table
Strategy | Impact |
---|---|
Lazy Loading Components | Improves initial load time by loading only necessary components. |
Use of Computed Properties | Reduces unnecessary recalculations, ensuring better responsiveness. |
Minimize v-for on Large Lists | Prevents excessive rendering when dealing with large datasets. |
Best Approaches for Mobile-Responsive Layouts in Vue.js Templates
Designing responsive layouts in Vue.js templates requires attention to both performance and flexibility, particularly when it comes to mobile devices. Modern Vue.js applications need to ensure that the user experience is seamless across various screen sizes and resolutions. Responsive design techniques in Vue.js templates typically involve utilizing media queries, flexible grid layouts, and appropriate element sizes to maintain readability and accessibility.
In this context, optimizing templates for mobile responsiveness means thinking about how elements behave on smaller screens. It’s essential to choose the right layout strategies and make sure the design adapts fluidly without compromising the app's performance.
Key Practices for Creating Responsive Vue.js Templates
- Use Flexbox and Grid Layouts: Both Flexbox and CSS Grid allow for flexible layouts that can adapt based on screen size. Vue.js allows you to manage layout changes dynamically through its reactive system.
- Apply Media Queries: Media queries are a fundamental tool for tailoring the layout to different screen sizes. By defining breakpoints for mobile, tablet, and desktop views, you can adjust font sizes, padding, and layout structures accordingly.
- Leverage Vue.js Directives for Conditional Rendering: Use
v-if
andv-show
to conditionally render components based on the screen size or device. This helps in optimizing mobile views by hiding or showing specific elements only when necessary.
"Optimizing for mobile isn't just about shrinking the design – it's about understanding how elements interact on smaller screens and adjusting accordingly."
Best Tools for Mobile Responsiveness in Vue.js
- Vue Media Queries: Utilize plugins or custom directives like
v-media-query
for more efficient media query management inside Vue components. - Vue Responsive Images: Implement the
srcset
attribute for responsive image loading, which ensures that images are loaded appropriately based on screen size and resolution. - Mobile-First Approach: Begin with mobile layouts as the default, then scale up for larger screens. This approach helps keep mobile performance optimal by prioritizing load times and reducing unnecessary content on smaller devices.
Example of a Mobile-Responsive Table
Device | Breakpoints | Layout Type |
---|---|---|
Mobile | max-width: 600px | Single-column layout |
Tablet | min-width: 601px and max-width: 1024px | Two-column layout |
Desktop | min-width: 1025px | Multi-column layout |
Common Issues in Vue Templates and How to Address Them
Vue templates provide a convenient way to define the structure of your UI, but developers can often encounter pitfalls when using them. These issues can range from performance problems to subtle bugs that are hard to track down. Being aware of these potential mistakes and knowing how to avoid them is crucial for maintaining clean, efficient, and maintainable code.
By understanding some common challenges in Vue templates, you can improve both the speed of development and the quality of your application. Let's explore a few common issues and the strategies to prevent them.
1. Overusing Inline Functions
One of the most common mistakes when working with Vue templates is the excessive use of inline functions within template expressions. While it might seem convenient, it leads to performance issues because Vue re-evaluates these functions on every re-render, even if the data hasn't changed.
Tip: Instead of defining functions inline, use methods or computed properties in your Vue component's script section.
- Inline functions are recalculated on every re-render.
- Methods and computed properties allow for better performance and reusability.
- Avoid using functions like
@click="increment()"
directly in the template. Instead, create a methodincrement()
in the script section.
2. Not Using Keys in Loops
When rendering lists using v-for
, it’s essential to use the :key
directive to uniquely identify each element in the list. Failing to do so can result in issues like incorrect re-rendering or slow updates, especially when the list is dynamically updated.
Tip: Always provide a unique key for elements in a list to help Vue track each item’s identity.
- Unique keys help Vue optimize list updates and prevent unnecessary DOM manipulations.
- Without keys, Vue may struggle to properly handle state when elements are added, removed, or reordered.
- Keys can be simple data attributes, like IDs, or generated using a combination of properties.
3. Complex Template Logic
Embedding too much logic directly in templates can lead to poor readability and maintainability. It also increases the risk of errors, especially when working with complex conditional statements or loops.
Tip: Move complex logic into computed properties or methods to keep templates clean and easy to understand.
Problem | Solution |
---|---|
Heavy logic in templates | Use methods and computed properties in the script section to handle the logic. |
Long conditionals in templates | Break them down into simpler expressions or delegate them to the component’s methods. |
Maintaining and Updating Your Vue Template-Based Application
As your application evolves, keeping it up to date is crucial for ensuring performance, security, and compatibility with new features. Regular updates and proper maintenance help prevent issues like deprecated features, security vulnerabilities, and slow performance. By adhering to a systematic approach, you can ensure smooth updates without disrupting the user experience.
To successfully manage updates for your Vue template-based application, follow a series of best practices that will minimize the risk of introducing bugs and other unforeseen issues. Staying organized and proactive in maintaining your app is essential for long-term stability and growth.
Key Steps for Efficient Maintenance
- Regularly Update Dependencies: Ensure that all dependencies, including Vue itself and related libraries, are updated periodically. Use tools like npm or yarn to check for outdated packages and update them accordingly.
- Perform Code Refactoring: Over time, refactor your code to maintain readability and improve performance. Removing unused code and optimizing the structure can help maintain scalability and improve debugging.
- Monitor Compatibility: Keep track of breaking changes introduced in new versions of Vue and related packages. Regularly check release notes to ensure compatibility with your app’s components.
Tips for a Smooth Update Process
- Start with minor updates first, ensuring that you address smaller fixes before applying major changes.
- Use version control systems like Git to track changes and roll back updates if necessary.
- Always test your app thoroughly in a development environment before pushing updates to production.
Important: Before upgrading to a new version of Vue or other core libraries, thoroughly review the migration guide to avoid breaking changes in your application.
Tracking Performance and Security
Maintaining the performance and security of your application is just as important as keeping it up to date. Consider the following tools and techniques for ongoing monitoring:
Tool/Technique | Description |
---|---|
Vue Devtools | Use Vue Devtools to monitor component behavior and performance issues in real-time. |
Security Audits | Run security audits with tools like npm audit or Snyk to identify vulnerabilities in your dependencies. |
Code Linters | Integrate linters like ESLint to ensure consistent and error-free code as you update your application. |